1.图像基础
1.1基本概念
(1)像素:计算机屏幕上所能显示的最小单位,用来表示图像的单位
(2)RGB:R:red,G:green,B:Blue,范围:0~255
1.2基本操作
- 读取图片:cv2.imread()
- 读取图片的形状:img.shape,返回一个(rows,height,channels)
- 获取图片的大小:img.size,返回一个rowsXheightXchannels
- 显示图片:cv2.imshow(“名称”,img)
- 等待:cv2.waitKey(0)
- 关闭:cv2.destroyAllWindows()
import cv2 as cv
img = cv.imread("image/kids.jpg")
print(img.shape)
print(img.size)
(b,g,r) = img[6,40]
print(b,g,r)
cv.imshow("图片",img)
cv.waitKey(0)
cv.destroyAllWindows()
1.3灰度图片操作
- 读取图片:cv2.imread(img,cv2.IMREAD_GRAYSCALE)
1.4BGR顺序
import cv2 as cv
import matplotlib.pyplot as plt
img1 = cv.imread("image/kids.jpg")
b,g,r = cv.split(img1)
img2 = cv.merge([r,g,b])
plt.subplot(121)
plt.imshow(img1)
plt.subplot(122)
plt.imshow(img2)
plt.show()
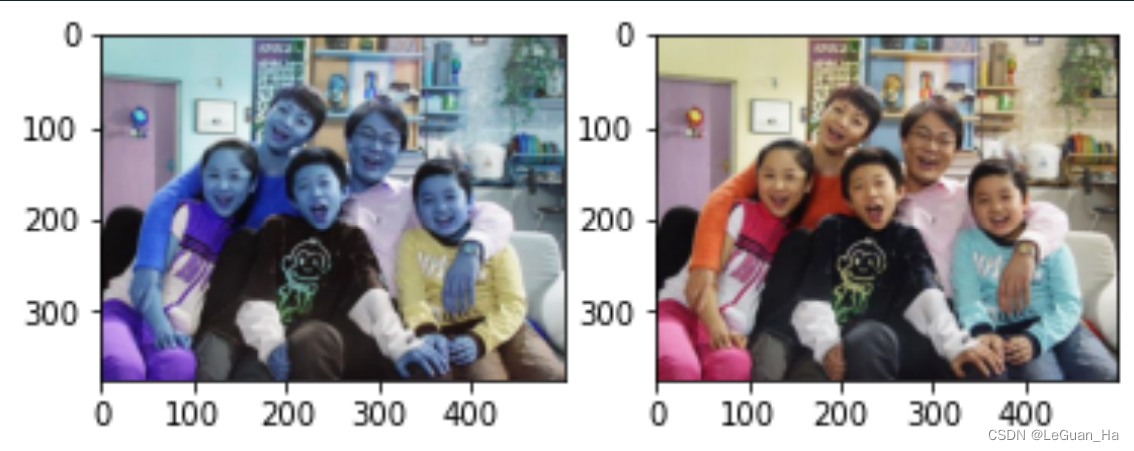
2.图像操作
2.1读取图片
import cv2 as cv
img = cv.imread("image/kids.jpg")
cv.imshow("LOGO", img)
cv.waitKey(0)
cv.destroyAllWindows()
2.2读取、处理、保存图片
import cv2 as cv
import matplotlib.pyplot as plt
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("img_input", help="read one image")
parser.add_argument("img_output", help="save the process image")
args = vars(parser.parse_args())
img = cv.imread(args["img_input"])
img_gray = cv.cvtColor(img, cv.COLOR_BGR2GRAY)
cv.imwrite(args["img_output"], img_gray)
cv.imshow("Original Image", img)
cv.imshow("Gray Image", img_gray)
cv.waitKey(0)
cv.destroyAllWindows()
3.视频操作
3.1从摄像头读取视频
import cv2 as cv
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("index_camera", help="the camera ID", type=int)
args = parser.parse_args()
print("the camera index:", args.index_camera)
capture = cv.VideoCapture(args.index_camera)
frame_width = capture.get(cv.CAP_PROP_FRAME_WIDTH)
frame_height = capture.get(cv.CAP_PROP_FRAME_HEIGHT)
fps = capture.get(cv.CAP_PROP_FPS)
print("帧的宽度:{}", format(frame_width))
print("帧的高度:{}", format(frame_height))
print("FPS: {}", format(fps))
if capture.isOpened() is False:
print("Camera Error!")
while capture.isOpened():
ret, frame = capture.read()
gray_frame = cv.cvtColor(frame, cv.COLOR_BGR2GRAY)
cv.imshow("frame", frame)
cv.imshow("gray frame", gray_frame)
if cv.waitKey(20) & 0xFF == ord("q"):
break
capture.release()
cv.destroyAllWindows()
3.2从视频文件读取
import cv2 as cv
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("video_path", help="the path to the video file")
args = parser.parse_args()
capture = cv.VideoCapture(args.video_path)
ret, frame = capture.read()
while ret:
cv.imshow("video", frame)
ret, frame = capture.read()
if cv.waitKey(20) & 0xFF == ord('q'):
break
capture.release()
cv.destroyAllWindows()
3.3保存摄像头读取到的视频
import cv2 as cv
import argparse
