1.jdbc原理示意图
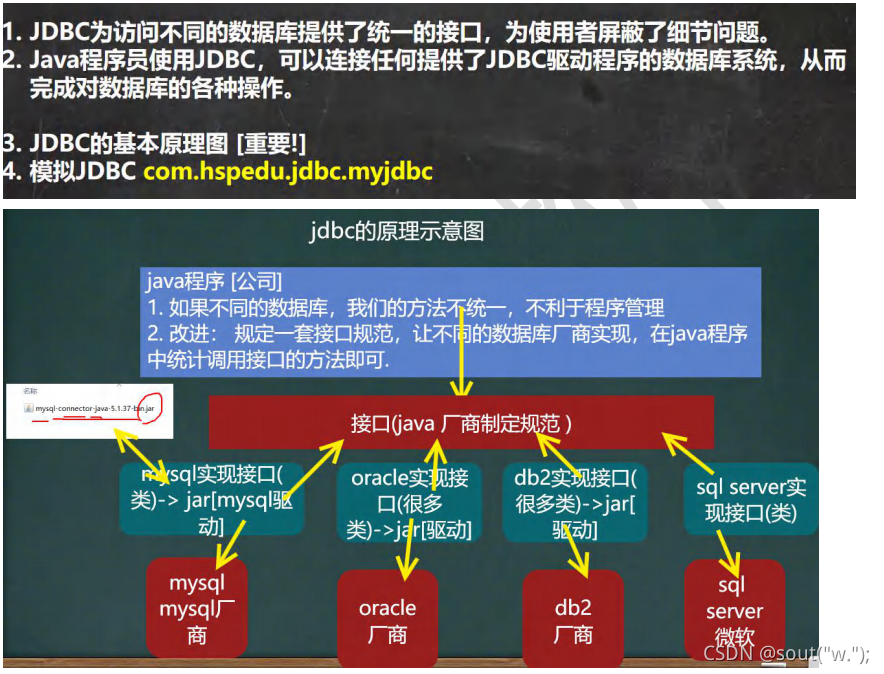
2.JDBC快速入门
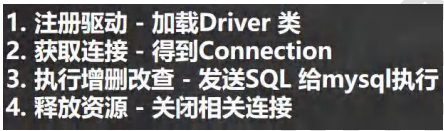 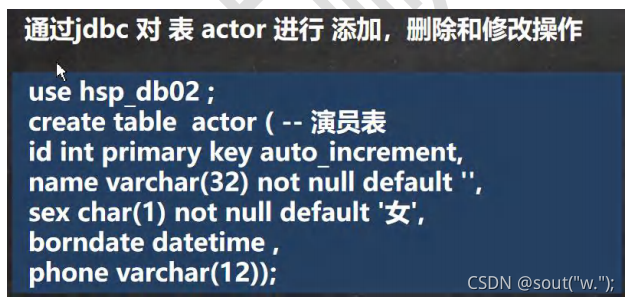 url中  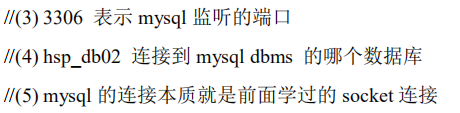
package com.lwb.jdbc.myjdbc;
import com.mysql.jdbc.Driver;
import java.sql.Connection;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Properties;
public class jdbc01 {
public static void main(String[] args) throws SQLException {
Driver driver = new Driver();
String url = "jdbc:mysql://localhost:3306/lwb_db02";
Properties properties = new Properties();
properties.setProperty("user","root");
properties.setProperty("password","lwb");
Connection connect = driver.connect(url, properties);
String sql = "INSERT INTO actor VALUES (1,'刘德华','男','1964-06-23','1100')";
Statement statement = connect.createStatement();
int i = statement.executeUpdate(sql);
System.out.println(i>0?"成功":"失败");
statement.close();
connect.close();
}
}
3.数据库连接的五种方式
package com.lwb.jdbc.myjdbc;
import com.mysql.jdbc.Driver;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Properties;
public class jdbcConn {
public static void main(String[] args) throws SQLException, IllegalAccessException, InstantiationException, ClassNotFoundException, IOException {
connect01();
connect02();
connect03();
connect04();
connect05();
}
public static void connect01() throws SQLException {
Driver driver = new Driver();
String url = "jdbc:mysql://localhost:3306/lwb_db02";
Properties properties = new Properties();
properties.setProperty("user","root");
properties.setProperty("password","lwb");
Connection connect = driver.connect(url, properties);
System.out.println(connect);
connect.close();
}
public static void connect02() throws ClassNotFoundException, IllegalAccessException, InstantiationException, SQLException {
Class<?> aClass = Class.forName("com.mysql.jdbc.Driver");
Driver driver = (Driver)aClass.newInstance();
String url = "jdbc:mysql://localhost:3306/lwb_db02";
Properties properties = new Properties();
properties.setProperty("user","root");
properties.setProperty("password","lwb");
Connection connect = driver.connect(url, properties);
System.out.println(connect);
connect.close();
}
public static void connect03() throws ClassNotFoundException, IllegalAccessException, InstantiationException, SQLException {
Class<?> aClass = Class.forName("com.mysql.jdbc.Driver");
Driver driver = (Driver)aClass.newInstance();
String url = "jdbc:mysql://localhost:3306/lwb_db02";
String user = "root";
String password = "lwb";
DriverManager.registerDriver(driver);
Connection connection = DriverManager.getConnection(url, user, password);
System.out.println(connection);
connection.close();
}
public static void connect04() throws ClassNotFoundException, SQLException {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/lwb_db02";
String user = "root";
String password = "lwb";
