1.导入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.0.5</version>
</dependency>
2.配置文件
spring:
application:
name: springboot-rabbitmq
rabbitmq:
host: 192.168.112.130
port: 5672
username: ems
password: 123
virtual-host: /ems
datasource:
url: jdbc:mysql://localhost:3306/1808a2?serverTimezone=GMT%2B8&characterEncoding=utf-8
username:
password:
driver-class-name: com.mysql.cj.jdbc.Driver
mybatis:
mapper-locations: com/baidu/dao/*.xml
type-aliases-package: com.baidu.dto
logging:
level:
com.baidu.dao : debug
3.数据库脚本
DROP TABLE IF EXISTS `tb_user`;
CREATE TABLE `tb_user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) DEFAULT NULL,
`pwd` varchar(255) DEFAULT NULL,
`phone` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8mb4;
INSERT INTO `tb_user` VALUES ('1', 'admin', 'admin', '18434290135');
4.启动rabbitmq
docker start MQid
浏览器访问 http://192.168.112.130:15672/ 使用默认来宾账户登录 guest
5.设置一个虚拟消息服务器
规则是/开头
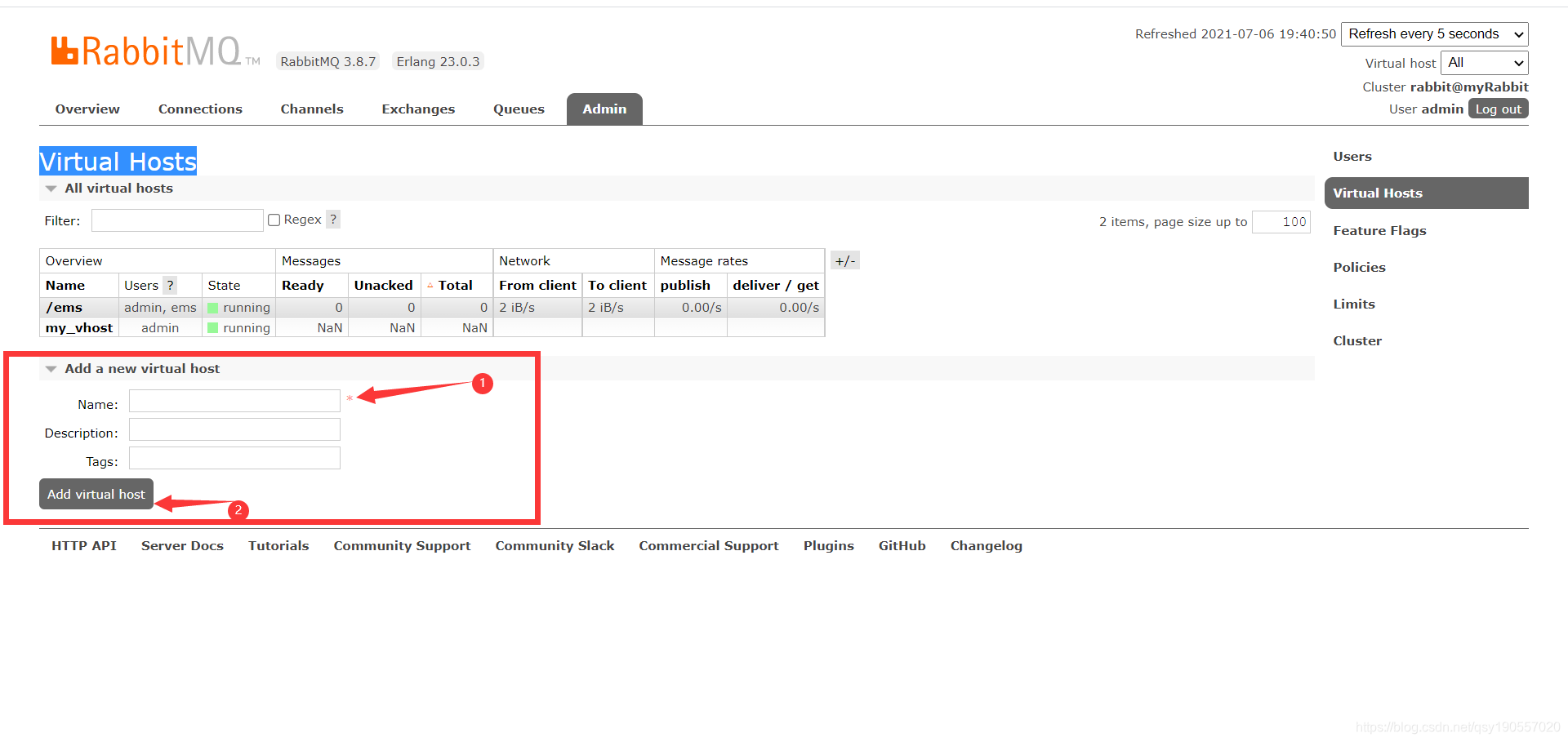
6.创建一个用户
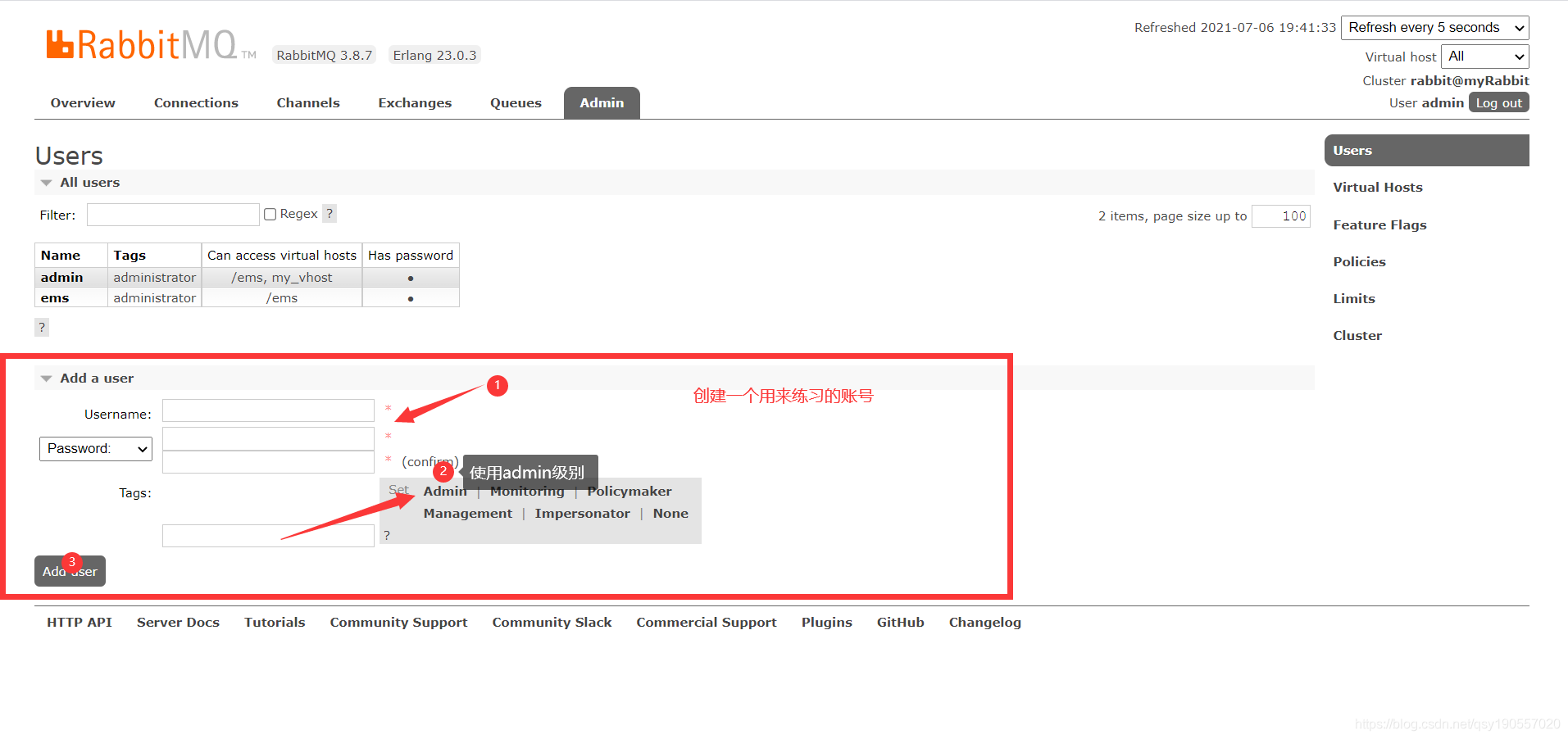
7.使用虚拟消息服务器
admin->Virtual Hosts->/ems
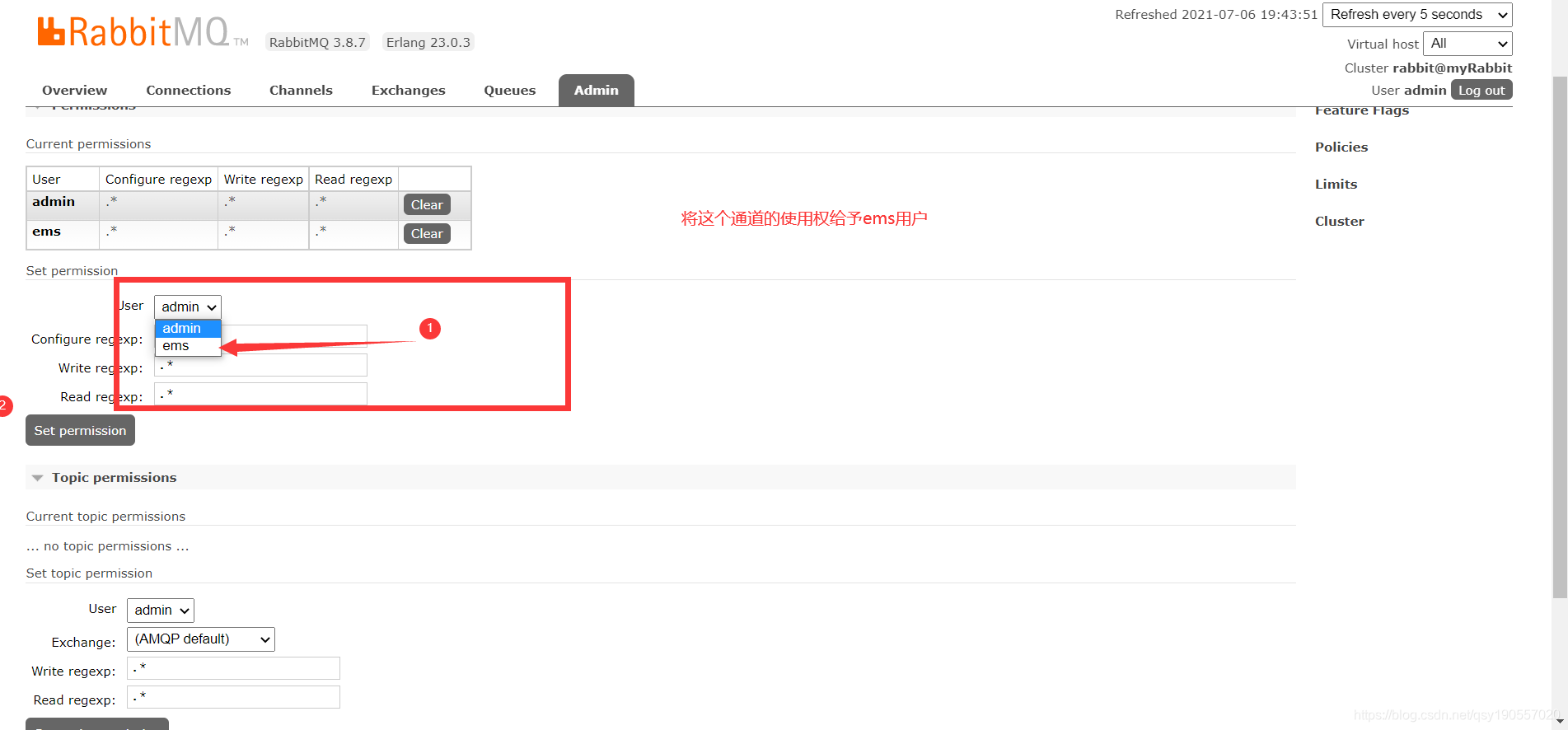
8.实现中间件发送短信
生产者代码
