1、什么是欧几里得点云聚类分割
??在本文中,我们将学习如何使用pcl::EuclideanClusterExtraction类提取欧几里得聚类集群。为了不使文章复杂化,它的某些元素,如平面分割算法,将不在这里解释。更多信息请查看平面模型分割。 ??欧几里得聚类理论基础: ??聚类方法需要将无组织的点云模型P分成更小的部分,从而显著减少P的整体处理时间。欧几里得意义上的简单数据聚类方法可以通过使用固定宽度的框或更一般的八叉树数据结构对空间进行三维网格细分实现。这种特殊的表示方式构建起来非常快,对于需要对所占用空间进行体积表示,或者每个生成的3D框(或八叉树叶)中的数据可以用不同的结构进行近似的情况非常有用。然而,在更一般的意义上,我们可以利用最近的邻居,并实现一种本质上类似于2维处理算法中的洪水填充算法的聚类技术。 ??假设我们有一个点云,上面有一张桌子和一些物体。我们希望找到并分割平面上的单个对象的点集。假设我们使用Kd-tree结构来寻找最近的邻居,算法步骤将是 ① 为输入点云数据集P创建一个kd树表示。 ② 设置一个空的聚类列表C,和一个需要检查的点集队列Q; ③ 对P中每一个点执行以下步骤: ??a. 添加
p
i
\boldsymbol{p}_i
pi?到当前队列Q ?? b.对于Q中的每个点
p
i
{p}_i
pi? ????在半径为r<
d
t
d_t
dt?的球面内寻找
P
i
{P}_i
Pi?内点邻域的集合
P
i
k
;
P^k_i;
Pik?; ????对于
P
i
k
P ^k_i
Pik?中的每个邻点
p
i
k
{p}^k_i
pik?,检查该点是否已经被处理过,如果没有将其添加到Q中; ??c.当Q中所有点的列表处理完毕后,将Q添加到集群C的列表中,并将Q重置为空列表。 ④当P中的所有点
p
i
{p}_i
pi?都已被处理,并且现在是点簇C列表的一部分时,算法终止。
整个流程类似于大水漫灌算法,核心思想是计算点距,把点距符合要求的点聚类。
2、如何使用PCL对点云进行集群分割
??可以下载pcd点云数据文件对以下代码进行测试。点击这里下载。 ??废话不多说,我们直接看代码中PCL聚类如何使用。
#include <pcl/ModelCoefficients.h>
#include <pcl/point_types.h>
#include <pcl/io/pcd_io.h>
#include <pcl/filters/extract_indices.h>
#include <pcl/filters/voxel_grid.h>
#include <pcl/features/normal_3d.h>
#include <pcl/search/kdtree.h>
#include <pcl/sample_consensus/method_types.h>
#include <pcl/sample_consensus/model_types.h>
#include <pcl/segmentation/sac_segmentation.h>
#include <pcl/segmentation/extract_clusters.h>
int
main ()
{
pcl::PCDReader reader;
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud (new pcl::PointCloud<pcl::PointXYZ>), cloud_f (new pcl::PointCloud<pcl::PointXYZ>);
reader.read ("table_scene_lms400.pcd", *cloud);
std::cout << "PointCloud before filtering has: " << cloud->size () << " data points." << std::endl;
pcl::VoxelGrid<pcl::PointXYZ> vg;
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_filtered (new pcl::PointCloud<pcl::PointXYZ>);
vg.setInputCloud (cloud);
vg.setLeafSize (0.01f, 0.01f, 0.01f);
vg.filter (*cloud_filtered);
std::cout << "PointCloud after filtering has: " << cloud_filtered->size () << " data points." << std::endl;
pcl::SACSegmentation<pcl::PointXYZ> seg;
pcl::PointIndices::Ptr inliers (new pcl::PointIndices);
pcl::ModelCoefficients::Ptr coefficients (new pcl::ModelCoefficients);
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_plane (new pcl::PointCloud<pcl::PointXYZ> ());
pcl::PCDWriter writer;
seg.setOptimizeCoefficients (true);
seg.setModelType (pcl::SACMODEL_PLANE);
seg.setMethodType (pcl::SAC_RANSAC);
seg.setMaxIterations (100);
seg.setDistanceThreshold (0.02);
int nr_points = (int) cloud_filtered->size ();
while (cloud_filtered->size () > 0.3 * nr_points)
{
seg.setInputCloud (cloud_filtered);
seg.segment (*inliers, *coefficients);
if (inliers->indices.size () == 0)
{
std::cout << "Could not estimate a planar model for the given dataset." << std::endl;
break;
}
pcl::ExtractIndices<pcl::PointXYZ> extract;
extract.setInputCloud (cloud_filtered);
extract.setIndices (inliers);
extract.setNegative (false);
extract.filter (*cloud_plane);
std::cout << "PointCloud representing the planar component: " << cloud_plane->size () << " data points." << std::endl;
extract.setNegative (true);
extract.filter (*cloud_f);
*cloud_filtered = *cloud_f;
}
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree (new pcl::search::KdTree<pcl::PointXYZ>);
tree->setInputCloud (cloud_filtered);
std::vector<pcl::PointIndices> cluster_indices;
pcl::EuclideanClusterExtraction<pcl::PointXYZ> ec;
ec.setClusterTolerance (0.02);
ec.setMinClusterSize (100);
ec.setMaxClusterSize (25000);
ec.setSearchMethod (tree);
ec.setInputCloud (cloud_filtered);
ec.extract (cluster_indices);
int j = 0;
for (std::vector<pcl::PointIndices>::const_iterator it = cluster_indices.begin (); it != cluster_indices.end (); ++it)
{
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_cluster (new pcl::PointCloud<pcl::PointXYZ>);
for (const auto& idx : it->indices)
cloud_cluster->push_back ((*cloud_filtered)[idx]);
cloud_cluster->width = cloud_cluster->size ();
cloud_cluster->height = 1;
cloud_cluster->is_dense = true;
std::cout << "PointCloud representing the Cluster: " << cloud_cluster->size () << " data points." << std::endl;
std::stringstream ss;
ss << "cloud_cluster_" << j << ".pcd";
writer.write<pcl::PointXYZ> (ss.str (), *cloud_cluster, false);
j++;
}
return (0);
}
欧几里得聚类分割效果如下: 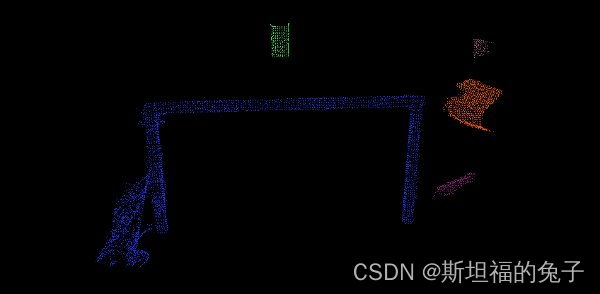
【博主简介】 ??斯坦福的兔子,男,天津大学机械工程工学硕士。毕业至今从事光学三维成像及点云处理相关工作。因工作中使用的三维处理库为公司内部库,不具有普遍适用性,遂自学开源PCL库及其相关数学知识以备使用。谨此将自学过程与君共享。 博主才疏学浅,尚不具有指导能力,如有问题还请各位在评论处留言供大家共同讨论。
|