图书管理系统(JAVA+Mysql)
一.编译环境 1.编译器:eclipse,windowsbuilder插件 2.数据库:mysql5.7
二.部分代码展示 1.代码架构 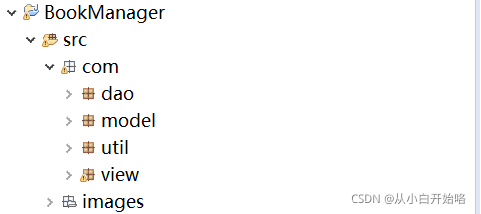 2.实体类
package com.model;
public class Book {
private int id;
private String bookName;
private String author;
private String sex;
private Float price;
private String bookTypeName;
private String bookDesc;
private Integer bookTypeId;
public Book() {
super();
}
public Book(int id, String bookName, String author, String sex, Float price, String bookDesc, Integer bookTypeId) {
super();
this.id = id;
this.bookName = bookName;
this.author = author;
this.sex = sex;
this.price = price;
this.bookDesc = bookDesc;
this.bookTypeId = bookTypeId;
}
public Book(String bookName, String author, Integer bookTypeId) {
super();
this.bookName = bookName;
this.author = author;
this.bookTypeId = bookTypeId;
}
public Book(String bookName, String author, String bookTypeName) {
super();
this.bookName = bookName;
this.author = author;
this.bookTypeName = bookTypeName;
}
public Book(String bookName, String author,String sex, Float price, String bookDesc,Integer bookTypeId) {
super();
this.bookName = bookName;
this.sex = sex;
this.author = author;
this.price = price;
this.bookTypeId = bookTypeId;
this.bookDesc = bookDesc;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getBookName() {
return bookName;
}
public void setBookName(String bookName) {
this.bookName = bookName;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public Float getPrice() {
return price;
}
public void setPrice(Float price) {
this.price = price;
}
public Integer getBookTypeId() {
return bookTypeId;
}
public void setBookTypeId(Integer bookTypeId) {
this.bookTypeId = bookTypeId;
}
public String getBookTypeName() {
return bookTypeName;
}
public void setBookTypeName(String bookTypeName) {
this.bookTypeName = bookTypeName;
}
public String getBookDesc() {
return bookDesc;
}
public void setBookDesc(String bookDesc) {
this.bookDesc = bookDesc;
}
}
3.数据库连接工具类
package com.util;
import java.sql.Connection;
import java.sql.DriverManager;
