ElasticSearch学习
1 安装运行
ElasticSearch是开箱即用,下载之后直接运行ElasticSearch.bat文件,默认端口是9200:,运行,然后打开请求9200端口,出现以下的描述说明安装成功: 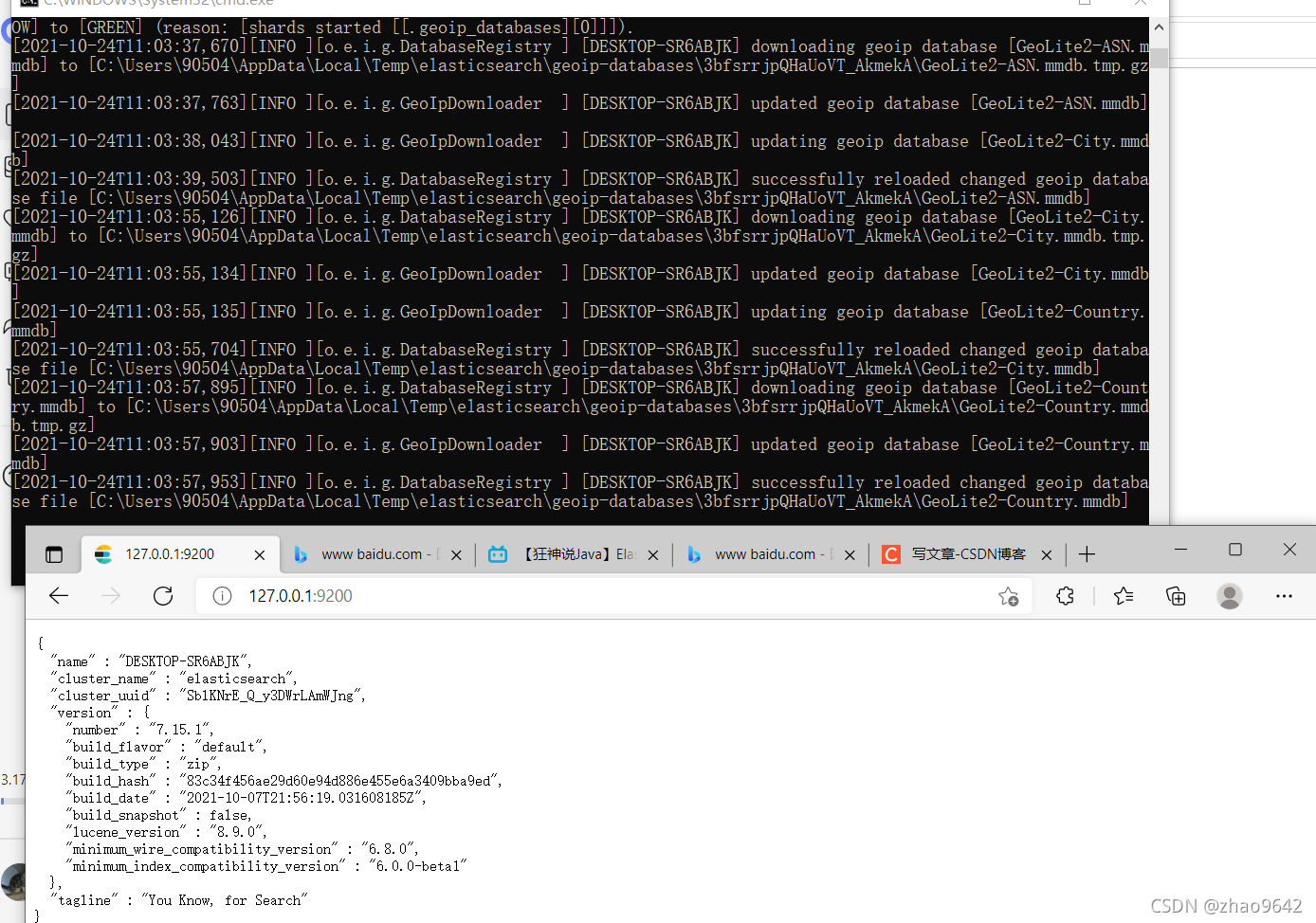
2 前端展示:
ElasticSearch 需要前端界面进行展示交互: 需要先安装NodeJS: https://github.com/mobz/elasticsearch-head,在这里我用的是git进行安装
git clone git://github.com/mobz/elasticsearch-head.git
cd elasticsearch-head
npm install
npm run start
然后打开 http://localhost:9100/ 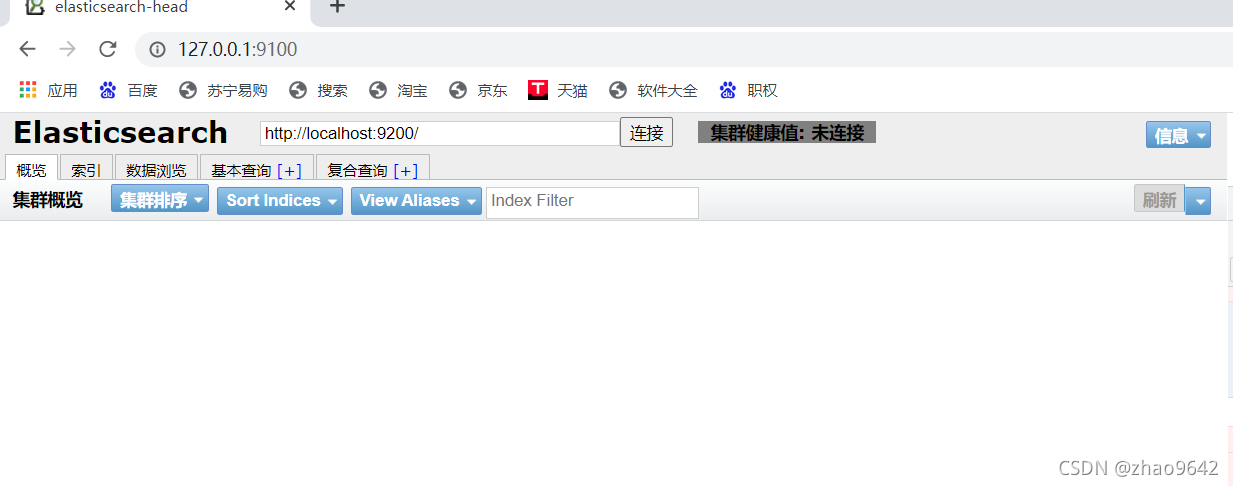 然后要解决跨域问题!
http.cors.enabled: true
http.cors.allow-origin: /.*/
这样子就可以解决跨域问题了
ES的基本操作,
这里借用狂神的笔记截图,上面不同的请求方法提供了不同的操作 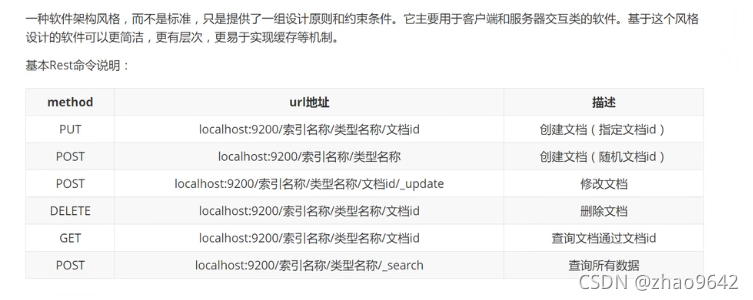
ES put创建新索引:
PUT /zlx/testindex/textDocument
{
"name":"zlx",
"usedFor":"test",
"id": "1"
}
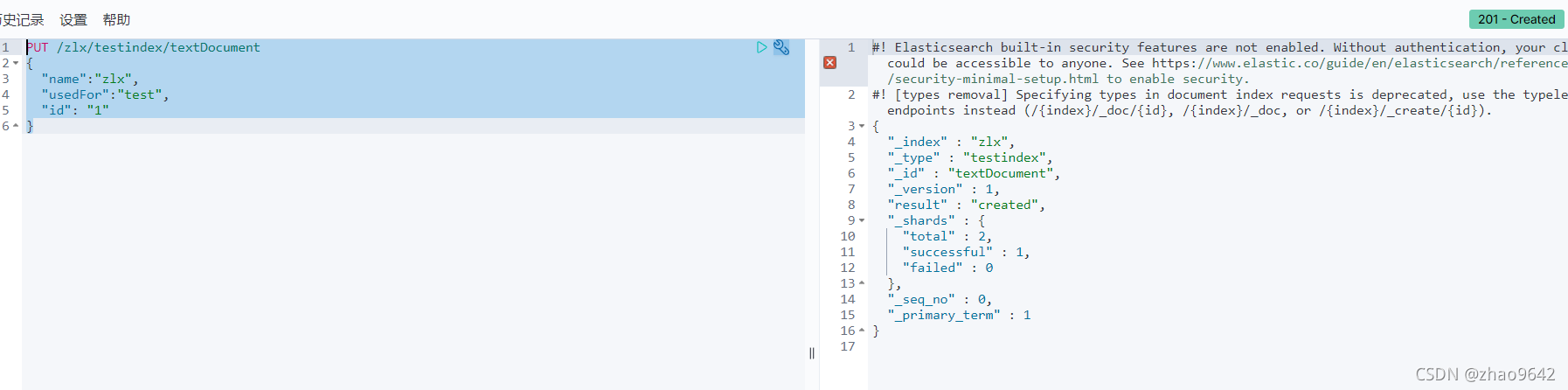 实际上上面的Kibana的作用是在测试的时候写ES的测试数据用的。重点是在SpringBoot 上集成。要说到集成,就需要先配置依赖环境:
SpringBoot ElasticSearch 依赖环境
<dependencies>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.76</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
接下来就是快乐的CRUD以及批量插入和搜索:
增
增加:
@Test
void CreateIndex() throws IOException {
CreateIndexRequest createIndexRequest=new CreateIndexRequest("zlx_index");
CreateIndexResponse createIndexResponse=client.indices().create(createIndexRequest, RequestOptions.DEFAULT);
System.out.println(createIndexResponse);
}
@Test