Connection connection = DriverManager.getConnection(url, user, password);
System.out.println(connection);
connection.close();
}
public static void connect05() throws IOException, ClassNotFoundException, SQLException {
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
Class.forName(driver);
Connection connection = DriverManager.getConnection(url, user, password);
System.out.println(connection);
connection.close();
}
}
4.ResultSet
 查询下面表 
package com.lwb.jdbc.myjdbc;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.sql.*;
import java.util.Properties;
public class resultSet {
public static void main(String[] args) throws IOException, ClassNotFoundException, SQLException {
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
Class.forName(driver);
Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement();
String sql = "select * from actor";
ResultSet resultSet = statement.executeQuery(sql);
while(resultSet.next()){
int id = resultSet.getInt(1);
String name = resultSet.getString(2);
String sex = resultSet.getString(3);
Date date = resultSet.getDate(4);
System.out.println(id+","+name+","+sex+","+date);
}
resultSet.close();
statement.close();
connection.close();
}
}
5.sql语句
5.1 sql注入
statement 会存在sql注入问题,开发过程中一般不会使用 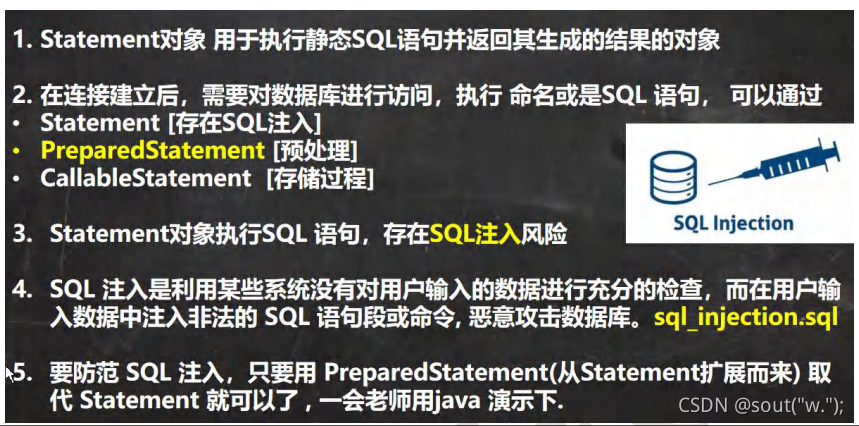
5.2 preparestatement
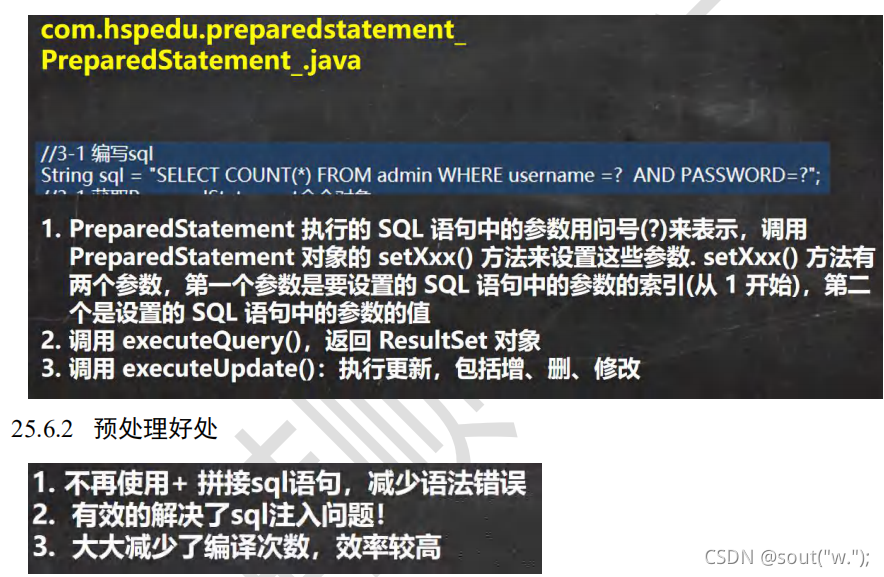
```java
package com.lwb.jdbc.myjdbc;
import java.io.*;
import java.sql.*;
import java.util.Properties;
import java.util.Scanner;
public class PrepareStatement {
public static void main(String[] args) throws ClassNotFoundException, SQLException, IOException {
Scanner sc = new Scanner(System.in);
System.out.println("请输入管理员的名字:");
String admin_name = sc.nextLine();
System.out.println("请输入管理员的密码:" );
String admin_pwd = sc.nextLine();
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
Class.forName(driver);
Connection connection = DriverManager.getConnection(url, user, password);
String sql ="select * from admin where name = ? and pwd = ?";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,admin_name);
preparedStatement.setString(2,admin_pwd);
ResultSet resultSet = preparedStatement.executeQuery();
if(resultSet.next()){
System.out.println("登录成功!");
}else{
System.out.println("登录失败!");
}
resultSet.close();
preparedStatement.close();
connection.close();
}
}
5.3 预处理DML
package com.lwb.jdbc.myjdbc;
import java.io.FileInputStream;
import java.io.IOException;
import java.sql.*;
import java.util.Properties;
import java.util.Scanner;
public class PrepareStatementDML {
public static void main(String[] args) throws ClassNotFoundException, SQLException, IOException {
Scanner sc = new Scanner(System.in);
System.out.println("请输入管理员的名字:");
String admin_name = sc.nextLine();
System.out.println("请输入管理员的密码:" );
String admin_pwd = sc.nextLine();
Properties properties = new Properties();
properties.load(new FileInputStream("src\\mysql.properties"));
