pytorch 莫烦
激励函数
Y = AF(Wx) 这里的AF()就是激励函数,其实就是另外一个非线性函数。比如relu,sigmoid,tanh
- 选择激励函数的窍门:当神经网络层只有两三层时,可选择任意的激励函数;当神经网络特别多层时,要慎重,小心梯度爆炸
- CNN时推荐relu
- RNN时推荐tanh或者relu
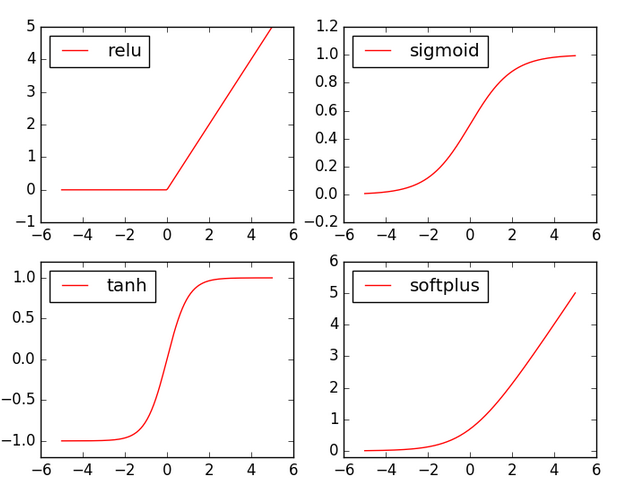
回归
class Net(torch.nn.Module):
def __init__(self, n_feature, n_hidden, n_output):
super(Net, self).__init__()
self.hidden = torch.nn.Linear(n_feature, n_hidden)
self.predict = torch.nn.Linear(n_hidden, n_output)
def forward(self, x):
x = F.relu(self.hidden(x))
x = self.predict(x)
return x
optimizer = torch.optim.SGD(net.parameters(), lr=0.2)
loss_func = torch.nn.MSELoss()
for t in range(100):
prediction = net(x)
loss = loss_func(prediction, y)
optimizer.zero_grad()
loss.backward()
optimizer.step()
分类
def forward(self, x):
x = F.relu(self.hidden(x))
x = self.out(x)
return x
loss_func = torch.nn.CrossEntropyLoss()
快速搭建
搭建神经网络不止class net()这种方法,有一个快速的方法torch.nn.Sequential()
net = torch.nn.Sequential(
torch.nn.Linear(1, 10),
torch.nn.ReLU(),
torch.nn.Linear(10, 1)
)
Sequential方法直接认定的就是relu()这种激励函数,而对于自己手写的net来说,可以在forward()方法中指定激励函数,就会更加灵活一些。
保存与提取
torch.save(net1, 'net.pkl')
torch.save(net1.state_dict(), 'net_params.pkl')
def restore_net():
net2 = torch.load('net.pkl')
prediction = net2(x)
- 提取网络参数
网络参数:能独立地反映网络特性的参数 提取所有网路参数
net3.load_state_dict(torch.load('net_params.pkl'))
prediction = net3(x)
批训练
DataLoader
torch_dataset = Data.TensorDataset(data_tensor=x, target_tensor=y)
loader = Data.DataLoader(
dataset=torch_dataset,
batch_size=BATCH_SIZE,
shuffle=True,
num_workers=2,
)
优化器
要让神经网络聪明起来!!!!
