思维导图:
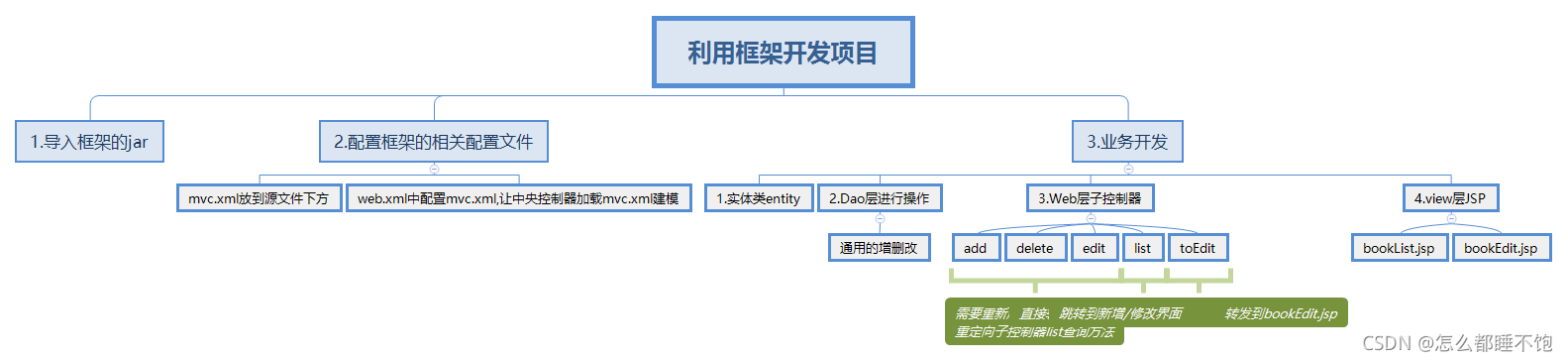
?展示界面: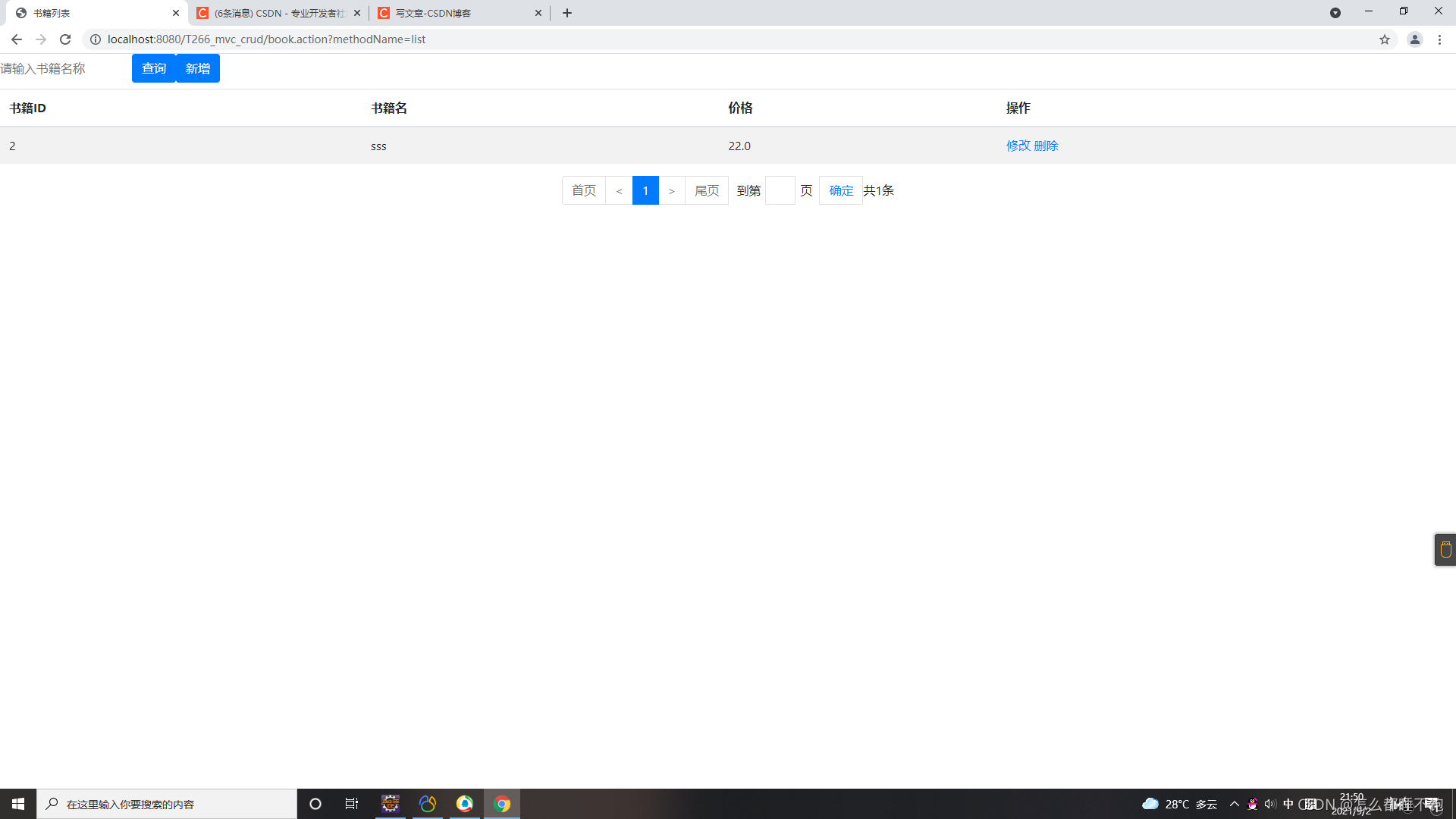
?BookDao:
package com.lx.dao;
import java.util.List;
import com.lx.entity.Book;
import com.lx.util.BaseDao;
import com.lx.util.PageBean;
import com.lx.util.StringUtils;
public class BookDao extends BaseDao<Book> {
public void add(Book book) throws Exception {
String sql = "insert into t_book value(?,?,?)";
super.executeUpdate(sql, book, new String[] { "bid", "bname", "price" });
}
public void delete(Book book) throws Exception {
String sql = "delete from t_book where bid=?";
super.executeUpdate(sql, book, new String[] { "bid" });
}
public void edit(Book book) throws Exception {
String sql = "update t_book set bname = ?,price = ? where bid = ?";
super.executeUpdate(sql, book, new String[] { "bname", "price", "bid" });
}
public List<Book> list(Book book, PageBean pageBean) throws Exception {
String sql = "select * from t_book where 1=1 ";
String bname = book.getBname();
int bid = book.getBid();
if (StringUtils.isNotBlank(bname)) {
sql += " and bname like '%" + bname + "%'";
}
if (bid != 0) {
sql += " and bid = " + bid;
}
return super.executeQuery(sql, Book.class, pageBean);
}
}
book类:
package com.lx.entity;
import java.io.Serializable;
public class Book implements Serializable {
private int bid;
private String bname;
private float price;
public int getBid() {
return bid;
}
public void setBid(int bid) {
this.bid = bid;
}
public String getBname() {
return bname;
}
public void setBname(String bname) {
this.bname = bname;
}
public float getPrice() {
return price;
}
public void setPrice(float price) {
this.price = price;
}
@Override
public String toString() {
return "Book [bid=" + bid + ", bname=" + bname + ", price=" + price + "]";
}
}
BaseDao增删改方法:
public void executeUpdate(String sql, T t, String[] attrs) throws Exception {
// attrs = new String[] {"bid","bname","price"};
// ?->bid;?->bname;?->price
Connection con = DBAccess.getConnection();
