Ò»¡¢Êý¾ÝÀàÐÍ
1.1¡¢ÖµÀàÐÍ
1.1.1¡¢²¼¶û
pragma solidity ^0.4.25;
contract TestBool {
bool flag;
int num1 = 100;
int num2 = 200;
// default false
function getFlag() public view returns(bool) {
return flag; // false
}
// ·Ç
function getFlag2() public view returns(bool) {
return !flag; // true
}
// Óë
function getFlagAnd() public view returns(bool) {
return (num1 != num2) && !flag; // true
}
// »ò
function getFlagOr() public view returns(bool) {
return (num1 == num2) || !flag; // true
}
}
1.1.2¡¢ÕûÊý
¼Ó¼õ³Ë³ý¡¢È¡Óà¡¢ÃÝÔËËã,
pragma solidity ^0.4.25;
// ÕûÐÍÌØÐÔÓëÔËËã
contract TestInteger {
int num1; // ÓзûºÅÕûÐÍ int256
uint num2; // ÎÞ·ûºÅÕûÐÍ uint256
function add(uint _a, uint _b) public pure returns(uint) {
return _a + _b;
}
function sub(uint _a, uint _b) public pure returns(uint) {
return _a - _b;
}
function mul(uint _a, uint _b) public pure returns(uint) {
return _a * _b;
}
function div(uint _a, uint _b) public pure returns(uint) {
return _a / _b; // ÔÚsolidityÖÐ,³ý·¨ÊÇ×öÕû³ý,ûÓÐСÊýµã
}
function rem(uint _a, uint _b) public pure returns(uint) {
return _a % _b;
}
function square(uint _a, uint _b) public pure returns(uint) {
return _a ** _b; // ÃÝÔËËãÔÚ0.8.0Ö®ºó,±äΪÓÒÓÅÏÈ,¼´a ** b ** c => a ** (b ** c)
}
function max() public view returns(uint) {
return uint(-1);
// return type(uint).max; // 0.8²»ÔÙÔÊÐíuint(-1)
}
}
λÔËËã,
pragma solidity ^0.4.25;
// λÔËËã
contract TestBitwise {
uint8 num1 = 3;
uint8 num2 = 4;
function bitAdd() public view returns(uint) {
return num1 & num2;
}
function bitOr() public view returns(uint) {
return num1 | num2;
}
function unBit() public view returns(uint) {
return ~num1;
}
function bitXor() public view returns(uint) {
return num1 ^ num2;
}
function bitRight() public view returns(uint) {
return num1 >> 1;
}
function bitLeft() public view returns(uint) {
return num1 << 1;
}
}
1.1.3¡¢¶¨³¤¸¡µãÐÍ
Ŀǰֻ֧³Ö¶¨Òå,²»Ö§³Ö¸³ÖµÊ¹ÓÃ,
fixed num; // ÓзûºÅ
ufixed num; // ÎÞ·ûºÅ
1.1.4¡¢µØÖ·ÀàÐÍ
address addr = msg.sender;
address addr = 0xdCad3a6d3569DF655070DEd06cb7A1b2Ccd1D3AF;
1.1.5¡¢ºÏÔ¼ÀàÐÍ
ÔÚºÏÔ¼TestTypeÖÐʹÓÃTestBitwiseºÏÔ¼,
TestBitwise t = TestBitwise(addr);
1.1.6¡¢Ã¶¾ÙÀàÐÍ
enum ActionChoices { Up, Down, Left, Right }
1.1.7¡¢¶¨³¤×Ö½ÚÊý×é
pragma solidity ^0.4.25;
// ¹Ì¶¨³¤¶ÈµÄ×Ö½ÚÊý×é(¾²Ì¬),ÒÔ¼°×ª»»ÎªstringÀàÐÍ
contract TestBytesFixed {
// public ×Ô¶¯Éú³ÉͬÃûµÄget·½·¨
bytes1 public num1 = 0x7a; // 1 byte = 8 bit
bytes1 public num2 = 0x68;
bytes2 public num3 = 0x128b; // 2 byte = 16 bit
// »ñÈ¡×Ö½ÚÊý×鳤¶È
function getLength() public view returns(uint) {
return num3.length; // 2
}
// ×Ö½ÚÊý×é±È½Ï
function compare() public view returns(bool) {
return num1 < num2;
}
// ×Ö½ÚÊý×éλÔËËã
function bitwise() public view returns(bytes1,bytes1,bytes1) { // ¶à·µ»ØÖµ
return ((num1 & num2), (num1 | num2), (~num1));
}
// ÏÈתΪbytes¶¯Ì¬Êý×é,ÔÙͨ¹ýstring¹¹Ôì 0x7a7a -> zz
function toString(bytes2 _val) public pure returns(string) {
bytes memory buf = new bytes(_val.length);
for (uint i = 0; i < _val.length; i++) {
buf[i] = _val[i];
}
return string(buf);
}
}
¹Ì¶¨³¤¶È×Ö½ÚÊý×éµÄÀ©³äºÍѹËõ,
pragma solidity ^0.4.25;
// ¹Ì¶¨³¤¶ÈµÄ×Ö½ÚÊý×é(¾²Ì¬)À©ÈݺÍѹËõ
contract TestBytesExpander {
// public ×Ô¶¯Éú³ÉͬÃûµÄget·½·¨
bytes6 name = 0x796f7269636b;
function changeTo1() public view returns(bytes1) {
return bytes1(name); // 0x79
}
function changeTo2() public view returns(bytes2) {
return bytes2(name); // 0x796f
}
function changeTo16() public view returns(bytes16) {
return bytes16(name); // 0x796f7269636b00000000000000000000
}
}
1.1.8¡¢º¯ÊýÀàÐÍ
function (<parameter types>) {internal|external|public|private} [pure|constant|view|payable] [returns (<return types>)]
1.2¡¢ÒýÓÃÀàÐÍ
1.2.1¡¢×Ö·û´®
pragma solidity ^0.4.25;
// ÐÞ¸ÄstringÀàÐ͵ÄÊý¾Ý
contract TestString {
string name = 'yorick'; // ×Ö·û´®¿ÉÒÔʹÓõ¥ÒýºÅ»òÕßË«ÒýºÅ
string name2 = "!@#$%^&"; // ÌØÊâ×Ö·ûÕ¼1¸öbyte
string name3 = "ÕÅÈý"; // ÖÐÎÄÔÚstringÖÐʹÓÃutf8µÄ±àÂ뷽ʽ´æ´¢,Õ¼ÓÃ3¸öbyte
function getLength() view public returns(uint) {
// ²»¿ÉÒÔÖ±½Ó»ñÈ¡stringµÄlength
return bytes(name).length; // 6
}
function getLength2() view public returns(uint) {
return bytes(name2).length; // 7
}
function getLength3() view public returns(uint) {
return bytes(name3).length; // 6
}
function getElmName() view public returns(bytes1) {
// ²»¿ÉÒÔÖ±½Óͨ¹ýÊý×éϱêname[0]»ñÈ¡
return bytes(name)[0]; // 0x79
}
function changeElmName() public {
bytes(name)[0] = "h";
}
function getName() view public returns(bytes) {
return bytes(name);
}
}
1.2.2¡¢±ä³¤×Ö½ÚÊý×é
pragma solidity ^0.4.25;
// ¶¯Ì¬µÄ×Ö½ÚÊý×é,ÒÔ¼°×ª»»ÎªstringÀàÐÍ
contract TestBytesDynamic {
bytes public dynamicBytes;
function setDynamicBytes(string memory val) public {
dynamicBytes = bytes(val);
}
function getVal() public view returns(string){
return string(dynamicBytes);
}
}
1.2.3¡¢Êý×é
¶¨³¤Êý×é,
pragma solidity ^0.4.25;
// ¶¨³¤Êý×é
contract TestArrFixed {
uint[5] arr = [1,2,3,4,5];
// ÐÞ¸ÄÊý×éÔªËØÄÚÈÝ
function modifyElements() public {
arr[0] = 12;
arr[1] = 14;
}
// ²é¿´Êý×é
function watchArr() public view returns(uint[5]) {
return arr;
}
// Êý×éÔªËØÇóºÍ¼ÆËã
function sumArr() public view returns(uint) {
uint sum = 0;
for (uint i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
