PIL (Python Image Library) 为 python 提供图像处理功能,以及基本图像操作(图像缩放、剪裁、旋转、颜色转换等) 在PIL中,最重要的模块为 Image
将图像转换为数组
在 python 中通过 Image 模块读取指定地址图片并转化为二维数组:
from PIL import Image
import numpy as np
img_path = r"D:\csdn相关内容\计算机视觉知识储备\PIL\volvo_xc90.jpg"
try:
img = Image.open(img_path)
except IOError:
print("Cannot open the img from the path",img_path)
img_matrix = np.asarray(img)
print(img_matrix)
print(img_matrix.shape)
img_matrix.shape 打印结果:
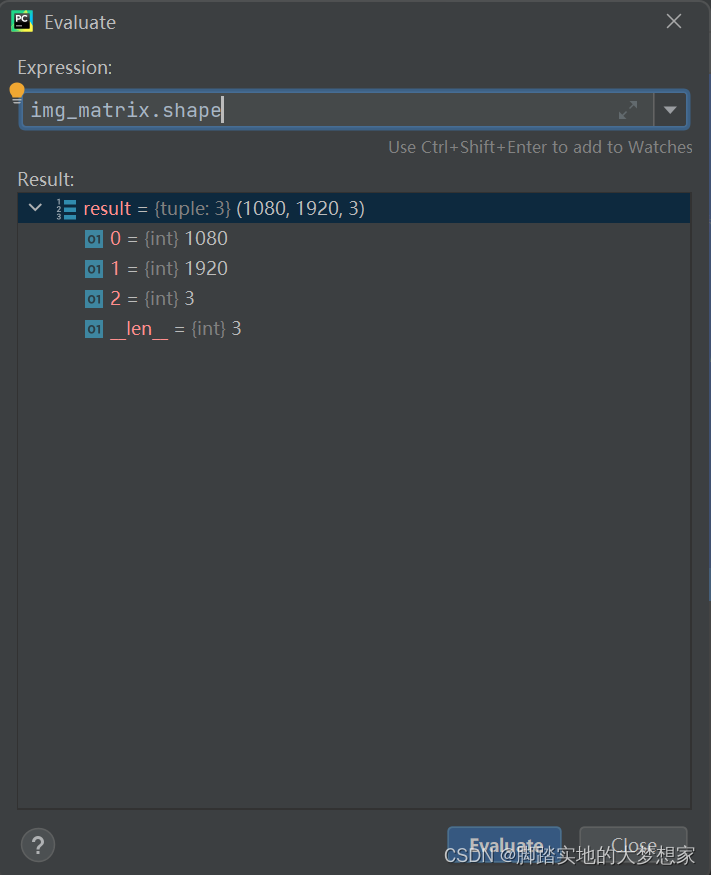
由打印结果我们可以知道这个图像的分辨率为
1920
?
1080
1920*1080
1920?1080 且为彩色图(3代表RGB三个Channels)。 而从数组的角度来说,该图像被转化成为了三维数组,数组大小为
1080
?
1920
?
3
1080*1920*3
1080?1920?3。
事实上,数组中的每个元素代表着图像某通道指定位置的颜色信息,
e
.
g
.
e.g.
e.g.
