TensorFlow中的计算图有三种,分别是静态计算图,动态计算图,以及Autograph 目前TensorFlow2默认采用的是动态计算图,即每使用一个算子后,该算子会被动态加入到隐含的默认计算图中立即执行得到结果(在TensorFlow1中,采用的是静态计算图,需要先使用TensorFlow的各种算子创建计算图,然后再开启一个会话Session,显式执行计算图)。 对于动态图的好处显而易见,它方便调试程序,让TensorFlow代码的表现和Python原生代码的表现一样,写起来就像写numpy一样,各种日志打印,控制流全部都是可以使用的,当然,这相对于静态图来讲牺牲了些效率,因为使用动态图会有许多次Python进程和TensorFlow的C++进程之间的通信,而静态计算图构建完成之后几乎全部在TensorFlow内核上使用C++代码执行,效率更高。此外静态图会对计算步骤进行一定的优化,剪去和结果无关的计算步骤。 如何理解TensorFlow计算图?
TF通过计算图将计算的定义和执行分隔开, 这是一种声明式(declaretive)的编程模型. 确实, 这种静态图的执行模式优点很多,但是在debug时确实非常不方便(类似于对编译好的C语言程序调用,此时是我们无法对其进行内部的调试), 因此有了Eager Execution 我们可以不必再等到see.run(*)才能看到执行结果, 可以方便在IDE随时调试代码,查看OPs执行结果. Tensorflow2——Eager模式简介以及运用 应用Tensorflow2.0的Eager模式快速构建神经网络
TensorFlow2 编程教程
TensorFlow2 编程教程
python的with用法 这个叫上下文管理 with语句后面跟着的语句可以设定初始化执行一些函数,在退出时也可以执行一些函数 with语句后面跟着的as是变量,保存的是初始化后的返回值【没有返回值可以不用as】 with语句最后的冒号下的范围是中间过程执行的语句。 中间的语句执行完之后可以执行退出收尾的一些函数,比如 关闭文件、获取输出信息、获取错误信息。 ```python class Sample: def enter(self): print “In enter()” return “Foo” def exit(self, type, value, trace): print “In exit()” def get_sample(): return Sample()
with get_sample() as sample: print “sample:”, sample ```
输出的是
In enter() sample: Foo In exit()
python的with用法
定义常量,普通的tensor
idx = tf.constant(1)
tf.constant(1.)
tf.constant([1])
tf.constant([True, False])
tf.constant('hello nick')
在tensorflow中,我们可以使用 tf.device() 指定模型运行的具体设备,可以指定运行在GPU还是CUP上,以及哪块GPU上。
with tf.device('cpu'):
a = tf.constant([1])
with tf.device('gpu'):
b = tf.constant([1])
a.device
a.gpu()
a.numpy()
a.shape
a.ndim
tf.rank(a)
a.name
数据类型判断
isinstance(a,tf.Tensor)
tf.is_tensor(a)
a.dtype,b.dtype,c.dtype
a = tf.range(5)
b = tf.Variable(a, name='input_data')
b.name
b.trainable
isinstance(b,tf.Tensor)
isinstance(b,tf.Variable)
tf.is_tensor(b)
创建Tensor
a = tf.constant([3])
tf.ones_like(a)
tf.ones(0)
tf.zeros(1)
tf.ones([2])
tf.zeros([2,2])
tf.Tensor(
[[0. 0.]
[0. 0.]], shape=(2, 2), dtype=float32)
tf.fill([2, 2], 0)
tf.Tensor(
[[0 0]
[0 0]], shape=(2, 2), dtype=int32)
创建数据分布
tf.random.normal([2, 2], mean=0, stddev=0.5)
tf.Tensor(
[[ 0.26348853 0.92483073]
[ 0.81219316 -0.52944314]], shape=(2, 2), dtype=float32)
tf.random.uniform([2, 2], minval=0, maxval=100, dtype=tf.int32)
tf.Tensor(
[[73 14]
[84 77]], shape=(2, 2), dtype=int32)
a=tf.range(10)
idx = tf.random.shuffle(a)
y = tf.range(4)
y = tf.one_hot(y, depth=10)
tf.Tensor(
[[1. 0. 0. 0. 0. 0. 0. 0. 0. 0.]
[0. 1. 0. 0. 0. 0. 0. 0. 0. 0.]
[0. 0. 1. 0. 0. 0. 0. 0. 0. 0.]
