存储过程
存储过程与函数相似,定义一些sql语句的集合,可以避免重复编写相同的sql语句,直接调用写好的存储过程来执行这些sql,同时存储过程是在MySQL服务器中存储和执行的,可以减少客户端与服务器端的数据传输
创建存储过程
格式:
create procedure proc_name(proc_param)
begin
routine_body
end;
其中, proc_name:存储过程名字;
proc_param:参数(由三部分组成),可以有多个参数, 格式(IN|OUT|INOUT param_name type), 参数一:IN为输入类型,OUT为输出类型,INOUT为输入/输出类型,三个选择其中之一; 参数二:param_name为参数名字; 参数三:type为参数类型;
routine_body:表示存储过程中的sql语句
【例】使用存储过程的方式,当学生表中删除一条学生记录的时候,成绩表中删除该学生的成绩,同时更新班级表中的人数
create procedure proc_delete_name(IN sid int)
begin
declare cid int;
select class_id into cid from student whrer id=sid;
delete from student where id=sid;
delete from grade where student_id=sid;
update class set student_count=student_count-1 where id=cid;
end;
其中declare表示创建变量(declare name type ),变量赋值有两种方式:
- 使用set方式:
set name=expr; - 使用查询语句赋值:
select col_name into name from table_name where condition;
光标
当存储过程中查询语句查出多条结果,可以使用光标(游标)来逐条读取查询结果中的记录
1 声明光标 格式:
declare cursor_name CURSOR
for select_statement;
其中,cursor_name为光标的名字,select_statement为查询语句
2 打开光标 MySQL中是使用open来打开光标,格式:
open cursor_name;
3 使用光标 MySQL中使用fetch来使用光标,格式:
fetch cursor_name into var_name;
其中,var_name为参数名,将光标中查询出来的结果存入到参数中,参数可以有多个。
【例】查询学生数据,输出出来
create procedure proc_query_student(IN sid int,OUT sname varchar(5),OUT cid int)
begin