- SGD
- Momentum
- AdaGrad
- RMSProp
- Adam
- SGD
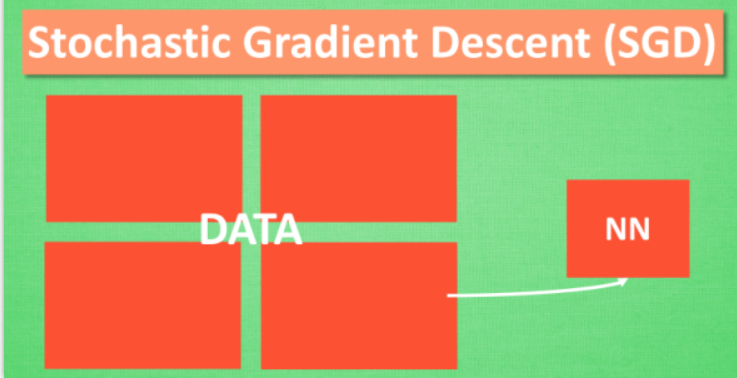 - Momentum
所以我们把这个人从平地上放到了一个斜坡上, 只要他往下坡的方向走一点点, 由于向下的惯性, 他不自觉地就一直往下走, 走的弯路也变少了. 这就是 Momentum 参数更新. 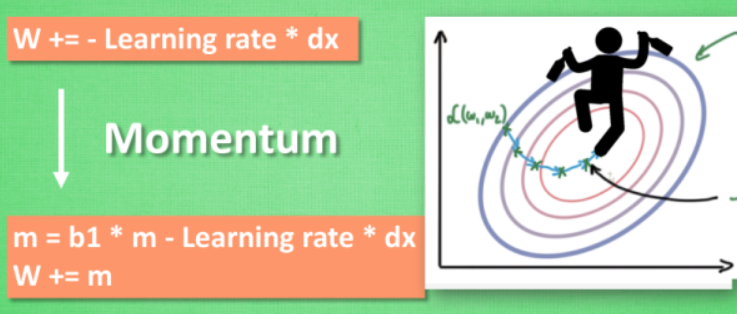 - AdaGrad
而是给他一双不好走路的鞋子, 使得他一摇晃着走路就脚疼, 鞋子成为了走弯路的阻力, 逼着他往前直着走 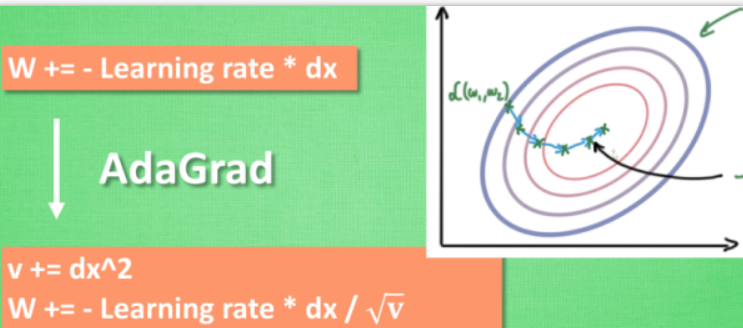 - RMSProp
是momentum和adagrad的集合体,同时具备两者的优势。但是RMSProp并没有包含momentum的一部分,所以在Adam中又进一步改进 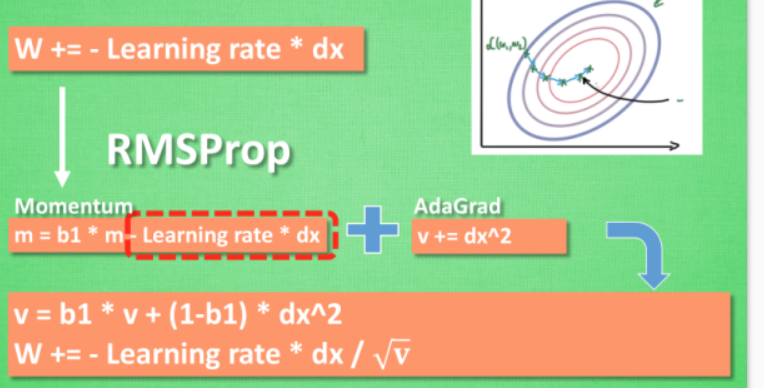 - Adam
对于Adam来说,能快好的达到目标,快速收敛到最好的地方 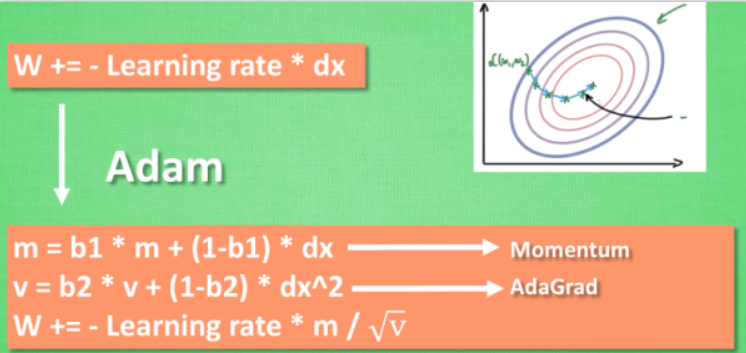
Optimizer
net_SGD = Net()
net_Momentum = Net()
net_RMSprop = Net()
net_Adam = Net()
opt_SGD = torch.optim.SGD(net_SGD.parameters(), lr=LR)
opt_Momentum = torch.optim.SGD(net_Momentum.parameters(), lr=LR, momentum=0.8)
opt_RMSprop = torch.optim.RMSprop(net_RMSprop.parameters(), lr=LR, alpha=0.9)
opt_Adam = torch.optim.Adam(net_Adam.parameters(), lr=LR, betas=(0.9, 0.99))
在实验中,对各个优化器还是都应该试一试,看看哪个更好
CNN
-
- 从下到上的顺序, 首先是输入的图片(image), 经过一层卷积层 (convolution), 然后在用池化(pooling)方式处理卷积的信息, 这里使用的是 max pooling 的方式.
