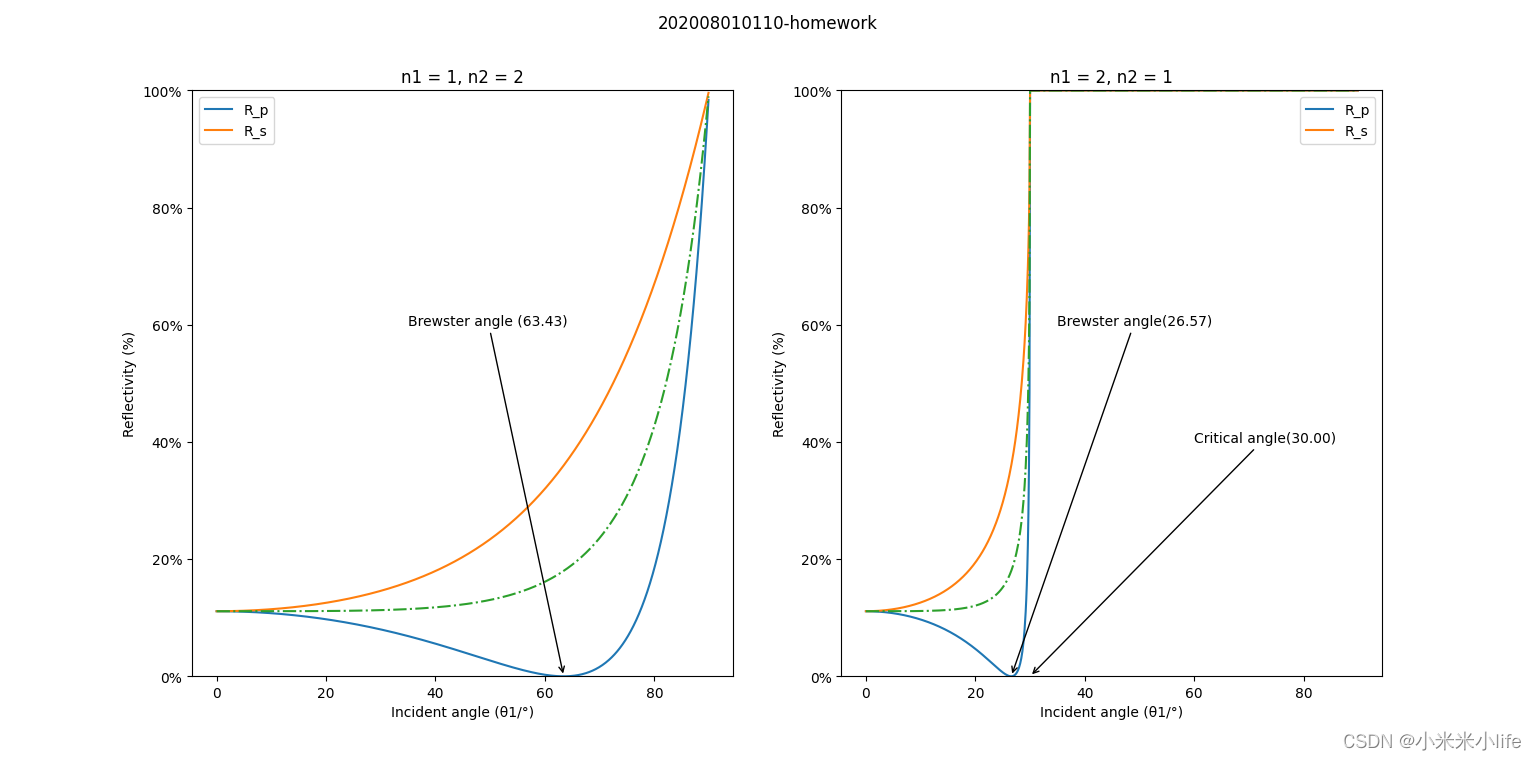
import matplotlib.pyplot as plt
from matplotlib.ticker import FuncFormatter
#数学公式包
"""
np.pi
np.cos(此处输入的是弧度制)
np.sqrt()
np.sin()
平方是**
"""
import numpy as np
"""
def 函数名字(输入参数)
return
"""
#公式
def fresnel(theta, n1, n2):
theta = theta*np.pi/180
xTheta = np.cos(theta)
mid = np.sqrt(1-(n1/n2*np.sin(theta))**2) #中间变量
rp = (n2*xTheta-n1*mid)/(n2*xTheta+n1*mid) #p分量振幅反射率
rs = (n1*xTheta-n2*mid)/(n1*xTheta+n2*mid) #
tp = 2*n1*xTheta/(n2*xTheta+n1*mid)
ts = 2*n1*xTheta/(n1*xTheta+n2*mid)
return rp, rs, tp, ts
#画图
def testFres1(n1=1,n2=1.45): #默认n2为1.45
theta = np.arange(0,90,0.1)+0j #
a = theta*np.pi/180
rp,rs,tp,ts = fresnel(theta,n1,n2)
fig = plt.figure(1)
plt.subplot(1,2,1)
plt.plot(theta,rp,'-',label='rp')
plt.plot(theta,rs,'-.',label='rs')
plt.plot(theta,np.abs(rp),'--',label='|rp|')
plt.plot(theta,np.abs(rs),':',label='|rs|')
plt.legend()
plt.subplot(1,2,2)
plt.plot(theta,tp,'-',label='tp')
plt.plot(theta,ts,'-.',label='ts')
plt.plot(theta,np.abs(tp),'--',label='|tp|')
plt.plot(theta,np.abs(ts),':',label='|ts|')
plt.legend()
plt.show()
def testFres2(n1=1,n2=1.5):
theta = np.arange(0,90,0.1)+0j
a = theta*np.pi/180
rp,rs,tp,ts = fresnel(theta,n1,n2)
Rp = np.abs(rp)**2
Rs = np.abs(rs)**2
Rn = (Rp+Rs)/2
fig = plt.figure(1)
plt.subplot(1,2,1)
plt.plot(theta,Rp,'-',label='R_p')
#plt.plot(theta,Rs,'-.',label='R_s')
plt.plot(theta,Rn,'-',label='R_n')
plt.legend()
plt.show()
def testFres3(n1=1.5,n2=1):
theta = np.arange(0,90,0.1)+0j
a = theta*np.pi/180
rp,rs,tp,ts = fresnel(theta,n1,n2)
Rp = np.abs(rp)**2
Rs = np.abs(rs)**2
Rn = (Rp+Rs)/2
fig = plt.figure(2)
plt.subplot(1,2,1)
plt.plot(theta,Rp,'-',label='R_p')
plt.plot(theta,Rs,'-.',label='R_s')
plt.plot(theta,Rn,'-',label='R_n')
plt.legend()
plt.show()
def testFres():
