例子
创建学生表格 create table student ( sno char(5), sname char(10), ssex char(2), sage int, sdept char(20), constraint pk_student primary key(sno), constraint student_age check(sage>=1 and sage<=150) )engine=innoDB charset=utf8 collate=utf8_bin;
插入数据 insert into student(sno,sname,sage,ssex,sdept) values(‘10001’,‘李勇’,20,‘男’,‘CS’); insert into student(sno,sname,sage,ssex,sdept) values(‘10002’,‘刘晨’,19,‘女’,‘CS’); insert into student(sno,sname,sage,ssex,sdept) values(‘10003’,‘王敏’,18,‘女’,‘MA’); insert into student(sno,sname,sage,ssex,sdept) values(‘10004’,‘张立’,19,‘男’,‘IS’); 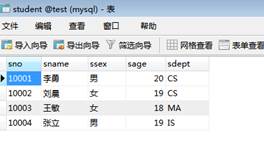 创建课程表 create table course ( cno int, cname char(20), cpno int, ccredit int, constraint pk_course primary key(cno) )engine=innoDB charset=utf8 collate=utf8_bin;
插入课程数据 insert into course(cno,cname,cpno,ccredit) values(1,‘数据库’,5,4); insert into course(cno,cname,cpno,ccredit) values(2,‘数学’,null,2); insert into course(cno,cname,cpno,ccredit) values(3,‘信息系统’,1,4); insert into course(cno,cname,cpno,ccredit) values(4,‘操作系统’,6,3); insert into course(cno,cname,cpno,ccredit) values(5,‘数据结构’,7,4); insert into course(cno,cname,cpno,ccredit) values(6,‘数据处理’,null,2); insert into course(cno,cname,cpno,ccredit) values(7,‘PASCAL语言’,6,4); 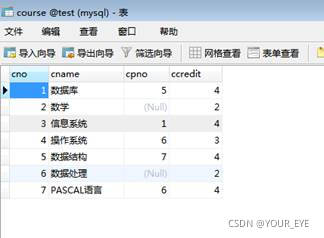 创建成绩表 create table sc( sno char(5), cno int, grade int, constraint pk_sc primary key(sno,cno), constraint fk_student foreign key(sno) references student(sno), constraint fk_course foreign key(cno) references course(cno), constraint sc_grade check(grade>=0 and grade<=100) )engine=innoDB charset=utf8 collate=utf8_bin;
插入成绩 insert into sc(sno,cno,grade) values(‘10001’,1,92); insert into sc(sno,cno,grade) values(‘10001’,2,85); insert into sc(sno,cno,grade) values(‘10001’,3,88); insert into sc(sno,cno,grade) values(‘10002’,2,90); insert into sc(sno,cno,grade) values(‘10002’,3,80);
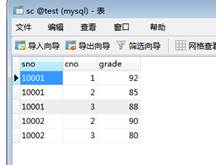
1.在select语句中使用*号通配符查询所有字段
set names gbk; 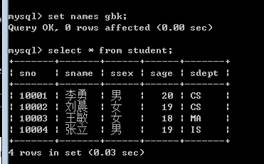
2.在select语句中指定字段
select sno,sname from student 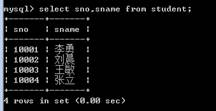
3.查询指定记录
select * from student where sname=‘李勇’; select sname from student where sage >18 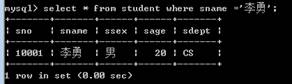 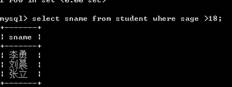
4.IN关键字//用于char格式
select * from student where sno in(‘10001’,‘10004’); 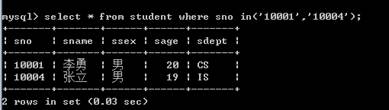
5.between and 关键字//用于int格式
select * from student where sage between 19 and 20; 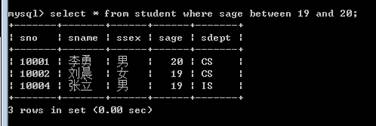
6.字符串匹配
select * from student where sno like ‘1_’; //’_'是单个未知字符的查询符号 select * from student where sno like ‘1%4’; 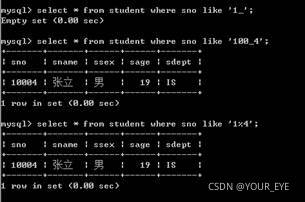
7.空值查询
select * from course where cpno is null select * from courese where cpno is not null 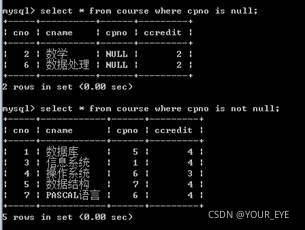