parser = argparse.ArgumentParser()
parser.add_argument("video_output", help="the path to the output video")
args = parser.parse_args()
capture = cv.VideoCapture(0)
if capture.isOpened() is False:
print("Camera Error")
frame_width = capture.get(cv.CAP_PROP_FRAME_WIDTH)
frame_height = capture.get(cv.CAP_PROP_FRAME_HEIGHT)
fps = capture.get(cv.CAP_PROP_FPS)
fourcc = cv.VideoWriter_fourcc(*"XVID")
output_gray = cv.VideoWriter(args.video_output, fourcc, int(fps), (int(frame_width), int(frame_height)), False)
while capture.isOpened():
ret, frame = capture.read()
if ret is True:
gray_frame = cv.cvtColor(frame, cv.COLOR_BGR2GRAY)
output_gray.write(gray_frame)
cv.imshow("gray", gray_frame)
if cv.waitKey(1) & 0xFF == ord('q'):
break
else:
break
capture.release()
output_gray.release()
cv.destroyAllWindows()
4.图像变换
4.1图像的放大、缩小
import matplotlib.pyplot as plt
import cv2
import matplotlib
matplotlib.use('TkAgg')
img = cv2.imread("image/kids.jpg")
plt.imshow(img)
height, width, channel = img.shape
print(height, width, channel)
resized_img = cv2.resize(img, (width * 2, height * 2), interpolation=cv2.INTER_LINEAR)
plt.imshow(resized_img)
small_img = cv2.resize(img, None, fx=0.5, fy=0.5, interpolation=cv2.INTER_LINEAR)
plt.imshow(small_img)
4.2图片平移
import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
img = cv2.imread("image/kids.jpg")
height, width = img.shape[:2]
M1 = np.float32([[1, 0, 100], [0, 1, 50]])
move_img = cv2.warpAffine(img, M1, (width, height))
plt.imshow(move_img)
plt.show()
4.3图像旋转
import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
img = cv2.imread("image/dogsp.jpeg")
height, width = img.shape[:2]
center = (width // 2, height // 2)
M3 = cv2.getRotationMatrix2D(center, 180, 1)
rotation_img = cv2.warpAffine(img, M3, (width, height))
plt.imshow(rotation_img)
plt.show()
4.4仿射变换
import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
img = cv2.imread("image/dogsp.jpeg")
height, width = img.shape[:2]
p1 = np.float32([[120, 35], [215, 45], [135, 120]])
p2 = np.float32([[135, 45], [300, 110], [130, 230]])
M4 = cv2.getAffineTransform(p1, p2)
trans_img = cv2.warpAffine(img, M4, (width, height))
plt.imshow(trans_img)
plt.show()
4.5图像裁剪
import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
img = cv2.imread("image/dogsp.jpeg")
crop_img = img[20:500,200:400]
plt.imshow(crop_img)
plt.show()
4.6位运算
-
与运算 import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
rectangle = np.zeros((300, 300), dtype='uint8')
rect_img = cv2.rectangle(rectangle, (25, 25), (275, 275), 255, -1)
rectangle = np.zeros((300, 300), dtype='uint8')