theta = np.arange(0,90,0.1)+0j #
a = theta*np.pi/180
rp,rs,tp,ts = fresnel(theta,1,2)
Rp = np.abs(rp)**2
Rs = np.abs(rs)**2
Rn = (Rp+Rs)/2
plt.suptitle("202008010110-homework")
plt.subplot(1,2,1)
plt.title("n1 = 1, n2 = 2")
plt.plot(theta,Rp,'-',label='R_p')
plt.plot(theta,Rs,'-',label='R_s')
plt.plot(theta,Rn,'-.',label='')
plt.ylim(0,1)#y轴上限为1,下限为0
def to_percent(temp, position):#百分比上下限
return '%1.0f'%(100*temp) + '%'
plt.gca().yaxis.set_major_formatter(FuncFormatter(to_percent))
plt.legend() #图例
plt.xlabel("Incident angle (θ1/°)")
plt.ylabel("Reflectivity (%)")
#设置注释
plt.annotate('Brewster angle (%.2f)'%(180*np.arctan(2)/np.pi), xy=(180*np.arctan(2)/np.pi, 0), xytext=(35, 0.6),
xycoords='data',
arrowprops=dict(facecolor='black',
arrowstyle = "->",)
)
rp,rs,tp,ts = fresnel(theta,2,1)
Rp = np.abs(rp)**2
Rs = np.abs(rs)**2
Rn = (Rp+Rs)/2
plt.subplot(1,2,2)
plt.title("n1 = 2, n2 = 1")
plt.plot(theta,Rp,'-',label='R_p')
plt.plot(theta,Rs,'-',label='R_s')
plt.plot(theta,Rn,'-.',label='')
plt.ylim(0,1)#上限为1,下限为0
def to_percent(temp, position):#百分比上下限
return '%1.0f'%(100*temp) + '%'
plt.gca().yaxis.set_major_formatter(FuncFormatter(to_percent))
plt.legend() #图例
plt.xlabel("Incident angle (θ1/°)")
plt.ylabel("Reflectivity (%)")
#设置注释
plt.annotate('Brewster angle(%.2f)'%(180*np.arctan(0.5)/np.pi), xy=(180*np.arctan(0.5)/np.pi, 0), xytext=(35, 0.6),
xycoords='data',
arrowprops=dict(facecolor='black',
arrowstyle = "->",)
)
plt.annotate('Critical angle(%.2f)'%(180*np.arcsin(0.5)/np.pi), xy=(180*np.arcsin(0.5)/np.pi, 0), xytext=(60, 0.4),
xycoords='data',
arrowprops=dict(facecolor='black',
arrowstyle = "->",)
)
plt.show()
#主函数
#testFres2()
#testFres3()
testFres()
(14条消息) python光学仿真之菲涅耳公式_微小冷的博客-CSDN博客_菲涅尔公式
(14条消息) Python学习笔记(4)——Matplotlib中的annotate(注解)的用法_赵赵赵颖的博客-CSDN博客_annotate
|