img_matrix[0,0,0]
代表着通道0(Red通道)的左上角第一个元素的颜色信息。这里假设读者已知图像的颜色信息的取值范围为
[
0
:
255
]
[0:255]
[0:255]。由打印可知R通道值为157。同样可以打印G通道以及B通道,将三个通道的颜色信息融合在一起,即为左上角第一个元素的综合颜色信息。
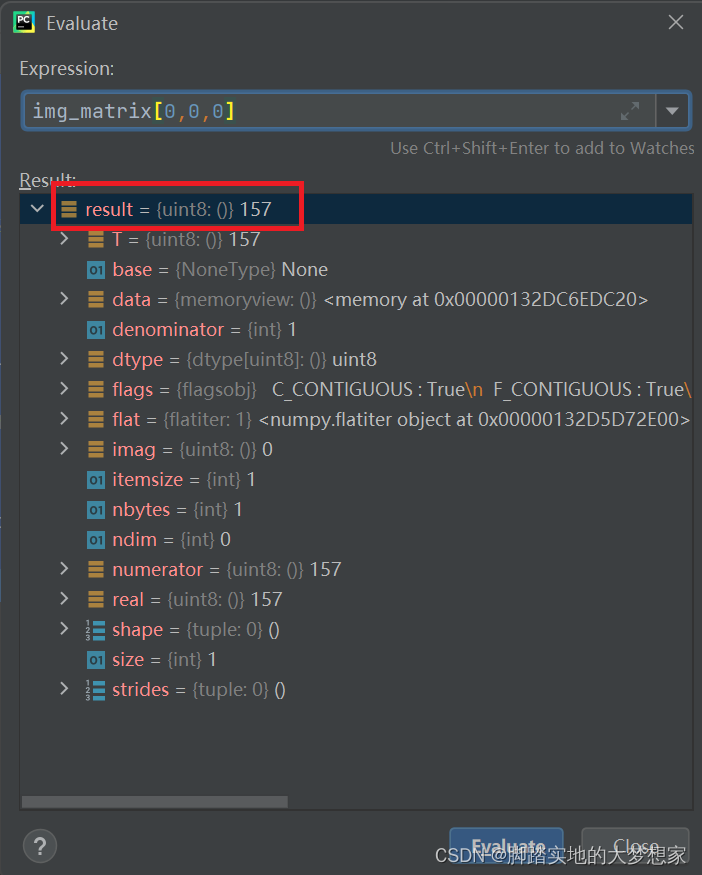
转换图像的格式
通过 .save() 方法,我们可以将图片保存为指定格式的图片。
from PIL import Image
img_path = r"D:\csdn相关内容\计算机视觉知识储备\PIL\volvo_xc90.jpg"
out_img_name = img_path.split(".")[0] + ".png"
Image.open(img_path).save(out_img_name)
创建缩率图
通过 thumbnail 函数,可以将图片保存为指定大小的缩率图;缩小为 thumbnail 接受的元组参数中指定最长边大小等比例缩放。