[0. 0. 0. 1. 0. 0. 0. 0. 0. 0.]], shape=(4, 10), dtype=float32)
out = tf.random.uniform([4, 10])
y = tf.range(4,8)
y = tf.one_hot(y, depth=10)
loss = tf.keras.losses.mse(y, out)
loss = tf.reduce_mean(loss)
高维数据选择
a = tf.ones([2, 3, 4, 6])
tf.Tensor(
[[[[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]]
[[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]]
[[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]]]
[[[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]]
[[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]]
[[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]]]], shape=(2, 3, 4, 6), dtype=float32)
print(a[0][1])
tf.Tensor(
[[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]
[1. 1. 1. 1. 1. 1.]], shape=(4, 6), dtype=float32)
print(a[0].shape)
print(a[0, ...].shape)
print(a[0, ..., 2].shape)
print(a[:, 0, :, :] .shape)
print(a[:, :, :-1, :3] .shape)
print(a[:, :, ::2, 0:6:2] .shape)
步长和倒序
a = tf.range(4)
[0, 1, 2, 3]
a[::-1]
[3, 2, 1, 0]
a[::-2]
[3, 1]
a[2::-2]
[2, 0]
a = tf.range(9)
print(a[3::-2])
[3 1]
a = tf.range(9)
print(a[4::-2])
[4 2 0]
a = tf.range(9)
print(a[::-2])
[8 6 4 2 0]
a = tf.range(9)
print(a[:2:-2])
[8 6 4]
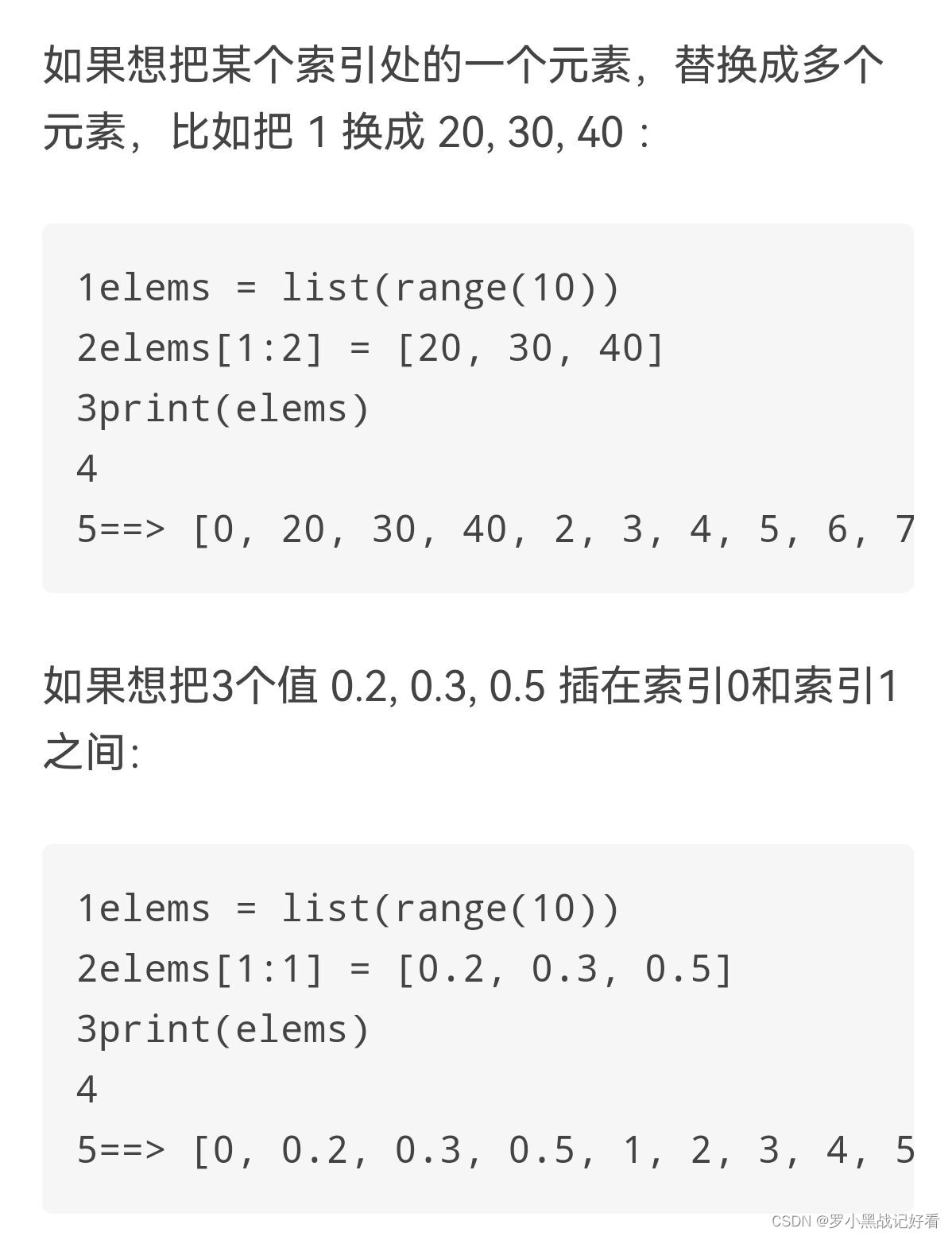
reshape 重组数据
a = tf.random.normal([4, 28, 28, 3])
tf.reshape(a, [4, -1, 3]).shape
tf.reshape(a, [4, -1]).shape
tf.reshape(tf.reshape(a, [4, -1]), [4, 14, 56, 3]).shape
a = tf.random.normal((4, 3, 2, 1))
tf.transpose(a).shape
tf.transpose(a, perm=[0, 1, 3, 2]).shape
Expand_dims(增加维度)
小贴士: [1, 4, 35, 8] + [1, 4, 35, 8] = [2, 4, 35, 8]
a = tf.random.normal([4, 25, 8])
tf.expand_dims(a, axis=0).shape
tf.expand_dims(a, axis=3).shape
Squeeze(挤压维度)
小贴士:只删除维度为1的维度
tf.squeeze(tf.zeros([1,2,1,1,3])).shape
a = tf.zeros([1,2,1,3])
tf.squeeze(a,axis=0).shape
tf.squeeze(a,axis=2).shape
TensorFlow之设备(device)详解 浅谈keras中的目标函数和优化函数MSE用法
|