- 然后在经过一次同样的处理, 把得到的第二次处理的信息传入两层全连接的神经层 (fully connected),这也是一般的两层神经网络层,最后在接上一个分类器(classifier)进行分类预测. 这仅仅是对卷积神经网络在图片处理上一次简单的介绍.
-
- 卷积层(Convolutional Layer) - 主要作用是提取特征
- 池化层(Max Pooling Layer) - 主要作用是下采样(downsampling),却不会损坏识别结果
- 全连接层(Fully Connected Layer) - 主要作用是分类预测
class CNN(nn.Module):
def __init__(self):
super(CNN, self).__init__()
self.conv1 = nn.Sequential(
nn.Conv2d(
in_channels=1,
out_channels=16,
kernel_size=5,
stride=1,
padding=2,
),
nn.ReLU(),
nn.MaxPool2d(kernel_size=2),
)
self.conv2 = nn.Sequential(
nn.Conv2d(16, 32, 5, 1, 2),
nn.ReLU(),
nn.MaxPool2d(2),
)
self.out = nn.Linear(32 * 7 * 7, 10)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
x = x.view(x.size(0), -1)
output = self.out(x)
return output
这个 CNN 整体流程是 卷积(Conv2d) -> 激励函数(ReLU) -> 池化, 向下采样 (MaxPooling) -> 再来一遍 -> 展平多维的卷积成的特征图 -> 接入全连接层 (Linear) -> 输出
RNN
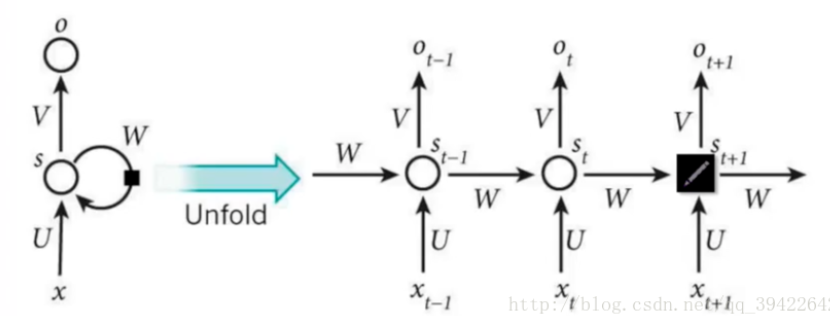 RNN是在有顺序的数据上进行学习的,在反向传递得到误差的时候,每一步都会乘以自己的一个参数W,若W是小于1,则误差传递到初始时间的时候会接近0,即梯度消失;反之,则是梯度爆炸!hong!然后LSTM是为了解决这个问题而提出来的
LSTM循环神经网络
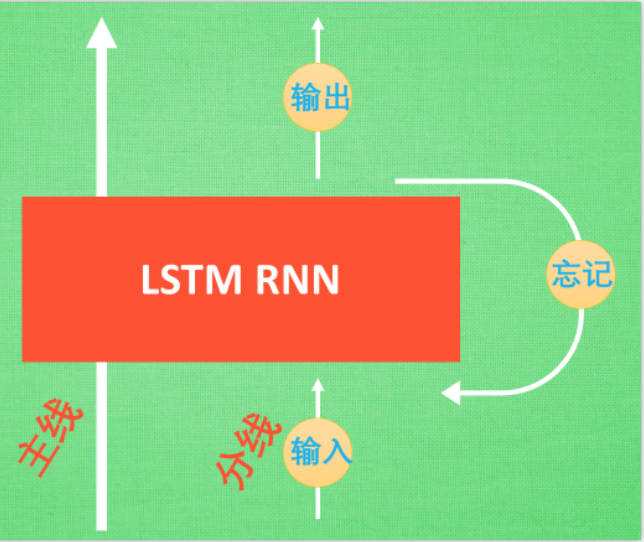
RNN分类问题
class RNN(nn.Module):
def __init__(self):
super(RNN, self).__init__()
self.rnn = nn.LSTM(
input_size=28,
hidden_size=64,
num_layers=1,
batch_first=True,
)
self.out = nn.Linear(64, 10)
def forward(self, x):
r_out, (h_n, h_c) = self.rnn(x, None)
out = self.out(r_out[:, -1, :])
return out
RNN整体的流程是: 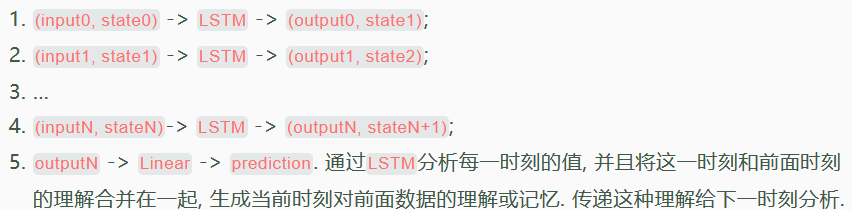
RNN回归问题
class RNN(nn.Module):
def __init__(self):
super(RNN, self).__init__()
self.rnn = nn.RNN(
input_size=1,
hidden_size=32,
num_layers=1,
batch_first=True,
)
self.out = nn.Linear(32, 1)
def forward(self, x, h_state):
