一、项目描述
二、代码详解
2.1 预定义参数
-
导包 import os
import cv2
import argparse
import numpy as np
from imutils import contours
-
设置参数
- 图像路径参数
def parse():
"""设置自己的参数"""
parser = argparse.ArgumentParser(description="set your identity parameters")
parser.add_argument("-i", "--image", default="./images", type=str, help="path to input image")
parser.add_argument("-t", "--template", default="./ocr_a_reference.png", type=str,
help="path to template OCR-A image")
opt = parser.parse_args()
return opt
- 信用卡类别参数
FIRST_NUMBER = {
"3": "American Express",
"4": "Visa",
"5": "MasterCard",
"6": "Discover Card"
}
2.2 辅助函数
- 绘图
def cv_show(name, img):
"""绘图,避免重复造轮子"""
cv2.imshow(name, img)
cv2.waitKey(0)
cv2.destroyAllWindows()
- 轮廓排序(同imutils.contours.sort_contours())
def sort_contours(cnts, method="left-to-right"):
"""对轮廓进行排序"""
reverse = False
i = 0
if method == "right-to-left" or method == "bottom-to-top":
reverse = True
if method == "top-to-bottom" or method == "bottom-to-top":
i = 1
boundingBoxes = [cv2.boundingRect(c) for c in cnts]
(cnts, boundingBoxes) = zip(*sorted(zip(cnts, boundingBoxes),
key=lambda b: b[1][i],
reverse=reverse))
return cnts, boundingBoxes
- 缩放图像尺寸
def resize(image, width=None, height=None, inter=cv2.INTER_AREA):
"""根据自定的宽/高进行等比例缩放图像"""
dim = None
h, w = image.shape[:2]
if width is None and height is None:
return image
if width is None:
r = height / float(h)
dim = (int(w * r), height)
else:
r = width / float(w)
dim = (width, int(h * r))
resized = cv2.resize(image, dim, interpolation=inter)
return resized
2.3 模板图像处理
-
模板图像  -
主要步骤:
- 读取模板图像,并转换为灰度图
img = cv2.imread(opt.template)
ref = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)

- 将模板图像的灰度图二值化
ref = cv2.threshold(ref, 10, 255, cv2.THRESH_BINARY_INV)[1]

- 寻找二值图中的每个外轮廓
refCnts, hierarchy = cv2.findContours(ref.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)

- 对所有外轮廓进行排序。从左到右,从上到下
refCnts = sort_contours(refCnts, method="left-to-right")[0]
- 依次遍历每一个轮廓,并存储每个类别模板
digits = {}
for i, c in enumerate(refCnts):
(x, y, w, h) = cv2.boundingRect(c)
roi = ref[y:y + h, x:x + w]
roi = cv2.resize(roi, (57, 88))
digits[i] = roi
-
代码实现 def deal_template(opt):
"""预处理模板图像,将每个类别的模板图像保存在字典中"""
img = cv2.imread(opt.template)
ref = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ref = cv2.threshold(ref, 10, 255, cv2.THRESH_BINARY_INV)[1]
refCnts, hierarchy = cv2.findContours(ref.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(img, refCnts, -1, (0, 0, 255), 3)
print(np.array(refCnts, dtype=object).shape)
refCnts = sort_contours(refCnts, method="left-to-right")[0]
digits = {}
for i, c in enumerate(refCnts):
(x, y, w, h) = cv2.boundingRect(c)
roi = ref[y:y + h, x:x + w]
roi = cv2.resize(roi, (57, 88))
digits[i] = roi
return digits
2.4 识别信用卡图像
-
信用卡图像 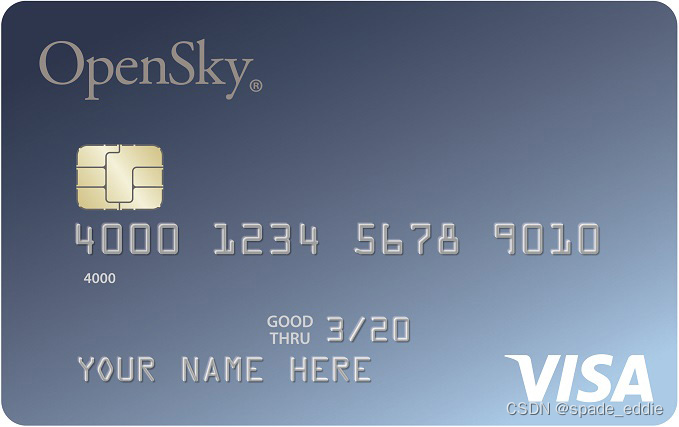 -
主要步骤
- 将待识别图像进行缩放并转换为灰度图
image = cv2.imread(img_path)
image = resize(image, width=300)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
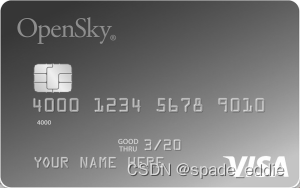
- 对灰度图进行礼帽操作,突出更明亮的区域
tophat = cv2.morphologyEx(gray, cv2.MORPH_TOPHAT, rectKernel)
