java图书管理程序 包含 1、GUI图形化界面 2、数据库增删改查
程序界面如下: 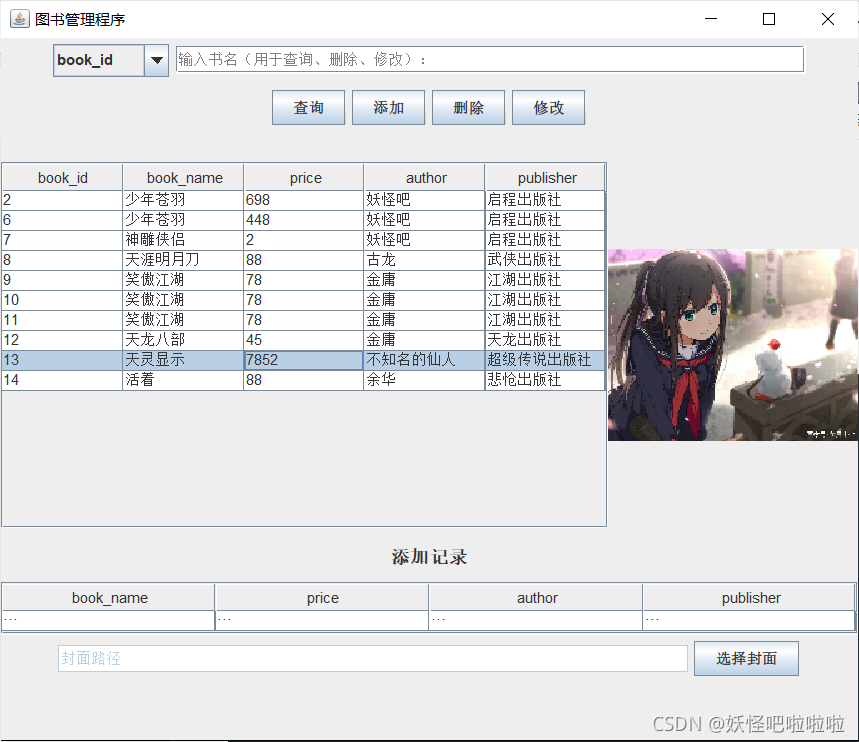
import javax.swing.*;
import javax.swing.filechooser.*;
import java.awt.*;
import java.awt.event.*;
import java.sql.*;
import java.util.*;
import java.io.*;
public class LibraryTest implements ActionListener
{
private String searchAllsql = "select * from books";
private String searchonesql_book_id = "select * from books where book_id = ?";
private String searchonesql_book_name = "select * from books where book_name = ?";
private String searchonesql_price = "select * from books where price = ?";
private String searchonesql_author = "select * from books where author = ?";
private String searchonesql_publisher = "select * from books where publisher = ?";
private String deletesql = "delete from books where book_name = ?";
private String addSql = "insert into books values (null,?,?,?,?,?)";
private String changeSql = "update books set book_name = ?, price = ?,author = ?, publisher = ? where book_id = ?";
private String url, account, password;
private Connection conn;
private Statement stmt;
public LibraryTest()
{
try{
Properties prop = new Properties();
prop.load(new FileInputStream("E:/test/mysql.ini"));
url = prop.getProperty("url");
account = prop.getProperty("account");
password = prop.getProperty("password");
conn = DriverManager.getConnection(url, account, password);
stmt = conn.createStatement();
}
catch(Exception e)
{
e.printStackTrace();
}
}
private JFrame mainWin = new JFrame("图书管理程序");
private JScrollPane scrollPane;
private JTable table;
private MyMouseListener myMouseListener = new MyMouseListener();
private JTable inputTable ;
private JLabel showCover = new JLabel();
private JTextField bookTitle;
String hint = "输入书名(用于查询、删除、修改):";
JTextField coverPath;
JComboBox<String> searchCondition;
String condition;
public void initWindow() throws Exception
{
JButton search = new JButton("查询");
JButton additem = new JButton("添加");
JButton deleteitem = new JButton("删除");
JButton changeitem = new JButton("修改");
JPanel leftControl = new JPanel(new FlowLayout());
leftControl.add(search);
leftControl.add(additem);
leftControl.add(deleteitem);
leftControl.add(changeitem);
search.addActionListener(this);
additem.addActionListener(this);
deleteitem.addActionListener(this);
changeitem.addActionListener(this);
JPanel searchBook = new JPanel();
ResultSet rs = stmt.executeQuery(searchAllsql);
ResultSetMetaData rsmd= rs.getMetaData();
int columnCount = rsmd.getColumnCount()-1;
Vector<String> conditions = new Vector<>();
for( int i =0 ; i < columnCount ; i++)
{
conditions.add(rsmd.getColumnName(i+1));
if( i == 0)
condition = rsmd.getColumnName(i+1);
}
searchCondition = new JComboBox(conditions);
searchCondition.addItemListener(event ->
{
condition = searchCondition.getSelectedItem().toString();
mainWin.validate();
});
JLabel searchLabel = new JLabel(hint);
bookTitle = new JTextField(50);