circle_img = cv2.circle(rectangle, (150, 150), 150, 255, -1)
and_img = cv2.bitwise_and(rect_img,circle_img)
plt.imshow(and_img)
plt.show()
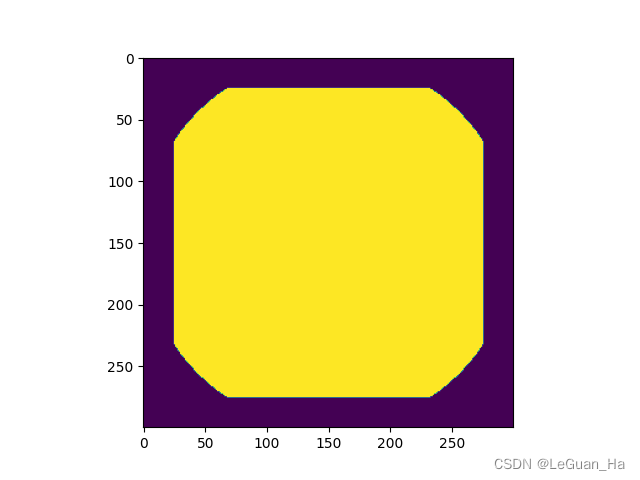
-
或运算 import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
rectangle = np.zeros((300, 300), dtype='uint8')
rect_img = cv2.rectangle(rectangle, (25, 25), (275, 275), 255, -1)
rectangle = np.zeros((300, 300), dtype='uint8')
circle_img = cv2.circle(rectangle, (150, 150), 150, 255, -1)
or_img = cv2.bitwise_or(rect_img,circle_img)
plt.imshow(or_img)
plt.show()
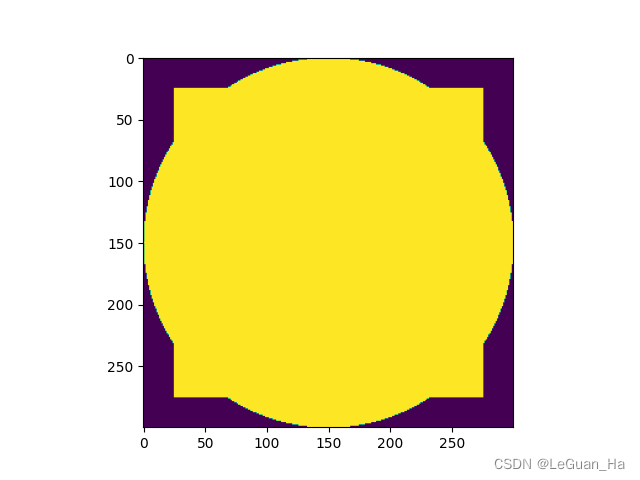
-
异或运算 import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
rectangle = np.zeros((300, 300), dtype='uint8')
rect_img = cv2.rectangle(rectangle, (25, 25), (275, 275), 255, -1)
rectangle = np.zeros((300, 300), dtype='uint8')
circle_img = cv2.circle(rectangle, (150, 150), 150, 255, -1)
xor_img = cv2.bitwise_xor(rect_img,circle_img)
plt.imshow(xor_img)
plt.show()
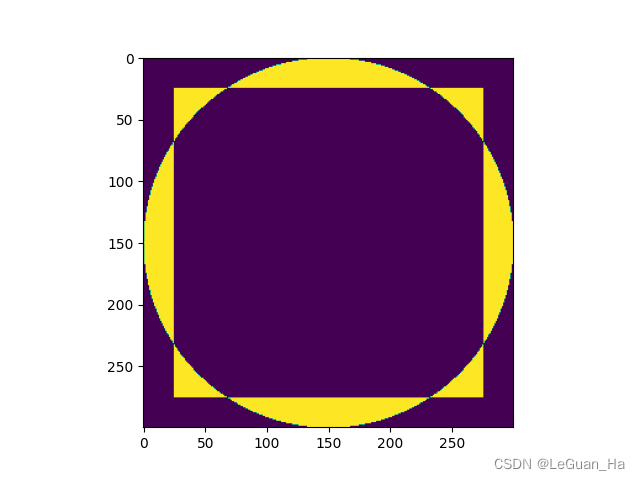
4.7图像分离与融合
import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
img = cv2.imread("image/kids.jpg")
(B,G,R) = cv2.split(img)
plt.imshow(B)
zeros = np.zeros(img.shape[:2],dtype='unit8')
plt.imshow(cv2.merge([zeros,zeros,R]))
plt.imshow(cv2.merge([B,zeros,zeros]))
4.8颜色空间
import matplotlib.pyplot as plt
import cv2
import matplotlib
import numpy as np
matplotlib.use('TkAgg')
img = cv2.imread("image/kids.jpg")
gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
plt.imshow(gray)
plt.show()
hsv = cv2.cvtColor(img,cv2.COLOR_BGR2HSV)
plt.imshow(hsv)
plt.show()
lab = cv2.cvtColor(img,cv2.COLOR_BGR2LAB)
plt.imshow(lab)
plt.show()
5.灰度直方图
- 直方图是图像中像素强度分布的图形表达方式
- 直方图统计了每一个强度值所具有的像素个数
cv2.calcHist(images,channels,mask,histSize,ranges)
- images:整数类型(unit8和float32)的原图(list形式表示)
- channels:通道的索引,例如[0]代表灰度图片
- mask:计算图片指定区域的直方图,如果mask为none,那么计算整张图片
- histSize(bins):每个色调值(范围:0~255)对应的像素数量/频率
- range:强度值的范围,[0,255]
import cv2
import cv2
import matplotlib.pyplot as plt
import numpy as np
def show_image(image, title, pos):
image_RGB = image[:, :, ::-1]
plt.title(title)
plt.subplot(2, 3, pos)
plt.imshow(image_RGB)
def show_histogram(hist, title, pos, color):
plt.title(title)
plt.subplot(2, 3, pos)
plt.xlabel("Bins")
plt.ylabel("Pixels")
plt.xlim([0, 256])
plt.plot(hist, color=color)
def main():
plt.figure(figsize=(15, 6))
plt.suptitle("Gray Image Histogram", fontsize=14, fontweight="bold")
img = cv2.imread("cat.jpeg")
img_gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
hist_image = cv2.calcHist([img_gray], [0], None, [256], [0, 256])
img_BGR = cv2.cvtColor(img_gray, cv2.COLOR_GRAY2BGR)
show_image(img_BGR, "BGR image", 1)