// Êý×鳤¶È
function getLength() public view returns(uint) {
return arr.length;
}
// deleteÖØÖÃÊý×éijϱêµÄÔªËØÖµ,²»»áÕæÕýɾ³ý¸ÃÔªËØ
function deleteElm(uint idx) public {
delete arr[idx];
}
// deleteÖØÖÃÕû¸öÊý×é
function deleteArr() public {
delete arr;
}
/** ¶¨³¤Êý×é²»ÔÊÐí¸Ä±ä³¤¶ÈºÍpush
// ѹËõÊý×éºó,ÓÒ²à¶àÓàÔªËØ±»¶ªÆú
function changeLengthTo1() public {
arr.length = 1;
}
// À©ÈÝÊý×éºó,ÓÒ²àÔªËØ²¹0
function changeLengthTo10() public {
arr.length = 10;
}
// ×·¼ÓÐÂÔªËØ
function pushElm(uint _elm) public {
arr.push(_elm);
}
*/
}
±ä³¤Êý×é,
pragma solidity ^0.4.25;
// ±ä³¤Êý×é
contract TestArrDynamic {
uint[] arr = [1,2,3,4,5];
// ²é¿´Êý×é
function watchArr() public view returns(uint[]) {
return arr;
}
// Êý×鳤¶È
function getLength() public view returns(uint) {
return arr.length;
}
// ѹËõÊý×éºó,ÓÒ²à¶àÓàÔªËØ±»¶ªÆú
function changeLengthTo1() public {
arr.length = 1;
}
// À©ÈÝÊý×éºó,ÓÒ²àÔªËØ²¹0
function changeLengthTo10() public {
arr.length = 10;
}
// ×·¼ÓÐÂÔªËØ
function pushElm(uint _elm) public {
arr.push(_elm);
}
// deleteÖØÖÃÊý×éijϱêµÄÔªËØÖµ,²»»áÕæÕýɾ³ý¸ÃÔªËØ
function deleteElm(uint idx) public {
delete arr[idx];
}
// deleteÖØÖÃÕû¸öÊý×é
function deleteArr() public {
delete arr;
}
}
¶þάÊý×é,
pragma solidity ^0.4.25;
/**
¶þάÊý×é
solidityµÄ¶þάÊý×éÓëÆäËûÓïÑÔ²»Í¬,[2] [3]±íʾ3ÐÐ2ÁÐ,¶øÆäËûÓïÑÔΪ2ÐÐ3ÁÐ;
¶þά¶¯Ì¬Êý×éÓëһάÊý×éÀàËÆ,¿ÉÒԸıäÆäÊý×鳤¶È;
*/
contract TestArr2Dimensional {
uint[2][3] arr = [[1,2],[3,4],[5,6]];
function getRowSize() public view returns(uint) {
return arr.length; // 3
}
function getColSize() public view returns(uint) {
return arr[0].length; // 2
}
function watchArr() public view returns(uint[2][3]) {
return arr; // 1,2,3,4,5,6
}
function sumArr() public view returns(uint) {
uint sum = 0;
for (uint i = 0; i < getRowSize(); i++) {
for (uint j = 0; j < getColSize(); j++) {
sum += arr[i][j];
}
}
return sum;
}
function modifyArr() public {
arr[0][0] = 99;
}
}
Êý×é×ÖÃæÖµ,
pragma solidity ^0.4.25;
// Êý×é×ÖÃæÖµ
contract TestArrLiteral {
// ×îС´æ´¢Æ¥Åä,䳬¹ý255,ËùÒÔʹÓÃuint8´æ´¢
function getLiteral8() pure public returns(uint8[3]) {
return [1,2,3];
}
// ³¬¹ý255,ËùÒÔʹÓÃuint16´æ´¢
function getLiteral16() pure public returns(uint16[3]) {
return [256,2,3]; // [255,2,3] ²»±»uint16ÔÊÐí
}
// Ç¿ÖÆ×ª»»Îªuint256
function getLiteral256() pure public returns(uint[3]) {
return [uint(1),2,3]; // ¸øÈÎÒâÔªËØÇ¿×ª¼´¿É,·ñÔò²»±»ÔÊÐí
}
// ¼ÆËãÍâ½ç´«ÈëµÄÄÚÈÝ
function addLiterals(uint[3] arr) pure external returns(uint) {
uint sum = 0;
for (uint i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
}
1.2.4¡¢½á¹¹Ìå
pragma solidity ^0.4.25;
// ½á¹¹Ìå³õʼ»¯µÄÁ½ÖÖ·½·¨
contract TestStruct {
struct Student {
uint id;
string name;
mapping(uint=>string) map;
}
// ĬÈÏΪstorageÀàÐÍ,Ö»ÄÜͨ¹ýstorageÀàÐͲÙ×÷½á¹¹ÌåÖеÄmappingÀàÐÍÊý¾Ý
Student storageStu;
// mappingÀàÐÍ¿ÉÒÔ²»ÓÃÔÚ¶¨ÒåµÄʱºò¸³Öµ,¶øÆäËûÀàÐͲ»¸³ÖµÔò»á±¨´í
function init() public pure returns(uint, string) {
Student memory stu = Student(100, "Jay");
return (stu.id, stu.name);
}
function init2() public pure returns(uint, string) {
Student memory stu = Student({name: "Jay", id: 100});
return (stu.id, stu.name);
}
function init3() public returns(uint, string, string) {
Student memory stu = Student({name: "Jay", id: 100});
// Ö±½Ó²Ù×÷½á¹¹ÌåÖеÄmapping²»±»ÔÊÐí: Student memory out of storage
// stu.map[1] = "artist";
// ͨ¹ýstorageÀàÐ͵ıäÁ¿²Ù×÷½á¹¹ÌåÖеÄmapping
storageStu = stu;
storageStu.map[1] = "artist";
return (storageStu.id, storageStu.name, storageStu.map[1]);
}
// ½á¹¹Ìå×÷ΪÈë²Îʱ,ΪmemoryÀàÐÍ,²¢ÇÒ²»ÄÜʹÓÃpublic»òexternalÐÞÊκ¯Êý:Internal or recursive type is not allowed for public or external functions.
// ¸³ÖµÊ±Ò²ÒªÖ¸¶¨Îªmemory,·ñÔò±¨´í
function testIn(Student stu) internal returns(uint) {
return stu.id;
}
// ½á¹¹Ìå×÷Ϊ³ö²Î,ͬÑùÖ»ÄÜprivate»òinternalÉùÃ÷ÄÚ²¿Ê¹ÓÃ
function testOut(Student stu) private returns(Student) {
return stu;
}
}
1.3¡¢Ó³Éä
contract TestMapping {
mapping(address => uint) private scores; // <ѧÉú,·ÖÊý>µÄµ¥²ãÓ³Éä
mapping(address => mapping(bytes32 => uint8)) private _scores; // <ѧÉú,<¿ÆÄ¿,·ÖÊý>>µÄÁ½²ãÓ³Éä
function getScore() public view returns(address, uint) {
address addr = msg.sender;
return (addr, scores[addr]);
}
function setScore() public {
scores[msg.sender] = 100;
}
}
¶þ¡¢×÷ÓÃÓò(·ÃÎÊÐÞÊηû)
contract TestAccessCtrl {
constructor () public {}
uint public num1 = 1; // ×Ô¶¯ÎªpublicÉú³ÉͬÃûµÄgetº¯Êý,µ«ÔÚ±àÂëʱ²»¿ÉÖ±½Óµ÷ÓÃnum1()
uint private num2 = 2;
uint num3 = 3; // ²»Ð´ÔòĬÈÏprivate
function funcPublic() public returns(string) {
return "public func";
}
function funcPrivate() private returns(string) {
return "private func";
}
function funcInternal() internal returns(string) {
return "internal func";
}
function funcExternal() external returns(string) {
return "external func";
}
function test1(uint choice) public returns(string) {
if (choice == 1) return funcPublic();
if (choice == 2) return funcPrivate();
if (choice == 3) return funcInternal();
//if (choice == 4) return funcExternal(); // external²»ÔÊÐíÖ±½ÓÔÚÄÚ²¿ÓÃ
if (choice == 4) return this.funcExternal(); // ¼ä½Óͨ¹ýthis²Å¿ÉÒÔµ÷ÓÃexternal
}
}
contract TestAccessCtrlSon is TestAccessCtrl {
function test2(uint choice) public returns(string) {