public class DbUtil {
private String dbUrl = "jdbc:mysql://localhost:3306/db_book?useUnicode=true&characterEncoding=utf-8";
private String dbUserName = "root";
private String dbPassword = "123456";
private String jdbcName = "com.mysql.jdbc.Driver";
public Connection getCon() throws Exception{
Class.forName(jdbcName);
Connection con = DriverManager.getConnection(dbUrl, dbUserName, dbPassword);
return con;
}
public void closeCon(Connection con) throws Exception{
if (con != null){
con.close();
}
}
public static void main(String[] args) {
DbUtil dbUtil = new DbUtil();
try {
dbUtil.getCon();
System.out.println("数据库连接成功");
} catch (Exception e) {
e.printStackTrace();
System.out.println("数据库连接失败");
}
}
}
4.图书数据库操作类
package com.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import com.model.Book;
import com.util.StringUtil;
public class BookDao {
public int add(Connection con ,Book book)throws Exception{
String sql = "insert into t_book values(null,?,?,?,?,?,?)";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, book.getBookName());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getSex());
pstmt.setFloat(4, book.getPrice());
pstmt.setString(5, book.getBookDesc());
pstmt.setInt(6, book.getBookTypeId());
return pstmt.executeUpdate();
}
public ResultSet list(Connection con,Book book) throws Exception{
StringBuffer sb = new StringBuffer("select * from t_book b , t_bookType bt where b.bookTypeId=bt.id");
if(!StringUtil.isEmpty(book.getBookName())){
sb.append(" and b.bookName like '%"+book.getBookName()+"%'");
}
if(!StringUtil.isEmpty(book.getAuthor())){
sb.append(" and b.author like '%"+book.getAuthor()+"%'");
}
if (book.getBookTypeId()!=null&& book.getBookTypeId()!=-1){
sb.append(" and b.bookTypeId="+book.getBookTypeId());
}
PreparedStatement pstmt = con.prepareStatement(sb.toString());
return pstmt.executeQuery();
}
public int delete(Connection con,String id) throws Exception{
String sql = "delete from t_book where id=?";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, id);