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
String url = properties.getProperty("url");
Class.forName(driver);
Connection connection = DriverManager.getConnection(url, user, password);
String sql = "delete from admin where name = ?";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,admin_name);
int row = preparedStatement.executeUpdate();
if(row>0){
System.out.println("执行成功!");
}
preparedStatement.close();
connection.close();
}
}
6.JDBC API小结
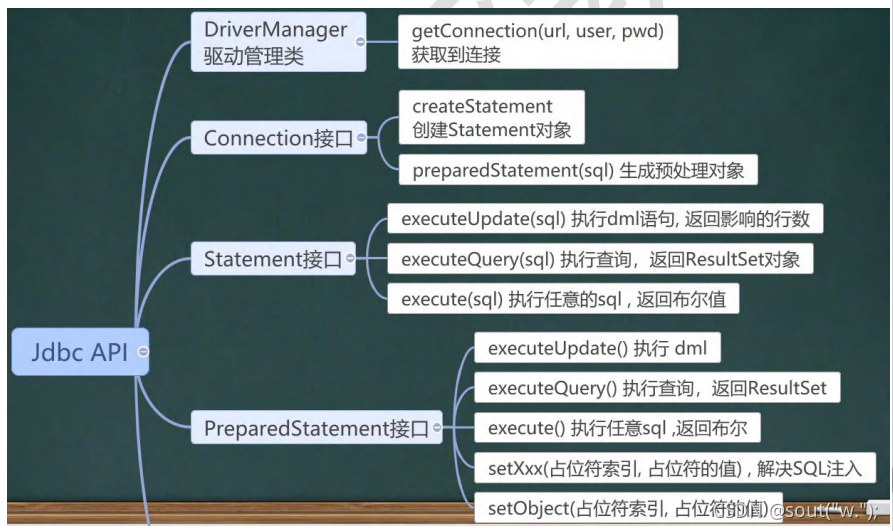 
7.JDBCUtils封装
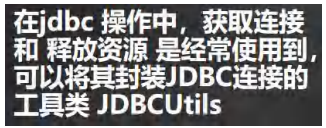
7.1 封装代码
public static void testSelect() {
Connection connection = null;
PreparedStatement preparedStatement =null;
ResultSet resultSet = null;
try {
connection = JDBCUtils.getConnection();
String sql = "select * from actor";
preparedStatement = connection.prepareStatement(sql);
resultSet = preparedStatement.executeQuery();
while(resultSet.next()){
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
System.out.println(id+","+name);
}
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtils.close(resultSet,preparedStatement,connection);
}
}
7.2 封装测试
package com.lwb.jdbc.util;
import java.sql.*;
public class JDBCUtils_Use {
public static void main(String[] args) {
testSelect();
}
public static void testDML(){
Connection connection = null;
PreparedStatement preparedStatement =null;
try {
connection = JDBCUtils.getConnection();
String sql = "update actor set name = ? where id = ?";
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1,"林伟波");
preparedStatement.setInt(2,1);
preparedStatement.executeUpdate();
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtils.close(null,preparedStatement,connection);
}
}
public static void testSelect() {
Connection connection = null;
PreparedStatement preparedStatement =null;
ResultSet resultSet = null;
try {
connection = JDBCUtils.getConnection();
String sql = "select * from actor";
preparedStatement = connection.prepareStatement(sql);
resultSet = preparedStatement.executeQuery();
while(resultSet.next()){
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
System.out.println(id+","+name);
}
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtils.close(resultSet,preparedStatement,connection);
}
}
}
8.事务
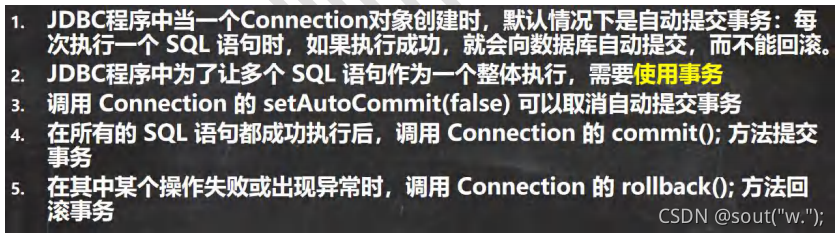

package com.lwb.jdbc.myjdbc;
import com.lwb.jdbc.util.JDBCUtils;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class Transaction_ {
public static void main(String[] args) {
transaction();
}
public static void notransaction(){
Connection connection = null;
String sql1 = "update account set balance = balance - 100 where id = 1";
String sql2 = "update account set balance = balance + 100 where id = 2";
PreparedStatement preparedStatement = null;
try {
connection = JDBCUtils.getConnection();
preparedStatement = connection.prepareStatement(sql1);