if (choice == 1) return funcPublic(); // publicÔÊÐíÅÉÉúºÏԼʹÓÃ
//if (choice == 2) return funcPrivate(); // private²»ÔÊÐíÅÉÉúºÏԼʹÓÃ
if (choice == 3) return funcInternal(); // internalÔÊÐíÅÉÉúºÏԼʹÓÃ
//if (choice == 4) return funcExternal(); // externalÒ²²»ÔÊÐíÅÉÉúºÏÔ¼Ö±½ÓʹÓÃ
}
}
contract TestAccessCtrl2 {
function test2(uint choice) public returns(string) {
TestAccessCtrl obj = new TestAccessCtrl();
return obj.funcExternal(); // externalÖ»ÔÊÐíÔÚÍⲿºÏÔ¼ÖÐÕâÑù¼ä½Óµ÷ÓÃ
}
}
2.1¡¢private
½öÔÚµ±Ç°ºÏԼʹÓÃ,ÇÒ²»¿É±»¼Ì³Ð,˽ÓÐ״̬±äÁ¿Ö»ÄÜ´Óµ±Ç°ºÏÔ¼ÄÚ²¿·ÃÎÊ,ÅÉÉúºÏÔ¼ÄÚ²»ÄÜ·ÃÎÊ¡£
2.2¡¢public
ͬʱ֧³ÖÄÚ²¿ºÍÍⲿµ÷Óá£ÐÞÊÎ״̬±äÁ¿Ê±,×Ô¶¯Éú³ÉͬÃûgetº¯Êý,
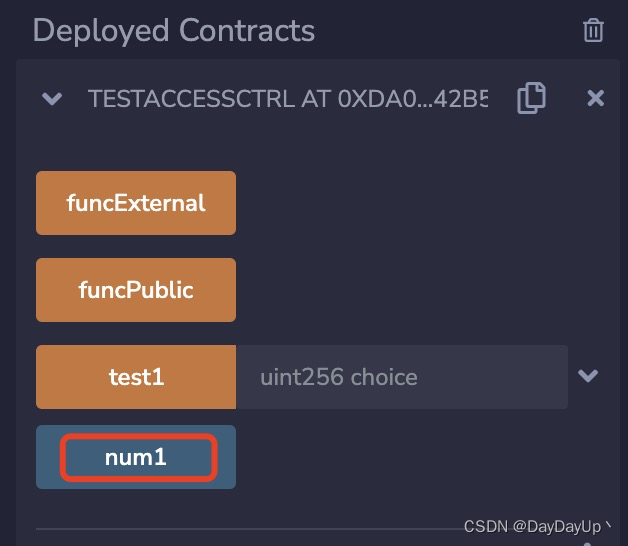
µ«ÔÚ±àÂëʱ²»¿ÉÖ±½Óµ÷ÓÃnum1() ¡£
2.3¡¢internal
Ö»Ö§³ÖÄÚ²¿µ÷ÓÃ,Ò²°üÀ¨ÆäÅÉÉúºÏÔ¼ÄÚ·ÃÎÊ¡£
2.4¡¢external
Íⲿ²Å¿Éµ÷ÓÃ,ÄÚ²¿ÏëÒªµ÷ÓÿÉÒÔÓÃthis ¡£
Èý¡¢º¯ÊýÐÞÊηû
contract TestFuncDecorator {
uint public num = 1;
/// pure
function testPure(uint _num) public pure {
//uint num1 = num; // pure²»ÔÊÐí¶Á״̬±äÁ¿
//num = _num; // pure²»ÔÊÐíÐÞ¸Ä״̬±äÁ¿
}
/// view
function testView(uint _num) public view {
uint num1 = num; // ÔÊÐí¶Á״̬±äÁ¿
num = _num; // 0.4Óï·¨ÉÏÔÊÐíÐÞ¸Ä״̬±äÁ¿,µ«Êµ¼Ê²»»áÐÞ¸Ä,ËùÒÔnum»¹ÊÇ1
// 0.5¼°Ö®ºó²»ÔÊÐíÔÚviewÖÐÕâÑùÐÞ¸Ä,·ñÔò±àÒ벻ͨ¹ý
}
/// payable
function () public payable {}
function getBalance() public view returns(uint) { // balance»ñÈ¡ºÏÔ¼µØÖ·ÏµÄÒÔÌ«±ÒÓà¶î
return address(this).balance;
}
// ³äÖµº¯Êýpayable,Ö»ÓÐÌí¼ÓÕâ¸ö¹Ø¼ü×Ö,²ÅÄÜÔÚÖ´ÐÐÕâ¸öº¯Êýʱ,¸øÕâ¸öºÏÔ¼³äÒÔÌ«±Ò,·ñÔò¸Ãº¯Êý×Ô¶¯¾Ü¾øËùÓз¢Ë͸øËüµÄÒÔÌ«±Ò
function testPayable() payable public { // transferתÕË
address(this).transfer(msg.value);
}
}
3.1¡¢pure
±íÃ÷¸Ãº¯ÊýÊÇ´¿º¯Êý,Á¬×´Ì¬±äÁ¿¶¼²»ÓöÁ,º¯ÊýµÄÔËÐнö½öÒÀÀµÓÚ²ÎÊý¡£²»ÏûºÄgas¡£³Ðŵ²»¶ÁÈ¡»òÐÞ¸Ä״̬,·ñÔò±àÒë³ö´í¡£
3.2¡¢view
ÉèÖÃÁËviewÐÞÊηû,¾ÍÊÇÒ»´Îµ÷ÓÃ,²»ÐèÒªÖ´Ðй²Ê¶¡¢½øÈëEVM,¶øÊÇÖ±½Ó²éѯ±¾µØ½ÚµãÊý¾Ý,Òò´ËÐÔÄÜ»áµÃµ½ºÜ´óÌáÉý¡£²»ÏûºÄgas¡£²»»á·¢Æð½»Ò×,ËùÒÔ²»ÄÜʵ¼Ê¸Ä±ä״̬±äÁ¿¡£
3.3¡¢payable
ÔÊÐíº¯Êý±»µ÷ÓõÄʱºò,ÈúÏÔ¼½ÓÊÕÒÔÌ«±Ò¡£Èç¹ûδָ¶¨,¸Ãº¯Êý½«×Ô¶¯¾Ü¾øËùÓз¢Ë͸øËüµÄÒÔÌ«±Ò¡£
ËÄ¡¢¹¹Ô캯Êý
Ψһ,²»¿ÉÖØÔØ¡£Ò²¿ÉÒÔ½ÓÊÕÈë²Î¡£
pragma solidity ^0.4.25;
contract Test1 {
address private _owner;
constructor() public {
_owner = msg.sender;
}
/**constructor(int num) public { ÖØÔØ¹¹Ôì->±àÒë´íÎó
_owner = msg.sender;
}*/
}
contract Test2 {
uint public num;
constructor(uint x) public { // ´ø²Î¹¹Ôì,ÔÚdeployʱ´«Èë
num = x;
}
}
Îå¡¢ÐÞÊÎÆ÷modifier
·½·¨ÐÞÊÎÆ÷modifier ,ÀàËÆAOP´¦Àí¡£
pragma solidity ^0.4.25;
contract TestModifier {
address private _owner;
bool public endFlag; // Ö´ÐÐÍêtestºóµÄendFlagÈÔÊÇtrue
constructor() public {
_owner = msg.sender;
}
modifier onlyOwner { // ȨÏÞÀ¹½ØÆ÷,·ÇºÏÔ¼²¿ÊðÕ˺ÅÖ´ÐÐtest()Ôò±»À¹½Ø
require(_owner == msg.sender, "Auth: only owner is authorized.");
_; // ÀàËÆ±»´úÀíµÄtest()·½·¨µ÷ÓÃ
endFlag = true;
}
function test() public onlyOwner {
endFlag = false;
}
}
Áù¡¢Êý¾ÝλÖÃ
6.1¡¢memory
ÆäÉúÃüÖÜÆÚÖ»´æÔÚÓÚº¯Êýµ÷ÓÃÆÚ¼ä,¾Ö²¿±äÁ¿Ä¬ÈÏ´æ´¢ÔÚÄÚ´æ,²»ÄÜÓÃÓÚÍⲿµ÷Óá£ÄÚ´æÎ»ÖÃÊÇÁÙʱÊý¾Ý,±È´æ´¢Î»ÖñãÒË¡£ËüÖ»ÄÜÔÚº¯ÊýÖзÃÎÊ¡£
ͨ³£,ÄÚ´æÊý¾ÝÓÃÓÚ±£´æÁÙʱ±äÁ¿,ÒÔ±ãÔÚº¯ÊýÖ´ÐÐÆÚ¼ä½øÐмÆËã¡£Ò»µ©º¯ÊýÖ´ÐÐÍê±Ï,ËüµÄÄÚÈݾͻᱻ¶ªÆú¡£Äã¿ÉÒÔ°ÑËüÏëÏó³Éÿ¸öµ¥¶Àº¯ÊýµÄÄÚ´æ(RAM)¡£
6.2¡¢storage
״̬±äÁ¿±£´æµÄλÖÃ,Ö»ÒªºÏÔ¼´æÔÚ¾ÍÒ»Ö±±£´æÔÚÇø¿éÁ´ÖС£¸Ã´æ´¢Î»Öô洢ÓÀ¾ÃÊý¾Ý,ÕâÒâζןÃÊý¾Ý¿ÉÒÔ±»ºÏÔ¼ÖеÄËùÓк¯Êý·ÃÎÊ¡£¿ÉÒÔ°ÑËüÊÓΪ¼ÆËã»úµÄÓ²ÅÌÊý¾Ý,ËùÓÐÊý¾Ý¶¼ÓÀ¾Ã´æ´¢¡£
±£´æÔÚ´æ´¢Çø(Storage)ÖеıäÁ¿,ÒÔÖÇÄܺÏÔ¼µÄ״̬´æ´¢,²¢ÇÒÔÚº¯Êýµ÷ÓÃÖ®¼ä±£³Ö³Ö¾ÃÐÔ¡£ÓëÆäËûÊý¾ÝλÖÃÏà±È,´æ´¢ÇøÊý¾ÝλÖõijɱ¾½Ï¸ß¡£
6.3¡¢calldata
calldataÊDz»¿ÉÐ޸ĵÄÖ»¶ÁµÄ·Ç³Ö¾ÃÐÔÊý¾ÝλÖÃ,ËùÓд«µÝ¸øº¯ÊýµÄÖµ,¶¼´æ´¢ÔÚÕâÀï¡£´ËÍâ,calldataÊÇÍⲿº¯ÊýµÄ²ÎÊý(¶ø²»ÊÇ·µ»Ø²ÎÊý)µÄĬÈÏλÖá£
0.4µÄexternalÈë²ÎÉùÃ÷calldataÔò±¨´í,0.5¼°Ö®ºóµÄexternalÈë²Î±ØÐëÉùÃ÷calldata·ñÔò±¨´í¡£
´Ó Solidity 0.6.9 °æ±¾¿ªÊ¼,֮ǰ½öÓÃÓÚÍⲿº¯ÊýµÄcalldataλÖÃ,ÏÖÔÚ¿ÉÒÔÔÚÄÚ²¿º¯ÊýʹÓÃÁË¡£
6.4¡¢stack
¶ÑÕ»ÊÇÓÉEVM (EthereumÐéÄâ»ú)ά»¤µÄ·Ç³Ö¾ÃÐÔÊý¾Ý¡£EVMʹÓöÑÕ»Êý¾ÝλÖÃÔÚÖ´ÐÐÆÚ¼ä¼ÓÔØ±äÁ¿¡£¶ÑջλÖÃ×î¶àÓÐ1024¸ö¼¶±ðµÄÏÞÖÆ¡£
¡¾×ܽ᡿
»¨·Ñgas:storage > memory(calldata) > stack
״̬±äÁ¿×ÜÊÇ´æ´¢ÔÚ´æ´¢ÇøstorageÖС£(ÒþʽµØ±ê¼Ç״̬±äÁ¿µÄλÖÃ)
º¯Êý²ÎÊý(ÖµÀàÐÍ)°üÀ¨·µ»Ø²ÎÊý(ÖµÀàÐÍ)¶¼´æ´¢ÔÚÄÚ´æmemoryÖÐ
ÖµÀàÐ͵ľֲ¿±äÁ¿:Õ»(stack)
ÖµÀàÐ͵ľֲ¿±äÁ¿´æ´¢ÔÚÄÚ´æÖС£µ«ÊÇ,¶ÔÓÚÒýÓÃÀàÐÍ,ÐèÒªÏÔʽµØÖ¸¶¨Êý¾ÝλÖÃ
²»ÄÜÏÔʽÉùÃ÷¾ßÓÐÖµÀàÐ͵ľֲ¿±äÁ¿Îªmemory»¹ÊÇstorage
Æß¡¢Ê¼þevent
¶¨ÒåʹÓÃevent ,ÀàËÆÒ»¸öº¯ÊýÉùÃ÷,µ÷ÓÃÊÔͼemit ,
contract Test {
event testEvent(uint a, uint b, uint c, uint result);
function calc(uint a, uint b, uint c) public returns(uint) {
uint result = a ** b ** c;
emit testEvent(a, b, c, result);
return result;
}
}
ʼþ»áÊä³öÔÚlogs ÖÐ,
[
{
"from": "0x19a0870a66B305BE9917c0F14811C970De18E6fC",
"topic": "0x271cb5fa8dca917938dbd3f2522ef54cf70092ead9e1871225a2b3b407f9a81a",
"event": "testEvent",
"args": {
"0": "2",
"1": "1",
"2": "3",
"3": "8",
"a": "2",
"b": "1",
"c": "3",
"result": "8"
}
}
]
ͨ¹ý¸øeventÐβÎÌí¼Óindexed ,±ãÓÚʼþÌõ¼þµÄɸѡ,indexed ²»Äܳ¬¹ýÈý¸ö,·ñÔò±àÒë³ö´í,
event testEvent(uint indexed a, uint b, uint c, uint result);
°Ë¡¢µ¥Î»ºÍÈ«¾Ö±äÁ¿
ʱ¼äµ¥Î»²»¼ÓĬÈÏs ,ÒÔÌ«±ÒĬÈÏwei ,
function testUnit() pure public {
require(1 == 1 seconds);
require(1 minutes == 60 seconds);
require(1 hours == 60 minutes);
require(1 days == 24 hours);
require(1 weeks == 7 days);
require(1 years == 365 days); // years ´Ó 0.5.0 °æ±¾¿ªÊ¼²»ÔÙÖ§³Ö
require(1 ether == 1000 finney);
require(1 finney == 1000 szabo); // ´Ó0.7.0¿ªÊ¼ finney ºÍ szabo ±»ÒƳýÁË