from PIL import Image
img_path = r"D:\csdn相关内容\计算机视觉知识储备\PIL\volvo_xc90.jpg"
out_img_name = "thumb_img.png"
img = Image.open(img_path)
img.thumbnail((960,960))
img.save(out_img_name)
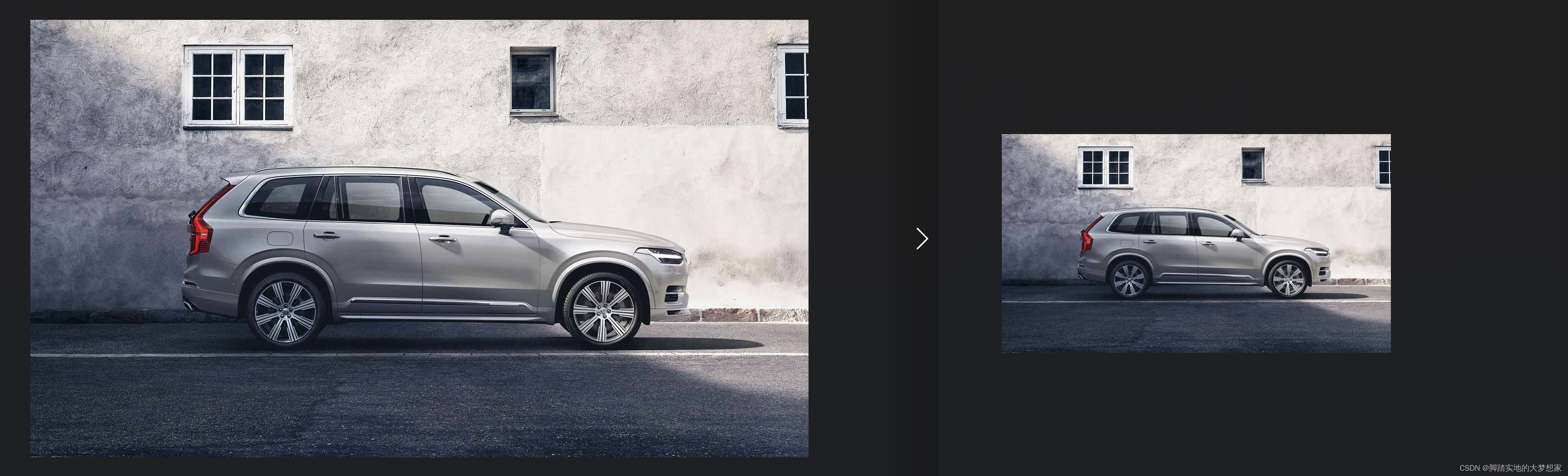
复制和粘贴图像区域
通过 crop() 函数可以从一幅图像中裁剪指定区域,crop() 函数的输入为一个四元组 (a, b, c, d),其中元素的含义可以理解为:(a,b) 为区域左上角坐标,(c,d) 为区域右下角坐标。
from PIL import Image
img_path = r"D:\csdn相关内容\计算机视觉知识储备\PIL\volvo_xc90.jpg"
out_img_name = "crop_img.png"