preparedStatement.executeUpdate();
int num = 1/0;
preparedStatement = connection.prepareStatement(sql2);
preparedStatement.executeUpdate();
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtils.close(null,preparedStatement,connection);
}
}
public static void transaction(){
Connection connection = null;
String sql1 = "update account set balance = balance - 100 where id = 1";
String sql2 = "update account set balance = balance + 100 where id = 2";
PreparedStatement preparedStatement = null;
try {
connection = JDBCUtils.getConnection();
connection.setAutoCommit(false);
preparedStatement = connection.prepareStatement(sql1);
preparedStatement.executeUpdate();
int num = 1/0;
preparedStatement = connection.prepareStatement(sql2);
preparedStatement.executeUpdate();
} catch (SQLException throwables) {
try {
connection.rollback();
} catch (SQLException e) {
e.printStackTrace();
}
throwables.printStackTrace();
}finally {
JDBCUtils.close(null,preparedStatement,connection);
}
}
}
9.批处理
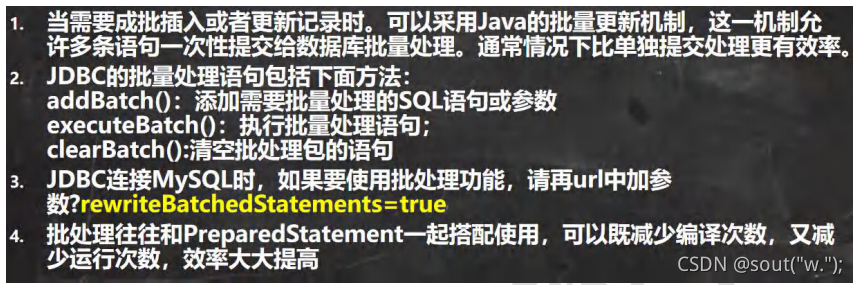 用有无批处理两种方法插入5000条语句
package com.lwb.jdbc.batch;
import com.lwb.jdbc.util.JDBCUtils;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class Batch_ {
public static void main(String[] args) throws SQLException {
batch_();
}
public static void nobatch() throws SQLException {
Connection connection = JDBCUtils.getConnection();
String sql = "insert into test values(?,?)";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
long start = System.currentTimeMillis();
for(int i = 0;i<5000;i++){
preparedStatement.setString(1,"111");
preparedStatement.setString(2,"tom"+i);
preparedStatement.executeUpdate();
}
long end = System.currentTimeMillis();
System.out.println("无批处理的时间:"+(end-start));
JDBCUtils.close(null,preparedStatement,connection);
}
public static void batch_() throws SQLException {
Connection connection = JDBCUtils.getConnection();
String sql = "insert into test values(?,?)";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
long start = System.currentTimeMillis();
for(int i = 0;i<5000;i++){
preparedStatement.setString(1,"111");
preparedStatement.setString(2,"tom"+i);
preparedStatement.addBatch();
if((i+1)%1000 == 0){
preparedStatement.executeBatch();
preparedStatement.clearBatch();
}
}
long end = System.currentTimeMillis();
System.out.println("批处理的时间:"+(end-start));
JDBCUtils.close(null,preparedStatement,connection);
}
}
10.数据库连接池
10.1 传统连接的弊端
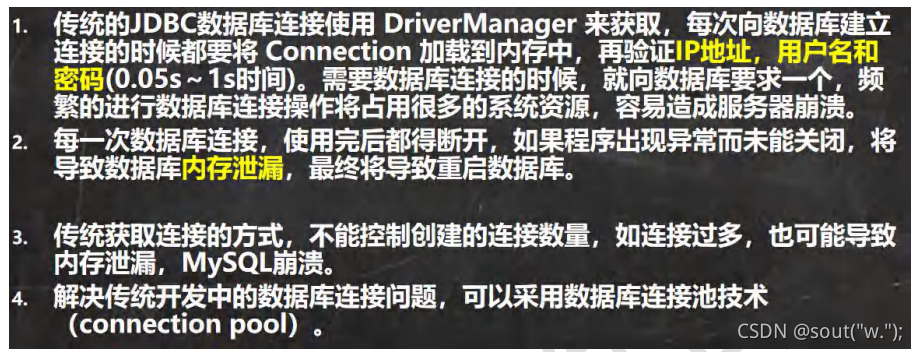
10.2 数据库连接池介绍
java程序创建一个数据库连接池,连接池里有若干连接,连接与数据库已经连通,当java程序需要使用时就从数据库连接池中取出一个连接。当连接全部被占用时,java程序想要取出连接就会进入等待队列,等待其他连接被放回数据库连接池时才会取出连接。取出和放回不会使连接与数据库的联系断开。 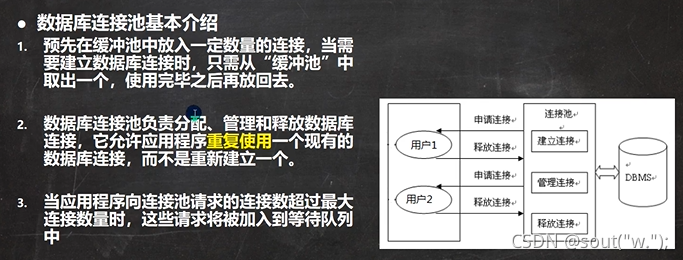 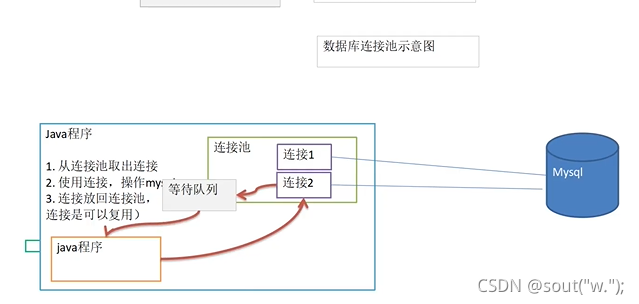 分类 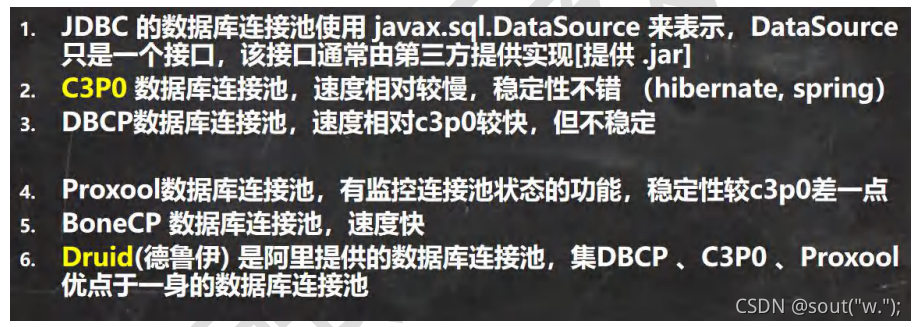
10.3 C3P0两种连接方式
package com.lwb.jdbc.dataSource;
import com.mchange.v2.c3p0.ComboPooledDataSource;