require(1 szabo == 1e12 wei);
//require(1 gwei == 1e9); // 0.7.0¿ªÊ¼¼ÓÈëgwei
}
È«¾Ö±äÁ¿,
pragma solidity ^0.8.0;
contract TestGlobalVariable {
function test1() public view returns(/**bytes32,*/ uint, uint, address, uint, uint, uint, uint) {
return (
// blockhash(block.number - 1), // Ö¸¶¨Çø¿éµÄÇø¿é¹þÏ£,½ö¿ÉÓÃÓÚ×îÐ嵀 256 ¸öÇø¿éÇÒ²»°üÀ¨µ±Ç°Çø¿é,·ñÔò·µ»Ø0¡£0.5ÒÆ³ýÁËblock.blockhash
block.basefee, // µ±Ç°Çø¿éµÄ»ù´¡·ÑÓÃ
block.chainid, // µ±Ç°Á´ id
block.coinbase, // ÍÚ³öµ±Ç°Çø¿éµÄ¿ó¹¤µØÖ·
block.difficulty, // µ±Ç°Çø¿éÄѶÈ
block.gaslimit, // µ±Ç°Çø¿é gas ÏÞ¶î
block.number, // µ±Ç°Çø¿éºÅ
block.timestamp // ×Ô unix epoch Æðʼµ±Ç°Çø¿éÒÔÃë¼ÆµÄʱ¼ä´Á,0.7.0ÒÆ³ýÁËnow
);
}
function test2() public payable returns(bytes memory, address, bytes4, uint, uint256, uint, address) {
return (
msg.data, // ÍêÕûµÄ calldata
msg.sender, // ÏûÏ¢·¢ËÍÕß(µ±Ç°µ÷ÓÃ)
msg.sig, // calldata µÄǰ 4 ×Ö½Ú(Ò²¾ÍÊǺ¯Êý±êʶ·û)
msg.value, // ËæÏûÏ¢·¢Ë굀 wei µÄÊýÁ¿
gasleft(), // Ê£ÓàµÄ gas,0.5ÒÆ³ýÁËmsg.gas
tx.gasprice, // ½»Ò×µÄ gas ¼Û¸ñ
tx.origin // ½»Ò×·¢ÆðÕß(ÍêÈ«µÄµ÷ÓÃÁ´)
);
}
}
¾Å¡¢Òì³£´¦Àí
9.1¡¢assert
assert(1 == 1 seconds);
9.2¡¢require
require(1 == 1 seconds);
require(1 == 1 seconds, "err");
9.3¡¢revert
if (x != y) revert("x should equal to b");
9.4¡¢try/catch
Solidity0.6°æ±¾Ö®ºó¼ÓÈë¡£try/catch½öÊÊÓÃÓÚÍⲿµ÷ÓÃ,ÁíÍâ,try´óÀ¨ºÅÄڵĴúÂëÊDz»Äܱ»catch²¶»ñµÄ¡£
pragma solidity ^0.6.10;
contract TestTryCatch {
function execute (uint256 amount) external returns(bool){
try this.onlyEvent(amount){
return true;
} catch {
return false;
}
}
function onlyEvent (uint256 a) public {
//code that can revert
require(a % 2 == 0, "Ups! Reverting");
}
}
¡¾assertºÍrequireµÄÑ¡Ôñ¡¿
ÔÚEVMÀï,´¦ÀíassertºÍrequireÁ½ÖÖÒì³£µÄ·½Ê½ÊDz»Ò»ÑùµÄ,ËäÈ»ËûÃǶ¼»á»ØÍË״̬,²»Í¬µã±íÏÖÔÚ:
- gasÏûºÄ²»Í¬¡£assertÀàÐ͵ÄÒì³£»áÏûºÄµôËùÓÐÊ£ÓàµÄgas,¶ørequire²»»áÏûºÄµôÊ£ÓàµÄgas(Ê£ÓàµÄgas»á·µ»¹¸øµ÷ÓÃÕß)
- ²Ù×÷·û²»Í¬¡£µ±assert·¢ÉúÒ쳣ʱ,Solidity»áÖ´ÐÐÒ»¸öÎÞЧ²Ù×÷(ÎÞЧָÁî0xfe)¡£µ±·¢ÉúrequireÀàÐ͵ÄÒ쳣ʱ,Solidity»áÖ´ÐÐÒ»¸ö»ØÍ˲Ù×÷(REVERTÖ¸Áî0xfd)
- ÓÅÏÈʹÓÃrequire()
- ÓÃÓÚ¼ì²éÓû§ÊäÈë¡£
- ÓÃÓÚ¼ì²éºÏÔ¼µ÷Ó÷µ»ØÖµ,Èçrequire(external.send(amount))¡£
- ÓÃÓÚ¼ì²é״̬,Èçmsg.send == owner¡£
- ͨ³£ÓÃÓÚº¯Êý¿ªÍ·¡£
- ²»ÖªµÀʹÓÃÄÄÒ»¸öµÄʱºò,¾ÍʹÓÃrequire¡£
- ÓÅÏÈʹÓÃassert()
- ÓÃÓÚ¼ì²éÒç³ö´íÎó,Èçz = x + y; assert(z >= x);
- ÓÃÓÚ¼ì²é²»Ó¦¸Ã·¢ÉúµÄÒì³£Çé¿ö¡£
- ÓÃÓÚÔÚ״̬¸Ä±äÖ®ºó,¼ì²éºÏԼ״̬¡£
- ¾¡Á¿ÉÙʹÓÃassert¡£
- ͨ³£ÓÃÓÚº¯ÊýÖмä»òÕßβ²¿¡£
Ê®¡¢ÖØÔØ
// ÖØÔØ
function addNums(uint x, uint y) public pure returns(uint) {
return x + y;
}
function addNums(uint x, uint y, uint z) public pure returns(uint) {
return x + y + z;
}
ʮһ¡¢¼Ì³Ð
×ÓºÏÔ¼is ¸¸ºÏÔ¼µÄ¸ñʽ,
pragma solidity ^0.4.25;
contract TestExtendA { // ¸¸ÀàҪдÔÚ×ÓÀà֮ǰ
uint public a;
constructor() public {
a = 1;
}
}
contract TestExtend is TestExtendA {
uint public b;
constructor() public {
b = 2;
}
}
Ö±½ÓÔڼ̳ÐÁбíÖÐÖ¸¶¨»ùÀàµÄ¹¹Ôì²ÎÊý,
contract A { // ¸¸ÀàҪдÔÚ×ÓÀà֮ǰ
uint public x;
constructor(uint _a) public { // ´ø²Î¹¹Ôì
x = _a;
}
}
contract B is A(1) { // Ö¸¶¨¸¸À๹Ôì²ÎÊý
uint public y;
constructor() public {
y = 2;
}
}
ͨ¹ýÅÉÉúºÏÔ¼(×ÓÀà)µÄ¹¹Ô캯ÊýÖÐʹÓÃÐÞÊηû·½Ê½µ÷ÓûùÀàºÏÔ¼,
contract A { // ¸¸ÀàҪдÔÚ×ÓÀà֮ǰ
uint public x;
constructor(uint _a) public { // ´ø²Î¹¹Ôì
x = _a;
}
}
// ·½Ê½Ò»:
contract B1 is A {
uint public b;
constructor() A(1) public { // ×ÓÀ๹ÔìʹÓø¸Àà´ø²ÎÐÞÊηûA(1)
b = 2;
}
}
// ·½Ê½¶þ:
contract B2 is A {
uint public b;
constructor(uint _b) A(_b / 2) public { // ×ÓÀà´ø²Î¹¹ÔìʹÓø¸Àà´ø²ÎÐÞÊηûA(_b / 2)
b = _b;
}
}
Á¬Ðø¼Ì³Ð¡¢¶àÖØ¼Ì³Ð,
/// Á¬Ðø¼Ì³Ð,Z¼Ì³ÐY,YÓּ̳ÐX
contract X {
uint public x;
constructor() public{
x = 1;
}
}
contract Y is X {
uint public y;
constructor() public{
y = 1;
}
}
// Èç¹ûÊǶà¸ö»ùÀàºÏÔ¼Ö®¼äÒ²Óм̳йØÏµ,ÄÇôisºóÃæµÄºÏÔ¼Êéд˳Ðò¾ÍºÜÖØÒª¡£Ë³ÐòÓ¦¸ÃÊÇ,»ùÀàºÏÔ¼ÔÚÇ°Ãæ,ÅÉÉúºÏÔ¼ÔÚºóÃæ,·ñÔòÎÞ·¨±àÒë¡£
// ʵ¼ÊÉÏZÖ»ÐèÒª¼Ì³ÐY¾ÍÐÐ
contract Z is X,Y { // ËùÒÔ±ØÐëÊÇX,Y¶ø²»ÊÇY,X
}
/// ¶àÖØ¼Ì³Ð,×ÓÀà¿ÉÒÔÓµÓжà¸ö»ùÀàµÄÊôÐÔ
contract Father {
uint public x = 180;
}
contract Mother {
uint public y = 170;
}
contract Son is Father, Mother {
}
Ê®¶þ¡¢³éÏóºÏÔ¼
0.6¿ªÊ¼Ö§³Ö¡£
Èç¹ûÒ»¸öºÏÔ¼Óй¹Ô캯Êý,ÇÒÊÇÄÚ²¿(internal)º¯Êý,»òÕߺÏÔ¼°üº¬Ã»ÓÐʵÏֵĺ¯Êý,Õâ¸öºÏÔ¼½«±»±ê¼ÇΪ³éÏóºÏÔ¼,ʹÓùؼü×Öabstract,³éÏóºÏÔ¼ÎÞ·¨³É¹¦²¿Êð,ËûÃÇͨ³£ÓÃ×÷»ùÀàºÏÔ¼¡£
³éÏóºÏÔ¼¿ÉÒÔÉùÃ÷Ò»¸övirtual ´¿Ð麯Êý,´¿Ð麯ÊýûÓоßÌåʵÏÖ´úÂëµÄº¯Êý¡£Æäº¯ÊýÉùÃ÷ÓÃ;½áβ,¶ø²»ÊÇÓÃ{} ½áβ¡£
Èç¹ûºÏÔ¼¼Ì³Ð×Ô³éÏóºÏÔ¼,²¢ÇÒûÓÐͨ¹ýÖØÐ´(override)À´ÊµÏÖËùÓÐδʵÏֵĺ¯Êý,ÄÇôËû±¾Éí¾ÍÊdzéÏóºÏÔ¼µÄ,Òþº¬ÁËÒ»¸ö³éÏóºÏÔ¼µÄÉè¼ÆË¼Â·,¼´ÒªÇóÈκμ̳ж¼±ØÐëʵÏÖÆä·½·¨¡£
//pragma solidity ^0.4.25; // 0.4/0.5²»¼æÈÝ
pragma solidity ^0.6.10;
abstract contract TestAbstractContract {
uint public a;
constructor(uint _a) internal {
a = _a;
}
function get () virtual public;
}
Ê®Èý¡¢ÖØÐ´
0.8ÒÔϲ»Ö§³Ö¡£
ºÏÔ¼ÖеÄÐ麯Êý(º¯ÊýʹÓÃÁËvirtual ÐÞÊεĺ¯Êý)¿ÉÒÔÔÚ×ÓºÏÔ¼ÖØÐ´¸Ãº¯Êý,ÒÔ¸ü¸ÄËûÃÇÔÚ¸¸ºÏÔ¼ÖеÄÐÐΪ¡£ÖØÐ´µÄº¯ÊýÐèҪʹÓùؼü×Öoverride ÐÞÊΡ£
pragma solidity ^0.8.0;
contract TestOverride {
function get() virtual public{}
}
contract Middle is TestOverride {
}
contract Inherited is Middle{
function get() public override{
}
}
¶ÔÓÚ¶àÖØ¼Ì³Ð,Èç¹ûÓжà¸ö¸¸ºÏÔ¼ÓÐÏàͬ¶¨ÒåµÄº¯Êý,override ¹Ø¼ü×Öºó±ØÐëÖ¸¶¨ËùÓеĸ¸ºÏÔ¼Ãû³Æ,
contract Base1 {
function get () virtual public{}
}
contract Base2 {
function get () virtual public{}
}
contract Middle2 is Base1, Base2{ // Ö¸¶¨ËùÓи¸ºÏÔ¼Ãû³Æ
