hibernate.cfg.xml文件内容:
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!-- 连接数据库的基本参数 -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<!-- <property name="hibernate.connection.url">jdbc:mysql:///hibernate</property>-->
<!-- <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernate?user=root&password=1234&serverTimezone=GMT%2B8</property>-->
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernate?useSSL=false&serverTimezone=UTC</property>
<property name="connection.autocommit">true</property>
<property name="hibernate.connection.username">root</property>
<property name="hibernate.connection.password">1234</property>
<!-- 配置Hibernate的方言 -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQL8Dialect</property>
<!-- 打印SQL -->
<property name="hibernate.show_sql">true</property>
<!-- 格式化SQL -->
<property name="hibernate.format_sql">true</property>
<!-- 自动创建表 -->
<property name="hibernate.hbm2ddl.auto">update</property>
<!--C3PO-->
<property name="connection.provider_class">org.hibernate.c3p0.internal.C3P0ConnectionProvider</property>
<!--在连接池中可用的数据库连接的最少数目 -->
<property name="c3p0.min_size">5</property>
<!--在连接池中所有数据库连接的最大数目 -->
<property name="c3p0.max_size">20</property>
<!--设定数据库连接的过期时间,以秒为单位,
如果连接池中的某个数据库连接处于空闲状态的时间超过了timeout时间,就会从连接池中清除 -->
<property name="c3p0.timeout">120</property>
<!--每3000秒检查所有连接池中的空闲连接 以秒为单位-->
<property name="c3p0.idle_test_period">3000</property>
<!--设置事务的隔离级别-->
<property name="hibernate.connection.isolation">4</property>
<!--创建一个session绑定到当前线程-->
<property name="current_session_context_class">thread</property>
<!--加载映射文件-->
<mapping resource="com/myxq/domain/Customer.hbm.xml"/>
</session-factory>
</hibernate-configuration>
Customer.hbm.xml文件内容:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.myxq.domain.Customer" table="customer" >
<!--建立类属性哪一个是主键 还要跟数据库当中主键进行对象-->
<id name="cust_id" column="cust_id" >
<generator class="native"/>
</id>
<!--建立类中的普通属性与数据库当中字段进行关联-->
<property name="cust_name" column="cust_name" />
<property name="cust_source" column="cust_source"/>
<property name="cust_industry" column="cust_industry"/>
<property name="cust_level" column="cust_level"/>
<property name="cust_phone" column="cust_phone"/>
<property name="cust_mobile" column="cust_mobile"/>
</class>
</hibernate-mapping>
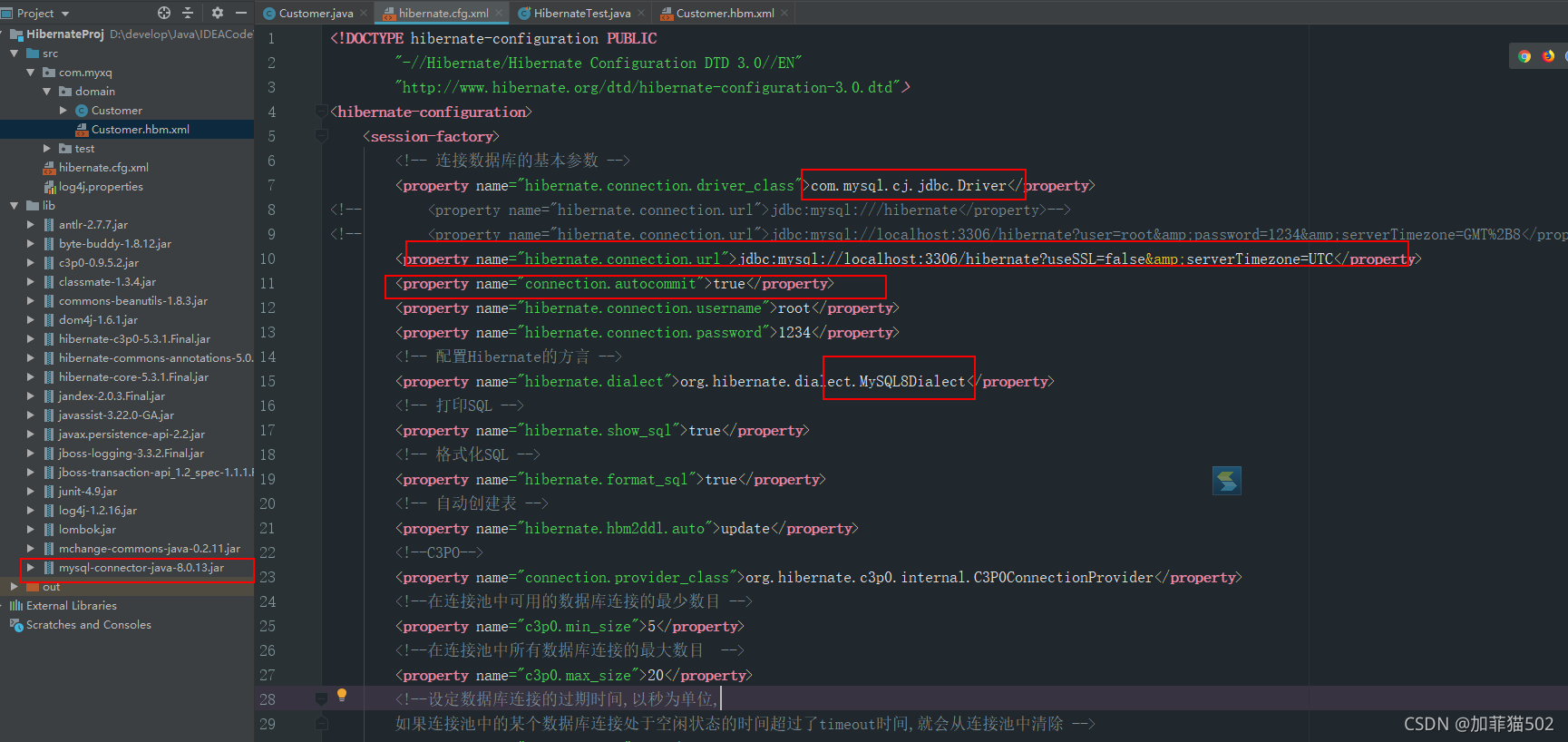
?
test.java:
package com.myxq.test;
import com.myxq.domain.Customer;
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
import org.junit.Test;
public class HibernateTest {
@Test
public void test(){
// 1.加载Hibernate的核心配置文件
Configuration configuration = new Configuration().configure();
// 2.创建一个SessionFactory对象:类似于JDBC中连接池
SessionFactory sessionFactory = configuration.buildSessionFactory();
// 3.通过SessionFactory获取到Session对象:类似于JDBC中Connection
Session session = sessionFactory.openSession();
// 5.编写代码
Customer customer = new Customer();
customer.setCust_name("测试");
customer.setCust_level("123");
session.save(customer);
System.out.println(customer.toString());
// 6.资源释放
session.close();
sessionFactory.close();
}
}
重点部分:
1.配置
<!-- 连接数据库的基本参数 -->
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/hibernate?useSSL=false&serverTimezone=UTC</property>
<property name="connection.autocommit">true</property>
<!-- 配置Hibernate的方言 -->
<property name="hibernate.dialect">org.hibernate.dialect.MySQL8Dialect</property>
2.jar包版本:需要使用版本8以上
mysql-connector-java-8.0.13.jar
|