import java.beans.PropertyVetoException;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.Properties;
public class C3P0 {
public static void main(String[] args) throws IOException, PropertyVetoException, SQLException {
testC3P02();
}
public static void testC3P0() throws SQLException, PropertyVetoException, IOException {
ComboPooledDataSource comboPooledDataSource = new ComboPooledDataSource();
Properties properties = new Properties();
properties.load(new FileInputStream("src//mysql.properties"));
String url = properties.getProperty("url");
String user = properties.getProperty("user");
String password = properties.getProperty("password");
String driver = properties.getProperty("driver");
comboPooledDataSource.setDriverClass(driver);
comboPooledDataSource.setJdbcUrl(url);
comboPooledDataSource.setUser(user);
comboPooledDataSource.setPassword(password);
comboPooledDataSource.setInitialPoolSize(30);
comboPooledDataSource.setMaxPoolSize(50);
long start = System.currentTimeMillis();
for(int i = 0;i<5000;i++) {
Connection connection = comboPooledDataSource.getConnection();
connection.close();
}
long end = System.currentTimeMillis();
System.out.println("连接时间:"+(end-start));
}
public static void testC3P02() throws SQLException {
ComboPooledDataSource comboPooledDataSource = new ComboPooledDataSource("hello");
System.out.println("开始执行。。");
long start = System.currentTimeMillis();
for(int i = 0;i<5000;i++) {
Connection connection = comboPooledDataSource.getConnection();
connection.close();
}
long end = System.currentTimeMillis();
System.out.println("耗时:"+(end-start));
}
}
10.4 德鲁伊连接池使用
package com.lwb.jdbc.dataSource;
import com.alibaba.druid.pool.DruidDataSource;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import javax.sql.DataSource;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.sql.Connection;
import java.util.Properties;
public class Druid_ {
public static void main(String[] args) throws Exception {
druid01();
}
public static void druid01() throws Exception {
Properties properties = new Properties();
properties.load(new FileInputStream("src//druid.properties"));
DataSource dataSource = DruidDataSourceFactory.createDataSource(properties);
Connection connection = dataSource.getConnection();
System.out.println("连接成功");
connection.close();
}
}
10.5 德鲁伊连接池工具类
工具类代码
package com.lwb.jdbc.dataSource;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import javax.sql.DataSource;
import java.io.FileInputStream;
import java.io.IOException;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Properties;
public class JDBCUtilsByDruid {
private static DataSource ds = null;
static{
Properties properties = new Properties();
try {
properties.load(new FileInputStream("src\\druid.properties"));
ds = DruidDataSourceFactory.createDataSource(properties);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static Connection getConnection(){
try {