@Autowired
private RabbitTemplate rabbitTemplate;
@Override
public ResultResponse SendCode(String phone) {
QueryWrapper<TBUser> wrapper = new QueryWrapper<TBUser>();
wrapper.lambda().eq(TBUser::getPhone,phone);
TBUser tbUser = tbUserMapper.selectOne(wrapper);
if (tbUser!=null){
rabbitTemplate.convertAndSend("hello",phone);
return ResultResponse.SUCCESS();
}else{
return ResultResponse.FAILED("该手机号不存在");
}
}
消费者代码
package com.baidu.hello;
import com.baidu.utils.AuthCodeUtil;
import org.springframework.amqp.rabbit.annotation.Queue;
import org.springframework.amqp.rabbit.annotation.RabbitHandler;
import org.springframework.amqp.rabbit.annotation.RabbitListener;
import org.springframework.stereotype.Component;
@Component
@RabbitListener(queuesToDeclare = @Queue("hello"))
public class HelloSendCode {
@RabbitHandler
public void sendCode(String phone){
String code = AuthCodeUtil.getCode("0-9", 6);
System.err.println("手机号 ="+phone+"的验证码为"+code);
}
}
自动创建的Hello队列,
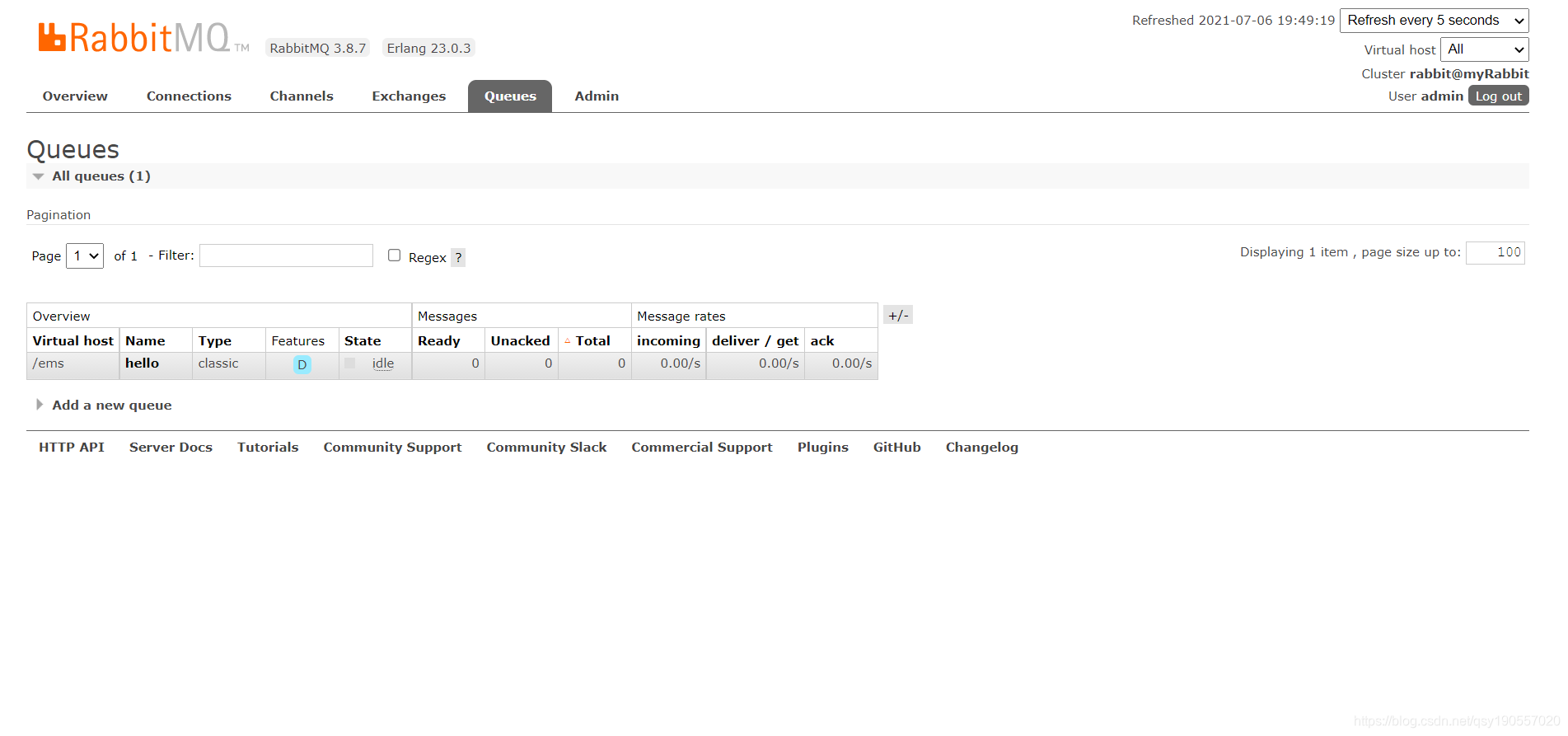
使用postman测试
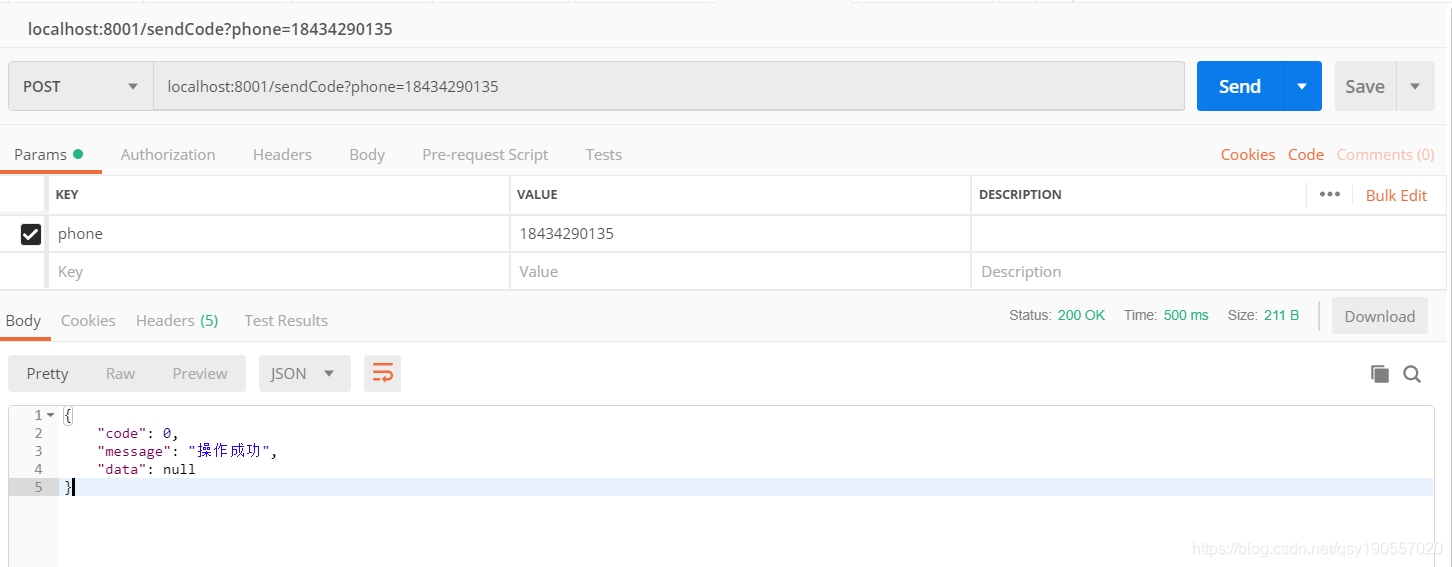
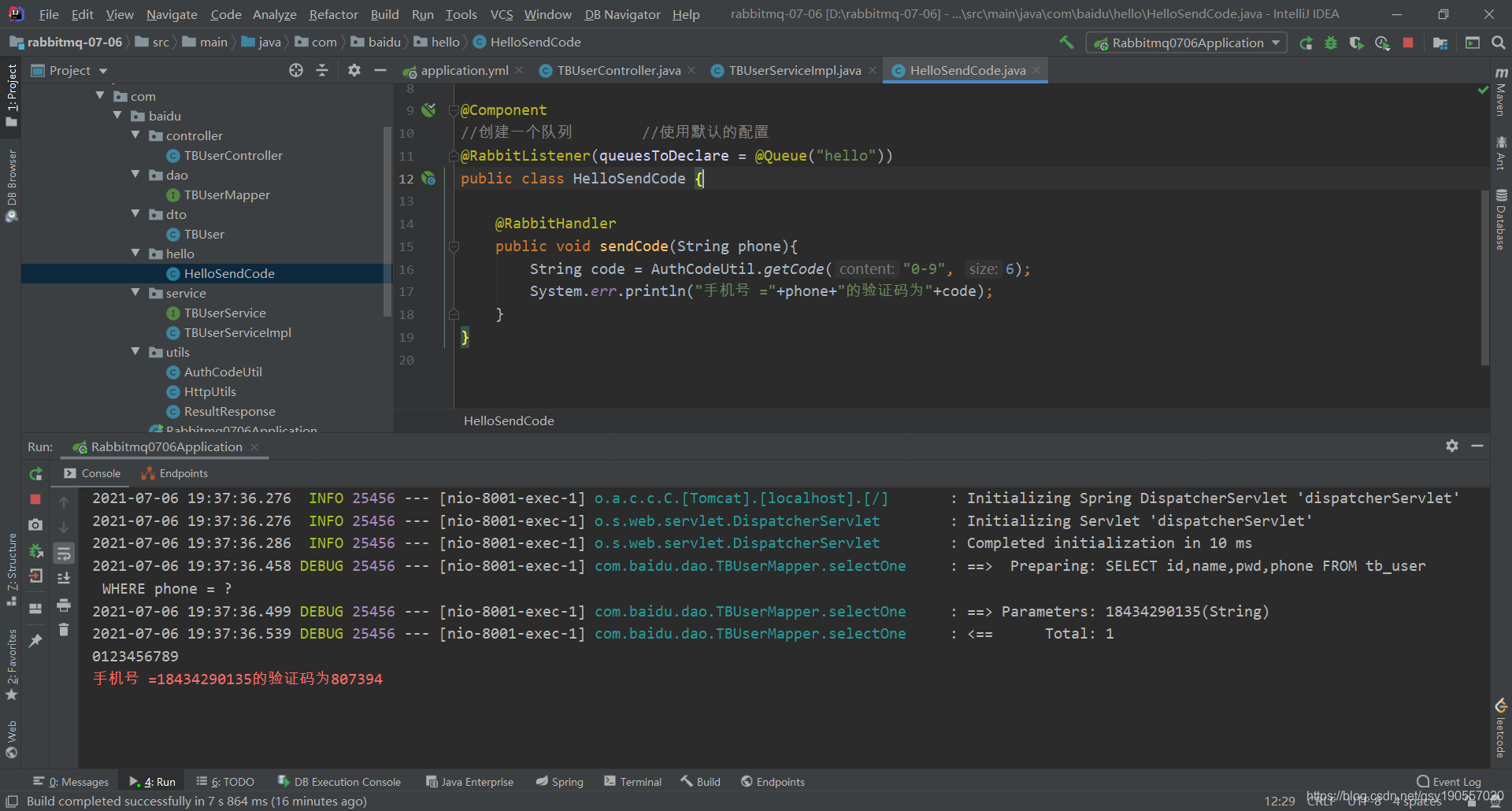
天天打码,天天进步!
|