bookTitle.addFocusListener(new TextFocusListener(bookTitle,hint));
searchBook.add(searchCondition, BorderLayout.WEST);
searchBook.add(bookTitle, BorderLayout.CENTER);
searchBook.add(leftControl,BorderLayout.SOUTH);
searchBook.setPreferredSize(new Dimension(50,100));
mainWin.add(searchBook,BorderLayout.NORTH);
updateTableAll();
table.addMouseListener( myMouseListener);
Vector<String> columnNames = new Vector<>();
Vector<Vector<String>> data = new Vector<>();
Vector<String> onerow = new Vector<>();
for( int i= 1; i < columnCount;i++)
{
columnNames.add(rsmd.getColumnName(i+1));
onerow.add("···");
}
data.add(onerow);
inputTable = new JTable(data,columnNames);
JScrollPane inputScroll = new JScrollPane(inputTable);
inputScroll.setPreferredSize(new Dimension(500,40));
JPanel jp = new JPanel(new GridLayout(4,1));
jp.setPreferredSize(new Dimension(700,170));
JLabel inputLabel = new JLabel("添加记录",JLabel.CENTER);
inputLabel.setFont(new Font("Dialog",1,15));
coverPath =new JTextField(50);
coverPath.setEnabled(false);
coverPath.setText("封面路径");
JButton inputImgBn = new JButton("选择封面");
inputImgBn.addActionListener(this);
JPanel pathPanel = new JPanel();
pathPanel.add(coverPath);
pathPanel.add(inputImgBn);
showCover.setPreferredSize(new Dimension(200,200));
mainWin.add(showCover,BorderLayout.EAST);
jp.add(inputLabel);
jp.add(inputScroll);
jp.add(pathPanel);
mainWin.add(jp,BorderLayout.SOUTH);
mainWin.setSize(700,600);
mainWin.setLocationRelativeTo(null);
mainWin.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
mainWin.setVisible(true);
}
public static void main(String[] args)
{
try{
new LibraryTest().initWindow();
}
catch(Exception e)
{
e.printStackTrace();
}
}
public void actionPerformed(ActionEvent e)
{
try{
String actionCommand = e.getActionCommand();
String title = bookTitle.getText().trim();
if( actionCommand.equals("查询"))
{
Vector<String> columnNames = new Vector<>();
Vector<Vector<String>> data = new Vector<>();
if(title.equals(hint) || title.equals(""))
{
updateTableAll();
}
else
{
String condition_sql = null;
if(condition.equals("book_id"))
condition_sql = searchonesql_book_id;
else if( condition.equals("book_name"))
condition_sql = searchonesql_book_name;
else if(condition.equals("price"))
condition_sql = searchonesql_price;
else if(condition.equals("author"))
condition_sql = searchonesql_author;
else if(condition.equals("publisher"))
condition_sql = searchonesql_publisher;
PreparedStatement pstmt = conn.prepareStatement(condition_sql);
pstmt.setString(1,title);
ResultSet rs = pstmt.executeQuery();
ResultSetMetaData rsmd = pstmt.getMetaData();
int columnCount = rsmd.getColumnCount()-1;
if( scrollPane != null)
{
mainWin.remove(scrollPane);
}
if(rs.next())
{
for( int i = 0; i < columnCount; i++)
{
columnNames.add(rsmd.getColumnName(i+1));
}
rs.beforeFirst();
while(rs.next())
{
Vector<String> data2 = new Vector<>();
for( int i = 0; i < columnCount; i++)