img = Image.open(img_path)
box = (500,500,1000,1000)
region = img.crop(box)
region = region.transpose(Image.ROTATE_90)
img.paste(region, box)
img.save(out_img_name)
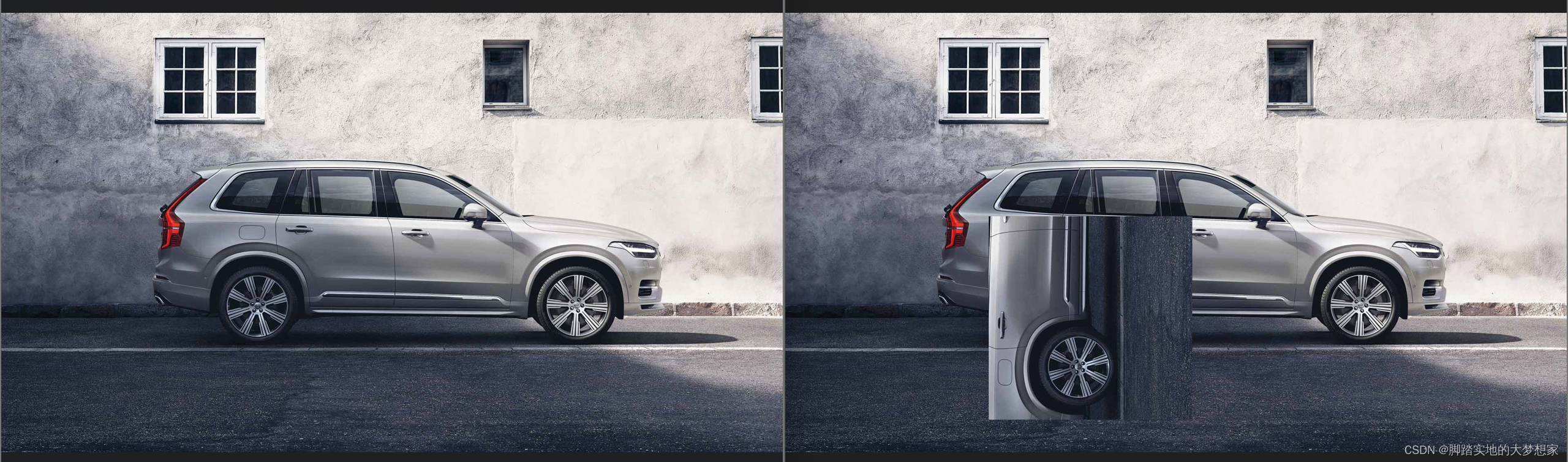
调整尺寸和旋转
调整尺寸 调整尺寸的大小与缩率不同的在于,缩略会保存图片的宽高比并进行缩放;而调整则不会保留宽高比缩放到新设定尺寸。 对于调整尺寸 (不保存宽高比) ,我们可以用 resize() 函数,resize() 函数的输入为一个二元组 (a,b),其中元素a代表新尺寸的宽,而b代表高。
from PIL import Image
img_path = r"D:\csdn相关内容\计算机视觉知识储备\PIL\volvo_xc90.jpg"
out_img_name = "resize_img.png"