function get() public override( Base1, Base2){
}
}
×¢Òâ:Èç¹ûº¯ÊýûÓбê¼ÇΪvirtual(³ý½Ó¿ÚÍâ,ÒòΪ½Ó¿ÚÀïÃæËùÓеĺ¯Êý»á×Ô¶¯±ê¼ÇΪvirtual),ÄÇôÅÉÉúºÏÔ¼ÊDz»ÄÜÖØÐ´À´¸ü¸Äº¯ÊýÐÐΪµÄ¡£ÁíÍâ,privateµÄº¯ÊýÊDz»¿É±ê¼ÇΪvirtualµÄ¡£
Ê®ËÄ¡¢½Ó¿Ú
0.8ÒÔϲ»Ö§³Ö¡£
½Ó¿ÚºÍ³éÏóºÏÔ¼ÀàËÆ,ÓëÖ®²»Í¬µÄÊÇ,½Ó¿Ú²»ÊµÏÖÈκκ¯Êý,ͬʱ»¹ÓÐÒÔÏÂÏÞÖÆ:
-
ÎÞ·¨¼Ì³ÐÆäËûºÏÔ¼»òÕß½Ó¿Ú -
ÎÞ·¨¶¨Òå¹¹Ô캯Êý -
ÎÞ·¨¶¨Òå±äÁ¿ -
ÎÞ·¨¶¨Òå½á¹¹Ìå -
ÎÞ·¨¶¨Òåö¾Ù
pragma solidity ^0.8.0;
interface TestInterface {
function transfer (address recipient, uint amount) external;
}
contract TestInterfaceSon {
function transfer(address recipient, uint amount) public {
}
}
¾ÍÏñ¼Ì³ÐÆäËûºÏÔ¼Ò»Ñù,ºÏÔ¼¿ÉÒԼ̳нӿÚ,½Ó¿ÚÖеĺ¯Êý»áÒþʽµØ±ê¼ÇΪvirtual,Òâζ×ÅËûÃÇ»á±»ÖØÐ´¡£
Ê®Îå¡¢¿â
¿ª·¢ºÏÔ¼µÄʱºò,×ÜÊÇ»áÓÐһЩº¯Êý¾³£±»¶à¸öºÏÔ¼µ÷ÓÃ,Õâ¸öʱºò¿ÉÒÔ°ÑÕâЩº¯Êý·âװΪһ¸ö¿â,¿âµÄ¹Ø¼ü×ÖÓÃlibrary À´¶¨Òå¡£
Èç¹ûºÏÔ¼ÒýÓõĿ⺯Êý¶¼ÊÇÄÚ²¿(internal)º¯Êý,ÄÇô±àÒëÆ÷ÔÚ±àÒëºÏԼʱ,»á°Ñ¿âº¯ÊýµÄ´úÂëǶÈëµ½ºÏÔ¼Àï,¾ÍÏñºÏÔ¼×Ô¼ºÊµÏÖÁËÕâЩº¯Êý,Õâʱ²¢²»»áµ¥¶À²¿Êð¡£
pragma solidity >=0.4.0 <0.7.0;
//pragma solidity ^0.4.25;
library TestLibrary{
function add (uint a,uint b) internal pure returns (uint){
uint c = a + b;
require(c > a, "SafeMath: addition overflow");
return c;
}
}
¿âµÄµ÷ÓÃ,
contract Test {
function add (uint x, uint y) public pure returns(uint){
return TestLibrary.add(x, y); // µ÷Óÿâ
}
}
³ýÁËʹÓÃÉÏÃæµÄTestLibrary.add(x, y) ÕâÖÖ·½Ê½À´µ÷Óÿ⺯Êý,»¹ÓÐÒ»¸öÊÇʹÓÃusing LibA for B ÕâÖÖ¸½×Å¿âµÄ·½Ê½¡£
Ëü±íʾ°ÑËùÓÐLibAµÄ¿âº¯Êý¹ØÁªµ½Êý¾ÝÀàÐÍB,ÕâÑù¾Í¿ÉÒÔÔÚBÀàÐÍÖ±½Óµ÷Óÿ⺯Êý¡£
contract Test {
using TestLibrary for uint;
//using TestLibrary for *;
function add2 (uint x,uint y) public pure returns (uint){
return x.add(y); // uintµÄÊý¾Ýx¾Í¿ÉÒÔÖ±½Óµ÷ÓÃadd(y)
}
}
0x1¡¢Ê¾Àý´úÂë
ÔÚhttps://remix.ethereum.org/ÏÂ,»ùÓÚ²»Í¬°æ±¾Óï·¨µÄ²îÒì,·Ö±ðÓÃÈý¸öºÏÔ¼Îļþ»ù±¾¸²¸Çµ½ÁËÒÔÉÏÓï·¨,
- Test04.sol => ^0.4.25
- Test06.sol => ^0.6.10
- Test08.sol => ^0.8.0
- Test04.sol,
pragma solidity ^0.4.25;
//pragma solidity ^0.8.0;
/** 1.1.1 */
contract TestBool {
bool flag;
int num1 = 100;
int num2 = 200;
// default false
function getFlag() public view returns(bool) {
return flag; // false
}
// ·Ç
function getFlag2() public view returns(bool) {
return !flag; // true
}
// Óë
function getFlagAnd() public view returns(bool) {
return (num1 != num2) && !flag; // true
}
// »ò
function getFlagOr() public view returns(bool) {
return (num1 == num2) || !flag; // true
}
}
/** 1.1.2 */
// ÕûÐÍÌØÐÔÓëÔËËã
contract TestInteger {
int num1; // ÓзûºÅÕûÐÍ int256
uint num2; // ÎÞ·ûºÅÕûÐÍ uint256
function add(uint _a, uint _b) public pure returns(uint) {
return _a + _b;
}
function sub(uint _a, uint _b) public pure returns(uint) {
return _a - _b;
}
function mul(uint _a, uint _b) public pure returns(uint) {
return _a * _b;
}
function div(uint _a, uint _b) public pure returns(uint) {
return _a / _b; // ÔÚsolidityÖÐ,³ý·¨ÊÇ×öÕû³ý,ûÓÐСÊýµã
}
function rem(uint _a, uint _b) public pure returns(uint) {
return _a % _b;
}
function square(uint _a, uint _b) public pure returns(uint) {
return _a ** _b; // ÃÝÔËËãÔÚ0.8.0Ö®ºó,±äΪÓÒÓÅÏÈ,¼´a ** b ** c => a ** (b ** c)
}
function max() public view returns(uint) {
return uint(-1);
// return type(uint).max; // 0.8²»ÔÙÔÊÐíuint(-1)
}
}
// λÔËËã
contract TestBitwise {
uint8 num1 = 3;
uint8 num2 = 4;
function bitAdd() public view returns(uint) {
return num1 & num2;
}
function bitOr() public view returns(uint) {
return num1 | num2;
}
function unBit() public view returns(uint) {
return ~num1;
}
function bitXor() public view returns(uint) {
return num1 ^ num2;
}
function bitRight() public view returns(uint) {
return num1 >> 1;
}
function bitLeft() public view returns(uint) {
return num1 << 1;
}
}
/** 1.1.3 - 1.1.6 */
contract TestType {
fixed num;
ufixed num2;
fixed8x8 decimal; // fixedMxN, M±íʾλ¿í,±ØÐëλ8µÄÕûÊý±¶,N±íʾʮ½øÖÆÐ¡Êý²¿·ÖµÄλÊý
address addr = msg.sender;
address addr2 = 0xdCad3a6d3569DF655070DEd06cb7A1b2Ccd1D3AF;
TestBitwise t = TestBitwise(addr);
enum ActionChoices { Up, Down, Left, Right }
}
/** 1.1.7 */
// ¹Ì¶¨³¤¶ÈµÄ×Ö½ÚÊý×é(¾²Ì¬),ÒÔ¼°×ª»»ÎªstringÀàÐÍ
contract TestBytesFixed {
// public ×Ô¶¯Éú³ÉͬÃûµÄget·½·¨
bytes1 public num1 = 0x7a; // 1 byte = 8 bit
bytes1 public num2 = 0x68;
bytes2 public num3 = 0x128b; // 2 byte = 16 bit
// »ñÈ¡×Ö½ÚÊý×鳤¶È
function getLength() public view returns(uint) {
return num3.length; // 2
}
// ×Ö½ÚÊý×é±È½Ï
function compare() public view returns(bool) {
return num1 < num2;
}
// ×Ö½ÚÊý×éλÔËËã
function bitwise() public view returns(bytes1,bytes1,bytes1) { // ¶à·µ»ØÖµ
return ((num1 & num2), (num1 | num2), (~num1));
}
// ÏÈתΪbytes¶¯Ì¬Êý×é,ÔÙͨ¹ýstring¹¹Ôì 0x7a7a -> zz
function toString(bytes2 _val) public pure returns(string) {
bytes memory buf = new bytes(_val.length);
for (uint i = 0; i < _val.length; i++) {
buf[i] = _val[i];
}
return string(buf);
}
}
// ¹Ì¶¨³¤¶ÈµÄ×Ö½ÚÊý×é(¾²Ì¬)À©ÈݺÍѹËõ
contract TestBytesExpander {
// public ×Ô¶¯Éú³ÉͬÃûµÄget·½·¨
bytes6 name = 0x796f7269636b;
function changeTo1() public view returns(bytes1) {
return bytes1(name); // 0x79
}
function changeTo2() public view returns(bytes2) {
return bytes2(name); // 0x796f
}
function changeTo16() public view returns(bytes16) {
return bytes16(name); // 0x796f7269636b00000000000000000000
}
}