{
data2.add(rs.getString(i+1));
}
data.add(data2);
}
table = new JTable(data,columnNames);
scrollPane = new JScrollPane(table);
mainWin.add(scrollPane);
mainWin.validate();
}
else
{
JOptionPane.showMessageDialog(mainWin,"没有搜索到记录","错误",JOptionPane.ERROR_MESSAGE);
}
}
}
else if( actionCommand.equals("添加"))
{
int result = JOptionPane.showConfirmDialog(mainWin,"是否添加这条记录?","提示",JOptionPane.OK_CANCEL_OPTION);
if(result == JOptionPane.YES_OPTION)
{
int rowCount = inputTable.getRowCount();
int columnCount = inputTable.getColumnCount();
PreparedStatement pstmt = conn.prepareStatement(addSql);
for( int j = 0; j < columnCount; j++)
{
String value = (inputTable.getValueAt(0,j)+"").trim();
if(j == 1 )
{
try{
int price = Integer.valueOf(value);
pstmt.setInt(j+1,price);
System.out.println((j+1)+""+value);
}
catch(Exception inte)
{
JOptionPane.showMessageDialog(mainWin,"价格必须是数字!","错误",JOptionPane.ERROR_MESSAGE);
return;
}
}
else
{
pstmt.setString(j+1,value);
System.out.println((j+1)+""+value);
}
}
String path = coverPath.getText().trim();
if(path.equals("封面路径"))
{
pstmt.setObject(columnCount+1, null);
System.out.println(columnCount+1+"");
pstmt.executeUpdate();
}
else
{
File file = new File(path);
if(file.exists())
{
try(
FileInputStream is = new FileInputStream(file))
{
pstmt.setBinaryStream(columnCount+1,is,(int)file.length());
pstmt.executeUpdate();
}
catch(Exception exc)
{
exc.printStackTrace();
}
}
}
if(pstmt.getUpdateCount()>0)
{
JOptionPane.showMessageDialog(mainWin,"添加成功","结果",JOptionPane.INFORMATION_MESSAGE);
updateTableAll();
}
else
{
JOptionPane.showMessageDialog(mainWin,"添加失败","结果",JOptionPane.INFORMATION_MESSAGE);
}
}
}
else if( actionCommand.equals("删除"))
{
int choose = JOptionPane.showConfirmDialog(mainWin,"确定要删除吗?","提示",JOptionPane.OK_CANCEL_OPTION);
if( choose == JOptionPane.YES_OPTION)
{
PreparedStatement pstmt = conn.prepareStatement(deletesql);
pstmt.setString(1,title);
pstmt.execute();
if(pstmt.getUpdateCount()>0)
{
JOptionPane.showMessageDialog(mainWin,"删除成功","结果",JOptionPane.INFORMATION_MESSAGE);
updateTableAll();
}
else
{
JOptionPane.showMessageDialog(mainWin,"删除失败", "结果",JOptionPane.ERROR_MESSAGE);
}
}
}
else if(actionCommand.equals("修改"))
{
if( table != null)
{
int updateCount = 0;
int result = JOptionPane.showConfirmDialog(mainWin,"是否修改?","提示",JOptionPane.OK_CANCEL_OPTION);
if(result == JOptionPane.YES_OPTION)
{
int rowCount = table.getRowCount();
int columnCount = table.getColumnCount();
for( int i = 0; i < rowCount; i++)
{
PreparedStatement pstmt = conn.prepareStatement(changeSql);
for( int j= 1 ; j< columnCount; j++)
{
String value = table.getValueAt(i,j)+"";
if(j==2)
{
try{
int price = Integer.valueOf(value);
pstmt.setInt(j,price);
}
catch(Exception inte)
{
JOptionPane.showMessageDialog(mainWin,"价格必须是数字!","错误",JOptionPane.ERROR_MESSAGE);
}
}
else
{
pstmt.setString(j,value);
}
}
int book_id=Integer.valueOf(table.getValueAt(i,0)+"");