void TestADDDocument() throws IOException {
User user=new User();
user.setName("zlx");
user.setAge(25);
IndexRequest request = new IndexRequest("zlx_index");
request.id("1");
request.timeout(TimeValue.timeValueSeconds(2));
request.source(JSON.toJSONString(user), XContentType.JSON);
IndexResponse response= client.index(request,RequestOptions.DEFAULT);
System.out.println(response.toString());
System.out.println(response.status());
}
删
删除
oid testDeleteIndex() throws IOException {
DeleteIndexRequest deleteIndexRequest =new DeleteIndexRequest("zlx_index");
AcknowledgedResponse response=client.indices().delete(deleteIndexRequest,RequestOptions.DEFAULT);
System.out.println(response.isAcknowledged());
}
public boolean deleteDoc(String index,String id) throws IOException {
DeleteRequest request = new DeleteRequest(index,id);
request.timeout(TimeValue.timeValueSeconds(1));
request.timeout("1s");
DeleteResponse deleteResponse = client.delete(
request, RequestOptions.DEFAULT);
return deleteResponse.status()== RestStatus.OK;
}
改
修改
@Test
void updateMessage() throws IOException {
UpdateRequest updateRequest =new UpdateRequest("zlx_index","1");
updateRequest.timeout("1s");
User user =new User(18,"zlx_update");
updateRequest.doc(user,XContentType.JSON);