declare tmp_name varchar(5);
declare tmp_cid int;
declare done int default 0;
declare cur_student CURSOR for select name,class_id from student where id=sid;
declare continue handler for not found set done=1;
open cur_student;
select done;
FETCH cur_student into tmp_name,tmp_cid;
select done;
close cur_student;
set sname=tmp_name,cid=tmp_cid;
end;
4 关闭光标 使用close进行关闭游标,格式:
close cur_name;
流程控制
存储过程中也可以使用控制语句,包括IF语句、CASE语句、LOOP语句、LEAVE语句、ITERATE语句、REPEAT语句、WHILE语句
1 IF语句 【例】输入年龄,如果大于20,输出年龄+1,等于20输出年龄+2,否则输出年龄+3
create procedure proc_test_if(IN age int,OUT output int)
begin
IF age>20 then set output=age+1;
ELSEIF age=20 then set output=age+2;
ELSE set output=age+3;
end if;
end;
2 CASE语句 【例】输入年龄,如果等于20,输出年龄+1,等于30输出年龄+2,等于30输出年龄+3
create procedure proc_test_loop(IN age int,OUT output int)
begin
case age
when 20 then set output=age+1;
when 30 then set output=age+2;
when 40 then set output=age+3;
end;
3 LOOP语句 loop可以实现循环,需要通过leave来跳出循环 【例】实现从1加到100
create procedure proc_test_loop(IN input int,OUT output int)
begin
set @count=0;
add_num:LOOP
set @count=@count+1;
select @count;
IF @count=100 then
LEAVE add_num;
end if;
end LOOP add_num;
end;
4 LEAVE语句 leave主要用于跳出循环,格式:leave label label为循环标志名
