OpenCV Native 开发环境搭建步骤请参考:
OpenCV Native开发环境搭建_tugouxp的专栏-CSDN博客
YOLOV3网络吃图格式为416*416 NCWH 三通道的RGB格式图,下面的程序可以将一张JPEG图像转换为对应的格式,基于OPENCV
#include <opencv2/opencv.hpp>
#include <iostream>
#include <vector>
#include <unistd.h>
using namespace std;
using namespace cv;
int main(int argc, char **argv)
{
Mat img = imread("output_416x416.jpg");
if(img.empty())
{
cout <<"please confirm the name of pic is right!" <<endl;
return -1;
}
Mat imgs0, imgs1, imgs2;
Mat imgv0, imgv1, imgv2;
Mat result0, result1, result2;
Mat imgs[3];
split(img, imgs);
imgs0 = imgs[0];
imgs1 = imgs[1];
imgs2 = imgs[2];
printf("rows %d, cols %d, channel %d, type %d.\n", img.rows, img.cols, img.channels(), img.type());
imshow("RGB-B", imgs0);
imshow("RGB-G", imgs1);
imshow("RGB-R", imgs2);
printf("rows %d, cols %d, channel %d, type %d.\n", imgs0.rows, imgs0.cols, imgs0.channels(), imgs0.type());
printf("rows %d, cols %d, channel %d, type %d.\n", imgs1.rows, imgs1.cols, imgs1.channels(), imgs1.type());
printf("rows %d, cols %d, channel %d, type %d.\n", imgs2.rows, imgs2.cols, imgs2.channels(), imgs2.type());
Mat zero = cv::Mat::zeros(img.rows, img.cols, CV_8UC1);
imgs[0] = zero;
imgs[2] = zero;
merge(imgs, 3, result1);