8.and查询
select * from student where ssex='男‘ and sdept=‘CS’; 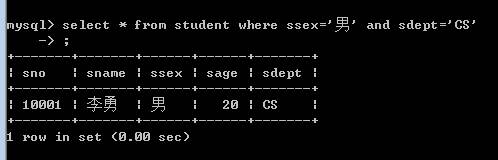
9.OR查询
select * from student where sdept=‘CS’ or sage=19; 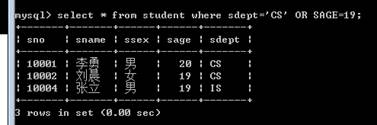
10.查询结果不重复
select sno from sc; select distinct sno from sc; 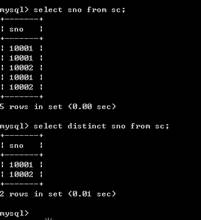
11.排序
12.查询学号为‘10001’学生的姓名和成绩
select sname,grade from student inner join sc on student.sno=sc.sno where student.sno=‘10001’ order by sname;
select sname,grade //投影 --1 from student inner join sc on student.sno=sc.sno //连接 --2 where student.sno=‘10001’ //选择 --3 order by sname; //排序 --4 运行顺序是 2 3 1
一 单表查询
结构 : select 列 from 表名 where … 最简单的顺序
1.查询所有的列
select * from sc seelct sno,cno,grade from sc *****代表所有的列
2.查询指定列
查询学生表所有的学号,学生名 select sno,sname from student;
3.查询指定条件的行
查询"王X"学生的学号(两个字符长度) select sno from student where sname like ‘王_’ '_'表示一个字符
4.查询所有"王"姓学生的学号(名字为多个字符)
select sno from student where sname like ‘王%’ '%'表示0个或多个字符
5.查询年龄从18到20岁的学生的学号
select sno from student where sage>=18 and sage<=20 或 select sno from student where sage between 18 and 20
6.消除重复行distinct关键字
查询成绩表的sno字段,不允许重复 select distinct sno from sc
7.排序
查询学生年龄按升序排序 select * from student order by sage asc; 查询学生年龄按降排序 select * from student order by sage desc;
8.IN关键字
查询年龄18,20学生的信息 select * from student where sage=18 or sage=20 或 select * from student where sage in (18,20) 提示:in关键字表示集合操作 or 表示或
聚集函数 对查询结果做统计操作
查询有多少个学生
select count(*) from student;
查询学生的平均年龄
select avg(sage) from student;
查询学生的年龄总和
select sum(sage) from student;
查询学生的年龄最大值
select max(sage) from student;
查询学生的年龄最小值
select min(sage) from student;
查询:按照学生所在系进行分组 统计 每个小组的平均年龄且每小组的人数不低于2个人
select sdept ,avg(sage) from student group by sdept having count(*)>=2;
select sdept ,avg(sage) //投影–1 group by sdept //分组–2 having count(*)>=2 //小组筛选–3 from student //连接 (单表没有连接(这里))–4 where … //选择 这里没有选择–5 order by …//排序 这个语句没有–6 运行的顺序是 4 5 2 3 6 1
更新操作
对学生表所有的年龄都加1
update student set sage=sage+1
对学号是‘10001’学生年龄加1
update student set sage=sage+1 where sno=‘10001’
删除操作
删除学生表所有数据
delete from student;
删除学生表学号为10001学生的数据
delete from student where sno=‘10001’
插入数据
insert into sc(sno,cno,grade) values(‘10005’,9,99)
分组 分组的目的是为了做统计 统计工作是由聚集函数完成的 所以分组要使用聚集函数
要按系别分组统计每个系的人数
select sdept,ssex,count(*) from student group by sdept,ssex; 注意:聚集函数前是分组的列
连接 select … from 表1,表2 where …;
步骤 步骤1 表1和表2做笛卡尔积 步骤2 对步骤1的结果做条件筛选(where条件) 步骤3 对步骤2的结果投影
学生表和成绩表的笛卡尔积
select * from student,sc; select count(*) from student,sc; 20
表1 6行 表2 7行 表1和表2的笛卡尔积6*7行(考试内容)
连接 学生表和成绩表连接 select sno ,grade from sc,student where sc.sno=student.sno
步骤1 表1和表2做笛卡尔积
步骤2 对步骤1的结果做条件筛选(where条件)
步骤3 对步骤2的结果投影
查询学生学生姓名为“王敏”的学生的所有成绩 查询学生学生姓名为“王敏”的学生的所学课程号 查询学生学生姓名为“王敏”的学生的所学课程名
|