r_out, h_state = self.rnn(x, h_state)
outs = []
for time_step in range(r_out.size(1)):
outs.append(self.out(r_out[:, time_step, :]))
return torch.stack(outs, dim=1), h_state
自编码(Autoencoder)
- 是一种非监督式学习,接受大量的输入信息,然后总结原数据的精髓。
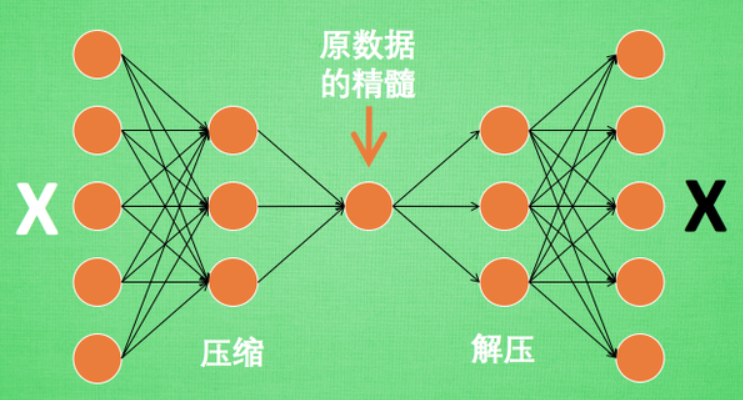 - 编码器Encoder
特征属性降维 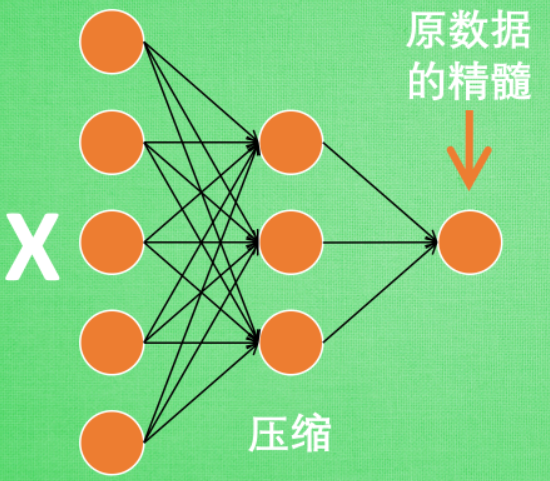 - 解码器Decoder
将精髓信息解压成原始信息
AutoEncoder
class AutoEncoder(nn.Module):
def __init__(self):
super(AutoEncoder, self).__init__()
self.encoder = nn.Sequential(
nn.Linear(28*28, 128), 28*28->128
nn.Tanh(),
nn.Linear(128, 64), 128->64
nn.Tanh(),
nn.Linear(64, 12), 64->12
nn.Tanh(),
nn.Linear(12, 3),
)
self.decoder = nn.Sequential(
nn.Linear(3, 12),
nn.Tanh(),
nn.Linear(12, 64),
nn.Tanh(),
nn.Linear(64, 128),
nn.Tanh(),
nn.Linear(128, 28*28),
nn.Sigmoid(),
)
def forward(self, x):
encoded = self.encoder(x)
decoded = self.decoder(encoded)
return encoded, decoded
autoencoder = AutoEncoder()
DQN
强化学习融合了神经网络+Q-learing 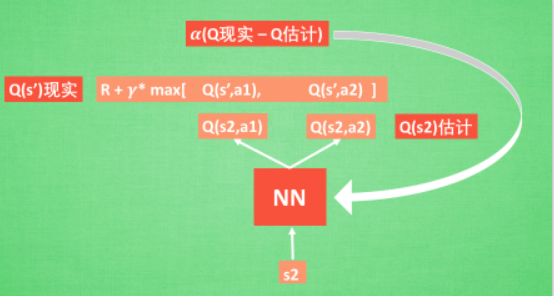
- 通过NN预测出Q(s2, a1) 和 Q(s2,a2) 的值,即Q估计
- 选取Q估计中最大值的动作来换取还清中的奖励reward
- Q现实是之前在Q-learing中的值
- 更新神经网络中的参数
class Net(nn.Module):
def __init__(self, ):
super(Net, self).__init__()
self.fc1 = nn.Linear(N_STATES, 10)
self.fc1.weight.data.normal_(0, 0.1)
self.out = nn.Linear(10, N_ACTIONS)
self.out.weight.data.normal_(0, 0.1)
def forward(self, x):
x = self.fc1(x)
x = F.relu(x)
actions_value = self.out(x)
return actions_value
class DQN(object):
def __init__(self):
def choose_action(self, x):
return action
def store_transition(self, s, a, r, s_):
def learn(self):
GAN
大白话解释GAN:新手画家随机灵感画画,新手鉴赏家接受画作(不知道是新手画还是著名画),说出判断,一边还告诉新手怎么画,然后新手就画的越来越像著名画家的画。 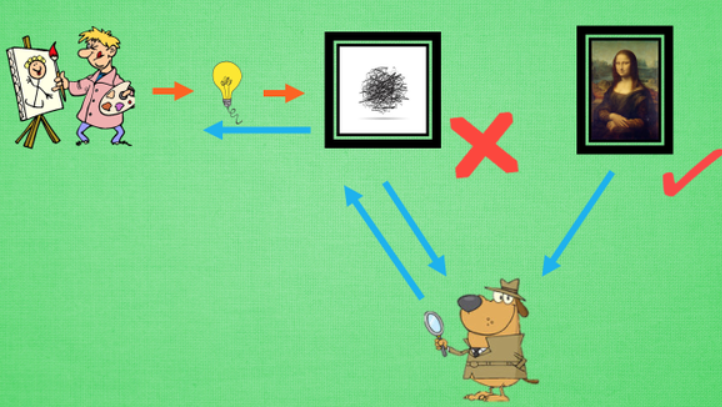
