图片的几何变换
1. 图片剪切
python中通过切片的方式就可以截取图片矩阵
import cv2
img = cv2.imread('./lena.jpg', cv2.IMREAD_UNCHANGED)
img1 = img[180:250, 180:310]
cv2.imshow('img', img)
cv2.imshow('img1', img1)
cv2.waitKey()
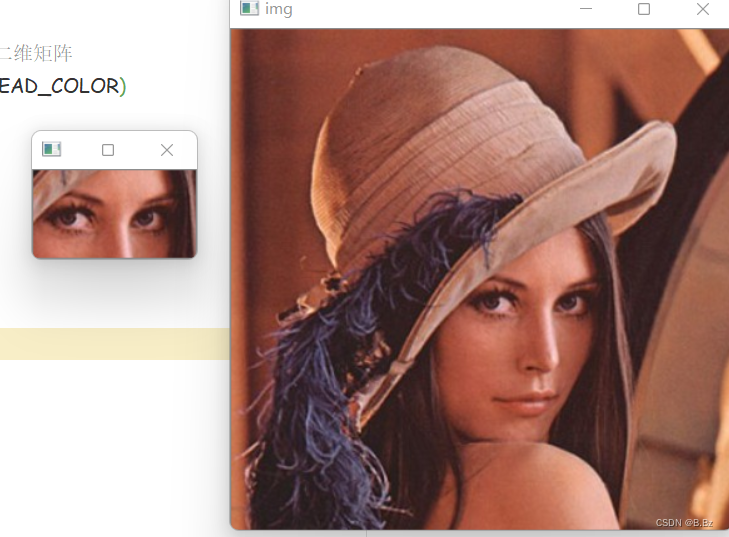
2. 图片镜像处理
import cv2
import numpy as np
img = cv2.imread('./lena.jpg')
height, width = img.shape[0:2]
new_img = np.zeros((height, width * 2, 3), np.uint8)
for row in range(height):
for col in range(width):
new_img[row, col] = img[row, col]
new_img[row, width * 2 - col - 1] = img[row, col]
cv2.imshow('img', img)
cv2.imshow('img1', new_img)
cv2.waitKey()
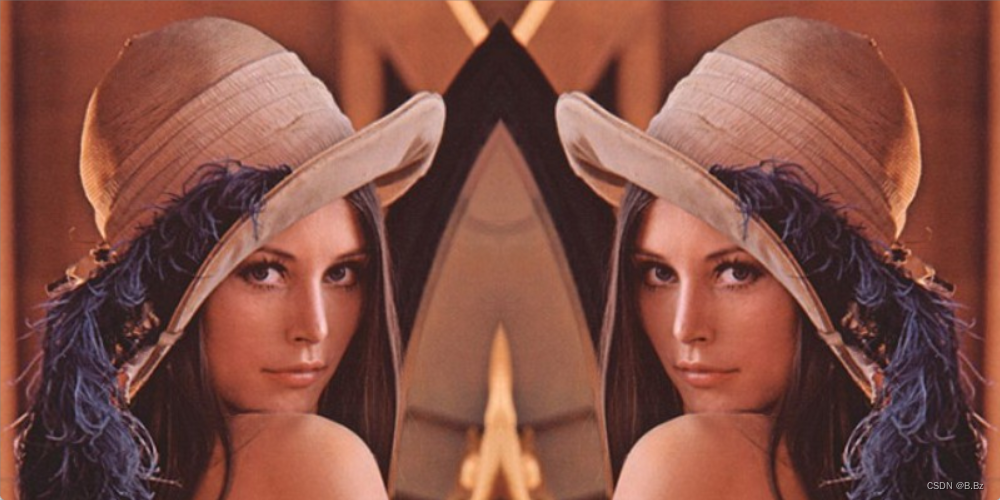
3. 图片缩放
resize方法:
- 参数1为图片矩阵
- 参数2为元组,放大或缩放后的高度和宽度
import cv2
img = cv2.imread('./lena.jpg')
height, width = img.shape[0:2]
new_height = int(height * 0.5)
new_width = int(width * 0.5)
new_img = cv2.resize(img, (new_height, new_width))