show_histogram(hist_image, "gray image histogram", 4, 'm')
plt.show()
if __name__ == '__main__':
main()
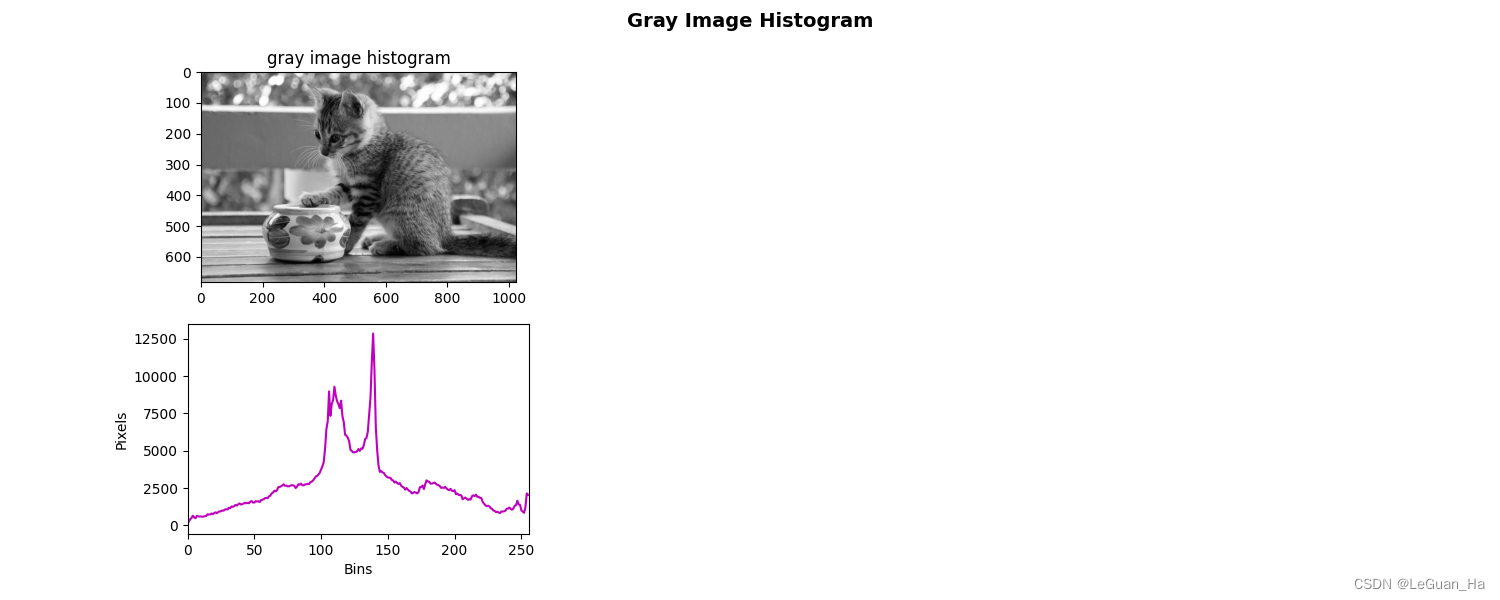
import cv2
import cv2
import matplotlib.pyplot as plt
import numpy as np
def show_image(image, title, pos):
image_RGB = image[:, :, ::-1]
plt.title(title)
plt.subplot(2, 3, pos)
plt.imshow(image_RGB)
def show_histogram(hist, title, pos, color):
plt.title(title)
plt.subplot(2, 3, pos)
plt.xlabel("Bins")
plt.ylabel("Pixels")
plt.xlim([0, 256])
plt.plot(hist, color=color)
def main():
plt.figure(figsize=(15, 6))
plt.suptitle("Gray Image Histogram", fontsize=14, fontweight="bold")
img = cv2.imread("cat.jpeg")
img_gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
hist_image = cv2.calcHist([img_gray], [0], None, [256], [0, 256])
img_BGR = cv2.cvtColor(img_gray, cv2.COLOR_GRAY2BGR)
show_image(img_BGR, "BGR image", 1)
show_histogram(hist_image, "gray image histogram", 4, 'm')
M = np.ones(img_gray.shape, np.uint8) * 50
added_img = cv2.add(img_gray, M)
added_img_hist = cv2.calcHist([added_img], [0], None, [256], [0, 256])
added_img_BGR = cv2.cvtColor(added_img, cv2.COLOR_GRAY2BGR)
show_image(added_img_BGR, "added histogram", 2)
show_histogram(added_img_hist, "added image hist", 5, "m")
subtract_img = cv2.subtract(img_gray, M)
subtract_img_hist = cv2.calcHist([subtract_img], [0], None, [256], [0, 256])
subtract_img_BGR = cv2.cvtColor(subtract_img, cv2.COLOR_GRAY2BGR)
show_image(subtract_img_BGR, "subtracted image", 3)
show_histogram(subtract_img_hist, "subtracted image hist", 6, 'm')
plt.show()
if __name__ == '__main__':
main()
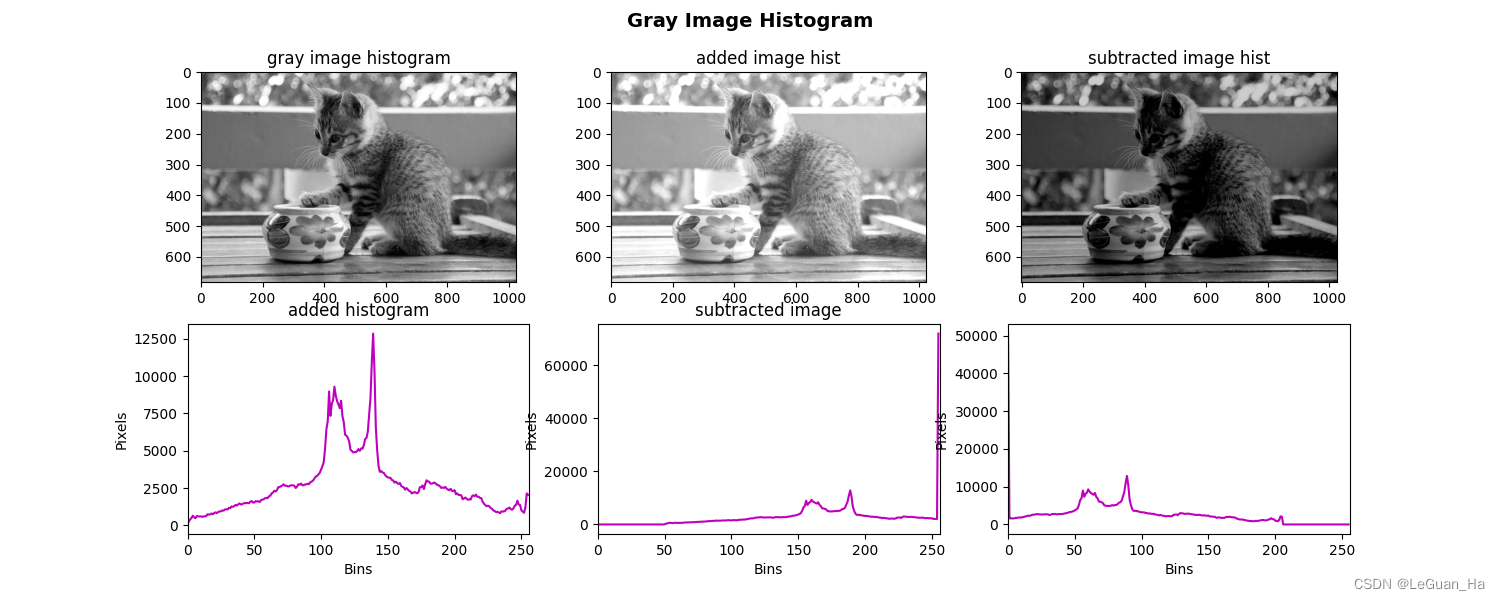
1.mask
import cv2
import matplotlib.pyplot as plt
import numpy as np
def show_image(image, title, pos):
img_RGB = image[:, :, ::-1]
plt.title(title)
plt.subplot(2, 2, pos)
plt.imshow(img_RGB)
def show_histogram(hist, title, pos, color):
plt.subplot(2, 2, pos)
plt.title(title)
plt.xlim([0, 256])
plt.plot(hist, color=color)
def main():
plt.figure(figsize=(12, 7))
plt.suptitle("Gray Image and Histogram with mask", fontsize=4, fontweight="bold")
img_gray = cv2.imread("cat.jpeg", cv2.COLOR_BGR2GRAY)
img_gray_hist = cv2.calcHist([img_gray], [0], None, [256], [0, 256])
show_image(img_gray, "image gray", 1)
show_histogram(img_gray_hist, "image gray histogram", 2, "m")
mask = np.zeros(img_gray.shape[:2], np.uint8)
mask[130:500, 600:1400] = 255
img_mask_hist = cv2.calcHist([img_gray], [0], mask, [256], [0, 256])
mask_img = cv2.bitwise_and(img_gray, img_gray, mask = mask)
show_image(mask_img, "gray image with mask", 3)
show_histogram(img_mask_hist, "histogram with masked gray image", 4, "m")