5 ITERATE语句 iterate也是主要用于跳出循环,代表跳出本次循环,进入下一次循环 格式:iterate label label为循环标志名
6 REPEAT语句 repeat代表满足某种条件,跳出循环 格式:
begin_label:repeat
statement_list;
until search_condition
end repeat begin_label
其中,begin_label为标志名;statement_list为循环体;search_condition为结束循环的条件
【例】实现从1加到100
create procedure proc_test_loop(IN input int,OUT output int)
begin
set @count=0;
add_num:REPEAT
SET @count=@count+1;
UNTIL @count=100
end REPEAT add_num;
end;
7 WHILE语句 while也是条件控制的循环语句,while是当满足某种条件时执行循环内的语句 格式:
begin_label:WHILE search_condition DO
statement_list;
end WHILE begin_label
其中,begin_label为标志名;search_condition为开始循环的条件; statement_list为循环体;
【例】实现从1加到100
create procedure proc_test_loop(IN input int,OUT output int)
begin
set @count=0;
add_num:WHILE @count<100 DO
SET @count=@count+1;
end WHILE add_num;
end;
查看存储过程
有三种方式查看存储过程
1 方式一 使用 show procedure status like 'pattern'; 其中pattern为匹配的存储过程的名称,可以省略不写 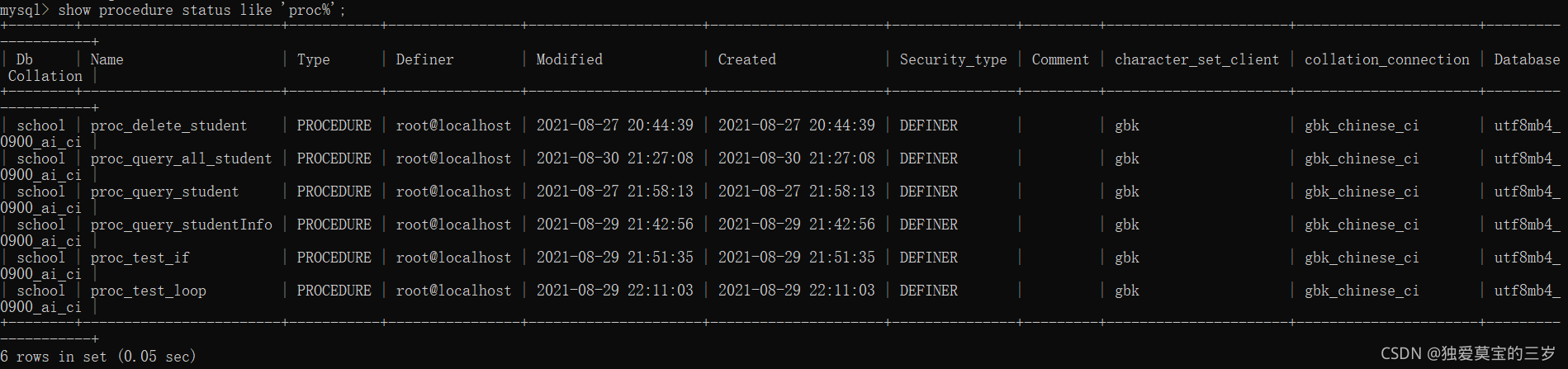 可查询出存储过程名字,创建时间,修改时间等信息
2 方式二 使用show create procedure proc_name; 可查询出存储过程的创建语句 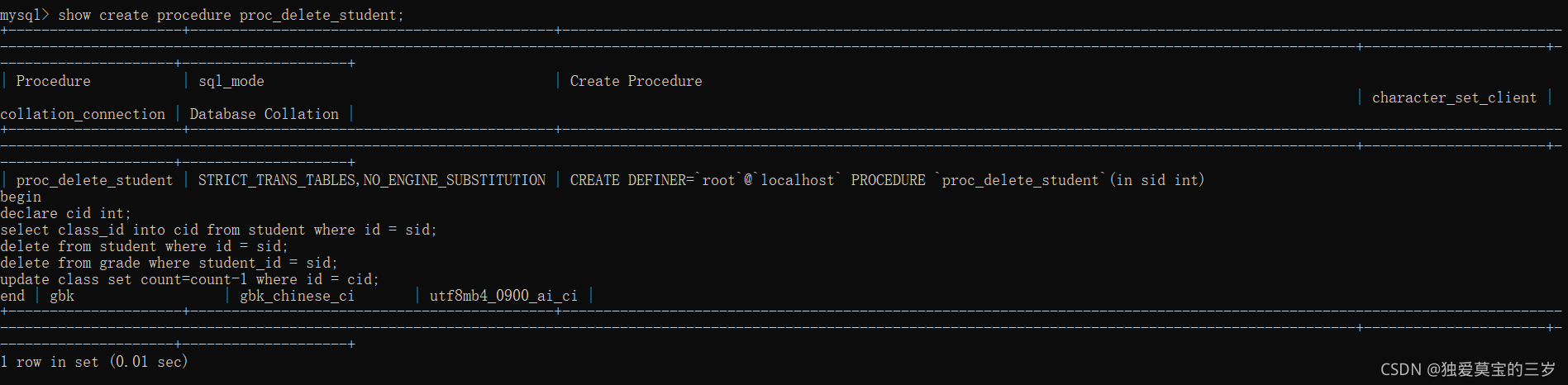 3 方式三 从information_schema.Routines表中查询: SELECT * FROM information_schema.Routines where ROUTINE_NAME='proc_name'; 其中proc_name为存储过程或函数的名字,也可支持模糊查询 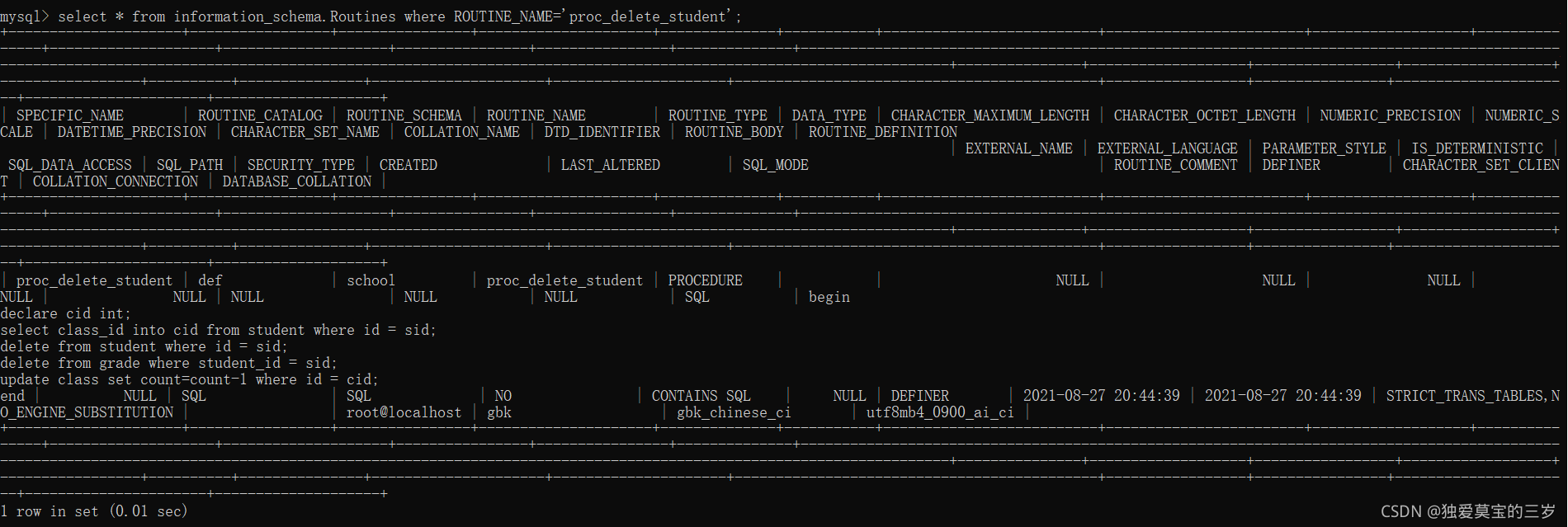
删除存储过程
格式:drop procedure proc_name;
|