MyBatis依赖的JAR包
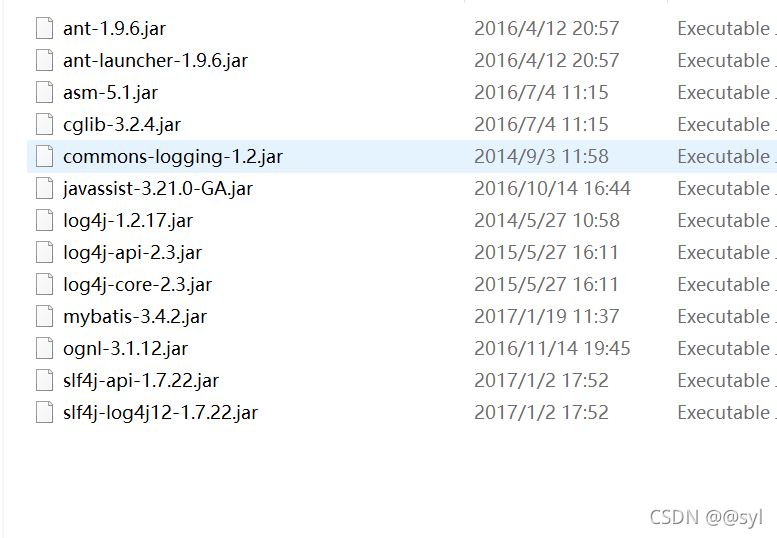
MyBatis默认使用log4j输出日志信息的,如果需要在控制台查看输出的SQL语句,需要在src目录下创建log4j.properties文件,将指定包下的所有类的日志级别设置为DEBUG。
# Global logging configuration
log4j.rootLogger=ERROR, stdout
# MyBatis logging configuration...
log4j.logger.com.syl=DEBUG
# Console output...
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%5p [%t] - %m%n
MyBatis的核心对象
MyBatis框架主要有两个核心对象:SqlSessionFactory和SqlSession。
SqlSessionFactory
org.apache.ibatis.session.SqlSessionFactory SqlSessionFactory主要作用就是用来创建SqlSession对象。通过如下方法创建SqlSessionFactory对象:
InputStream inputStream = Resources.getResourceAsStream("配置文件名");
SqlSessionFactory sqlSessionFactory =
new SqlSessionFactoryBuilder.build(inputStream);
SqlSession
org.apache.ibatis.session.SqlSession SqlSession是应用程序与持久层之间执行交互操作的一个单线程对象,简单点就是使用JDBC执行数据库操作的对象。通过创建好的SqlSessionFactory对象创建SqlSession对象。
SqlSession sqlSession = sqlSessionFactory.openSession();
MyBatis的配置文件
MyBatis的配置文件,包含了很多影响MyBatis行为的信息。通常设置为mybatis-config.xml文件。
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<properties resource="db.properties" />
<typeAliases>
<package name="com.syl.po" />
</typeAliases>
<environments default="mysql">
<environment id="mysql">
<transactionManager type="JDBC" />
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mybatis" />
<property name="username" value="root" />
<property name="password" value="root" />
</dataSource>
</environment>
</environments>
<Mappers>
<mapper package="com.syl.mapper.Customer.xml" />
</Mappers>
MyBatis的映射文件
MyBatis的映射文件,用于执行SQL语句,及其PO类属性名对数据库表字段的映射。通常设置为PO类名+Mapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.syl.mapper.CustomerMapper">
<resultMap type="com.syl.po.Customer" id="resultMap">
<id property="id" column="t_id"/>
<result property="name" column="t_name" />
<result property="age" column="t_age" />
</resultMap>
<select id="findCustomerById"
parameterType="Integer"
resultType="resultMap">
select * from t_customer where id=#{id}
</select>
<update.......>
</update>
<insert.......>
</insert>
<delete.......>
</delete>
</mapper>
MyBatis的动态SQL
动态SQL语句通常应用在映射文件中的SQL语句中,完成一些特殊的功能
< if>元素
判断语句
<select id="findCustomerByNameAndJobs"
parameterType="com.syl.po.Customer"
resultType="com.syl.po.Customer">
select * from t_customer where 1=1
<if test="username != null and username=''">
and username like cocat('%',#{username},'%')
</if>
<if test="jobs != null and jobs=''">
and jobs = #{jobs}
</if>
</select>
< choose>元素
判断语句
<select id="findCustomerByNameAndJobs"
parameterType="com.syl.po.Customer"
resultType="com.syl.po.Customer">
select * from t_customer where 1=1
<choose>
<when test="username != null nad username != ''">
and username like cocat('%',#{username},'%')
</when>
<when test="jobs != null nad jobs != ''">
and jobs=#{jobs}
</when>
<otherwise>
and phone is not null
</otherwiese>
</choose>
</select>
< where>、< trim>元素
SQL中的where子句,使用此语句,就不需要在SQL语句中使用条件where子句。
<select id="findCustomerByNameAndJobs"
parameterType="com.syl.po.Customer"
resultType="com.syl.po.Customer">
select * from t_customer
<where>
<if test="username != null and username=''">
and username like cocat('%',#{username},'%')
</if>
<if test="jobs != null and jobs=''">
and jobs = #{jobs}
</if>
</where>
</select>
< set>元素
update专属语句,MyBatis与Hibernate的不同之处,Hibernate使用update更新字段时,是需要发送全部字段的。MyBatis使用< set>元素,就可以指定更新某些字段。
<update id="updateCustomer"
parameterType="com.syl.po.Customer">
update t_customer
<set>
<if test="username != null and username=''">
username=#{username},
</if>
<if test="jobs != null and jobs=''">
jobs=#{jobs},
</if>
<if test="phone != null and phone=''">
phone=#{phone},
</if>
</set>
</update>
< foreach>元素
循环语句
<select id="findCustomerByIds
parameterType="List"
resultType="com.syl.po.Customer">
select * from t_customer where id in
<foreach item="id" index="index" collection="list" open="(" separator="," close=")" >
#{id}
</foreach>
</select>
@syl 2021/09/03 周五 22:30 晴 回宿舍吧,明天周末
|