Pytorch实现机器学习之线性回归2.0
一、Pytorch实现机器学习之线性回归1.0,特点在利用tensor手动实现线性回归反向传播。
点击打开《Pytorch实现机器学习之线性回归》文章
二、Pytorch实现机器学习之线性回归2.0,特点在利用autograd和Variable实现线性回归,并且反向传播自动调用backward实现。
import torch as t
from matplotlib import pyplot as plt
from IPython import display
from torch.autograd import Variable as V
t.manual_seed(10)
def get_randn_data(batch_size=8):
"""产生随机数x并给线性函数y=2x+3加上噪声,便于后面学习能否贴近结果"""
x = t.randn(batch_size,1)*20
y = x * 2 + (1 + t.randn(batch_size,1))*3
return x,y
w = V(t.randn(1,1),requires_grad=True)
b = V(t.zeros(1,1),requires_grad=True)
lr = 0.0001
listw = []
listb = []
for count in range(20000):
x,y = get_randn_data()
x,y = V(x),V(y)
y_p = x.mm(w) + b.expand_as(y)
loss = 0.5 * (y_p - y) ** 2
loss = loss.sum()
loss.backward()
w.data.sub_(lr * w.grad.data)
b.data.sub_(lr * b.grad.data)
w.grad.data.zero_()
b.grad.data.zero_()
if count % 1000 == 0:
plt.xlim(0,20)
plt.ylim(0,45)
display.clear_output(wait=True)
x1 = t.arange(0,20).view(-1,1)
x1 = x1.float()
y1 = x1.mm(w.data) + b.data.expand_as(x1)
plt.plot(x1.squeeze().numpy(),y1.squeeze().numpy())
x2,y2 = get_randn_data(batch_size=20)
plt.scatter(x2.squeeze().numpy(),y2.squeeze().numpy())
plt.show(block=False)
plt.pause(2)
plt.close()
listw.append((w.data.squeeze().numpy().tolist()))
listb.append((b.data.squeeze().numpy().tolist()))
plt.title("Point diagram of linear regression change of parameter value")
plt.xlabel("parameter w")
plt.ylabel("parameter b")
plt.scatter(listw,listb)
plt.show(block=False)
plt.pause(10)
plt.close()
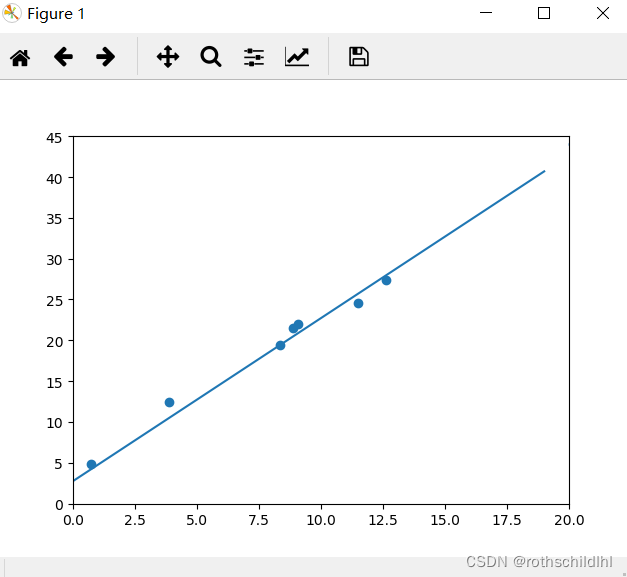 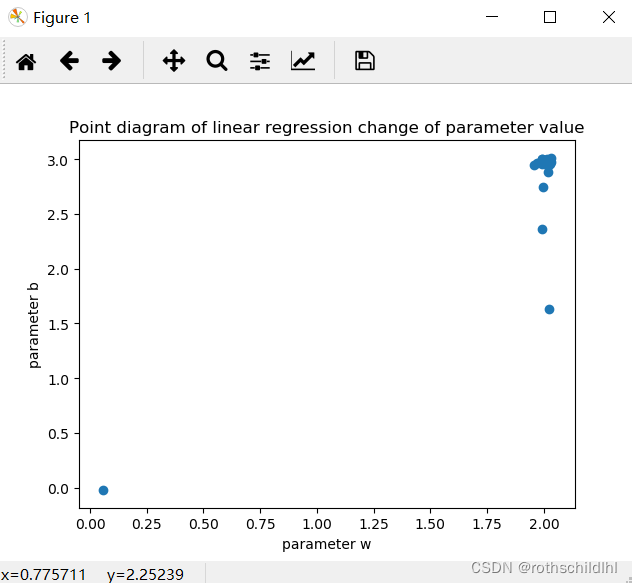
三、代码理解过程中可能需要用到的文章如下:
1.python以三维tensor为例详细理解unsqueeze和squeeze函数 2.Pytorch中设计随机数种子的必要性 3.Pytorch中autograd.Variable.backward的grad_varables参数个人理解浅见
|