plt.show()
if __name__ == '__main__':
main()
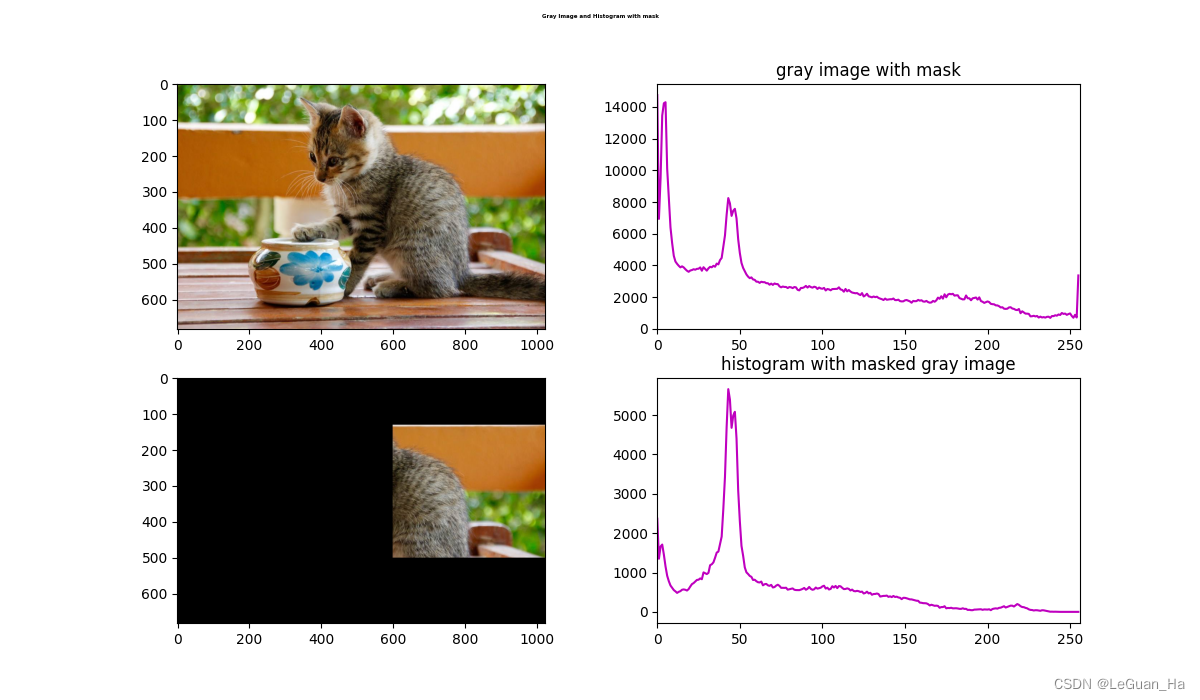
6.彩色直方图
import cv2
import matplotlib.pyplot as plt
import numpy as np
def show_image(image, title, pos):
plt.subplot(3, 2, pos)
plt.title(title)
image_RGB = image[:, :, ::-1]
plt.imshow(image_RGB)
plt.axis("off")
def show_histogram(hist, title, pos, color):
plt.subplot(3, 2, pos)
plt.title(title)
plt.xlim([0, 256])
for h, c in zip(hist, color):
plt.plot(h, color=c)
def calc_color_hist(image):
hist = []
hist.append(cv2.calcHist([image], [0], None, [256], [0, 256]))
hist.append(cv2.calcHist([image], [1], None, [256], [0, 256]))
hist.append(cv2.calcHist([image], [2], None, [256], [0, 256]))
return hist
def main():
plt.figure(figsize=(12, 8))
plt.suptitle("Color Histogram", fontsize=4, fontweight="bold")
img = cv2.imread("cat.jpeg")
img_hist = calc_color_hist(img)
show_image(img, "RGB Image", 1)
show_histogram(img_hist, "RGB Image Hist", 2, ('b', 'g', 'r'))
M = np.ones(img.shape, dtype="uint8") * 50
added_image = cv2.add(img, M)
added_image_hist = calc_color_hist(added_image)
show_image(added_image, 'added image', 3)
show_histogram(added_image_hist, 'added image hist', 4, ('b', 'g', 'r'))
subtracted_image = cv2.subtract(img, M)
subtracted_image_hist = calc_color_hist(subtracted_image)
show_image(subtracted_image, 'subtracted image', 5)
show_histogram(subtracted_image_hist, 'subtracted image hist', 6, ('b', 'g', 'r'))
plt.show()
if __name__ == '__main__':
main()
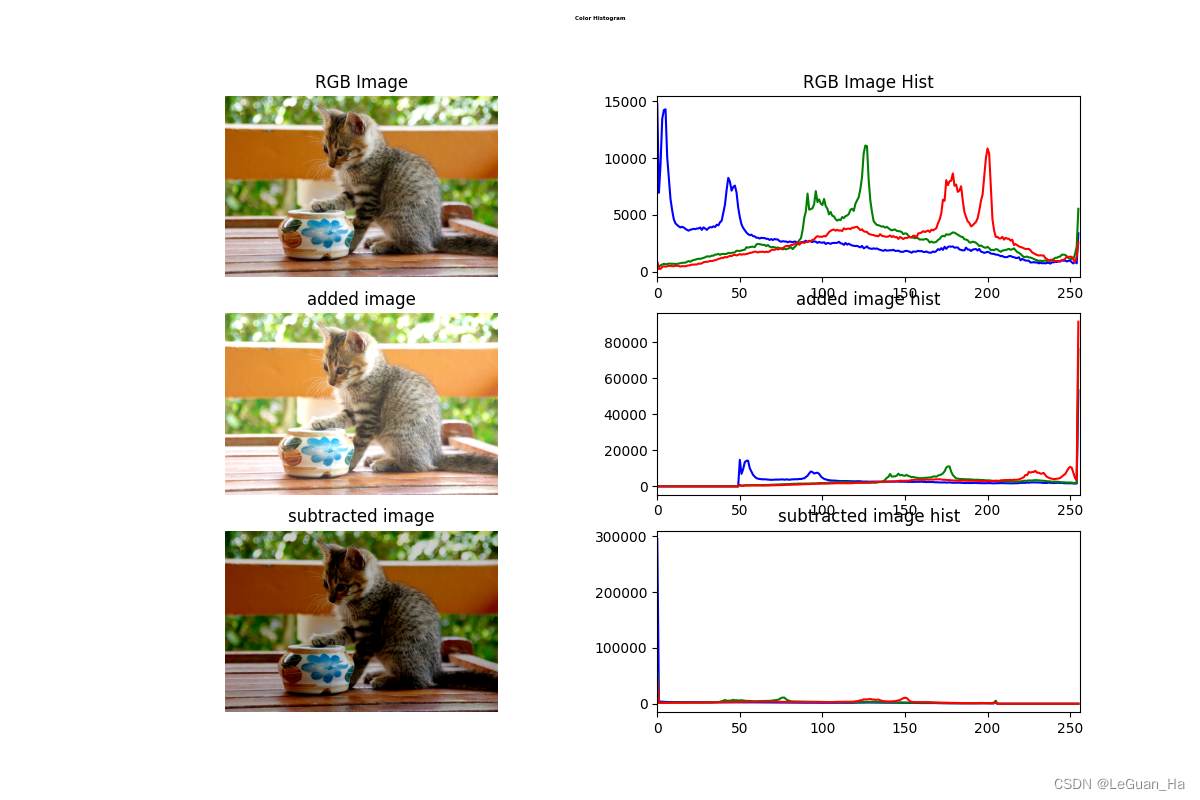
7.画出图形
import cv2
import numpy as np
import matplotlib.pyplot as plt
colors = {
'blue': (255, 0, 0),
'green': (0, 255, 0),
'red': (0, 0, 255),
'yellow': (0, 255, 255),
'white': (255, 255, 255)
}
def show_image(image, title):
img_RGB = image[:, :, ::-1]
plt.title(title)
plt.imshow(img_RGB)
plt.show()
1.直线
canvas = np.zeros((400, 400, 3), np.uint8)
canvas[:] = colors['white']
show_image(canvas, "Background")
cv2.line(canvas, (0, 0), (400, 400), colors['green'], 5)
cv2.line(canvas, (0, 400), (400, 0), colors['blue'], 5)
show_image(canvas, "cv2.line()")
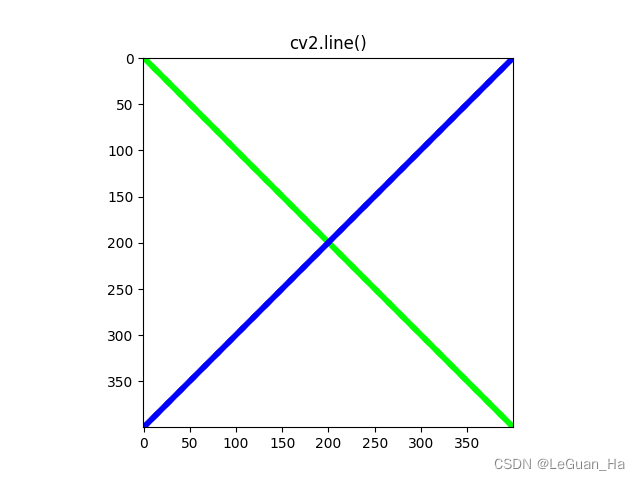
2.长方形
canvas = np.zeros((400, 400, 3), np.uint8)
canvas[:] = colors['white']
show_image(canvas, "Background")
cv2.rectangle(canvas, (10, 50), (70, 120), colors['green'], 3)
show_image(canvas, "cv2.rectangle()")
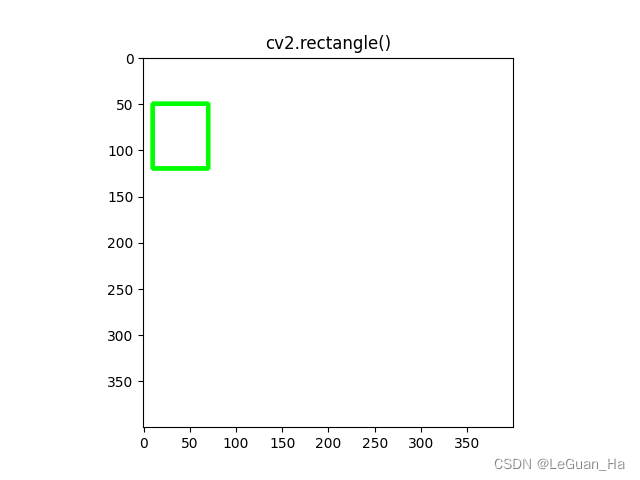
3.圆形
canvas = np.zeros((400, 400, 3), np.uint8)
canvas[:] = colors['white']
show_image(canvas, "Background")
cv2.circle(canvas, (200, 200), 100, colors['yellow'], 3)
show_image(canvas, "cv2.circle()")