img = Image.open(img_path).resize((1920,960))
img.save(out_img_name)
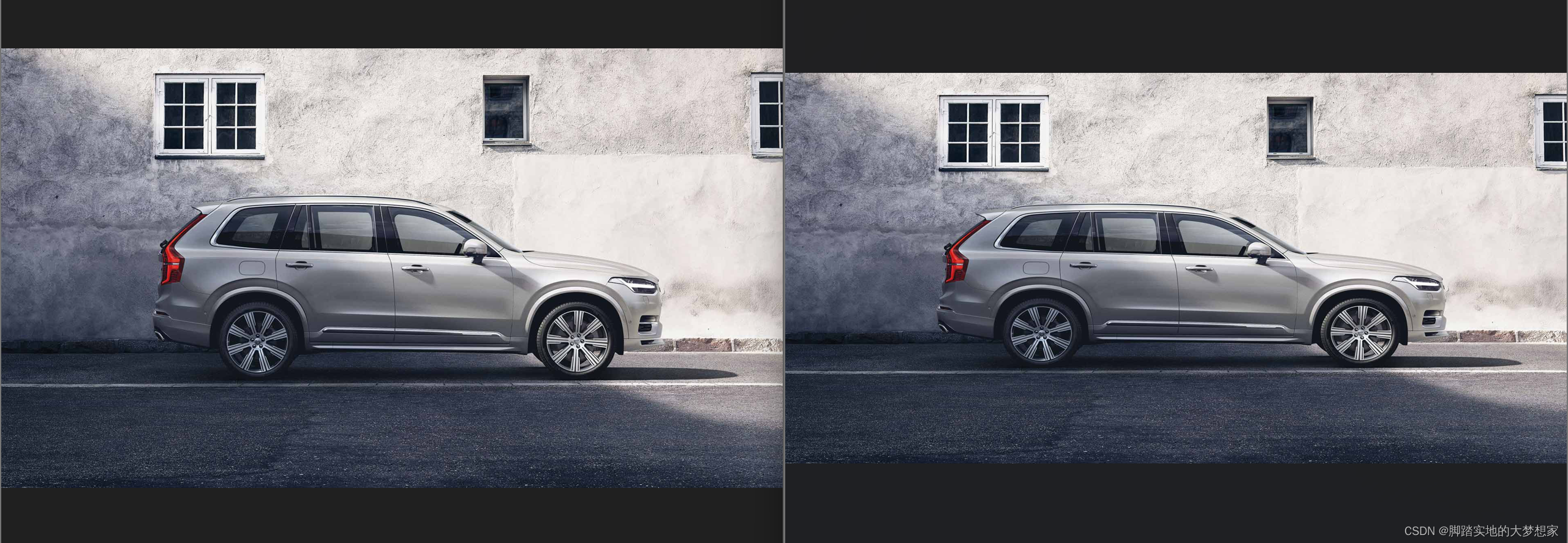
旋转 旋转通过函数 rotate(α),其中 α 代表旋转的角度,旋转角度为逆时针,旋转后的图像分辨率不发生改变:
from PIL import Image
img_path = r"D:\csdn相关内容\计算机视觉知识储备\PIL\volvo_xc90.jpg"
out_img_name = "rotate_img.png"
img = Image.open(img_path).rotate(45)
img.save(out_img_name)
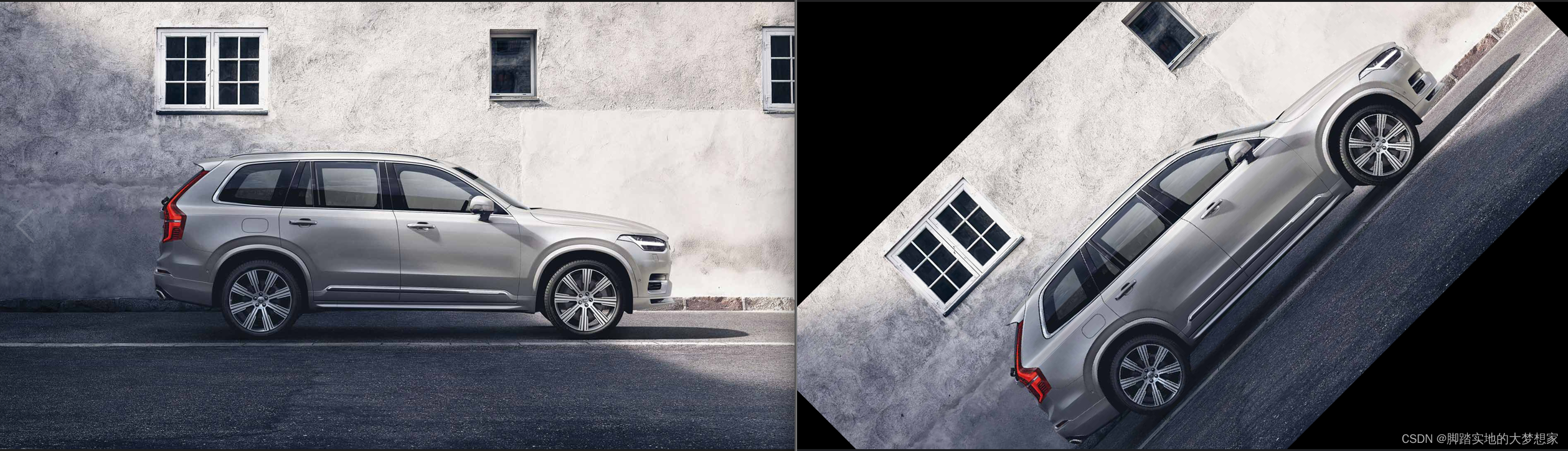
分辨率未发生改变: 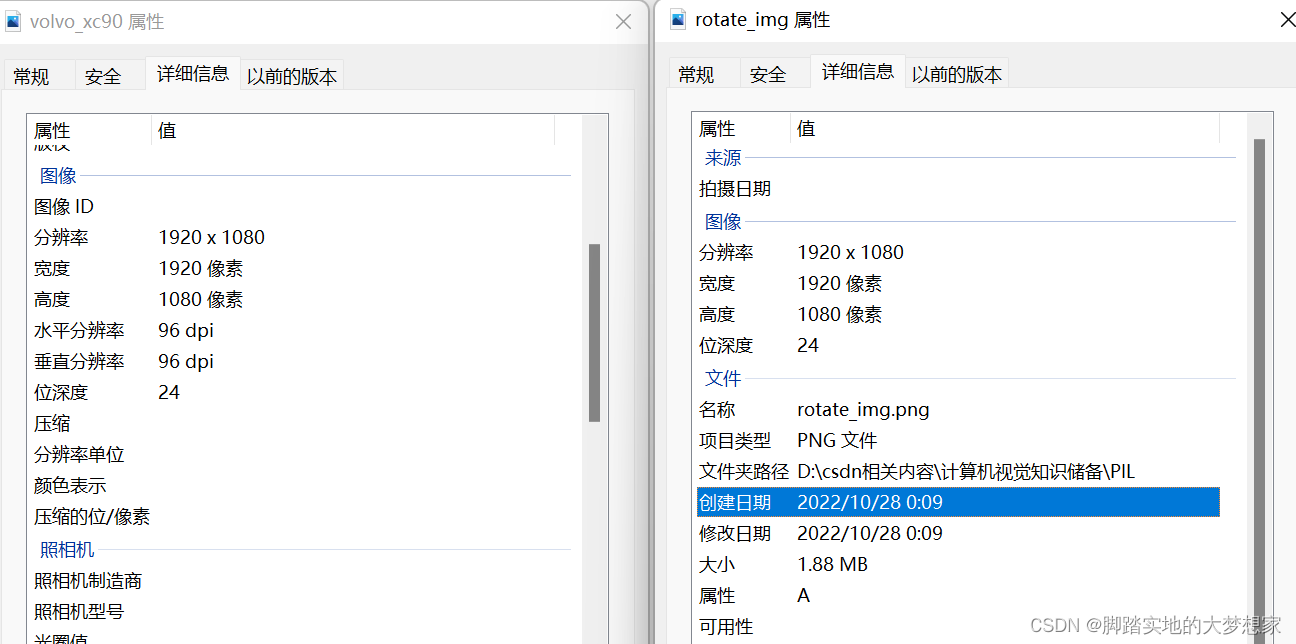 所以旋转后势必会丢失一些数据信息,上述案例中即左上角与右下角的部分像素信息:
import numpy as np
img_array = np.asarray(img)
print(img_array[0][0][0], img_array[0][0][1], img_array[0][0][2])
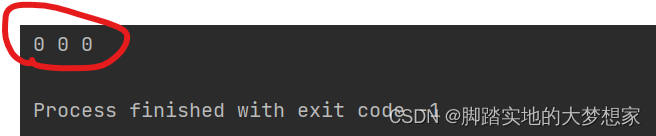
e
.
g
.
e.g.
e.g. 左上角元素的 RGB (0, 0, 0) 为黑色,图片旋转后将该部分信息将丢失,我们再将其旋转回:
img_back = Image.open(out_img_name).rotate(-45)
img_back.save("rotate_back_img.png")
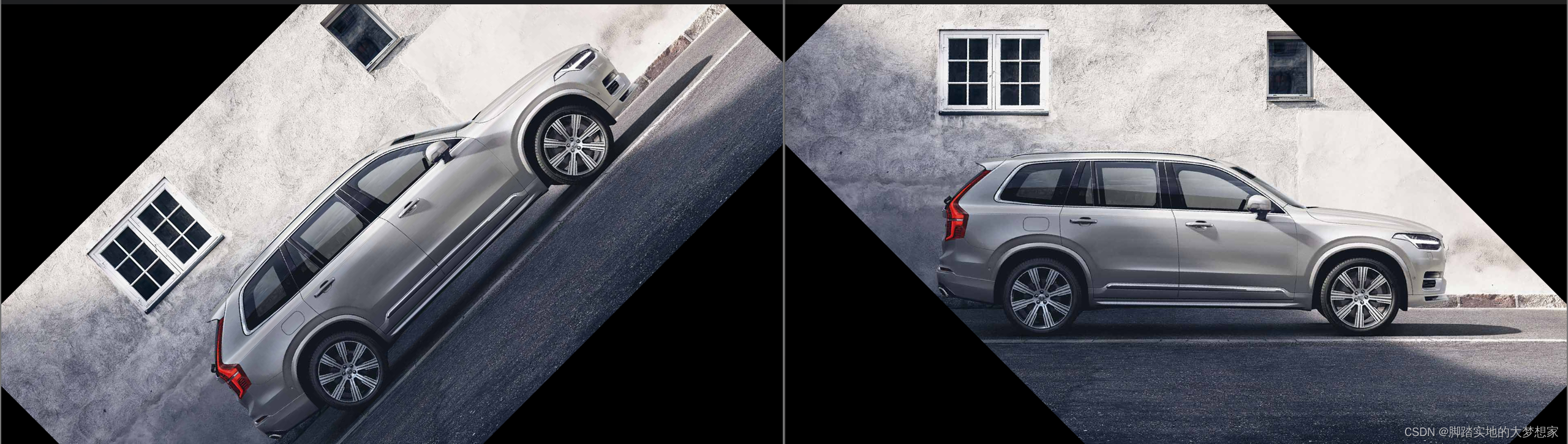
图片链接(免费下载):https://download.csdn.net/download/weixin_43098506/86835234 更多相关问题以及可补充内容,留言或联系!感谢好评!👏
|