UpdateResponse response=client.update(updateRequest,RequestOptions.DEFAULT);
System.out.println(response.toString());
}
查
在这里插入代码片
void GetRequest(){
GetRequest getRequest = new GetRequest("zlx_index", "1");
getRequest.fetchSourceContext(new FetchSourceContext(false));
getRequest.storedFields("_none_");
boolean exists = client.exists(getRequest, RequestOptions.DEFAULT);
}
void ShowMessage() throws IOException {
GetRequest request = new GetRequest("zlx_index","1");
GetResponse getResponse = client.get(request,
RequestOptions.DEFAULT);
System.out.println(getResponse.toString());
}
搜
@Test public void Search() throws IOException {
SearchRequest searchRequest = new SearchRequest("zlx_index");
SearchSourceBuilder searchSourceBuilder =new SearchSourceBuilder();
TermQueryBuilder termQueryBuilder=new TermQueryBuilder("name","zlx");
searchSourceBuilder.query(termQueryBuilder);
searchSourceBuilder.timeout(TimeValue.timeValueNanos(60));
searchRequest.source(searchSourceBuilder);
SearchResponse searchResponse =client.search(searchRequest,RequestOptions.DEFAULT);
System.out.println(JSON.toJSONString(searchResponse.getHits()));
System.out.println("-------------------------------------------");
for (SearchHit docu:searchResponse.getHits().getHits())
{
System.out.println(docu.getSourceAsMap());
}
}
|