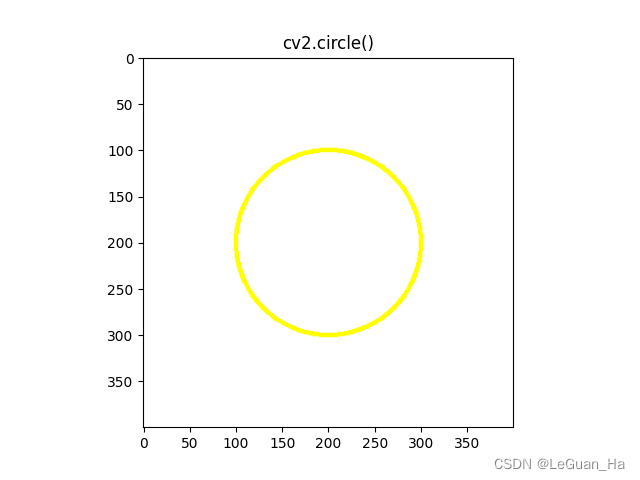
4.折线
canvas = np.zeros((400, 400, 3), np.uint8)
canvas[:] = colors['white']
show_image(canvas, "Background")
pts = np.array([[250, 5], [220, 80], [280, 80]], np.int32)
pts = pts.reshape((-1, 1, 2))
cv2.polylines(canvas, [pts], True, colors['green'], 3)
show_image(canvas, "cv2.polylines()")
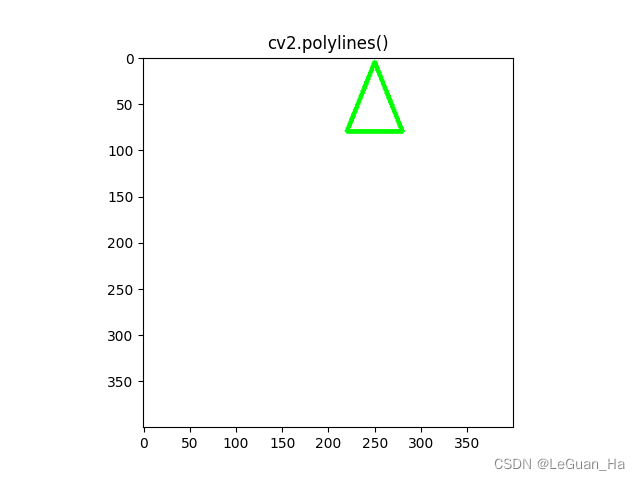
8.图片上显示文本
文本类型
- FONT_HERSHEY_SIMPLEX:正常大小无衬线字体
- FONT_HERSHEY_PLAIN:小号无衬线字体
- FONT_HERSHEY_DUPLEX:正常大小无衬线字体,比FONT_HERSHEY_SIMPLEX更复杂
- FONT_HERSHEY_COMPLEX:正常大小有衬线字体
- FONT_HERSHEY_TRIPLEX:正常大小有衬线字体,比FONT_HERSHEY_COMPLEX更复杂
- FONT_HERSHEY_COMPLEX_SMALL:FONT_HERSHEY_COMPLEX的小译本
- FONT_HERSHEY_SCRIPT_SIMPLEX:手写风格字体
- FONT_HERSHEY_SCRIPT_COMPLEX:手写风格字体
import matplotlib.pyplot as plt
import numpy as np
colors = {
'blue': (255, 0, 0),
'green': (0, 255, 0),
'red': (0, 0, 255),
'yellow': (0, 255, 255),
'white': (255, 255, 255)
}
def show_image(image, title):
image_RGB = image[:, :, ::-1]
plt.title(title)
plt.imshow(image_RGB)
plt.show()
canvas = np.zeros((400, 400, 3), np.uint8)
canvas.fill(255)
cv2.putText(canvas, "Hello World", (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, colors['red'], cv2.LINE_4)
cv2.putText(canvas, "NJTECH ", (50, 150), cv2.FONT_HERSHEY_SIMPLEX, 1, colors['red'], cv2.LINE_4)
show_image(canvas, "Canvas")
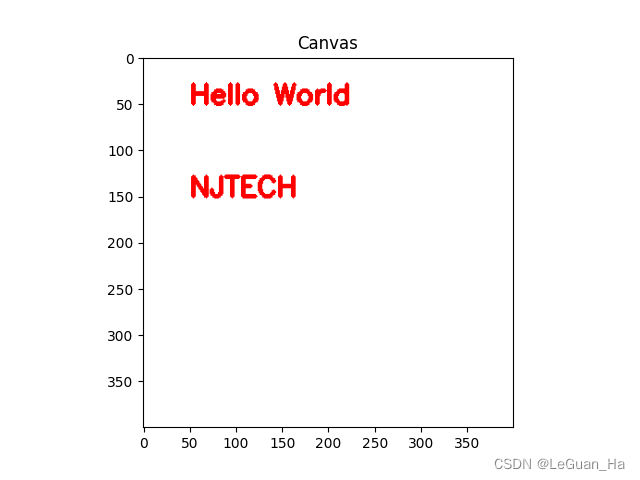
9.人脸识别
Haar Cascade哈尔级联
核心原理:
- 使用haar-like特征做检测
- Integral Image:积分图加速特征计算
- AdaBoost:选择关键特征,进行人脸和非人脸分类
- Cascade:级联,弱分类器称为强分类器
import cv2
import numpy as np
import matplotlib.pyplot as plt
def show_iamge(image, title, pos):
img_RGB = image[:,:,::-1]
plt.subplot(2, 2, pos)
plt.title(title)
plt.imshow(img_RGB)
plt.axis("off")
def plot_rectangle(image, faces):
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 3)
return image
def main():
image = cv2.imread("family.jpg")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
face_alt2 = cv2.CascadeClassifier("haarcascade_frontalface_alt2.xml")
face_alt2_detect = face_alt2.detectMultiScale(gray)
face_alt2_result = plot_rectangle(image.copy(), face_alt2_detect)
plt.figure(figsize=(9, 6))
plt.suptitle("Face detection with Haar Cascade", fontsize=14, fontweight="bold")
show_iamge(face_alt2_result, "face_alt2", 1)
plt.show()
if __name__ == '__main__':
main()
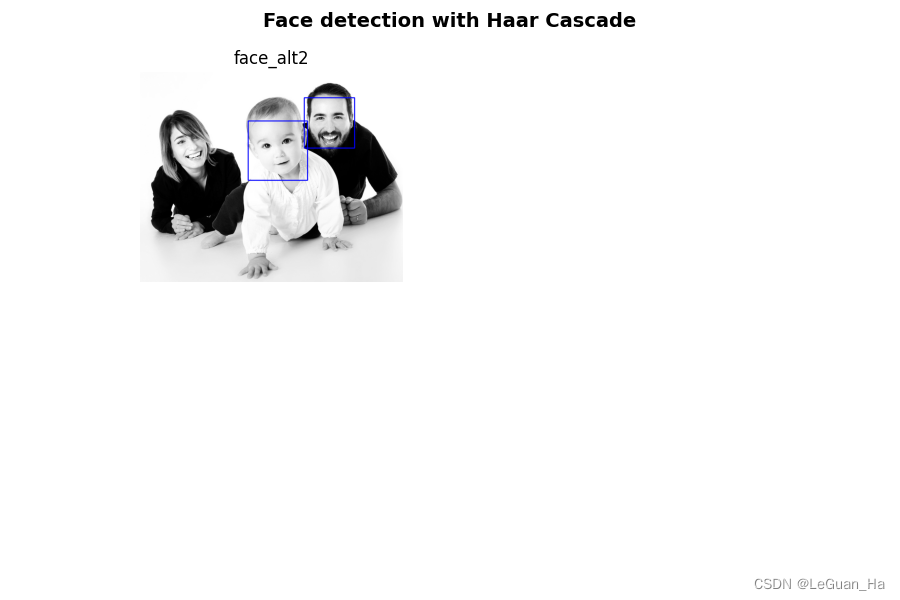
通过视频(摄像头)检测人脸:
import cv2
def plot_rectangle(image, faces):
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 3)
return image
def main():
capture = cv2.VideoCapture(0)
face_alt2 = cv2.CascadeClassifier("haarcascade_frontalface_alt2.xml")
if capture.isOpened() is False:
print("Camera Error !")