return ds.getConnection();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public static void close(ResultSet resultSet,Statement statement,Connection connection){
try {
if(resultSet!=null){
resultSet.close();
}
if(statement!=null){
statement.close();
}
if(connection!=null){
connection.close();
}
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
工具类使用
package com.lwb.jdbc.dataSource;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class JDBCUtilsDruid_use {
public static void main(String[] args) {
testDruidUtils();
}
public static void testDruidUtils() {
Connection connection = null;
PreparedStatement preparedStatement =null;
ResultSet resultSet = null;
try {
connection = JDBCUtilsByDruid.getConnection();
String sql = "select * from actor";
preparedStatement = connection.prepareStatement(sql);
resultSet = preparedStatement.executeQuery();
while(resultSet.next()){
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
System.out.println(id+","+name);
}
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtilsByDruid.close(resultSet,preparedStatement,connection);
}
}
}
10.6 DBUtils
10.6.1 引出
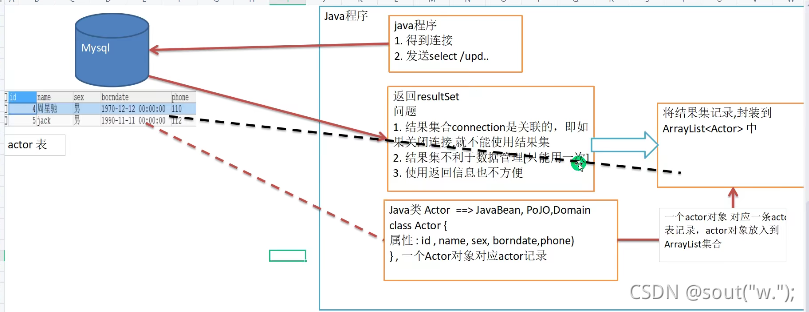
10.6.2 自己编写的代码解决ResultSet问题
(只截取方法部分)
public static void resultSetToArrayList() {
Connection connection = null;
PreparedStatement preparedStatement =null;
ResultSet resultSet = null;
ArrayList<Actor> list = new ArrayList<Actor>();
try {
connection = JDBCUtilsByDruid.getConnection();
String sql = "select * from actor";
preparedStatement = connection.prepareStatement(sql);
resultSet = preparedStatement.executeQuery();
while(resultSet.next()){
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
String sex = resultSet.getString("sex");
Date birthday = resultSet.getDate("birthday");
String phone = resultSet.getString("phone");
list.add(new Actor(id,name,sex,birthday,phone));
}
System.out.println(list);
} catch (SQLException throwables) {
throwables.printStackTrace();
}finally {
JDBCUtilsByDruid.close(resultSet,preparedStatement,connection);
}
}
10.6.3 DBUtils基本介绍和使用
基本介绍 实际上就是用来执行sql语句的 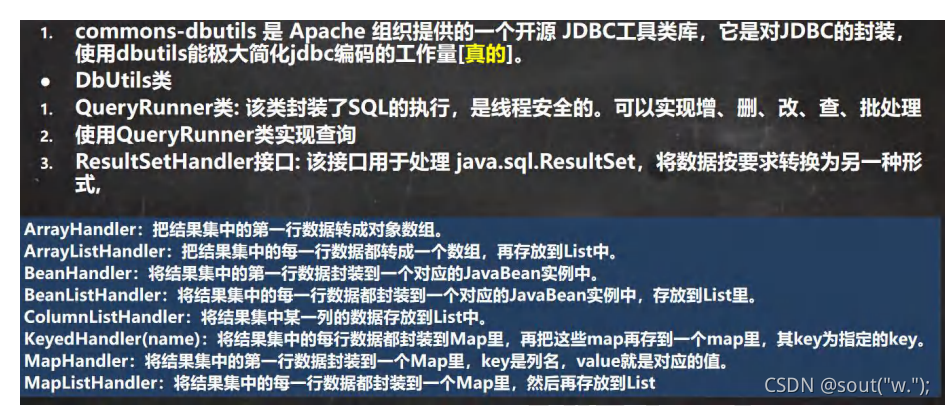 增删查改
package com.lwb.jdbc.dataSource;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.apache.commons.dbutils.handlers.ScalarHandler;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public class DBUtils_Use {
public static void main(String[] args) throws SQLException {
testDML();
}
public static void testQueryMary() throws SQLException {
Connection connection = JDBCUtilsByDruid.getConnection();
QueryRunner queryRunner = new QueryRunner();
String sql = "select * from actor where id >= ?";
List<Actor> list = queryRunner.query(connection, sql, new BeanListHandler<>(Actor.class), 1);
System.out.println("集合信息:");
for(Actor actor : list){
System.out.println(actor);
}
JDBCUtilsByDruid.close(null,null,connection);
}
public static void testQuerySingle() throws SQLException {
