注意为“区块”、“交易”、“UTXO”和“读写集”。
1. 区块
区块以DAG方式链接起来形成的链。因此,区块是区块链的基本单元。
- 功能
区块是区块链的基本单元,通常为了提高区块链网络的吞吐,矿工会在一个区块中打包若干个交易。一个区块通常由区块头以及区块体组成。
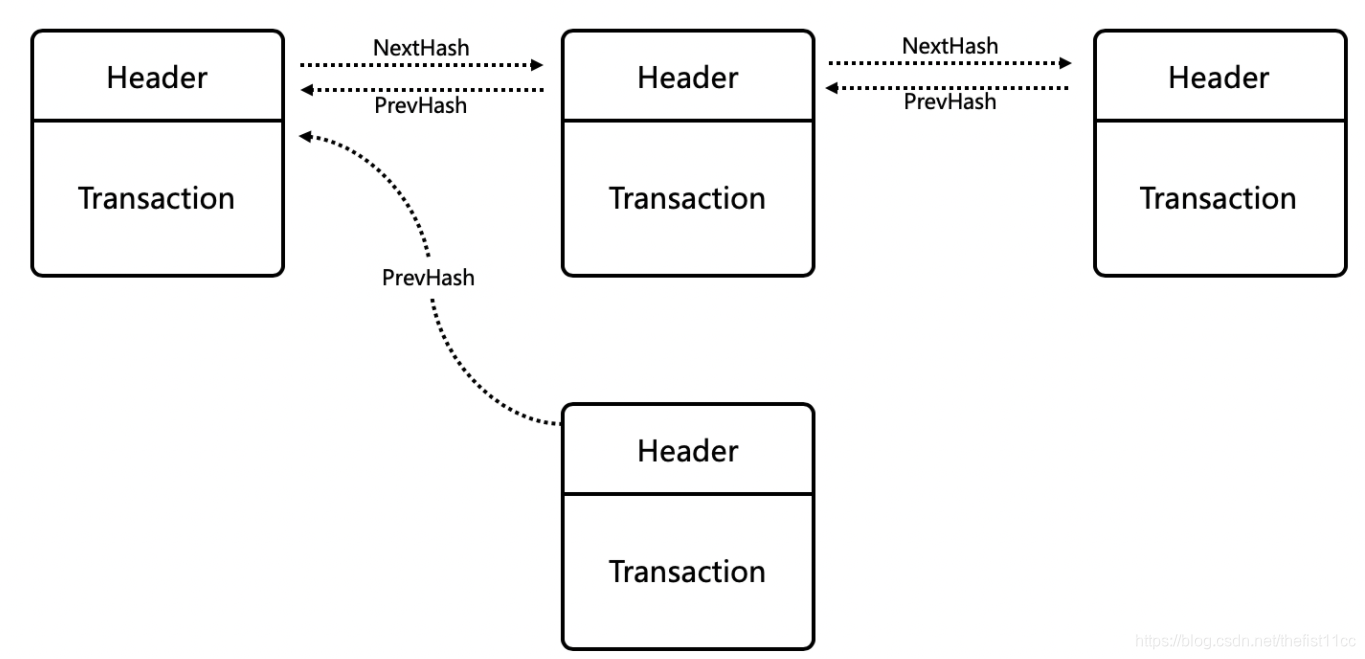
1message InternalBlock {
2
3
4 int32 version = 1;
5
6
7 int32 nonce = 2;
8
9
10 bytes blockid = 3;
11
12
13 bytes pre_hash = 4;
14
15
16 bytes proposer = 5;
17
18
19 bytes sign = 6;
20
21
22 bytes pubkey = 7;
23
24
25 bytes merkle_root = 8;
26
27
28 int64 height = 9;
29
30
31 int64 timestamp = 10;
32
33
34
35 repeated Transaction transactions = 11;
36
37
38 int32 tx_count = 12;
39
40 repeated bytes merkle_tree = 13;
41
42 int64 curTerm = 16;
43 int64 curBlockNum = 17;
44
45 map<string, string> failed_txs = 18;
46
47 int32 targetBits = 19;
48
49
50
51 bool in_trunk = 14;
52
53
54 bytes next_hash = 15;
55}
2. 交易
- 背景
区块链网络中的每个节点都是一个状态机,为了给每个节点传递状态,系统引入了交易,作为区块链网络状态更改的最小操作单元。 - 功能
通常表现为普通转账以及智能合约调用。 - 代码
交易的Proto如下
1message Transaction {
2
3
4 bytes txid = 1;
5
6
7 bytes blockid = 2;
8
9
10 repeated TxInput tx_inputs = 3;
11
12
13 repeated TxOutput tx_outputs = 4;
14
15
16 bytes desc = 6;
17
18
19 bool coinbase = 7;
20
21
22 string nonce = 8;
23
24
25 int64 timestamp = 9;
26
27 int32 version = 10;
28
29
30 bool autogen = 11;
31
32 repeated TxInputExt tx_inputs_ext = 23;
33
34 repeated TxOutputExt tx_outputs_ext = 24;
35
36 repeated InvokeRequest contract_requests = 25;
37
38
39 string initiator = 26;
