需要安装dlib,参考:dlib安装参坑记录 本人对于人工智能属于零基础,所以以下内容是在网上查阅的资料并整理出来的。 我想干啥:我想实现人脸识别。 需要做啥:人脸检测、人脸识别 人脸检测怎么做? 查阅到可以使用Haar特征分类器或dlib检测方法。 一开始使用Haar特征分类器时,发现很多方法我都使用不了。不知道是不是版本的原因。 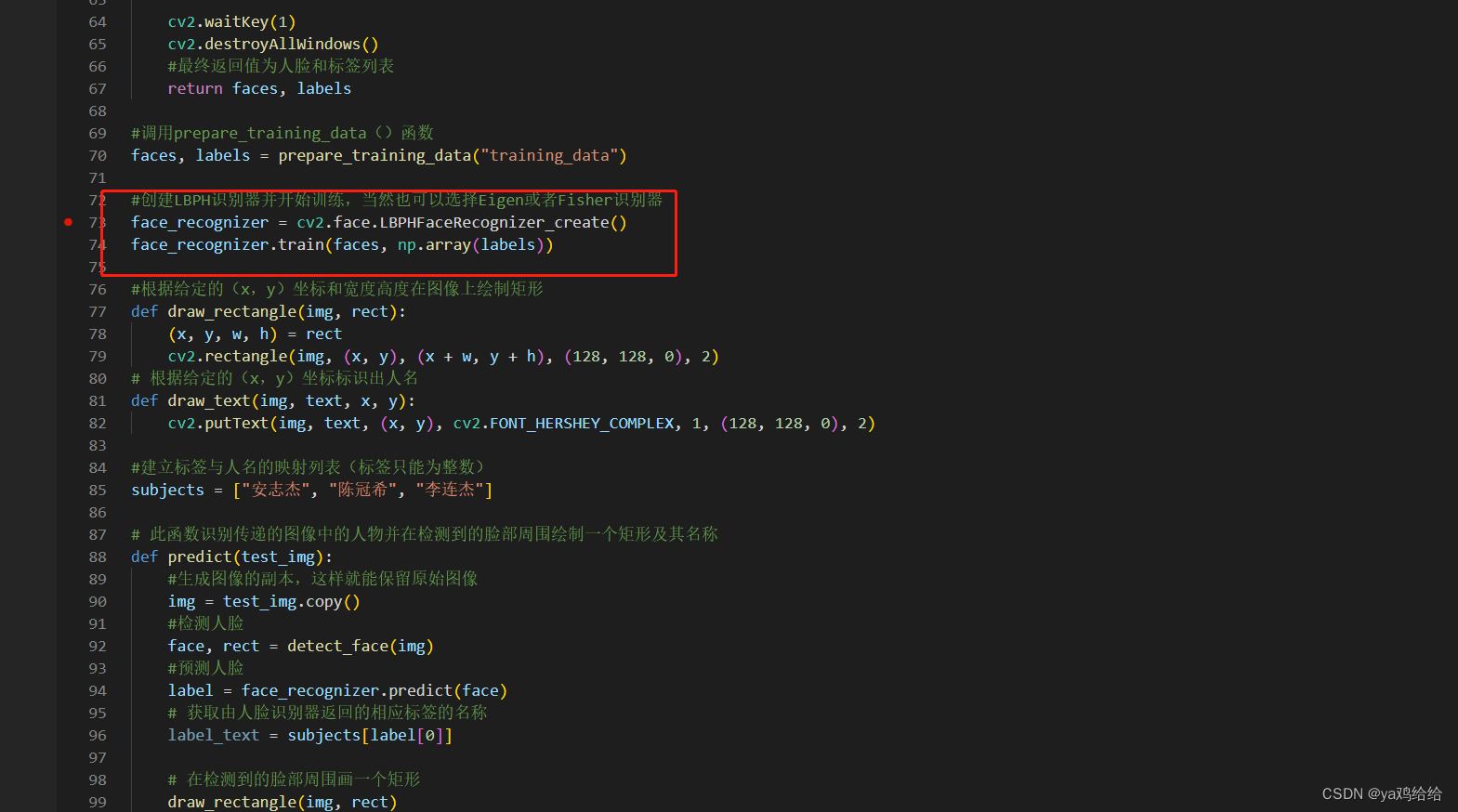
所以换到了dlib检测方法。 大概需要如下几步: a、灰度处理
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
b、获得dlib的正面人脸检测器
detector = dlib.get_frontal_face_detector()
c、进行检测
rects = detector(gray_img)
d、在图片中圈出人脸部分
cv2.rectangle(img,(x1,y1),(x2,y2),(0,252,0),2)
e、对步骤d中圈出的框进行文字描述
cv2.putText(img=image, text="Face: {}".format(i + 1), org=(x-10, y-10), fontFace=cv2.FONT_HERSHEY_SIMPLEX, fontScale=0.5, color=(0, 255, 0), thickness=2)
具体代码如下:
import dlib
import numpy as np
import cv2
def rect_to_bb(rect):
x1 = rect.left()
y1 = rect.top()
x2 = rect.right()
y2 = rect.bottom()
return (x1, y1, x2, y2)
def detect_face(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
detector = dlib.get_frontal_face_detector()
rects = detector(gray,1)
for (i, rect) in enumerate(rects):
(x, y, x2, y2) = rect_to_bb(rect)
cv2.rectangle(image, (x, y), (x2, y2), (0, 255, 0), 2)
cv2.putText(img=image, text="Face: {}".format(i + 1), org=(x-10, y-10), fontFace=cv2.FONT_HERSHEY_SIMPLEX, fontScale=0.5, color=(0, 255, 0), thickness=2)
cv2.imshow("Output", image)
cv2.waitKey(0)
if __name__ == "__main__":
test_img5 = cv2.imread("test_data/test5.jpg")
detect_face(test_img5)
原文参考自:https://blog.csdn.net/liuxiao214/article/details/83411820
|