Connection connection = JDBCUtilsByDruid.getConnection();
QueryRunner queryRunner = new QueryRunner();
String sql = "select * from actor where id = ?";
Actor actor = queryRunner.query(connection, sql, new BeanHandler<>(Actor.class), 1);
System.out.println(actor);
JDBCUtilsByDruid.close(null,null,connection);
}
public static void testScalar() throws SQLException {
Connection connection = JDBCUtilsByDruid.getConnection();
QueryRunner queryRunner = new QueryRunner();
String sql = "select name from actor where id = ?";
Object obj = queryRunner.query(connection, sql, new ScalarHandler(), 1);
System.out.println(obj);
JDBCUtilsByDruid.close(null,null,connection);
}
public static void testDML() throws SQLException {
Connection connection = JDBCUtilsByDruid.getConnection();
QueryRunner queryRunner = new QueryRunner();
String sql = "delete from actor where id = ?";
int affectedRow = queryRunner.update(connection, sql, 3);
System.out.println(affectedRow>0?"执行成功":"执行没有影响数据库");
JDBCUtilsByDruid.close(null,null,connection);
}
}
10.7 BasicDAO
10.7.1 引出

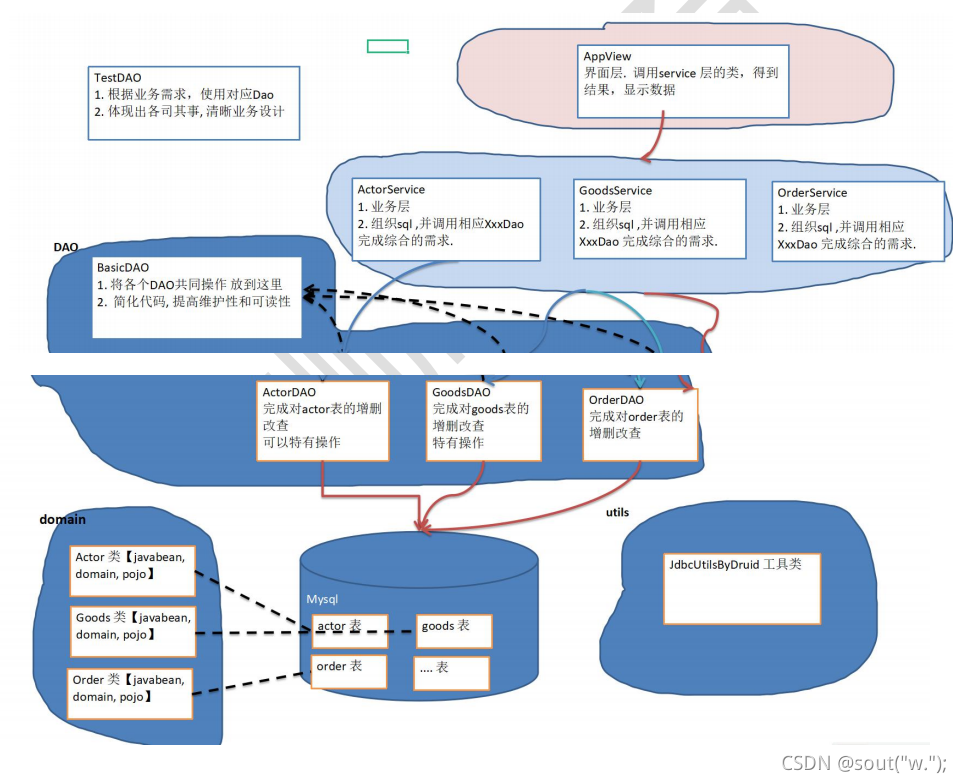 
10.7.2 应用实例
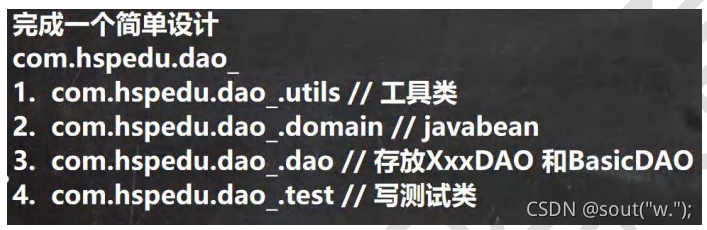 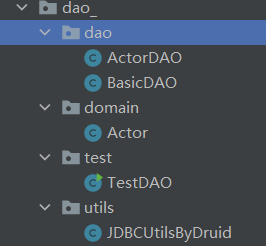 BasicDAO代码
package com.lwb.jdbc.dao_.dao;
import com.lwb.jdbc.dao_.utils.JDBCUtilsByDruid;
import com.lwb.jdbc.util.JDBCUtils;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanHandler;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.apache.commons.dbutils.handlers.ScalarHandler;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
public class BasicDAO<T> {
private QueryRunner queryRunner = new QueryRunner();
public int update(String sql,Object... parameters){
Connection connection = null;
try {
connection = JDBCUtilsByDruid.getConnection();
int row = queryRunner.update(connection, sql, parameters);
return row;
} catch (SQLException e) {
throw new RuntimeException(e);
}finally {
JDBCUtils.close(null,null,connection);
}
}
public List<T> queryMuti(String sql,Class<T> clazz,Object... parameters){
Connection connection = null;
try {
connection = JDBCUtilsByDruid.getConnection();
List<T> list = queryRunner.query(connection, sql, new BeanListHandler<T>(clazz),parameters);
return list;
} catch (SQLException e) {
throw new RuntimeException(e);
}finally {
JDBCUtils.close(null,null,connection);
}
}
public T querySingle(String sql,Class<T> clazz,Object... parameters){
Connection connection = null;
try {
connection = JDBCUtilsByDruid.getConnection();
return queryRunner.query(connection, sql, new BeanHandler<T>(clazz),parameters);
} catch (SQLException e) {
throw new RuntimeException(e);
}finally {
JDBCUtils.close(null,null,connection);
}
}
public Object queryScalar(String sql,Object... parameters){
Connection connection = null;
try {
connection = JDBCUtilsByDruid.getConnection();
return queryRunner.query(connection, sql, new ScalarHandler(),parameters);