/** 1.2.1 */
// ÐÞ¸ÄstringÀàÐ͵ÄÊý¾Ý
contract TestString {
string name = 'yorick'; // ×Ö·û´®¿ÉÒÔʹÓõ¥ÒýºÅ»òÕßË«ÒýºÅ
string name2 = "!@#$%^&"; // ÌØÊâ×Ö·ûÕ¼1¸öbyte
string name3 = "ÕÅÈý"; // ÖÐÎÄÔÚstringÖÐʹÓÃutf8µÄ±àÂ뷽ʽ´æ´¢,Õ¼ÓÃ3¸öbyte -> Çл»µ½0.8ÔòÖÐÎÄ×Ö·û±¨´í
// string memory str = unicode"Hello 😃"; // 0.7.0Ö§³ÖUnicode×Ö·û´®
// string memory str2 = unicode"\u20ac"; // 0.7.0Ö§³ÖUnicode×Ö·û´®
function getLength() view public returns(uint) {
// ²»¿ÉÒÔÖ±½Ó»ñÈ¡stringµÄlength
return bytes(name).length; // 6
}
function getLength2() view public returns(uint) {
return bytes(name2).length; // 7
}
function getLength3() view public returns(uint) {
return bytes(name3).length; // 6
}
function getElmName() view public returns(bytes1) {
// ²»¿ÉÒÔÖ±½Óͨ¹ýÊý×éϱêname[0]»ñÈ¡
return bytes(name)[0]; // 0x79
}
function changeElmName() public {
bytes(name)[0] = "h";
}
function getName() view public returns(bytes) {
return bytes(name);
}
}
/** 1.2.2 */
// ¶¯Ì¬µÄ×Ö½ÚÊý×é,ÒÔ¼°×ª»»ÎªstringÀàÐÍ
contract TestBytesDynamic {
bytes public dynamicBytes;
function setDynamicBytes(string memory val) public {
dynamicBytes = bytes(val);
}
function getVal() public view returns(string){
return string(dynamicBytes);
}
}
/** 1.2.3 */
// ¶¨³¤Êý×é
contract TestArrFixed {
uint[5] arr = [1,2,3,4,5];
// ÐÞ¸ÄÊý×éÔªËØÄÚÈÝ
function modifyElements() public {
arr[0] = 12;
arr[1] = 14;
}
// ²é¿´Êý×é
function watchArr() public view returns(uint[5]) {
return arr;
}
// Êý×éÔªËØÇóºÍ¼ÆËã
function sumArr() public view returns(uint) {
uint sum = 0;
for (uint i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
// Êý×鳤¶È
function getLength() public view returns(uint) {
return arr.length;
}
// deleteÖØÖÃÊý×éijϱêµÄÔªËØÖµ,²»»áÕæÕýɾ³ý¸ÃÔªËØ
function deleteElm(uint idx) public {
delete arr[idx];
}
// deleteÖØÖÃÕû¸öÊý×é
function deleteArr() public {
delete arr;
}
/** ¶¨³¤Êý×é²»ÔÊÐí¸Ä±ä³¤¶ÈºÍpush
// ѹËõÊý×éºó,ÓÒ²à¶àÓàÔªËØ±»¶ªÆú
function changeLengthTo1() public {
arr.length = 1;
}
// À©ÈÝÊý×éºó,ÓÒ²àÔªËØ²¹0
function changeLengthTo10() public {
arr.length = 10;
}
// ×·¼ÓÐÂÔªËØ
function pushElm(uint _elm) public {
arr.push(_elm);
}
*/
}
// ±ä³¤Êý×é
contract TestArrDynamic {
uint[] arr = [1,2,3,4,5];
// ²é¿´Êý×é
function watchArr() public view returns(uint[] memory) {
return arr;
}
// Êý×鳤¶È
function getLength() public view returns(uint) {
return arr.length;
}
// ѹËõÊý×éºó,ÓÒ²à¶àÓàÔªËØ±»¶ªÆú
function changeLengthTo1() public {
arr.length = 1;
}
// À©ÈÝÊý×éºó,ÓÒ²àÔªËØ²¹0
function changeLengthTo10() public {
arr.length = 10;
}
// ×·¼ÓÐÂÔªËØ
function pushElm(uint _elm) public {
arr.push(_elm);
}
/**
// µ¯³öÔªËØ
function popElm(uint _elm) public {
arr.pop(); // 0.4²»Ö§³Öpop
}
*/
// deleteÖØÖÃÊý×éijϱêµÄÔªËØÖµ,²»»áÕæÕýɾ³ý¸ÃÔªËØ
function deleteElm(uint idx) public {
delete arr[idx];
}
// deleteÖØÖÃÕû¸öÊý×é
function deleteArr() public {
delete arr;
}
}
/**
¶þάÊý×é
solidityµÄ¶þάÊý×éÓëÆäËûÓïÑÔ²»Í¬,[2] [3]±íʾ3ÐÐ2ÁÐ,¶øÆäËûÓïÑÔΪ2ÐÐ3ÁÐ;
¶þά¶¯Ì¬Êý×éÓëһάÊý×éÀàËÆ,¿ÉÒԸıäÆäÊý×鳤¶È;
*/
contract TestArr2Dimensional {
uint[2][3] arr = [[1,2],[3,4],[5,6]];
function getRowSize() public view returns(uint) {
return arr.length; // 3
}
function getColSize() public view returns(uint) {
return arr[0].length; // 2
}
function watchArr() public view returns(uint[2][3]) {
return arr; // 1,2,3,4,5,6
}
function sumArr() public view returns(uint) {
uint sum = 0;
for (uint i = 0; i < getRowSize(); i++) {
for (uint j = 0; j < getColSize(); j++) {
sum += arr[i][j];
}
}
return sum;
}
function modifyArr() public {
arr[0][0] = 99;
}
}
// Êý×é×ÖÃæÖµ
contract TestArrLiteral {
// ×îС´æ´¢Æ¥Åä,䳬¹ý255,ËùÒÔʹÓÃuint8´æ´¢
function getLiteral8() pure public returns(uint8[3]) {
return [1,2,3];
}
// ³¬¹ý255,ËùÒÔʹÓÃuint16´æ´¢
function getLiteral16() pure public returns(uint16[3]) {
return [256,2,3]; // [255,2,3] ²»±»uint16ÔÊÐí
}
// Ç¿ÖÆ×ª»»Îªuint256
function getLiteral256() pure public returns(uint[3]) {
return [uint(1),2,3]; // ¸øÈÎÒâÔªËØÇ¿×ª¼´¿É,·ñÔò²»±»ÔÊÐí
}
// ¼ÆËãÍâ½ç´«ÈëµÄÄÚÈÝ
function addLiterals(uint[3] arr) pure external returns(uint) {
uint sum = 0;
for (uint i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
}
/** 1.2.4 */
// ½á¹¹Ìå³õʼ»¯µÄÁ½ÖÖ·½·¨
contract TestStruct {
struct Student {
uint id;
string name;
mapping(uint=>string) map;
}
// ĬÈÏΪstorageÀàÐÍ,Ö»ÄÜͨ¹ýstorageÀàÐͲÙ×÷½á¹¹ÌåÖеÄmappingÀàÐÍÊý¾Ý
Student storageStu;
// mappingÀàÐÍ¿ÉÒÔ²»ÓÃÔÚ¶¨ÒåµÄʱºò¸³Öµ,¶øÆäËûÀàÐͲ»¸³ÖµÔò»á±¨´í
function init() public pure returns(uint, string) {
Student memory stu = Student(100, "Jay");
return (stu.id, stu.name);
}
function init2() public pure returns(uint, string) {
Student memory stu = Student({name: "Jay", id: 100});
return (stu.id, stu.name);
}
function init3() public returns(uint, string, string) {
Student memory stu = Student({name: "Jay", id: 100});
// Ö±½Ó²Ù×÷½á¹¹ÌåÖеÄmapping²»±»ÔÊÐí: Student memory out of storage
// stu.map[1] = "artist";
// ͨ¹ýstorageÀàÐ͵ıäÁ¿²Ù×÷½á¹¹ÌåÖеÄmapping
storageStu = stu;
storageStu.map[1] = "artist";
return (storageStu.id, storageStu.name, storageStu.map[1]);
}
// ½á¹¹Ìå×÷ΪÈë²Îʱ,ΪmemoryÀàÐÍ,²¢ÇÒ²»ÄÜʹÓÃpublic»òexternalÐÞÊκ¯Êý:Internal or recursive type is not allowed for public or external functions.