while True:
ret, frame = capture.read()
if ret:
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
face_alt2_detect = face_alt2.detectMultiScale(gray)
face_alt2_result = plot_rectangle(frame.copy(), face_alt2_detect)
cv2.imshow("face detection", face_alt2_result)
if cv2.waitKey(10) & 0xFF == ord('q'):
break
capture.release()
cv2.destroyWindow()
if __name__ == '__main__':
main()
10.基于dlib进行人脸识别
Dlib对于人脸特征提取支持很好,有很多训练好的人脸特征提取模型供开发者使用,所以Dlib人脸识别开发很适合做人脸项目开发。
HOG方向梯度直方图
- HOG是一种特征描述子,通常用于从图像数据中提取特征。它广泛用于计算机视觉任务的物体检测
- 特征描述子的作用:它是图像的简化表示,仅包含有关图像的重要信息。
import cv2
import dlib
import numpy as np
import matplotlib.pyplot as plt
def show_image(image, title):
img_RGB = image[:, :, ::-1]
plt.title(title)
plt.imshow(img_RGB)
plt.axis("off")
def plot_rectangle(image, faces):
for face in faces:
cv2.rectangle(image, (face.left(), face.top()), (face.right(), face.bottom()), (255,0,0), 4)
return image
def main():
img = cv2.imread("family.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
detector = dlib.get_frontal_face_detector()
dets_result = detector(gray, 1)
img_result = plot_rectangle(img.copy(), dets_result)
plt.figure(figsize=(9, 6))
plt.suptitle("face detection with dlib", fontsize=14, fontweight="bold")
show_image(img_result, "face detection")
plt.show()
if __name__ == '__main__':
main()
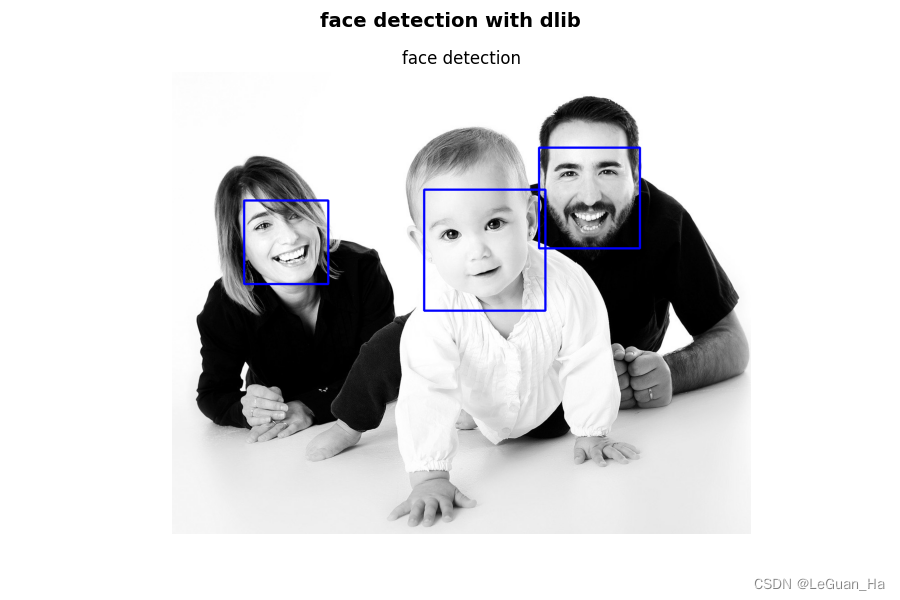
通过视频(摄像头)检测人脸:
import cv2
import dlib
def plot_rectangle(image, faces):
for face in faces:
cv2.rectangle(image, (face.left(), face.top()), (face.right(), face.bottom()), (255,0,0), 4)
return image
def main():
capture = cv2.VideoCapture(0)
if capture.isOpened() is False:
print("Camera Error !")
while True:
ret, frame = capture.read()
if ret:
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
detector = dlib.get_frontal_face_detector()
det_result = detector(gray, 1)
dets_image = plot_rectangle(frame, det_result)
cv2.imshow("face detection with dlib", dets_image)
if cv2.waitKey(1) == 27:
break
capture.release()
cv2.destroyAllWindows()
if __name__ == '__main__':
main()
11.关键点检测
人脸关键点检测——dlib
import cv2
import matplotlib.pyplot as plt
import dlib
image = cv2.imread("Tom2.jpeg")
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
faces = detector(gray, 1)
for face in faces:
cv2.rectangle(image, (face.left(), face.top()), (face.right(), face.bottom()), (0,255,0), 5)
shape = predictor(image, face)
for pt in shape.parts():
pt_position = (pt.x, pt.y)
cv2.circle(image, pt_position, 2, (0, 0, 255), -1)
plt.imshow(image)
plt.axis("off")
plt.show()
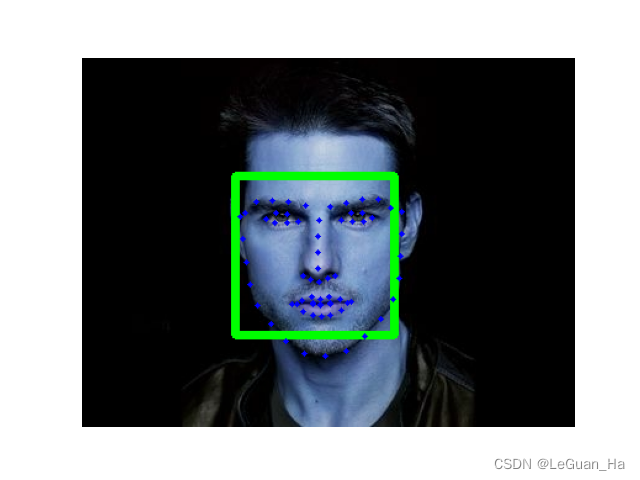
通过摄像头获取人脸的关键点:
import cv2
import dlib
capture = cv2.VideoCapture(0)
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
while True:
ret, frame = capture.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = detector(gray, 1)
for face in faces:
cv2.rectangle(frame, (face.left(), face.top()), (face.right(), face.bottom()), (0,255,0), 3)
shape = predictor(gray, face)
for pt in shape.parts():
pt_position = (pt.x, pt.y)
cv2.circle(frame, pt_position, 3, (255,0,0), -1)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cv2.imshow("face detection landmark", frame)
capture.release()
cv2.destroyAllWindows()
基于face_recognition进行人脸关键点检测
face_recognition 使用世界上最简单的人脸识别工具,它使用dlib最先进的人脸识别技术构建而成,并具有深度学习功能
import face_recognition
import cv2
import matplotlib.pyplot as plt
def show_image(image, title):
plt.title(title)
plt.imshow(image)
plt.axis("off")
def show_landmarks(image, landmarks):
for landmarks_dict in landmarks:
for landmarks_key in landmarks_dict.keys():
for point in landmarks_dict[landmarks_key]:
cv2.circle(image, point, 2, (0,0,255), -1)
return image
def main():
image = cv2.imread("Tom.jpeg")
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
face_marks = face_recognition.face_landmarks(gray, None, "large")
print(face_marks)
img_result = show_landmarks(image.copy(), face_marks)
plt.figure(figsize=(9,6))
plt.suptitle("Face Landmarks with face_recognition", fontsize=14, fontweight="bold")
show_image(img_result, "landmarks")
plt.show()
if __name__ == '__main__':
main()
12.目标跟踪
1.基于dlib库 —— 检测人脸、跟踪人脸
import cv2
import dlib
def main():
capture = cv2.VideoCapture(0)
detector = dlib.get_frontal_face_detector()
tractor = dlib.correlation_tracker()
tracking_state = False
while True:
ret, frame = capture.read()
if tracking_state is False:
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
dets = detector(gray, 1)
if len(dets) > 0:
tractor.start_track(frame, dets[0])
tracking_state = True
if tracking_state is True:
tractor.update(frame)
position = tractor.get_position()