} catch (SQLException e) {
throw new RuntimeException(e);
}finally {
JDBCUtils.close(null,null,connection);
}
}
}
ActorDAO代码
package com.lwb.jdbc.dao_.dao;
import com.lwb.jdbc.dao_.domain.Actor;
public class ActorDAO extends BasicDAO<Actor>{
}
Actor代码
package com.lwb.jdbc.dao_.domain;
import java.util.Date;
public class Actor {
private Integer id;
private String name;
private String sex;
private Date birthday;
private String phone;
public Actor() {
}
public Actor(Integer id, String name, String sex, Date birthday, String phone) {
this.id = id;
this.name = name;
this.sex = sex;
this.birthday = birthday;
this.phone = phone;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public Date getBirthday() {
return birthday;
}
public void setBirthday(Date birthday) {
this.birthday = birthday;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
@Override
public String toString() {
return "Actor{" +
"id=" + id +
", name='" + name + '\'' +
", sex='" + sex + '\'' +
", birthday=" + birthday +
", phone='" + phone + '\'' +
'}';
}
}
JDBCUtilsByDruid代码
package com.lwb.jdbc.dao_.utils;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import javax.sql.DataSource;
import java.io.FileInputStream;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Properties;
public class JDBCUtilsByDruid {
private static DataSource ds = null;
static{
Properties properties = new Properties();
try {
properties.load(new FileInputStream("src\\druid.properties"));
ds = DruidDataSourceFactory.createDataSource(properties);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static Connection getConnection(){
try {
return ds.getConnection();
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
public static void close(ResultSet resultSet,Statement statement,Connection connection){
try {
if(resultSet!=null){
resultSet.close();
}
if(statement!=null){
statement.close();
}
if(connection!=null){
connection.close();
}
} catch (SQLException e) {
throw new RuntimeException(e);
}
}
}
testDAO代码
package com.lwb.jdbc.dao_.test;
import com.lwb.jdbc.dao_.dao.ActorDAO;
import com.lwb.jdbc.dao_.domain.Actor;
import java.util.List;
public class TestDAO {
public static void main(String[] args) {
ActorDAO actorDAO = new ActorDAO();
List<Actor> actors = actorDAO.queryMuti("select * from actor where id >= ?", Actor.class, 1);
System.out.println("===多行查询===");
for (Actor actor : actors) {
System.out.println(actor);
}
Actor actor = actorDAO.querySingle("select * from actor where id = ?", Actor.class, 1);
System.out.println("===单行查询===");
System.out.println(actor);
Object obj = actorDAO.queryScalar("select name from actor where id = ?",1);
System.out.println("===单行单列查询===");
System.out.println(obj);
int update = actorDAO.update("insert into actor values(null,?,?,?,?)", "张无忌", "男", "1999-01-01", "115");
System.out.println(update>=0?"执行成功":"执行失败");
}
}
|