imshow("result1", result1);
FILE *file = fopen("network_input.bin", "wb+");
if(file == NULL)
{
cout << "fatal error,create file failure" << endl;
exit(-1);
}
cv::MatIterator_<uchar> it0 = imgs0.begin<uchar>();
cv::MatIterator_<uchar> it0_end = imgs0.end<uchar>();
for (int i = 0; it0 != it0_end; it0 ++, i ++)
{
unsigned char data = *it0;
int count = fwrite(&data, 1, 1, file);
if(count != 1)
{
cout << "write binary failure." << endl;
}
}
cv::MatIterator_<uchar> it1 = imgs1.begin<uchar>();
cv::MatIterator_<uchar> it1_end = imgs1.end<uchar>();
for (int i = 0; it1 != it1_end; it1 ++, i ++)
{
unsigned char data = *it1;
int count = fwrite(&data, 1, 1, file);
if(count != 1)
{
cout << "write binary failure." << endl;
}
}
cv::MatIterator_<uchar> it2 = imgs2.begin<uchar>();
cv::MatIterator_<uchar> it2_end = imgs2.end<uchar>();
for (int i = 0; it2 != it2_end; it2 ++, i ++)
{
unsigned char data = *it2;
int count = fwrite(&data, 1, 1, file);
if(count != 1)
{
cout << "write binary failure." << endl;
}
}
fsync(fileno(file));
fflush(file);
fclose(file);
waitKey(0);
return 0;
}
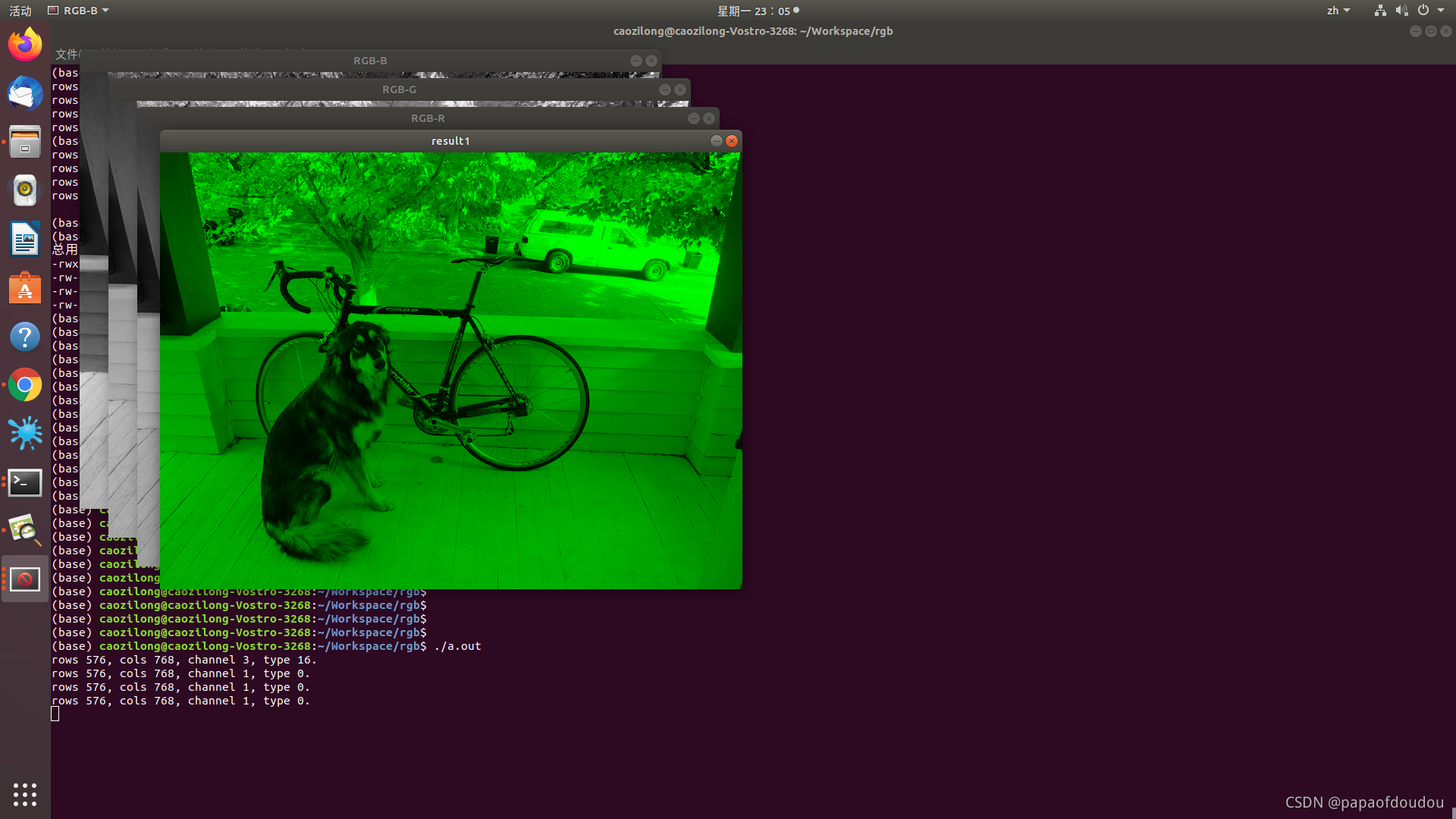

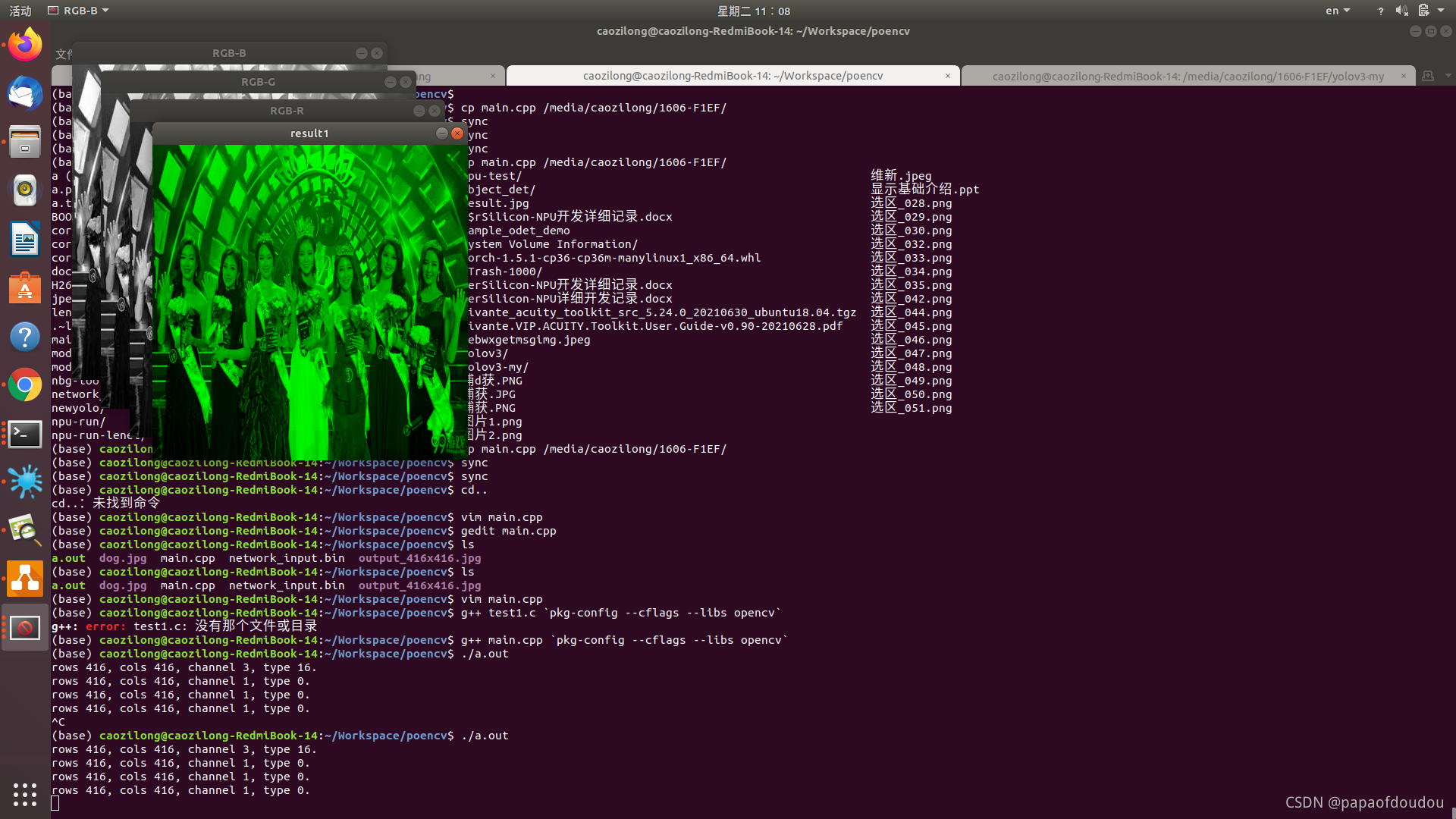
加上scale操作
scale 为416*416大小