cv2.imshow('img', img)
cv2.imshow('new_img', new_img)
cv2.waitKey()
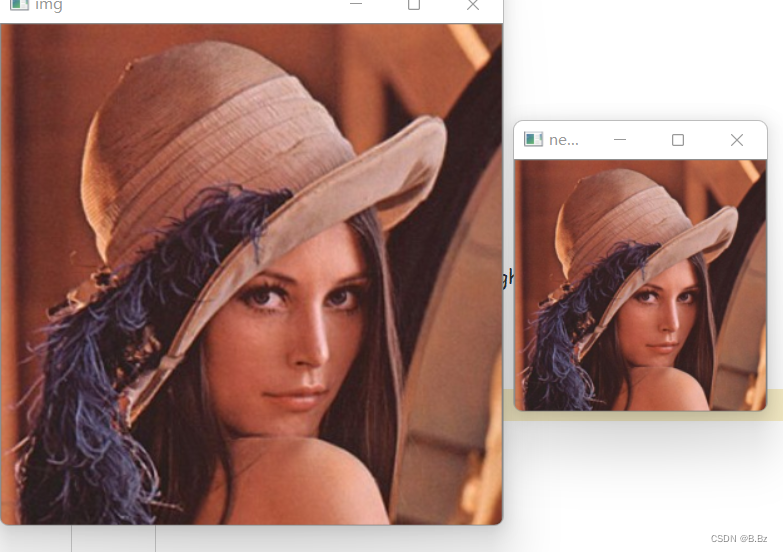
图片操作原理
在计算机程序中,其实是用矩阵来进行描述的,如果我们想对这张图片进行操作,其实就是要对矩阵进行运算。
常见的变换矩阵:
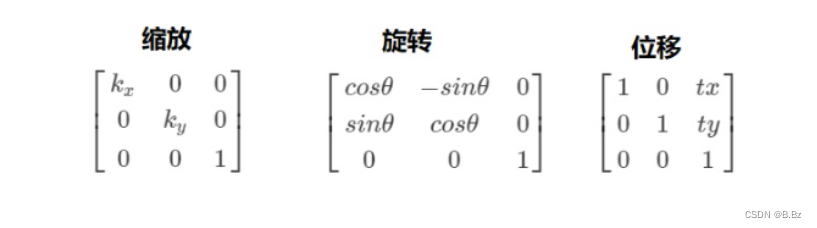
4. 图片位移
变换矩阵根据上图代入进去运算的 使用warpAffine方法使用变换矩阵
- 参数1为
图片矩阵 - 参数2为
变换矩阵 - 参数3为原图片的
宽度 和高度 组成的元组
import cv2
import numpy as np
img = cv2.imread('./lena.jpg')
height, width = img.shape[0:2]
new_height = int(height * 1)
new_width = int(width * 1)
matrixShift = np.float32([
[0.5, 0, width * 0.5 / 2],
[0, 0.5, height * 0.5 / 2]
])
shift_img = cv2.warpAffine(img, matrixShift, (width, height))
cv2.imshow('img', img)
cv2.imshow('shift_img', shift_img)
cv2.waitKey()
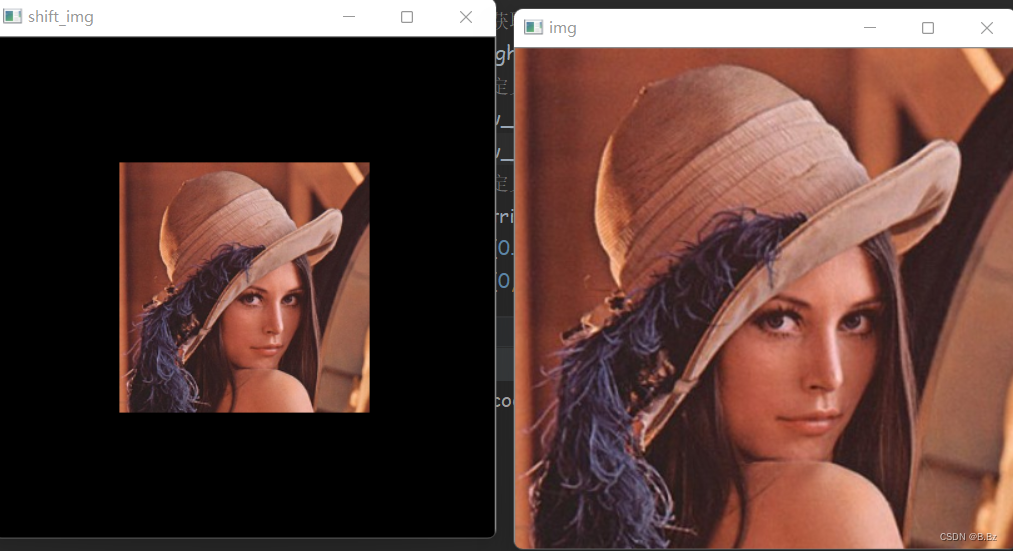
5. 图片旋转
cv2给定apigetRotationMatrix2D() :
参数1:旋转中心点
参数2:旋转度数
参数3:缩放倍数
import cv2
img = cv2.imread('./lena.jpg')
height, width = img.shape[0:2]