40
41 repeated string auth_require = 27;
42
43 repeated SignatureInfo initiator_signs = 28;
44
45 repeated SignatureInfo auth_require_signs = 29;
46
47 int64 received_timestamp = 30;
48
49 XuperSignature xuper_sign = 31;
50}
3. UTXO
- 背景
区块链中比较常见的两种操作,包括普通转账以及合约调用,这两种操作都涉及到了数据状态的引用以及更新。为了描述普通转账涉及到的数据状态的引用以及更新,引入了UTXO(Unspent Transaction Output)。 - 功能
一种记账方式,用来描述普通转账时涉及到的数据状态的引用以及更新。通常由转账来源数据(UtxoInput)以及转账去处数据(UtxoOutput)组成。 - 代码
UTXO的Proto如下
1message Utxo {
2
3 bytes amount = 1;
4
5 bytes toAddr = 2;
6
7 bytes toPubkey = 3;
8
9 bytes refTxid = 4;
10
11 int32 refOffset = 5;
12}
13
14
15message UtxoInput {
16 Header header = 1;
17
18
19 string bcname = 2;
20
21
22 string address = 3;
23
24
25 string publickey = 4;
26
27
28 string totalNeed = 5;
29
30
31 bytes userSign = 7;
32
33
34 bool needLock = 8;
35}
36
37
38message UtxoOutput {
39 Header header = 1;
40
41
42 repeated Utxo utxoList = 2;
43
44
45 string totalSelected = 3;
46}
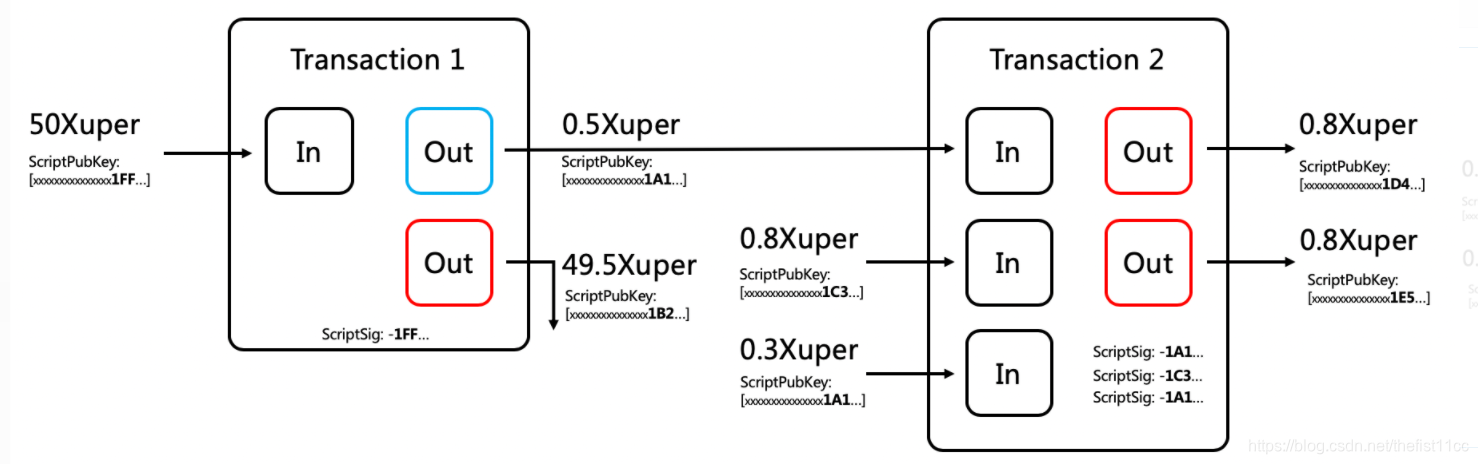
4. 读写集
- 背景:区块链中比较常见的两种操作,包括普通转账以及合约调用,这两种操作都涉及到了数据状态的引用以及更新。为了描述合约调用涉及到的数据状态的引用以及更新,引入了读写集。
- 功能:一种用来描述合约调用时涉及到的数据状态的引用以及更新的技术。通常由读集(TxInputExt)以及写集(TxOutputExt)组成。
- 代码:读写集的Proto如下
1
2message TxInputExt {
3
4 string bucket = 1;
5
6 bytes key = 2;
7
8 bytes ref_txid = 3;
9
10 int32 ref_offset = 4;
11}
12
13message TxOutputExt {
14
15 string bucket = 1;
16
17 bytes key = 2;
18
19 bytes value = 3;
20}
|