PreparedStatement pst = con.prepareStatement(sql);
// pst.setObject(1, book.getBid());
// pst.setObject(2, book.getBname());
// pst.setObject(3, book.getPrice());
for (int i = 0; i < attrs.length; i++) {
// attrs[0]=bid;
Field f = t.getClass().getDeclaredField(attrs[i]);
f.setAccessible(true);
// f.get(t); bid.get(book)
pst.setObject(i+1, f.get(t));
}
pst.executeUpdate();
}
子控制器:
package com.lx.web;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.lx.dao.BookDao;
import com.lx.entity.Book;
import com.lx.util.PageBean;
import com.tz.framework.ActionSupport;
import com.tz.framework.ModelDriver;
public class BookAction extends ActionSupport implements ModelDriver<Book>{
private Book book = new Book();
private BookDao bookDao = new BookDao();
@Override
public Book getModel() {
return book;
}
public String add(HttpServletRequest req, HttpServletResponse resp) {
try {
bookDao.add(book);
} catch (Exception e) {
e.printStackTrace();
}
return "toList";
}
public String delete(HttpServletRequest req, HttpServletResponse resp) {
try {
bookDao.delete(book);
} catch (Exception e) {
e.printStackTrace();
}
return "toList";
}
public String edit(HttpServletRequest req, HttpServletResponse resp) {
try {
bookDao.edit(book);
} catch (Exception e) {
e.printStackTrace();
}
return "toList";
}
public String list(HttpServletRequest req, HttpServletResponse resp) {
PageBean pageBean = new PageBean();
pageBean.setRequest(req);
try {
List<Book> list = bookDao.list(book, pageBean);
req.setAttribute("books", list);
req.setAttribute("pageBean", pageBean);
} catch (Exception e) {
e.printStackTrace();
}
return "list";
}
public String toEdit(HttpServletRequest req, HttpServletResponse resp) {
// 如果跳转的是新增界面无需查询,如果跳转的是修改界面,需要查询当前bid对应的数据,回显到界面
if(book.getBid() != 0) {
try {
// bid=16
List<Book> list = bookDao.list(book, null);
req.setAttribute("b", list.get(0));
} catch (Exception e) {
e.printStackTrace();
}
}
return "toEdit";
}
}
|