return pstmt.executeUpdate();
}
public int update(Connection con,Book book) throws Exception{
String sql = "update t_book set bookName=?,author=?,sex=?,price=?,bookDesc=?,bookTypeId=? where id=?";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, book.getBookName());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getSex());
pstmt.setFloat(4, book.getPrice());
pstmt.setString(5, book.getBookDesc());
pstmt.setInt(6, book.getBookTypeId());
pstmt.setInt(7, book.getId());
return pstmt.executeUpdate();
}
public boolean exitBookByBookTypeId(Connection con,String bookTypeId)throws Exception{
String sql = "select * from t_book where bookTypeId=?";
PreparedStatement pstmt = con.prepareStatement(sql);
pstmt.setString(1, bookTypeId);
ResultSet rs = pstmt.executeQuery();
return rs.next();
}
}
5.图书数据库增删改查
package com.view;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.ResultSet;
import javax.swing.ButtonGroup;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JInternalFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JRadioButton;
import javax.swing.JTextArea;
import javax.swing.JTextPane;
import javax.swing.LayoutStyle.ComponentPlacement;
import com.dao.BookDao;
import com.dao.BookTypeDao;
import com.model.Book;
import com.model.BookType;
import com.util.DbUtil;
import com.util.StringUtil;
public class BookAddInterFrm extends JInternalFrame {
private final ButtonGroup buttonGroup = new ButtonGroup();
private DbUtil dbUtil = new DbUtil();
private BookTypeDao bookTypeDao = new BookTypeDao();
private BookDao bookDao = new BookDao();
JComboBox bookTypeJcb;
JTextPane priseTxt;
JTextPane bookNameTxt;
JTextPane authorTxt;
JTextArea bookDescTxt;
JRadioButton manJrb;
JRadioButton femaleJrb;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
BookAddInterFrm frame = new BookAddInterFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public BookAddInterFrm() {
setIconifiable(true);
setClosable(true);
setTitle("\u56FE\u4E66\u6DFB\u52A0");
setBounds(100, 100, 592, 568);