// ¸³ÖµÊ±Ò²ÒªÖ¸¶¨Îªmemory,·ñÔò±¨´í
function testIn(Student stu) internal returns(uint) {
return stu.id;
}
// ½á¹¹Ìå×÷Ϊ³ö²Î,ͬÑùÖ»ÄÜprivate»òinternalÉùÃ÷ÄÚ²¿Ê¹ÓÃ
function testOut(Student stu) private returns(Student) {
return stu;
}
}
/** 1.3 */
contract TestMapping {
mapping(address => uint) private scores; // <ѧÉú,·ÖÊý>µÄµ¥²ãÓ³Éä
mapping(address => mapping(bytes32 => uint8)) private _scores; // <ѧÉú,<¿ÆÄ¿,·ÖÊý>>µÄÁ½²ãÓ³Éä
function getScore() public view returns(address, uint) {
address addr = msg.sender;
return (addr, scores[addr]);
}
function setScore() public {
scores[msg.sender] = 100;
}
}
/** ¶þ */
contract TestAccessCtrl {
constructor () public {}
uint public num1 = 1; // ×Ô¶¯ÎªpublicÉú³ÉͬÃûµÄgetº¯Êý,µ«ÔÚ±àÂëʱ²»¿ÉÖ±½Óµ÷ÓÃnum1()
uint private num2 = 2;
uint num3 = 3; // ²»Ð´ÔòĬÈÏprivate
function funcPublic() public returns(string) {
return "public func";
}
function funcPrivate() private returns(string) {
return "private func";
}
function funcInternal() internal returns(string) {
return "internal func";
}
function funcExternal() external returns(string) {
return "external func";
}
function test1(uint choice) public returns(string) {
if (choice == 1) return funcPublic();
if (choice == 2) return funcPrivate();
if (choice == 3) return funcInternal();
//if (choice == 4) return funcExternal(); // external²»ÔÊÐíÖ±½ÓÔÚÄÚ²¿ÓÃ
if (choice == 4) return this.funcExternal(); // ¼ä½Óͨ¹ýthis²Å¿ÉÒÔµ÷ÓÃexternal
}
}
contract TestAccessCtrlSon is TestAccessCtrl {
function test2(uint choice) public returns(string) {
if (choice == 1) return funcPublic(); // publicÔÊÐíÅÉÉúºÏԼʹÓÃ
//if (choice == 2) return funcPrivate(); // private²»ÔÊÐíÅÉÉúºÏԼʹÓÃ
if (choice == 3) return funcInternal(); // internalÔÊÐíÅÉÉúºÏԼʹÓÃ
//if (choice == 4) return funcExternal(); // externalÒ²²»ÔÊÐíÅÉÉúºÏÔ¼Ö±½ÓʹÓÃ
}
}
contract TestAccessCtrl2 {
function test2(uint choice) public returns(string) {
TestAccessCtrl obj = new TestAccessCtrl();
if (choice == 4) {
return obj.funcExternal(); // externalÖ»ÔÊÐíÔÚÍⲿºÏÔ¼ÖÐÕâÑù¼ä½Óµ÷ÓÃ
} else return "0x0";
}
}
/** Èý */
contract TestFuncDecorator {
uint public num = 1;
/// pure
function testPure(uint _num) public pure {
//uint num1 = num; // pure²»ÔÊÐí¶Á״̬±äÁ¿
//num = _num; // pure²»ÔÊÐíÐÞ¸Ä״̬±äÁ¿
}
/// view
function testView(uint _num) public view {
uint num1 = num; // ÔÊÐí¶Á״̬±äÁ¿
num = _num; // 0.4Óï·¨ÉÏÔÊÐíÐÞ¸Ä״̬±äÁ¿,µ«Êµ¼Ê²»»áÐÞ¸Ä,ËùÒÔnum»¹ÊÇ1
// 0.5¼°Ö®ºó²»ÔÊÐíÔÚviewÖÐÕâÑùÐÞ¸Ä,·ñÔò±àÒ벻ͨ¹ý
}
/// payable
function () public payable {}
function getBalance() public view returns(uint) { // balance»ñÈ¡ºÏÔ¼µØÖ·ÏµÄÒÔÌ«±ÒÓà¶î
return address(this).balance;
}
// ³äÖµº¯Êýpayable,Ö»ÓÐÌí¼ÓÕâ¸ö¹Ø¼ü×Ö,²ÅÄÜÔÚÖ´ÐÐÕâ¸öº¯Êýʱ,¸øÕâ¸öºÏÔ¼³äÒÔÌ«±Ò,·ñÔò¸Ãº¯Êý×Ô¶¯¾Ü¾øËùÓз¢Ë͸øËüµÄÒÔÌ«±Ò
function testPayable() payable public { // transferתÕË
address(this).transfer(msg.value);
}
}
/** ËÄ */
contract TestConstruct1 {
address private _owner;
constructor() public {
_owner = msg.sender;
}
/**constructor(int num) public { // ÖØÔØ¹¹Ôì->±àÒë´íÎó
_owner = msg.sender;
}*/
/**
function TestFuncDecorator(uint x) {} // 0.5֮ǰ»¹¿ÉÒÔÓÃͬÃûº¯Êý¶¨Òå
*/
}
contract TestConstruct2 {
uint public num;
constructor(uint x) public { // ´ø²Î¹¹Ôì,ÔÚdeployʱ´«Èë
num = x;
}
}
/** Îå */
contract TestModifier {
address private _owner;
bool public endFlag; // Ö´ÐÐÍêtestºóµÄendFlagÈÔÊÇtrue
constructor() public {
_owner = msg.sender;
}
modifier onlyOwner { // ȨÏÞÀ¹½ØÆ÷,·ÇºÏÔ¼²¿ÊðÕ˺ÅÖ´ÐÐtest()Ôò±»À¹½Ø
require(_owner == msg.sender, "Auth: only owner is authorized.");
_; // ÀàËÆ±»´úÀíµÄtest()·½·¨µ÷ÓÃ
endFlag = true;
}
function test() public onlyOwner {
endFlag = false;
}
}
/** Æß */
contract TestEvent {
event testEvent(uint indexed a, uint indexed b, uint indexed c, uint result); // indexed²»Äܳ¬¹ýÈý¸ö
function calc(uint a, uint b, uint c) public returns(uint) {
uint result = a ** b ** c;
emit testEvent(a, b, c, result); // ʼþ»áÊä³öÔÚlogsÖÐ
return result;
}
}
/** °Ë */
contract TestUnit {
function testUnit() pure public {
require(1 == 1 seconds);
require(1 minutes == 60 seconds);
require(1 hours == 60 minutes);
require(1 days == 24 hours);
require(1 weeks == 7 days);
require(1 years == 365 days); // years ´Ó 0.5.0 °æ±¾¿ªÊ¼²»ÔÙÖ§³Ö
require(1 ether == 1000 finney);
require(1 finney == 1000 szabo);
require(1 szabo == 1e12 wei);
//require(1 gwei == 1e9); // 0.7.0¿ªÊ¼¼ÓÈëgwei
}
}
/** ¾Å */
contract TestException {
function testAssert(int x) public pure {
assert(x >= 0);
}
function testRequire(int x) public pure {
require(x >= 0);
//require(x >= 0, "x < 0");
}
function testRevert(int x, int y) public pure {
if (x != y) revert("x should equal to b");
}
}
/** Ê® */
contract TestOverload { // ÖØÔØ
function addNums(uint x, uint y) public pure returns(uint) {
return x + y;
}
function addNums(uint x, uint y, uint z) public pure returns(uint) {
return x + y + z;
}
}
/** ʮһ */
contract TestExtendA { // ¸¸ÀàTestExtendAҪдÔÚ×ÓÀàTestExtend֮ǰ
uint public a;
constructor() public {
a = 1;
}
}
contract TestExtend is TestExtendA {
uint public b;
constructor() public {
b = 2;
}
}
/// Ö±½ÓÔڼ̳ÐÁбíÖÐÖ¸¶¨»ùÀàµÄ¹¹Ôì²ÎÊý
contract A { // ¸¸ÀàҪдÔÚ×ÓÀà֮ǰ
uint public x;
constructor(uint _a) public { // ´ø²Î¹¹Ôì
x = _a;
}
}
contract B is A(1) { // Ö¸¶¨¸¸À๹Ôì²ÎÊý
uint public y;
constructor() public {
y = 2;
}
}
/// ͨ¹ýÅÉÉúºÏÔ¼(×ÓÀà)µÄ¹¹Ô캯ÊýÖÐʹÓÃÐÞÊηû·½Ê½µ÷ÓûùÀàºÏÔ¼
// ·½Ê½Ò»:
contract B1 is A {
uint public b;
constructor() A(1) public { // ×ÓÀ๹ÔìʹÓø¸Àà´ø²ÎÐÞÊηûA(1)
b = 2;
}
}
// ·½Ê½¶þ:
contract B2 is A {
uint public b;
constructor(uint _b) A(_b / 2) public { // ×ÓÀà´ø²Î¹¹ÔìʹÓø¸Àà´ø²ÎÐÞÊηûA(_b / 2)
b = _b;
}
}
/// Á¬Ðø¼Ì³Ð,Z¼Ì³ÐY,YÓּ̳ÐX
contract X {
uint public x;
constructor() public{
x = 1;
