1,furthest point sampling or farthest point sampling
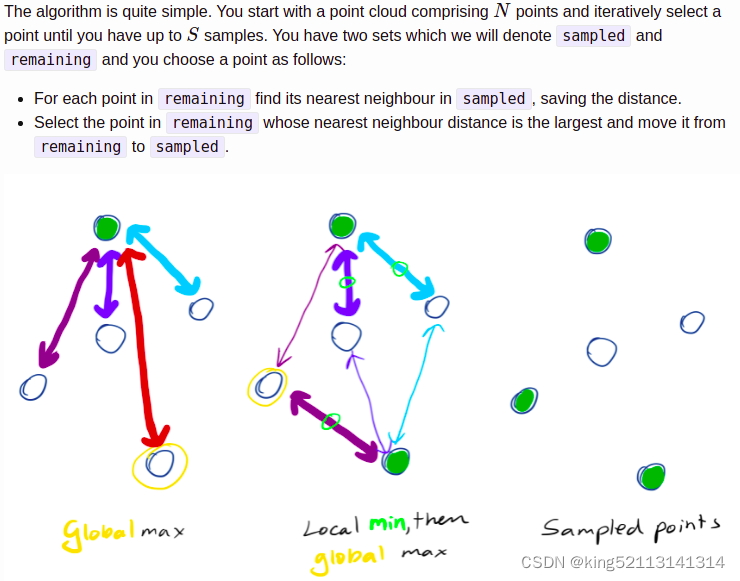
Furthest Point Sampling | Minibatch AI
def fps(points, n_samples):
"""
points: [N, 3] array containing the whole point cloud
n_samples: samples you want in the sampled point cloud typically << N
"""
points = np.array(points)
# Represent the points by their indices in points
points_left = np.arange(len(points)) # [P]
# Initialise an array for the sampled indices
sample_inds = np.zeros(n_samples, dtype='int') # [S]
# Initialise distances to inf
dists = np.ones_like(points_left) * float('inf') # [P]
# Select a point from points by its index, save it
selected = 0
sample_inds[0] = points_left[selected]
# Delete selected
points_left = np.delete(points_left, selected) # [P - 1]
# Iteratively select points for a maximum of n_samples
for i in range(1, n_samples):
# Find the distance to the last added point in selected
# and all the others
last_added = sample_inds[i-1]
dist_to_last_added_point = (
(points[last_added] - points[points_left])**2).sum(-1) # [P - i]
# If closer, updated distances
dists[points_left] = np.minimum(dist_to_last_added_point,
dists[points_left]) # [P - i]
# We want to pick the one that has the largest nearest neighbour
# distance to the sampled points
selected = np.argmax(dists[points_left])
sample_inds[i] = points_left[selected]
# Update points_left
points_left = np.delete(points_left, selected)
return points[sample_inds]
2,PointNet
PointNet
https://www.youtube.com/watch?v=Ew24Rac8eYE&ab_channel=%E5%B0%86%E9%97%A8-TechBeat%E6%8A%80%E6%9C%AF%E7%A4%BE%E5%8C%BA
https://medium.com/@luis_gonzales/an-in-depth-look-at-pointnet-111d7efdaa1a?
Point cloud classification with PointNet?
https://github.com/luis-gonzales/pointnet_own?
细嚼慢咽读论文:PointNet论文及代码详细解析 - 知乎?
3,PointNet++
PointNet++?
GitHub - dgriffiths3/pointnet2-tensorflow2: Pointnet++ modules implemented as tensorflow 2 keras layers.?基于PyTorch实现PointNet++ - 知乎
GitHub - HarborZeng/pointnet2-keras: pointnet2 implemented using keras and tensorflow?
Colab上运行pointnet++-小黄鸭花生屋?
GitHub - yangyanli/PointCNN: PointCNN: Convolution On X-Transformed Points (NeurIPS 2018)?
搞懂PointNet++,这篇文章就够了! - 知乎?
|