pstmt.setInt(columnCount, book_id);
pstmt.execute();
updateCount += pstmt.getUpdateCount();
}
if( updateCount>0)
{
JOptionPane.showMessageDialog(mainWin,"修改成功","结果",JOptionPane.INFORMATION_MESSAGE);
}
else
{
JOptionPane.showMessageDialog(mainWin,"修改失败","结果",JOptionPane.INFORMATION_MESSAGE);
}
}
}
else
{
JOptionPane.showMessageDialog(mainWin,"未查询,请先查询!","错误",JOptionPane.ERROR_MESSAGE);
}
}
else if( actionCommand.equals("选择封面"))
{
JFileChooser chooser = new JFileChooser();
FileNameExtensionFilter filter = new FileNameExtensionFilter(
"JPG & GIF Image","jpg","gif","png");
chooser.setFileFilter(filter);
int returnVal = chooser.showOpenDialog(mainWin);
if( returnVal == JFileChooser.APPROVE_OPTION)
{
coverPath.setText(chooser.getSelectedFile().getPath());
}
}
}
catch(SQLException sqle)
{
sqle.printStackTrace();
}
}
public void updateTableAll()
{
try{
Vector<String> columnNames = new Vector<>();
Vector<Vector<String>> data = new Vector<>();
if(scrollPane != null)
{
mainWin.remove(scrollPane);
}
ResultSet rs = stmt.executeQuery(searchAllsql);
ResultSetMetaData rsmd = rs.getMetaData();
int columnCount = rsmd.getColumnCount();
for( int i = 0 ; i< columnCount-1 ; i++)
{
columnNames.add(rsmd.getColumnName(i+1));
}
while(rs.next())
{
Vector<String> onerow = new Vector<>();
for(int i = 0; i< columnCount-1; i++)
{
onerow.add(rs.getString(i+1));
}
data.add(onerow);
}
table = new JTable(data, columnNames);
table.addMouseListener(myMouseListener);
scrollPane = new JScrollPane(table);
mainWin.add(scrollPane);
mainWin.validate();
}
catch(Exception e)
{
e.printStackTrace();
}
}
class MyMouseListener extends MouseAdapter
{
public void mouseClicked(MouseEvent e)
{
int clickRow = table.getSelectedRow();
int id =Integer.valueOf( table.getValueAt(clickRow, 0)+"");
try{
PreparedStatement pstmt = conn.prepareStatement(searchonesql_book_id);
pstmt.setInt(1,id);
ResultSet rs = pstmt.executeQuery();
if( rs.next())
{
Blob imgBlob = rs.getBlob(6);
if( imgBlob !=null)
{
var icon = new ImageIcon(imgBlob.getBytes(1L,(int)imgBlob.length()));
double rate =(double) icon.getIconHeight()/(double)icon.getIconWidth();
Image image = icon.getImage().getScaledInstance(
showCover.getWidth(), (int)(showCover.getWidth()*rate),Image.SCALE_DEFAULT);
icon = new ImageIcon(image);
showCover.setIcon(icon);
}
else
{
showCover.setIcon(null);
}
}
}
catch(Exception e2)
{
e2.printStackTrace();
}
}
}
}
class TextFocusListener implements FocusListener
{
private JTextField tf;
private String hint;
public TextFocusListener(JTextField tf,String hint)
{
this.tf = tf;
this.hint = hint;
tf.setText(hint);
tf.setForeground(Color.GRAY);
}
public void focusGained(FocusEvent e)
{
if(tf.getText().trim().equals(hint))
{
tf.setText("");
tf.setForeground(Color.BLACK);
}
}
public void focusLost(FocusEvent e)
{
if( tf.getText().trim().equals(""))
{
tf.setText(hint);
tf.setForeground(Color.GRAY);
}
}
}
|