M = cv2.getRotationMatrix2D((width / 2, height / 2), 45, 0.5)
new_img = cv2.warpAffine(img, M, (width, height))
cv2.imshow('img', new_img)
cv2.waitKey()
6. 图片仿射变换
import numpy as np
import cv2
img = cv2.imread('./img/itheima.jpg')
height, width = img.shape[0:2]
matrixSrc = np.float32([[0, 0], [0, height - 1], [width - 1, 0]])
matrixDst = np.float32([[50, 100], [300, height - 200], [width - 300, 100]])
matrixAffine = cv2.getAffineTransform(matrixSrc, matrixDst)
new_img = cv2.warpAffine(img, matrixAffine, (width, height))
cv2.imshow('img', new_img)
cv2.waitKey()
7. 图像金字塔
一幅图片的金字塔是一系列以金字塔形状排列的,分辨率逐步降低,而且源于同一张原始图的图像集合。其通过梯次向下采样获得,直到达到某个种植条件才停止采样 。层级越高,图像越小,分辨率越低。上采样则相反。
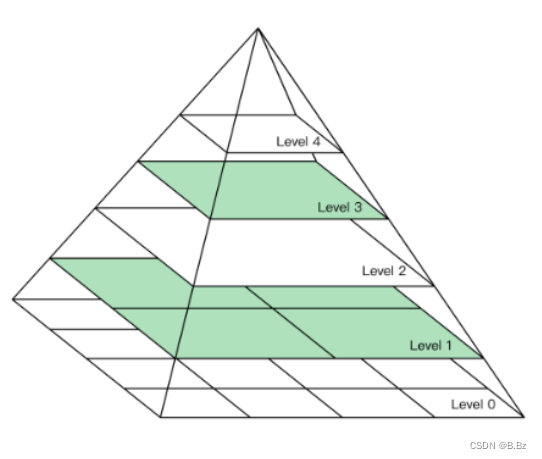
import cv2
cv2.pyrDown(img)
import cv2
cv2.pyrUp(img)
采样和resize()方法是有区别的:
resize方法把图像缩放,会越来越糊,有马赛克一样的小方块,但是往下采样只是分辨率降低,变得模糊,不会出现小方块,还是可以看得见轮廓。
|