}
}
contract Y is X {
uint public y;
constructor() public{
y = 1;
}
}
// Èç¹ûÊǶà¸ö»ùÀàºÏÔ¼Ö®¼äÒ²Óм̳йØÏµ,ÄÇôisºóÃæµÄºÏÔ¼Êéд˳Ðò¾ÍºÜÖØÒª¡£Ë³ÐòÓ¦¸ÃÊÇ,»ùÀàºÏÔ¼ÔÚÇ°Ãæ,ÅÉÉúºÏÔ¼ÔÚºóÃæ,·ñÔòÎÞ·¨±àÒë¡£
// ʵ¼ÊÉÏZÖ»ÐèÒª¼Ì³ÐY¾ÍÐÐ
contract Z is X,Y { // ËùÒÔ±ØÐëÊÇX,Y¶ø²»ÊÇY,X
}
/// ¶àÖØ¼Ì³Ð,×ÓÀà¿ÉÒÔÓµÓжà¸ö»ùÀàµÄÊôÐÔ
contract Father {
uint public x = 180;
}
contract Mother {
uint public y = 170;
}
contract Son is Father, Mother {
}
/** Ê®Îå */
library TestLibrary{
function add (uint a,uint b) internal pure returns (uint){
uint c = a + b;
require(c > a, "Math: addition overflow");
return c;
}
}
// ¿âµÄµ÷ÓÃ
contract TestLibraryCall {
function add(uint x, uint y) public pure returns(uint){
return TestLibrary.add(x, y); // µ÷Óÿâ
}
}
// ³ýÁËʹÓÃÉÏÃæµÄTestLibrary.add(x, y)ÕâÖÖ·½Ê½À´µ÷Óÿ⺯Êý,»¹ÓÐÒ»¸öÊÇʹÓÃusing LibA for BÕâÖÖ¸½×Å¿âµÄ·½Ê½¡£
// Ëü±íʾ°ÑËùÓÐLibAµÄ¿âº¯Êý¹ØÁªµ½Êý¾ÝÀàÐÍB,ÕâÑù¾Í¿ÉÒÔÔÚBÀàÐÍÖ±½Óµ÷Óÿ⺯Êý¡£
contract TestLibraryUsing {
using TestLibrary for uint;
//using TestLibrary for *;
function add2(uint x,uint y) public pure returns (uint){
return x.add(y); // uintµÄÊý¾Ýx¾Í¿ÉÒÔÖ±½Óµ÷ÓÃadd(y)
}
}
- Test06.sol,
pragma solidity ^0.6.10;
/** 1.2.3 */
contract TestArrDynamic {
uint[] arr = [1,2,3,4,5];
// µ¯³öÔªËØ
function popElm() public {
arr.pop();
}
function watchArr() public view returns(uint[] memory) {
return arr;
}
/**
0.6¿ªÊ¼²»ÔÙ¿ÉÒÔͨ¹ýÐÞ¸Älength¸Ä±äÊý×鳤¶È,ÐèҪͨ¹ýpush(),push(value),popµÄ·½Ê½,»òÕ߸³ÖµÒ»¸öÍêÕûµÄÊý×é
*/
/**function changeLengthTo1() public {
arr.length = 1;
}*/
}
/** ¾Å */
// Solidity0.6°æ±¾Ö®ºó¼ÓÈë¡£try/catch½öÊÊÓÃÓÚÍⲿµ÷ÓÃ,ÁíÍâ,try´óÀ¨ºÅÄڵĴúÂëÊDz»Äܱ»catch²¶»ñµÄ¡£
contract TestTryCatch {
function execute (uint256 amount) external returns(bool) {
try this.run(amount) { // ÕâÀïµÄº¯ÊýÒì³£»á±»²¶»ñ
return true; // ÕâÀïµÄÒì³£²»Ôٻᱻ²¶»ñ
} catch {
return false;
}
}
function run(uint256 a) public {
//code that can revert
require(a % 2 == 0, "Ups! Reverting");
}
}
/** Ê®¶þ */
// 0.6ºóÖ§³Ö¡£³éÏóºÏÔ¼²»ÄÜʹÓÃnew´´½¨
abstract contract TestAbstractContract {
uint public a;
constructor(uint _a) internal {
a = _a;
}
function get () virtual public;
}
- Test08.sol,
pragma solidity ^0.8.0;
/** 1.2.1 */
contract TestString {
function test() public view returns(string memory, string memory, string memory) {
string memory str = unicode"Hello 😃"; // Hello 😃
string memory str2 = unicode"\u20ac"; // €
string memory str3 = hex"414243444546474849"; // ABCDEFGHI
// string memory name3 = "ÕÅÈý"; // 0.8²»ÔÊÐíÖÐÎÄ×Ö·û,±ØÐë¸ÄΪunicode
return (str, str2, str3);
}
}
/** °Ë */
contract TestGlobalVariable {
function test1() public view returns(/**bytes32,*/ uint, uint, address, uint, uint, uint, uint) {
return (
// blockhash(block.number - 1), // Ö¸¶¨Çø¿éµÄÇø¿é¹þÏ£,½ö¿ÉÓÃÓÚ×îÐ嵀 256 ¸öÇø¿éÇÒ²»°üÀ¨µ±Ç°Çø¿é,·ñÔò·µ»Ø0¡£0.5ÒÆ³ýÁËblock.blockhash
block.basefee, // µ±Ç°Çø¿éµÄ»ù´¡·ÑÓÃ
block.chainid, // µ±Ç°Á´ id
block.coinbase, // ÍÚ³öµ±Ç°Çø¿éµÄ¿ó¹¤µØÖ·
block.difficulty, // µ±Ç°Çø¿éÄѶÈ
block.gaslimit, // µ±Ç°Çø¿é gas ÏÞ¶î
block.number, // µ±Ç°Çø¿éºÅ
block.timestamp // ×Ô unix epoch Æðʼµ±Ç°Çø¿éÒÔÃë¼ÆµÄʱ¼ä´Á,0.7.0ÒÆ³ýÁËnow
);
}
function test2() public payable returns(bytes memory, address, bytes4, uint, uint256, uint, address) {
return (
msg.data, // ÍêÕûµÄ calldata
msg.sender, // ÏûÏ¢·¢ËÍÕß(µ±Ç°µ÷ÓÃ)
msg.sig, // calldata µÄǰ 4 ×Ö½Ú(Ò²¾ÍÊǺ¯Êý±êʶ·û)
msg.value, // ËæÏûÏ¢·¢Ë굀 wei µÄÊýÁ¿
gasleft(), // Ê£ÓàµÄ gas,0.5ÒÆ³ýÁËmsg.gas
tx.gasprice, // ½»Ò×µÄ gas ¼Û¸ñ
tx.origin // ½»Ò×·¢ÆðÕß(ÍêÈ«µÄµ÷ÓÃÁ´)
);
}
}
/** Ê®Èý */
// ºÏÔ¼ÖеÄÐ麯Êý(º¯ÊýʹÓÃÁËvirtualÐÞÊεĺ¯Êý)¿ÉÒÔÔÚ×ÓºÏÔ¼ÖØÐ´¸Ãº¯Êý,ÒÔ¸ü¸ÄËûÃÇÔÚ¸¸ºÏÔ¼ÖеÄÐÐΪ¡£ÖØÐ´µÄº¯ÊýÐèҪʹÓùؼü×ÖoverrideÐÞÊΡ£
// 0.8ÒÔϲ»Ö§³Ö¡£
contract TestOverride {
function get() virtual public{}
}
contract Middle is TestOverride {
}
contract Inherited is Middle {
function get() public override {
}
}
// ¶ÔÓÚ¶àÖØ¼Ì³Ð,Èç¹ûÓжà¸ö¸¸ºÏÔ¼ÓÐÏàͬ¶¨ÒåµÄº¯Êý,override¹Ø¼ü×Öºó±ØÐëÖ¸¶¨ËùÓеĸ¸ºÏÔ¼Ãû³Æ
contract Base1 {
function get() virtual public {}
}
contract Base2 {
function get() virtual public {}
}
contract Middle2 is Base1, Base2 { // Ö¸¶¨ËùÓи¸ºÏÔ¼Ãû³Æ
function get() public override (Base1, Base2){
}
}
/** Ê®ËÄ */
// 0.8ÒÔϲ»Ö§³Ö¡£
interface TestInterface {
function transfer(address recipient, uint amount) external;
}
contract TestInterfaceSon {
function transfer(address recipient, uint amount) public {
}
}
0x2¡¢¸÷°æ±¾Ö÷Òª±ä»¯
0.5.0
- sha3¸ÄÓÃkeccak256, keccak256Ö»ÔÊÐí½ÓÊÕÒ»¸ö²ÎÊý,ʹÓÃabi.encodePackedµÈ×éºÏparams
- ¹¹Ô캯ÊýÓÉͬÃû¿Õ²Î·½·¨±ä³Éconstructor
0.6.0
- ½ö±ê¼ÇvirtualµÄ½Ó¿Ú²Å¿ÉÒÔ±»¸²¸Ç,¸²¸ÇʱÐèҪʹÓÃйؼü×Öoverride,Èç¹û¶à¸ö»ùÀàͬ·½·¨Ãûʱ,ÐèÒªÏñÕâÑùÁгö override(Base1, Base2)
- ²»ÄÜͨ¹ýÐÞ¸ÄlengthÀ´ÐÞ¸ÄÊý×鳤¶È,ÐèҪͨ¹ýpush(),push(value),popµÄ·½Ê½,»òÕ߸³ÖµÒ»¸öÍêÕûµÄÊý×é
- ʹÓÃabstract±êʶ³éÏóºÏÔ¼,³éÏóºÏÔ¼²»ÄÜʹÓÃnew´´½¨
- »Øµ÷º¯ÊýÓÉfunction()²ð·ÖΪfallback()ºÍreceive()
- ÐÂÔötry/catch,¿É¶Ôµ÷ÓÃʧ°Ü×öÒ»¶¨´¦Àí
- Êý×éÇÐÆ¬,ÀýÈç: abi.decode(msg.data[4:], (uint, uint)) ÊÇÒ»¸ö¶Ôº¯Êýµ÷ÓÃpayload½øÐнâÂëµ×²ã·½·¨
- payable(x) °Ñ address ת»»Îª address payable
0.7.0
- call·½Ê½µ÷Ó÷½·¨ÓÉx.f.gas(1000).value(1 ether)(arg1,arg2)¸Ä³É x.f{gas:1000,value:1 ether}(arg1,arg2)
- now ²»ÍƼöʹÓÃ,¸ÄÓÃblock.timestamp
- gweiÔö¼ÓΪ¹Ø¼ü×Ö
- ×Ö·û´®Ö§³ÖASCII×Ö·û,Unicode×Ö·û´®
- ¹¹Ô캯Êý²»ÔÚÐèÒª publicÐÞÊηû,ÈçÐè·ÀÖ¹´´½¨,¿É¶¨Òå³Éabstract
- ²»ÔÊÐíÔÚͬһ¼Ì³Ð²ã´Î½á¹¹ÖоßÓÐͬÃûͬ²ÎÊýÀàÐ͵Ķà¸öʼþ
- using A for B,Ö»ÔÚµ±Ç°ºÏÔ¼ÓÐЧ, ÒÔǰÊÇ»á¼Ì³ÐµÄ,ÏÖÔÚÐèҪʹÓõĵط½,¶¼µÃÉùÃ÷Ò»´Î
0.8.0
- ÆúÓÃsafeMath,ĬÈϼÓÁËÒç³ö¼ì²é,ÈçÐè²»Òª¼ì²éʹÓÃ
unchecked { ... } , ¿ÉÒÔ½ÚÊ¡¶ª¶ªÊÖÐø·Ñ - ĬÈÏÖ§³ÖABIEncoderV2,²»ÔÙÐèÒªÉùÃ÷
- ÇóÃÝÊÇÓÒ½áºÏµÄ,¼´±í´ïʽ
a**b**c ±»½âÎöΪa**(b**c) ¡£ÔÚ 0.8.0 ֮ǰ,Ëü±»½âÎöΪ(a**b)**c - assert ²»ÔÚÏûºÄÍê ÍêÕûµÄgas,¹¦ÄܺÍrequire»ù±¾Ò»ÖÂ,µ«ÊÇtry/catch´íÎóÀïÃæÌåÏÖ²»Ò»Ñù,»¹ÓÐÒ»¶¨×÷Óá
- ²»ÔÙÔÊÐíʹÓÃuint(-1),¸ÄÓÃtype(uint).max
|