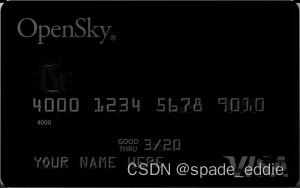
- 利用sobel算子进行x方向边缘检测(y方向效果不好),取绝对值并归一化
gradX = cv2.Sobel(tophat, ddepth=cv2.CV_32F, dx=1, dy=0, ksize=-1)
gradX = np.absolute(gradX)
minVal, maxVal = np.min(gradX), np.max(gradX)
gradX = (255 * ((gradX - minVal) / (maxVal - minVal)))
gradX = gradX.astype("uint8")
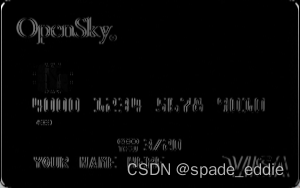
- 通过闭运算(先膨胀,再腐蚀)将sobel检测的文字边缘连在一起
gradX = cv2.morphologyEx(gradX, cv2.MORPH_CLOSE, rectKernel)
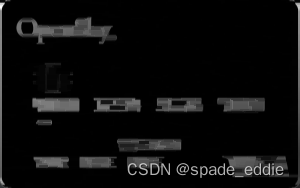
- 二值化图像,使用THRESH_OTSU自动寻找合适的阈值
thresh = cv2.threshold(gradX, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
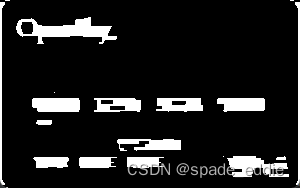
- 对二值图像进行闭运算,合并连接区域
thresh = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, sqKernel)
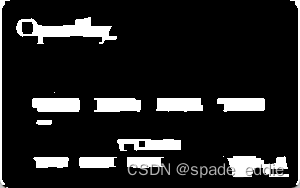
- 查找二值图像中的轮廓
threshCnts, hierarchy = cv2.findContours(thresh.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnts = threshCnts
cur_img = image.copy()
cv2.drawContours(cur_img, cnts, -1, (0, 0, 255), 3)
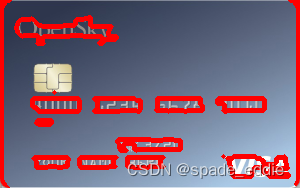
- 根据先验长宽信息,保留符合识别区域的轮廓(区域的最小外接矩),并将轮廓从左到右排序
locs = []
for (i, c) in enumerate(cnts):
(x, y, w, h) = cv2.boundingRect(c)
ar = w / float(h)
if 2.5 < ar < 4.0:
if 40 < w < 55 and 10 < h < 20:
locs.append((x, y, w, h))
locs = sorted(locs, key=lambda x: x[0])
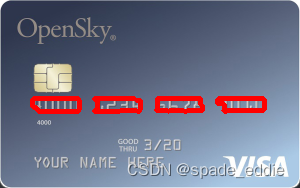 9. 遍历轮廓中的每个数字,进行模板匹配 output = []
for i, (gX, gY, gW, gH) in enumerate(locs):
groupOutput = []
group = gray[gY - 5:gY + gH + 5, gX - 5:gX + gW + 5]
group = cv2.threshold(group, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
digitCnts, hierarchy = cv2.findContours(group.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
digitCnts = contours.sort_contours(digitCnts, method="left-to-right")[0]
for c in digitCnts:
(x, y, w, h) = cv2.boundingRect(c)
roi = group[y:y + h, x:x + w]
roi = cv2.resize(roi, (57, 88))
scores = []
for digit, digitROI in digits.items():
result = cv2.matchTemplate(roi, digitROI, cv2.TM_CCOEFF)
_, score, _, _ = cv2.minMaxLoc(result)
scores.append(score)
groupOutput.append(str(np.argmax(scores)))
cv2.rectangle(image, (gX - 5, gY - 5), (gX + gW + 5, gY + gH + 5), (0, 0, 255), 1)
cv2.putText(image, "".join(groupOutput), (gX, gY - 15), cv2.FONT_HERSHEY_SIMPLEX, 0.65, (0, 0, 255), 2)
output.extend(groupOutput)
cv_show("Image", image)
print("Credit Card Type: {}".format(FIRST_NUMBER[output[0]]))
print("Credit Card #: {}".format("".join(output)))
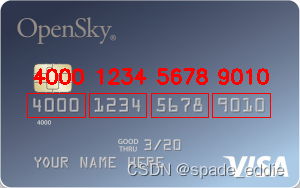 -
代码实现 def template_math(img_path):
image = cv2.imread(img_path)
image = resize(image, width=300)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
rectKernel = cv2.getStructuringElement(cv2.MORPH_RECT, (9, 3))
sqKernel = cv2.getStructuringElement(cv2.MORPH_RECT, (5, 5))
tophat = cv2.morphologyEx(gray, cv2.MORPH_TOPHAT, rectKernel)
gradX = cv2.Sobel(tophat, ddepth=cv2.CV_32F, dx=1, dy=0, ksize=-1)
gradX = np.absolute(gradX)
minVal, maxVal = np.min(gradX), np.max(gradX)
gradX = (255 * ((gradX - minVal) / (maxVal - minVal)))
gradX = gradX.astype("uint8")
print(np.array(gradX).shape)
gradX = cv2.morphologyEx(gradX, cv2.MORPH_CLOSE, rectKernel)