cv2.rectangle(frame, (int(position.left()), int(position.top())),
(int(position.right()), int(position.bottom())), (0, 255, 0), 3)
key = cv2.waitKey(20) & 0xFF
if key == ord('q'):
break
cv2.imshow("face tracking",frame)
capture.release()
cv2.destroyAllWindows()
if __name__ == '__main__':
main()
增加保存视频以及显示提示信息后代码:
import cv2
import dlib
def show_info(frame, tracking_state):
pos1 = (20, 40)
pos2 = (20, 80)
cv2.putText(frame, "'1':reset", pos1, cv2.FONT_HERSHEY_COMPLEX, 0.5, (255, 255, 255))
if tracking_state is True:
cv2.putText(frame,"tracking now ...",pos2,cv2.FONT_HERSHEY_COMPLEX, 0.5, (255, 0, 0))
else:
cv2.putText(frame, "no tracking ...", pos2, cv2.FONT_HERSHEY_COMPLEX, 0.5, (0, 255, 0))
def main():
capture = cv2.VideoCapture(0)
detector = dlib.get_frontal_face_detector()
tractor = dlib.correlation_tracker()
tracking_state = False
frame_width = capture.get(cv2.CAP_PROP_FRAME_WIDTH)
frame_height = capture.get(cv2.CAP_PROP_FRAME_HEIGHT)
frame_fps = capture.get(cv2.CAP_PROP_FPS)
fourcc = cv2.VideoWriter_fourcc(*"XVID")
output = cv2.VideoWriter("record.avi", fourcc, int(frame_fps), (int(frame_width), int(frame_height)), True)
while True:
ret, frame = capture.read()
show_info(frame,tracking_state)
if tracking_state is False:
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
dets = detector(gray, 1)
if len(dets) > 0:
tractor.start_track(frame, dets[0])
tracking_state = True
if tracking_state is True:
tractor.update(frame)
position = tractor.get_position()
cv2.rectangle(frame, (int(position.left()), int(position.top())),
(int(position.right()), int(position.bottom())), (0, 255, 0), 3)
key = cv2.waitKey(20) & 0xFF
if key == ord('q'):
break
if key == ord('1'):
tracking_state = False
cv2.imshow("face tracking", frame)
output.write(frame)
capture.release()
cv2.destroyAllWindows()
if __name__ == '__main__':
main()
2.基于dlib库 —— 选定目标物体,跟踪目标
import cv2
import dlib
def show_info(frame, tracking_state):
pos1 = (10, 20)
pos2 = (10, 40)
pos3 = (10, 60)
info1 = "put left button, select an area, starct tracking"
info2 = " '1' : starct tracking , '2' : stop tacking , 'q' : exit "
cv2.putText(frame, info1, pos1, cv2.FONT_HERSHEY_COMPLEX, 0.5, (255,255,255))
cv2.putText(frame, info2, pos2, cv2.FONT_HERSHEY_COMPLEX, 0.5, (255,255,255))
if tracking_state:
cv2.putText(frame, "tracking now ...", pos3, cv2.FONT_HERSHEY_COMPLEX, 0.5, (255,0,0))
else:
cv2.putText(frame, "stop tracking ...", pos3, cv2.FONT_HERSHEY_COMPLEX, 0.5, (0,255,0))
points = []
def mouse_event_handler(event, x, y, flags, parms):
global points
if event == cv2.EVENT_LBUTTONDOWN:
points = [(x, y)]
elif event == cv2.EVENT_LBUTTONUP:
points.append((x,y))
capture = cv2.VideoCapture(0)
nameWindow = "Ojbect Tracking"
cv2.namedWindow(nameWindow)
cv2.setMouseCallback(nameWindow, mouse_event_handler)
tracker = dlib.correlation_tracker()
tracking_state = False
while True:
ret, frame = capture.read()
show_info(frame, tracking_state)
if len(points) == 2 :
cv2.rectangle(frame, points[0], points[1], (0,255,0), 3)
dlib_rect = dlib.rectangle(points[0][0], points[0][1], points[1][0], points[1][1])
if tracking_state is True:
tracker.update(frame)
pos = tracker.get_position()
cv2.rectangle(frame, (int(pos.left()),int(pos.top())), (int(pos.right()), int(pos.bottom())), (255, 0, 0), 3)
key = cv2.waitKey(1) & 0xFF
if key == ord('1'):
if len(points) == 2:
tracker.start_track(frame, dlib_rect)
tracking_state = True
points = []
if key == ord('2'):
points = []
tracking_state = False
if key == ord('q'):
break
cv2.imshow(nameWindow, frame)
capture.release()
cv2.destroyAllWindows()
v2.EVENT_LBUTTONUP:
points.append((x,y))
capture = cv2.VideoCapture(0)
nameWindow = "Ojbect Tracking"
cv2.namedWindow(nameWindow)
cv2.setMouseCallback(nameWindow, mouse_event_handler)
tracker = dlib.correlation_tracker()
tracking_state = False
while True:
ret, frame = capture.read()
show_info(frame, tracking_state)
if len(points) == 2 :
cv2.rectangle(frame, points[0], points[1], (0,255,0), 3)
dlib_rect = dlib.rectangle(points[0][0], points[0][1], points[1][0], points[1][1])
if tracking_state is True:
tracker.update(frame)
pos = tracker.get_position()
cv2.rectangle(frame, (int(pos.left()),int(pos.top())), (int(pos.right()), int(pos.bottom())), (255, 0, 0), 3)
key = cv2.waitKey(1) & 0xFF
if key == ord('1'):
if len(points) == 2:
tracker.start_track(frame, dlib_rect)
tracking_state = True
points = []
if key == ord('2'):
points = []
tracking_state = False
if key == ord('q'):
break
cv2.imshow(nameWindow, frame)
capture.release()
cv2.destroyAllWindows()
|