#include <opencv2/opencv.hpp>
#include <iostream>
#include <vector>
#include <unistd.h>
using namespace std;
using namespace cv;
int main(int argc, char **argv)
{
Mat img = imread("dog.jpg");
if(img.empty())
{
cout <<"please confirm the name of pic is right!" <<endl;
return -1;
}
Size dsize = Size(416, 416);
Mat img2 = Mat(dsize, 16);
resize(img, img2, dsize);
//printf("%s line %d, img2.type = %d.\n", __func__, __LINE__, img2.type());
//printf("%s line %d, img.type = %d.\n", __func__, __LINE__, img.type());
Mat imgs0, imgs1, imgs2;
Mat result0, result1, result2;
Mat imgs[3];
split(img2, imgs);
imgs0 = imgs[0];
imgs1 = imgs[1];
imgs2 = imgs[2];
imshow("RGB-B", imgs0);
imshow("RGB-G", imgs1);
imshow("RGB-R", imgs2);
printf("rows %d, cols %d, channel %d, type %d.\n", img2.rows, img2.cols, img2.channels(), img2.type());
printf("rows %d, cols %d, channel %d, type %d.\n", imgs0.rows, imgs0.cols, imgs0.channels(), imgs0.type());
printf("rows %d, cols %d, channel %d, type %d.\n", imgs1.rows, imgs1.cols, imgs1.channels(), imgs1.type());
printf("rows %d, cols %d, channel %d, type %d.\n", imgs2.rows, imgs2.cols, imgs2.channels(), imgs2.type());
Mat zero = cv::Mat::zeros(img2.rows, img2.cols, CV_8UC1);
