一、引言
问题:现存的CNN要求固定尺寸的输入图片,需要通过对原图进行裁剪或者变形来实现,这两种方式可能出现不同的问题:(i)裁剪的区域可能没法包含物体的整体;(ii)变形操作造成目标无用的几何失真。如果识别目标尺寸变化多样,那么提前定义好的尺寸就可能不太适合。 产生原因:CNN主要由卷积层和全连接层组成,其中卷积层输出尺寸为一个关于输入大小的变量,而全连接层则产生固定大小的输出,也需要固定大小的权重和输入,所以CNN网络的限制在全连接层需要固定长度的输入。
解决方案:SPP,Spytial Pyramid Pooling,空间金字塔池化;
二、原理
功能:remove the fixed-size constraint of the network:对于任意大小特征图(feature map)进行池化的方法,产生固定长度的输出到全连接层,避免裁剪或者变形,与特征提取的卷积层独立;
特征图:原始照片经过卷积层后的输出就是feature maps。Feature maps包含了两个方面的信息:(i)对某些特征的响应强度;(ii)对应的空间位置。
SPP的做法是在卷积层后增加一个SPP layer,spp layer将features map拉成要给固定长度的feature vector。然后将feature vector输入到fully-connected layers中。SPP和R-CNN在流程上的区别如下图:

?SPP这样做有两个优点:1.解决的proposal regions(卷积区域)尺寸问题,即对不同输入尺寸产生固定长度的输出;2.可以池化不同尺度的特征,因为输入尺寸的灵活,先计算出feature maps,在表示每个proposal region时候特征图的结果可以共享,节约计算时间。3.使用多层级spatial bins而非单一大小的池化窗口,对形变有鲁棒性.?SPP表示如下:
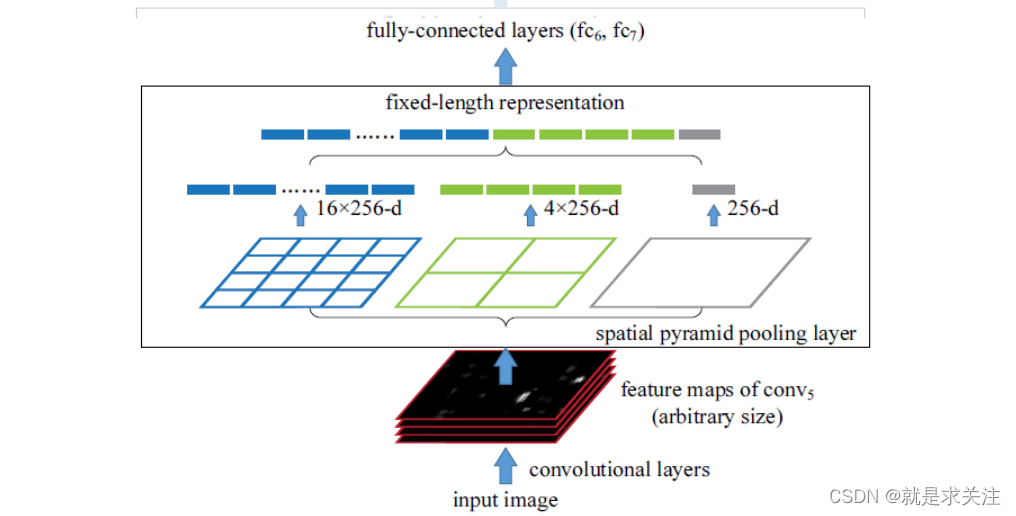
?在卷积层后跟一个SPP layer,形成一个固定长度的特征向量后再输入全连接层。 比如任意大小的原始图像在经过卷积层后生成feature maps,假设最后一个卷积层是conv5,使用了256个filters,生成的feature maps就是(W, H, 256)的大小,每层feature map的大小是(W, H),一共有256层feature map。假设其中有个proposal region对应到feature maps上的大小为(w, h, 256),输入到spatial pyramid pooling layer,SPP layer将feature maps分成4*4,2*2,1*1三个level的bin,经过max pooling后将每层feature map就变成16,4,1三个level的featurevector,因为一共有256层feature maps,所以再将每层的feature vector组合在一起,变成16*256-d、4*256-d、1*256-d三个level的vector。最后将这些vectors再组合再一起作为SPPlayer的输出。
可以总结为如下步骤:
1. 如上图所示,当我们输入一张图片的时候,我们利用不同大小的刻度,对一张图片进行了划分。上面示意图中,利用了三种不同大小的刻度(4*4,2*2,1*1),对一张输入的图片进行了划分,最后总共可以得到16+4+1=21个块,我们即将从这21个块中,每个块提取出一个特征,这样刚好就是我们要提取的21维特征向量。
2. 第一张图片,我们把一张完整的图片,分成了16个块,也就是每个块的大小就是(w/4,h/4);
3.第二张图片,划分了4个块,每个块的大小就是(w/2,h/2);
4.第三张图片,把一整张图片作为了一个块,也就是块的大小为(w,h)。
5.空间金字塔最大池化的过程,其实就是从这21个图片块中,分别计算每个块的最大值,从而得到一个输出神经元。最后把一张任意大小的图片转换成了一个固定大小的21维特征(当然你可以设计其它维数的输出,增加金字塔的层数,或者改变划分网格的大小)。上面的三种不同刻度的划分,每一种刻度我们称之为:金字塔的一层,每一个图片块大小我们称之为:windows size了。如果你希望,金字塔的某一层输出n*n个特征,那么你就要用windows size大小为:(w/n,h/n)进行池化了。
3.代码实现
神经网络框架版本(tensorflow==1.14;keras==2.2.4)
原始Spatial Pyramid Pooling,SPP代码实现如下:
from keras.engine.topology import Layer
import keras.backend as K
class SpatialPyramidPooling(Layer):
def __init__(self, pool_list, **kwargs):
self.dim_ordering = K.image_dim_ordering()
assert self.dim_ordering in {'tf', 'th'}, 'dim_ordering must be in {tf, th}'
self.pool_list = pool_list
self.num_outputs_per_channel = sum([i * i for i in pool_list])
super(SpatialPyramidPooling, self).__init__(**kwargs)
def build(self, input_shape):
if self.dim_ordering == 'th':
self.nb_channels = input_shape[1]
elif self.dim_ordering == 'tf':
self.nb_channels = input_shape[3]
def compute_output_shape(self, input_shape):
return (input_shape[0], self.nb_channels * self.num_outputs_per_channel)