JLabel label = new JLabel("\u56FE\u4E66\u540D\u79F0");
JLabel lblNewLabel = new JLabel("\u56FE\u4E66\u4F5C\u8005");
JLabel lblNewLabel_1 = new JLabel("\u4F5C\u8005\u6027\u522B");
bookNameTxt = new JTextPane();
authorTxt = new JTextPane();
manJrb = new JRadioButton("\u7537");
buttonGroup.add(manJrb);
manJrb.setSelected(true);
femaleJrb = new JRadioButton("\u5973");
buttonGroup.add(femaleJrb);
JLabel lblNewLabel_2 = new JLabel("\u56FE\u4E66\u4EF7\u683C");
JLabel label_1 = new JLabel("\u56FE\u4E66\u63CF\u8FF0");
bookDescTxt = new JTextArea();
bookTypeJcb = new JComboBox();
JLabel label_2 = new JLabel("\u56FE\u4E66\u7C7B\u522B");
JButton btnNewButton = new JButton("\u91CD\u7F6E");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
resetValue();
}
});
btnNewButton.setIcon(new ImageIcon(BookAddInterFrm.class.getResource("/images/\u91CD\u7F6E.png")));
JButton btnNewButton_1 = new JButton("\u6DFB\u52A0");
btnNewButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
bookAddActionPerformed(e);
}
});
btnNewButton_1.setIcon(new ImageIcon(BookAddInterFrm.class.getResource("/images/\u6DFB\u52A0.png")));
priseTxt = new JTextPane();
GroupLayout groupLayout = new GroupLayout(getContentPane());
groupLayout.setHorizontalGroup(
groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup()
.addGap(60)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup()
.addGroup(groupLayout.createParallelGroup(Alignment.TRAILING)
.addComponent(label_1)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addComponent(label)
.addComponent(lblNewLabel_1))
.addComponent(label_2))
.addGap(18)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addComponent(bookTypeJcb, GroupLayout.PREFERRED_SIZE, 73, GroupLayout.PREFERRED_SIZE)
.addGroup(groupLayout.createParallelGroup(Alignment.TRAILING)
.addComponent(bookDescTxt, Alignment.LEADING)
.addGroup(Alignment.LEADING, groupLayout.createSequentialGroup()
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addComponent(bookNameTxt, GroupLayout.PREFERRED_SIZE, 98, GroupLayout.PREFERRED_SIZE)
