环境
- Python 3.8.8
- PyCharm 2021
- opencv-python
效果
原始圆环: 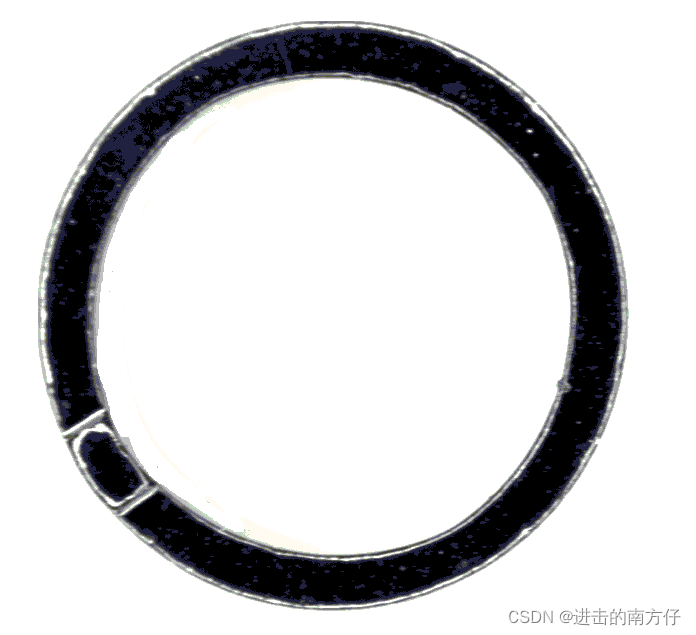 展平之后: 
基本原理
x
=
c
e
n
t
e
r
.
x
+
r
h
o
?
c
o
s
θ
y
=
c
e
n
t
e
r
.
y
?
r
h
o
?
s
i
n
θ
x = center.x+rho * cos\theta\\ y=center.y - rho * sin\theta\\
x=center.x+rho?cosθy=center.y?rho?sinθ
- 因为在计算过程中像素值可能是 float 类型,所以要将矩形的数据类型转回 np.uint8 类型。
代码
import cv2 as cv
import numpy as np
import copy
def circle_flatten() :
img = cv.imread('images/circle_band.bmp')
img_gray = cv.cvtColor(img, cv.COLOR_BGR2GRAY)
circles = cv.HoughCircles(img_gray, cv.HOUGH_GRADIENT, 1, 50, param1 = 170, param2 = 100).squeeze()
circle_radius = circles[ : , 2]
radius_biggest_index = np.argsort(circle_radius)[-1]
print(radius_biggest_index)
circle = np.uint16(np.around(circles[radius_biggest_index]))
cv.circle(img, (circle[0], circle[1]), radius = circle[2], color = (0, 0, 255), thickness = 5)
cv.circle(img, (circle[0], circle[1]), radius = 2, color = (255, 0, 0), thickness = 2)
height = int(circle_radius[radius_biggest_index] * np.pi * 2)
width = int(circle_radius[radius_biggest_index] / 3)
rectangle = np.zeros([width, height])
print(rectangle.shape)
print(img_gray.shape)
for row in range(width) :
for col in range(height) :
theta = np.pi * 2.0 / height * (col + 1)
rho = circle_radius[radius_biggest_index] - row - 1
position_x = int(circle[0] + rho * np.cos(theta) + 0.5)
position_y = int(circle[1] - rho * np.sin(theta) + 0.5)
rectangle[row, col] = img_gray[position_y, position_x]
rectangle = np.uint8(rectangle)
cv.imwrite('flatten.png', rectangle)
cv.imshow('rectangle', rectangle)
cv.imshow('img', img)
cv.waitKey(0)
if __name__ == '__main__':
circle_flatten()
|