thresh = cv2.threshold(gradX, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
thresh = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, sqKernel)
threshCnts, hierarchy = cv2.findContours(thresh.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnts = threshCnts
cur_img = image.copy()
cv2.drawContours(cur_img, cnts, -1, (0, 0, 255), 3)
locs = []
for (i, c) in enumerate(cnts):
(x, y, w, h) = cv2.boundingRect(c)
ar = w / float(h)
if 2.5 < ar < 4.0:
if 40 < w < 55 and 10 < h < 20:
locs.append((x, y, w, h))
locs = sorted(locs, key=lambda x: x[0])
output = []
for i, (gX, gY, gW, gH) in enumerate(locs):
groupOutput = []
group = gray[gY - 5:gY + gH + 5, gX - 5:gX + gW + 5]
group = cv2.threshold(group, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
digitCnts, hierarchy = cv2.findContours(group.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
digitCnts = contours.sort_contours(digitCnts, method="left-to-right")[0]
for c in digitCnts:
(x, y, w, h) = cv2.boundingRect(c)
roi = group[y:y + h, x:x + w]
roi = cv2.resize(roi, (57, 88))
scores = []
for digit, digitROI in digits.items():
result = cv2.matchTemplate(roi, digitROI, cv2.TM_CCOEFF)
_, score, _, _ = cv2.minMaxLoc(result)
scores.append(score)
groupOutput.append(str(np.argmax(scores)))
cv2.rectangle(image, (gX - 5, gY - 5), (gX + gW + 5, gY + gH + 5), (0, 0, 255), 1)
cv2.putText(image, "".join(groupOutput), (gX, gY - 15), cv2.FONT_HERSHEY_SIMPLEX, 0.65, (0, 0, 255), 2)
output.extend(groupOutput)
cv_show("Image", image)
print("Credit Card Type: {}".format(FIRST_NUMBER[output[0]]))
print("Credit Card #: {}".format("".join(output)))
return output
三、项目完整代码
import os
import cv2
import argparse
import numpy as np
from imutils import contours
def parse():
"""设置自己的参数"""
parser = argparse.ArgumentParser(description="set your identity parameters")
parser.add_argument("-i", "--image", default="./images", type=str, help="path to input image")
parser.add_argument("-t", "--template", default="./ocr_a_reference.png", type=str,
help="path to template OCR-A image")
opt = parser.parse_args()
return opt
def cv_show(name, img):
"""绘图,避免重复造轮子"""
cv2.imshow(name, img)
cv2.waitKey(0)
cv2.destroyAllWindows()
def sort_contours(cnts, method="left-to-right"):
"""对轮廓进行排序"""
reverse = False
i = 0
if method == "right-to-left" or method == "bottom-to-top":
reverse = True
if method == "top-to-bottom" or method == "bottom-to-top":
i = 1
boundingBoxes = [cv2.boundingRect(c) for c in cnts]
(cnts, boundingBoxes) = zip(*sorted(zip(cnts, boundingBoxes),
key=lambda b: b[1][i],
reverse=reverse))
return cnts, boundingBoxes
def resize(image, width=None, height=None, inter=cv2.INTER_AREA):
"""根据自定的宽/高进行等比例缩放图像"""
dim = None
h, w = image.shape[:2]
if width is None and height is None:
return image
if width is None:
r = height / float(h)
dim = (int(w * r), height)
else:
r = width / float(w)
dim = (width, int(h * r))
resized = cv2.resize(image, dim, interpolation=inter)
return resized
def deal_template(opt):
"""预处理模板图像,将每个类别的模板图像保存在字典中"""
img = cv2.imread(opt.template)
