工作中用的RabbitMQ ,但并没有深入探究它里面的所有消息队列类型,今天有时间来整体的尝试一番!
第一种消息模型: 一个生产者、一个消费者,不使用交换机! 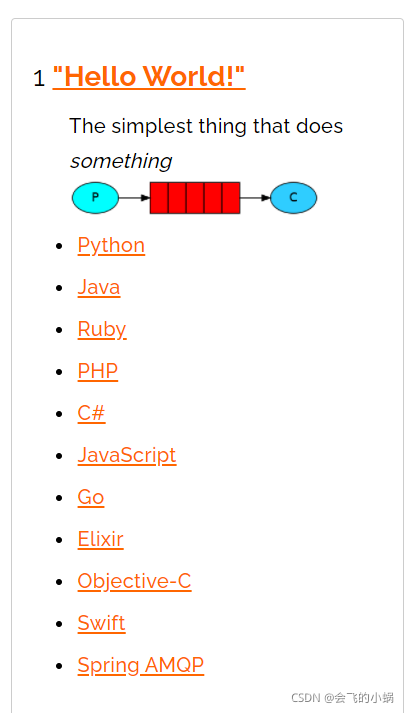
首先需要引入包:
<!--rabbitmq 依赖客户端-->
<dependency>
<groupId>com.rabbitmq</groupId>
<artifactId>amqp-client</artifactId>
<version>5.8.0</version>
</dependency>
创建工具类:
package Utils;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import com.rabbitmq.client.ConnectionFactory;
import java.io.IOException;
import java.util.concurrent.TimeoutException;
public class RabbitMqUtil {
private static ConnectionFactory connectionFactory;
static{
connectionFactory=new ConnectionFactory();
connectionFactory.setHost("192.168.56.10");
connectionFactory.setPort(5672);
connectionFactory.setVirtualHost("/ems");
connectionFactory.setUsername("ems");
connectionFactory.setPassword("123");
}
public static Connection getConnection() throws IOException, TimeoutException {
return connectionFactory.newConnection();
}
public static void closeConnectionAndChanel(Channel channel,Connection connection) throws IOException, TimeoutException {
if(channel==null){
channel.close();
connection.close();
}
}
}
生产者:
package rabbitMq;
import Utils.RabbitMqUtil;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import java.io.IOException;
import java.util.concurrent.TimeoutException;
public class TestRabbitMqP {
public static void main(String[] args) throws IOException, TimeoutException {
String mes="HELLO WORD !!";
Connection connection = RabbitMqUtil.getConnection();
Channel channel = connection.createChannel();
channel.queueDeclare("hello",false,false,false,null);
channel.basicPublish("","hello",null,mes.getBytes());
RabbitMqUtil.closeConnectionAndChanel(channel,connection);
}
}
消费者:
package rabbitMq;
import Utils.RabbitMqUtil;
import com.rabbitmq.client.*;
import com.sun.scenario.effect.impl.sw.sse.SSEBlend_SRC_OUTPeer;
import java.io.IOException;
import java.util.concurrent.TimeoutException;
public class TestRabbitMqC {
public static void main(String[] args) throws IOException, TimeoutException {
String mes="HELLO WORD !!";
Connection connection = RabbitMqUtil.getConnection();
Channel channel = connection.createChannel();
channel.queueDeclare("hello",false,false,false,null);
channel.basicConsume("hello",true,new DefaultConsumer(channel){
@Override
public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException {
System.out.println("==========================="+new String(body));
}
});
}
}
|