.addGroup(groupLayout.createSequentialGroup()
.addComponent(manJrb)
.addGap(18)
.addComponent(femaleJrb)))
.addGap(41)
.addGroup(groupLayout.createParallelGroup(Alignment.TRAILING, false)
.addGroup(Alignment.LEADING, groupLayout.createSequentialGroup()
.addComponent(lblNewLabel_2)
.addGap(18)
.addComponent(priseTxt))
.addGroup(Alignment.LEADING, groupLayout.createSequentialGroup()
.addComponent(lblNewLabel)
.addGap(18)
.addComponent(authorTxt, GroupLayout.PREFERRED_SIZE, 130, GroupLayout.PREFERRED_SIZE))))))
.addContainerGap(32, GroupLayout.PREFERRED_SIZE))
.addGroup(groupLayout.createSequentialGroup()
.addComponent(btnNewButton_1)
.addPreferredGap(ComponentPlacement.RELATED, 174, Short.MAX_VALUE)
.addComponent(btnNewButton)
.addGap(130))))
);
groupLayout.setVerticalGroup(
groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup()
.addGap(39)
.addGroup(groupLayout.createParallelGroup(Alignment.TRAILING)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addComponent(bookNameTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(label))
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addComponent(authorTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(lblNewLabel)))
.addGap(61)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup()
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(manJrb)
.addComponent(lblNewLabel_1)
.addComponent(femaleJrb)
.addComponent(lblNewLabel_2))
.addGap(31)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(bookTypeJcb, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(label_2)))
.addComponent(priseTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addGap(65)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(bookDescTxt, GroupLayout.PREFERRED_SIZE, 127, GroupLayout.PREFERRED_SIZE)
.addComponent(label_1))
.addPreferredGap(ComponentPlacement.RELATED, 40, Short.MAX_VALUE)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE)
.addComponent(btnNewButton_1)
.addComponent(btnNewButton))
.addGap(51))
);
getContentPane().setLayout(groupLayout);
fillBookType();