ref = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ref = cv2.threshold(ref, 10, 255, cv2.THRESH_BINARY_INV)[1]
refCnts, hierarchy = cv2.findContours(ref.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(img, refCnts, -1, (0, 0, 255), 3)
print(np.array(refCnts, dtype=object).shape)
refCnts = sort_contours(refCnts, method="left-to-right")[0]
digits = {}
for i, c in enumerate(refCnts):
(x, y, w, h) = cv2.boundingRect(c)
roi = ref[y:y + h, x:x + w]
roi = cv2.resize(roi, (57, 88))
digits[i] = roi
return digits
def template_math(img_path):
image = cv2.imread(img_path)
image = resize(image, width=300)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv_show("image", image)
rectKernel = cv2.getStructuringElement(cv2.MORPH_RECT, (9, 3))
sqKernel = cv2.getStructuringElement(cv2.MORPH_RECT, (5, 5))
tophat = cv2.morphologyEx(gray, cv2.MORPH_TOPHAT, rectKernel)
gradX = cv2.Sobel(tophat, ddepth=cv2.CV_32F, dx=1, dy=0, ksize=-1)
gradX = np.absolute(gradX)
minVal, maxVal = np.min(gradX), np.max(gradX)
gradX = (255 * ((gradX - minVal) / (maxVal - minVal)))
gradX = gradX.astype("uint8")
print(np.array(gradX).shape)
gradX = cv2.morphologyEx(gradX, cv2.MORPH_CLOSE, rectKernel)
thresh = cv2.threshold(gradX, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
thresh = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, sqKernel)
threshCnts, hierarchy = cv2.findContours(thresh.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnts = threshCnts
cur_img = image.copy()
cv2.drawContours(cur_img, cnts, -1, (0, 0, 255), 3)
locs = []
for (i, c) in enumerate(cnts):
(x, y, w, h) = cv2.boundingRect(c)
ar = w / float(h)
if 2.5 < ar < 4.0:
if 40 < w < 55 and 10 < h < 20:
locs.append((x, y, w, h))
locs = sorted(locs, key=lambda x: x[0])
output = []
for i, (gX, gY, gW, gH) in enumerate(locs):
groupOutput = []
group = gray[gY - 5:gY + gH + 5, gX - 5:gX + gW + 5]
group = cv2.threshold(group, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
digitCnts, hierarchy = cv2.findContours(group.copy(), cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
digitCnts = contours.sort_contours(digitCnts, method="left-to-right")[0]
for c in digitCnts:
(x, y, w, h) = cv2.boundingRect(c)
roi = group[y:y + h, x:x + w]
roi = cv2.resize(roi, (57, 88))
scores = []
for digit, digitROI in digits.items():
result = cv2.matchTemplate(roi, digitROI, cv2.TM_CCOEFF)
_, score, _, _ = cv2.minMaxLoc(result)
scores.append(score)
groupOutput.append(str(np.argmax(scores)))
cv2.rectangle(image, (gX - 5, gY - 5), (gX + gW + 5, gY + gH + 5), (0, 0, 255), 1)
cv2.putText(image, "".join(groupOutput), (gX, gY - 15), cv2.FONT_HERSHEY_SIMPLEX, 0.65, (0, 0, 255), 2)
output.extend(groupOutput)
cv_show("Image", image)
print("Credit Card Type: {}".format(FIRST_NUMBER[output[0]]))
print("Credit Card #: {}".format("".join(output)))
return output
if __name__ == '__main__':
FIRST_NUMBER = {
"3": "American Express",
"4": "Visa",
"5": "MasterCard",
"6": "Discover Card"
}
opt = parse()
digits = deal_template(opt)
for basename in os.listdir(opt.image):
image_path = opt.image + "/" + basename
output = template_math(image_path)
|