G = nn.Sequential(
nn.Linear(N_IDEAS, 128),
nn.ReLU(),
nn.Linear(128, ART_COMPONENTS),
)
D = nn.Sequential(
nn.Linear(ART_COMPONENTS, 128),
nn.ReLU(),
nn.Linear(128, 1),
nn.Sigmoid(),
)
Dropout缓解过拟合
torch.nn.Dropout(0.5) 这里的 0.5 指的是随机有 50% 的神经元会被关闭/丢弃.
net_dropped = torch.nn.Sequential(
torch.nn.Linear(1, N_HIDDEN),
torch.nn.Dropout(0.5),
torch.nn.ReLU(),
torch.nn.Linear(N_HIDDEN, N_HIDDEN),
torch.nn.Dropout(0.5),
torch.nn.ReLU(),
torch.nn.Linear(N_HIDDEN, 1),
)
批标准化(Batch Normalization)BN
- 将分散的数据统一的一种做法,使数据具有统一规格。
- BN被添加在每一个全连接和激励函数之间,对每一层神经网络进行标准化
class Net(nn.Module):
def __init__(self, batch_normalization=False):
super(Net, self).__init__()
self.do_bn = batch_normalization
self.fcs = []
self.bns = []
self.bn_input = nn.BatchNorm1d(1, momentum=0.5)
for i in range(N_HIDDEN):
input_size = 1 if i == 0 else 10
fc = nn.Linear(input_size, 10)
setattr(self, 'fc%i' % i, fc)
self._set_init(fc)
self.fcs.append(fc)
if self.do_bn:
bn = nn.BatchNorm1d(10, momentum=0.5)
setattr(self, 'bn%i' % i, bn)
self.bns.append(bn)
self.predict = nn.Linear(10, 1)
self._set_init(self.predict)
def _set_init(self, layer):
init.normal_(layer.weight, mean=0., std=.1)
init.constant_(layer.bias, B_INIT)
def forward(self, x):
pre_activation = [x]
if self.do_bn: x = self.bn_input(x)
layer_input = [x]
for i in range(N_HIDDEN):
x = self.fcs[i](x)
pre_activation.append(x)
if self.do_bn: x = self.bns[i](x)
x = ACTIVATION(x)
layer_input.append(x)
out = self.predict(x)
return out, layer_input, pre_activation
nets = [Net(batch_normalization=False), Net(batch_normalization=True)]
|