}
private void bookAddActionPerformed(ActionEvent evt) {
String bookName = this.bookNameTxt.getText();
String bookDesc = this.bookDescTxt.getText();
String author = this.authorTxt.getText();
String prise = this.priseTxt.getText();
if (StringUtil.isEmpty(bookName)){
JOptionPane.showMessageDialog(null,"图书名称不能为空");
return;
}
if (StringUtil.isEmpty(author)){
JOptionPane.showMessageDialog(null,"图书作者不能为空");
return;
}
if (StringUtil.isEmpty(prise)){
JOptionPane.showMessageDialog(null,"图书价格不能为空");
return;
}
String sex = "";
if(manJrb.isSelected()){
sex = "男";
}else{
sex = "女";
}
BookType bookType = (BookType)bookTypeJcb.getSelectedItem();
int bookTypeId = bookType.getId();
Book book = new Book(bookName,author,sex, Float.parseFloat(prise),bookDesc,bookTypeId);
Connection con = null;
try {
con = dbUtil.getCon();
int n =bookDao.add(con, book);
if (n==1){
JOptionPane.showMessageDialog(null,"图书添加成功");
resetValue();
}else{
JOptionPane.showMessageDialog(null,"图书添加失败");
}
} catch (Exception e) {
e.printStackTrace();
} finally{
try {
dbUtil.closeCon(con);
} catch (Exception e) {
e.printStackTrace();
}
}
}
private void fillBookType(){
Connection con = null;
BookType bookType = null;
try {
con = dbUtil.getCon();
ResultSet rs = bookTypeDao.list(con, new BookType());
while(rs.next()){
bookType = new BookType();
bookType.setId(rs.getInt("id"));
bookType.setBookTypeName(rs.getString("bookTypeName"));
this.bookTypeJcb.addItem(bookType);
}
} catch (Exception e) {
} finally{
try {
dbUtil.closeCon(con);
} catch (Exception e) {
e.printStackTrace();
}
}
}
private void resetValue(){
this.bookNameTxt.setText("");
this.bookDescTxt.setText("");
this.authorTxt.setText("");
this.priseTxt.setText("");
this.manJrb.setSelected(true);
if(bookTypeJcb.getItemCount()>0){
this.bookTypeJcb.setSelectedIndex(0);
}
}
}
三.效果展示 1.登录界面 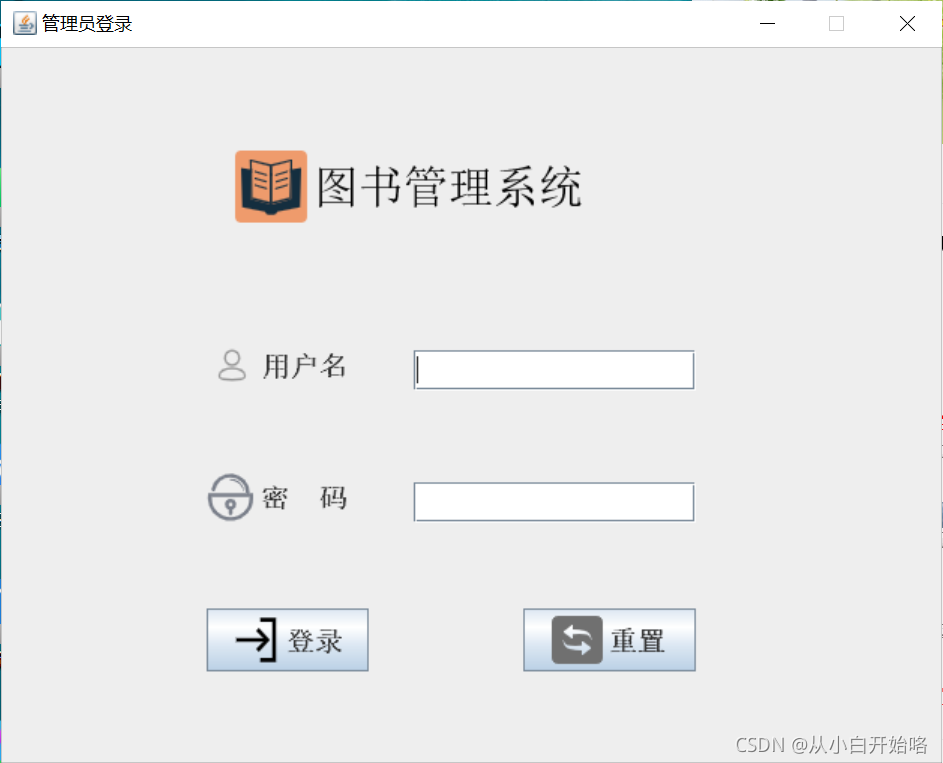 2.图书添加 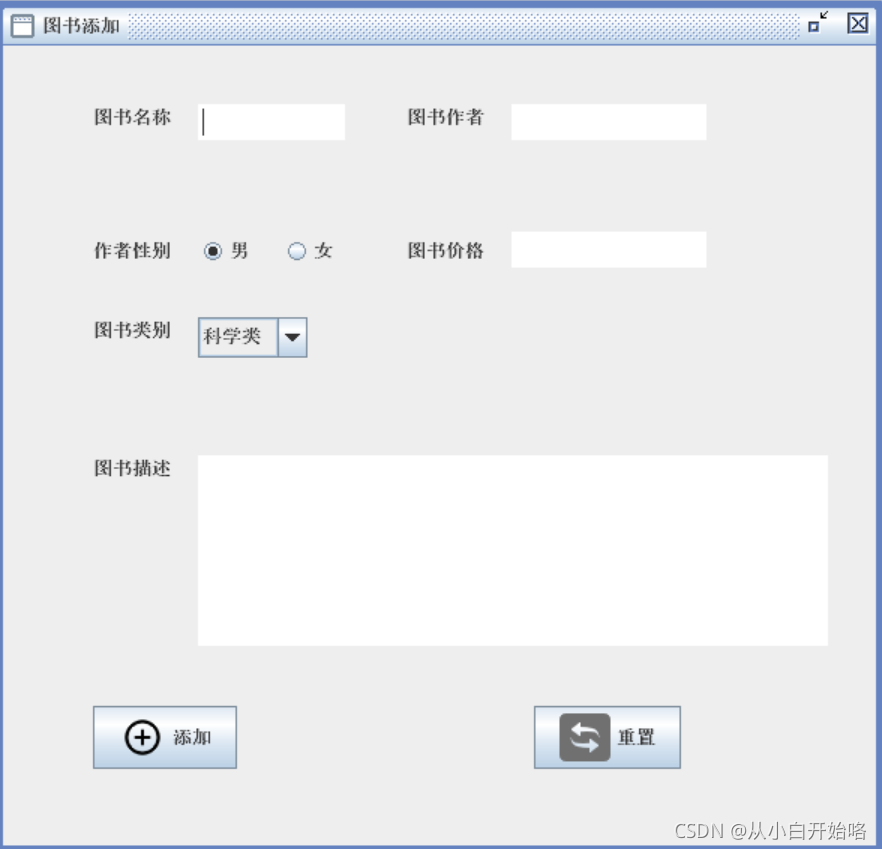 3.图书管理 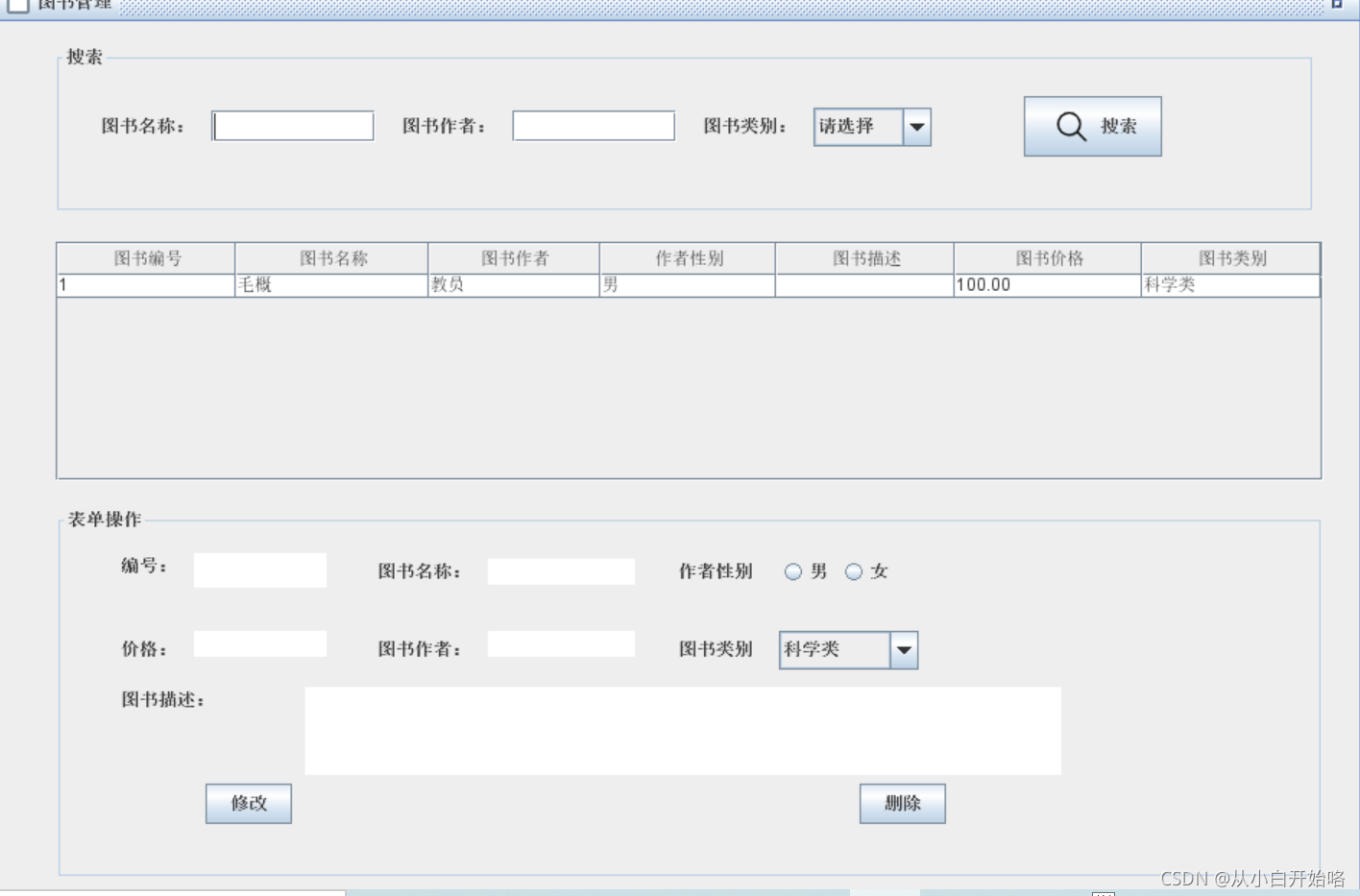 4.图书类别管理 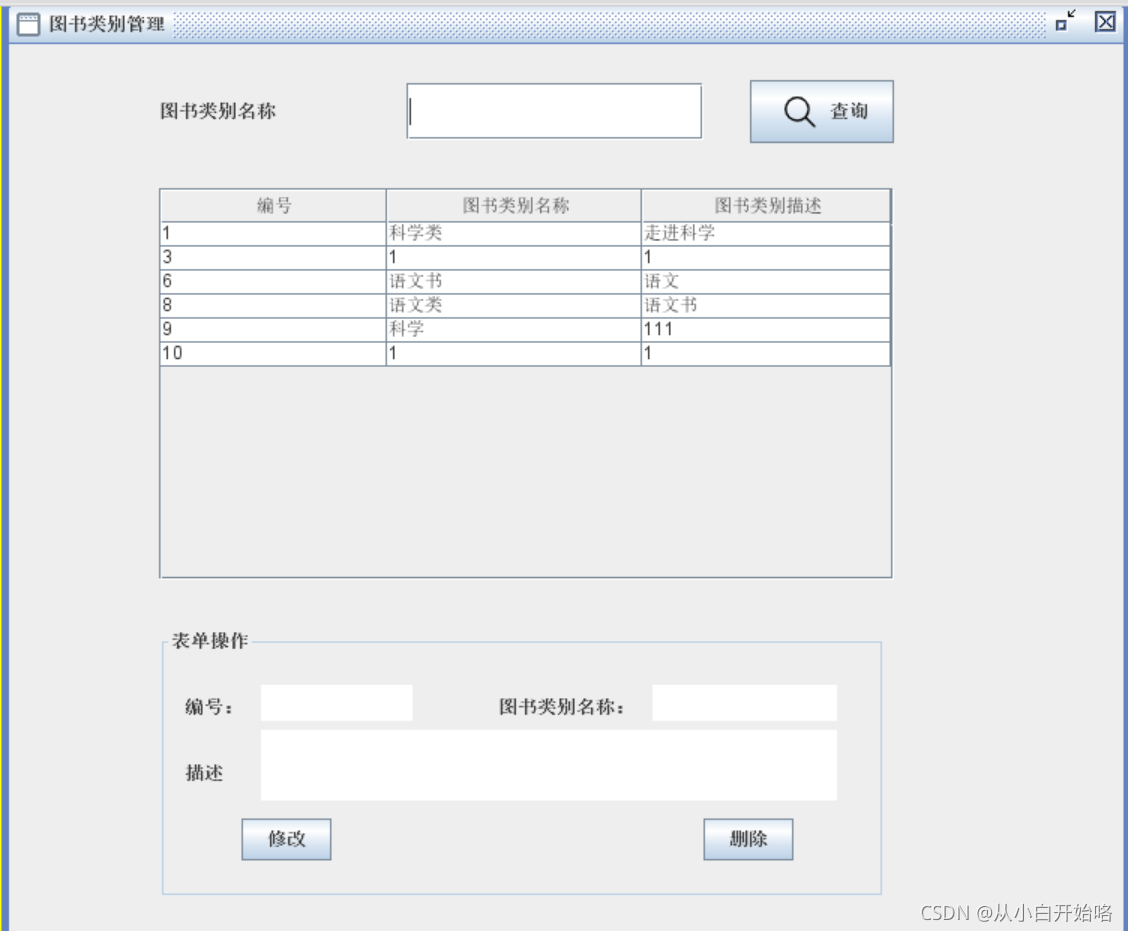 源码QQ群:938064268
|