def get_config(self):
config = {'pool_list': self.pool_list}
base_config = super(SpatialPyramidPooling, self).get_config()
return dict(list(base_config.items()) + list(config.items()))
def call(self, x, mask=None):
input_shape = K.shape(x)
if self.dim_ordering == 'th':
num_rows = input_shape[2]
num_cols = input_shape[3]
elif self.dim_ordering == 'tf':
num_rows = input_shape[1]
num_cols = input_shape[2]
row_length = [K.cast(num_rows, 'float32') / i for i in self.pool_list]
col_length = [K.cast(num_cols, 'float32') / i for i in self.pool_list]
outputs = []
if self.dim_ordering == 'th':
for pool_num, num_pool_regions in enumerate(self.pool_list):
for jy in range(num_pool_regions):
for ix in range(num_pool_regions):
x1 = ix * col_length[pool_num]
x2 = ix * col_length[pool_num] + col_length[pool_num]
y1 = jy * row_length[pool_num]
y2 = jy * row_length[pool_num] + row_length[pool_num]
x1 = K.cast(K.round(x1), 'int32')
x2 = K.cast(K.round(x2), 'int32')
y1 = K.cast(K.round(y1), 'int32')
y2 = K.cast(K.round(y2), 'int32')
new_shape = [input_shape[0], input_shape[1], y2 - y1, x2 - x1]
x_crop = x[:, :, y1:y2, x1:x2]
xm = K.reshape(x_crop, new_shape)
pooled_val = K.max(xm, axis=(2, 3))
outputs.append(pooled_val)
elif self.dim_ordering == 'tf':
for pool_num, num_pool_regions in enumerate(self.pool_list):
for jy in range(num_pool_regions):
for ix in range(num_pool_regions):
x1 = ix * col_length[pool_num]
x2 = ix * col_length[pool_num] + col_length[pool_num]
y1 = jy * row_length[pool_num]
y2 = jy * row_length[pool_num] + row_length[pool_num]
x1 = K.cast(K.round(x1), 'int32')
x2 = K.cast(K.round(x2), 'int32')
y1 = K.cast(K.round(y1), 'int32')
y2 = K.cast(K.round(y2), 'int32')
new_shape = [input_shape[0], y2 - y1, x2 - x1, input_shape[3]]
x_crop = x[:, y1:y2, x1:x2, :]
xm = K.reshape(x_crop, new_shape)
pooled_val = K.max(xm, axis=(1, 2))
outputs.append(pooled_val)
if self.dim_ordering == 'th':
outputs = K.concatenate(outputs)
elif self.dim_ordering == 'tf':
outputs = K.concatenate(outputs)
return outputs
如何调用呢?参考如下:
model = Sequential()
model.add(Convolution2D(32, 3, 3, border_mode='same', input_shape=(3, None, None)))
model.add(Activation('relu'))
model.add(Convolution2D(32, 3, 3))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Convolution2D(64, 3, 3, border_mode='same'))
model.add(Activation('relu'))
model.add(Convolution2D(64, 3, 3))
model.add(Activation('relu'))
model.add(SpatialPyramidPooling([1, 2, 4]))
model.add(Dense(num_classes))
model.add(Activation('softmax'))
model.compile(loss='categorical_crossentropy', optimizer='sgd')
测试代码实现可以在下面链接下载:
keras-spp代码实现.rar-深度学习文档类资源-CSDN下载
?
|