编写智能合约时,通常状态的变化是通过触发一个事件来表达,The Graph则是捕捉区块链事件并提供一个查询事件的GraphQL接口,让我们可以方便的跟踪数据的变化。 实际上很多 DEFI 协议及都是The Graph来基于查询数据。 https://thegraph.academy/developers/local-development/ 我们以此教程为基础做出一下额外的补充。 工具准备:nodejs、yarn、docker、truffle、graph-cli
1. 合约开发与部署
-
合约的开发环境搭建 npm install -g truffle ganache-cli -
合约编写 该合约为手写的测试合约,主要定义了两个事件,PairCreated和AddLiquidity。我们用thegarph来监听以下的事件。
pragma solidity ^0.8.0;
contract Pair{
string private _name;
uint private _liquidity;
constructor(string memory name){
_name = name;
}
function addLiquidity(uint amount) public {
_liquidity+=amount;
}
function getLiquidity() public view returns(uint){
return _liquidity;
}
function getName() public view returns(string memory){
return _name;
}
}
contract Dex {
address private _owner;
uint private _index = 0;
mapping(address => Pair) private _pairs;
mapping(uint => address) private _indexs;
modifier onlyOwner(){
require(msg.sender == _owner,"must be owner");
_;
}
event PairCreated(string name, address pairAddress);
event AddLiquidity(address pairAddress, uint liquidity);
function createPair(string memory name) public {
Pair pair = new Pair(name);
_pairs[address(pair)] = pair;
_indexs[_index] = address(pair);
_index++;
emit PairCreated(name, address(pair));
}
function addLiquidity(address pairAddress,uint liquidity) public {
_pairs[pairAddress].addLiquidity(liquidity);
emit AddLiquidity(pairAddress,liquidity);
}
function getCount() public view returns(uint){
return _index;
}
function pairAddress(uint index) public view returns(address){
return _indexs[index];
}
function getPairName(address pairAddress) public view returns(string memory){
return _pairs[pairAddress].getName();
}
function getLiquidity(address pairAddress) public view returns(uint liquidity){
return _pairs[pairAddress].getLiquidity();
}
}
-
通过truffle部署到自建的ganache区块链上 truffle migrate -
通过remix调用合约写入数据触发事件
2. 创建索引数据的Subgraph
TheGraph中定义如何为数据建立索引,称为Subgraph,它包含三个组件:
- Manifest 清单(subgraph.yaml) - 定义配置项
specVersion: 0.0.1
description: study
repository: https://github.com/graphprotocol/example-subgraph
schema:
file: ./schema.graphql
dataSources:
- kind: ethereum/contract
name: study
network: mainnet
source:
address: '0xf80e65c3c1B49626bae2ECE7e67A3e1AdBD480C2'
abi: Dex
mapping:
kind: ethereum/events
apiVersion: 0.0.5
language: wasm/assemblyscript
entities:
- Pairs
abis:
- name: Dex
file: ./build/contracts/Dex.json
eventHandlers:
- event: PairCreated(string,address)
handler: handleNewPairCreated
- event: AddLiquidity(address,uint256)
handler: handleAddLiquidity
file: ./src/mapping.ts
- Schema 模式(schema.graphql) - 定义数据
type Pair @entity {
id: ID!
displayName: String!
liquidity: Int!
}
-
生成代码 graph codegen -
Mapping 映射(mapping.ts) - 定义事件到数据的转换
import {AddLiquidity, PairCreated} from '../generated/study/Dex'
import {Pair} from '../generated/schema'
export function handleNewPairCreated(event: PairCreated): void {
let pair = new Pair(event.params.pairAddress.toHexString())
pair.displayName = event.params.name
pair.liquidity = 0
pair.save()
}
export function handleAddLiquidity(event: AddLiquidity): void {
let id = event.params.pairAddress.toHexString()
let pair = Pair.load(id)
if (pair == null) {
return
}
pair.liquidity += event.params.liquidity.toI32()
pair.save()
}
3. 部署Subgraph到TheGraph,实现数据索引
- 以搭建本地节点为例,运行graph-node
git clone https://github.com/graphprotocol/graph-node/
cd graph-node/docker
docker compose up
- 安装项目依赖&&部署到graph节点上
yarn && yarn codegen
yarn create-local
yarn deploy-local
4. 在graphql查询数据
query{
pairs(first:10){
id,
displayName,
liquidity
}
}
显示结果如下 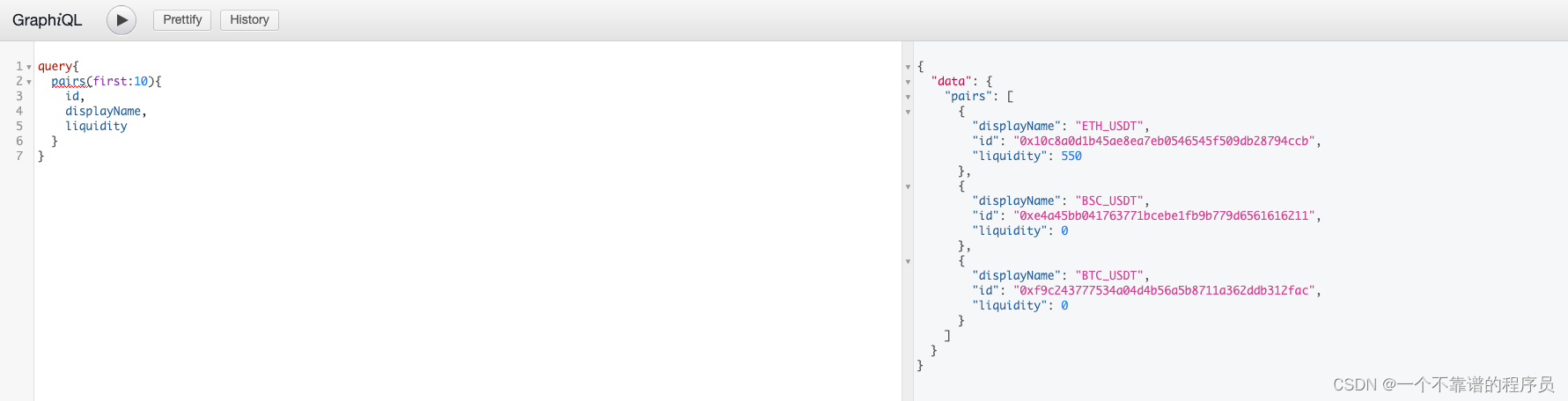
5. 踩坑指南
- Failed to deploy to Graph node http://127.0.0.1:8020/: Ethereum network not supported by registrar: mainnet.
是因为我本地起到的ganache区块链网络,graph-node在容器内无法找到宿主机的IP,更改了docker/docker-compose.yml里的配置ethereum: ‘mainnet:http://docker.for.mac.host.internal:8545’
|