imgs[0] = zero;
imgs[2] = zero;
merge(imgs, 3, result1);
imshow("result1", result1);
FILE *file = fopen("network_input.bin", "wb+");
if(file == NULL)
{
cout << "fatal error,create file failure" << endl;
exit(-1);
}
cv::MatIterator_<uchar> it0 = imgs0.begin<uchar>();
cv::MatIterator_<uchar> it0_end = imgs0.end<uchar>();
for (int i = 0; it0 != it0_end; it0 ++, i ++)
{
unsigned char data = *it0;
int count = fwrite(&data, 1, 1, file);
if(count != 1)
{
cout << "write binary failure." << endl;
}
}
cv::MatIterator_<uchar> it1 = imgs1.begin<uchar>();
cv::MatIterator_<uchar> it1_end = imgs1.end<uchar>();
for (int i = 0; it1 != it1_end; it1 ++, i ++)
{
unsigned char data = *it1;
int count = fwrite(&data, 1, 1, file);
if(count != 1)
{
cout << "write binary failure." << endl;
}
}
cv::MatIterator_<uchar> it2 = imgs2.begin<uchar>();
cv::MatIterator_<uchar> it2_end = imgs2.end<uchar>();
for (int i = 0; it2 != it2_end; it2 ++, i ++)
{
unsigned char data = *it2;
int count = fwrite(&data, 1, 1, file);
if(count != 1)
{
cout << "write binary failure." << endl;
}
}
fsync(fileno(file));
fflush(file);
fclose(file);
waitKey(0);
return 0;
}
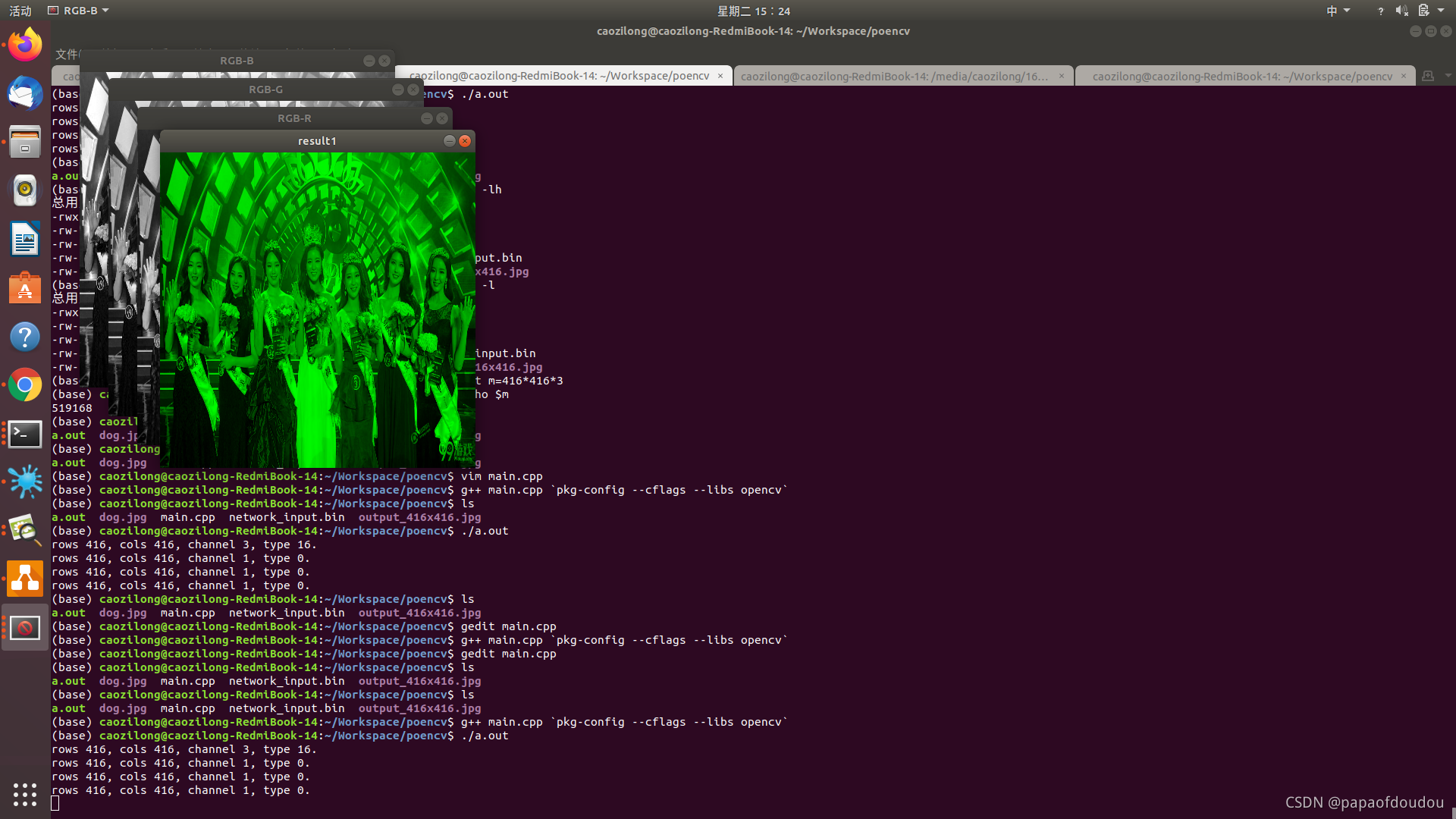
结束!
|