1.查询
效果展示:
前端页:
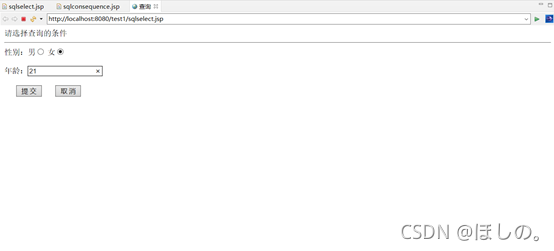
后台页:
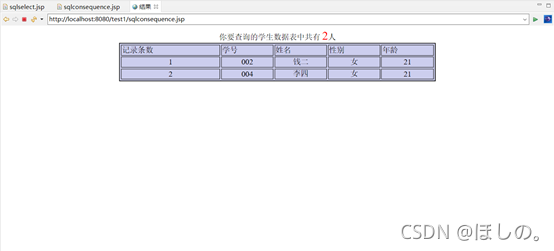
代码:
前端:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>查询</title>
</head>
<body>
请选择查询的条件<hr>
<form action="sqlconsequence.jsp" method="post">
性别:男<input type="radio" value="男" name="sex">
女<input type="radio" value="女" name="sex"><br><br>
年龄:<input type="text" name="age"><br><br>
<input type="submit" value="提 交">
<input type="reset" value="取 消">
</form>
</body>
</html>
?后台:
<%@ page import="java.sql.*"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>结果</title>
</head>
<body>
<%
// 先连接数据库
String driverName = "com.mysql.jdbc.Driver"; //驱动程序名
String userName = "root"; //数据库用户名
String userPwd = "123456"; //密码
String dbName = "website"; //数据库名
String url1="jdbc:mysql://localhost:3306/"+dbName;
String url2 ="?user="+userName+"&password="+userPwd;
String url3="&useUnicode=true&characterEncoding=UTF-8";//访问数据库的汉字编码
String url =url1+url2+url3; //形成带数据库读写编码的数据库连接字
Class.forName(driverName); //加载并注册驱动程序
Connection conn=DriverManager.getConnection(url); //创建连接对象
request.setCharacterEncoding("UTF-8");//设置字符编码,避免出现乱码
String sex = request.getParameter("sex");
int age=Integer.parseInt(request.getParameter("age"));
String sql = "select * from student where sex=? and age=?";
PreparedStatement preparedStatement = conn.prepareStatement(sql,ResultSet.TYPE_SCROLL_INSENSITIVE,ResultSet.CONCUR_READ_ONLY);//解决游标不能移动问题
preparedStatement.setString(1,sex);
preparedStatement.setInt(2,age);
ResultSet resultSet = preparedStatement.executeQuery();//执行sql语句用来返回单个 ResultSet 对象
resultSet.last();//移动到最后一条记录
%>
<center>
你要查询的学生数据表中共有
<font size="5" color="red"><%=resultSet.getRow()%></font>人
<table border="2" bgcolor="ccceee" width="650">
<tr>
<td>记录条数</td>
<td>学号</td>
<td>姓名</td>
<td>性别</td>
<td>年龄</td>
</tr>
<%
resultSet.beforeFirst();
while (resultSet.next()){
%> <tr align="center">
<td><%=resultSet.getRow()%></td>
<td><%=resultSet.getString("no")%></td>
<td><%=resultSet.getString("name")%></td>
<td><%=resultSet.getString("sex")%></td>
<td><%=resultSet.getInt("age")%></td>
</tr>
<%}%>
</table>
</center>
<%
if (resultSet!=null){
resultSet.close();
}
if (preparedStatement!=null){
preparedStatement.close();
}
if (conn!=null){
conn.close();
}
%>
</body>
</html>
</body>
</html>
2.添加
效果展示:
前端页:
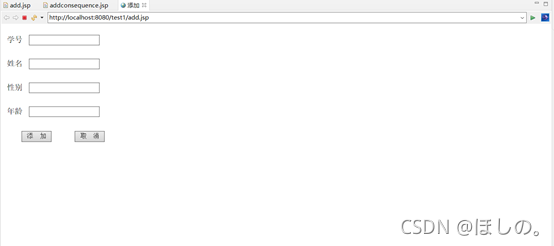
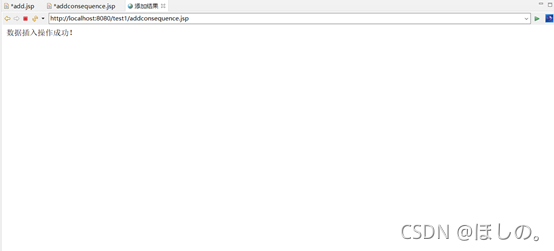
代码:
前端:
<%@page contentType="text/html" pageEncoding="GB2312"%>
<html>
<head> <title>添加</title> </head>
<body>
<form action= "addconsequence.jsp" method="post">
<table border="0" width="238" height="252">
<tr>
<td>学号 </td>
<td><input type="text" name="no"></td>
</tr>
<tr>
<td>姓名</td>
<td><input type="text" name="name"></td>
</tr>
<tr>
<td>性别</td>
<td><input type="text" name="sex" ></td>
</tr>
<tr>
<td>年龄</td>
<td><input type="text" name="age"></td>
</tr>
<tr align="center">
<td colspan="2">
<input type="submit" value="添 加">
<input type="reset" value="取 消">
</td>
</tr>
</table>
</form>
</body>
</html>
后端:
<%@ page language="java" import="java.sql.*" pageEncoding="GB2312"%>
<html>
<head>
<title>添加结果</title>
</head>
<body>
<%
String driverName = "com.mysql.jdbc.Driver"; //驱动程序名
String userName = "root"; //数据库用户名
String userPwd = "123456"; //密码
String dbName = "website"; //数据库名
String url1="jdbc:mysql://localhost:3306/"+dbName;
String url2 ="?user="+userName+"&password="+userPwd;
String url3="&useUnicode=true&characterEncoding=GB2312";
String url =url1+url2+url3; //形成带数据库读写编码的数据库连接字
Class.forName(driverName);
Connection conn=DriverManager.getConnection(url);
String sql="insert into student(no,name, sex, age) values(?,?,?,?)";
PreparedStatement pstmt= conn.prepareStatement(sql,ResultSet.TYPE_SCROLL_INSENSITIVE,ResultSet.CONCUR_READ_ONLY); //括号内解决游标不能移动
request.setCharacterEncoding("GB2312");//设置字符编码,避免出现乱码
String no=request.getParameter("no");
String name=request.getParameter("name");
String sex=request.getParameter("sex");
int age=Integer.parseInt(request.getParameter("age"));
pstmt.setString(1,no);
pstmt.setString(2,name);
pstmt.setString(3,sex);
pstmt.setInt(4,age);
try{
int n=pstmt.executeUpdate();
if(n==1){%>
数据插入操作成功!<br>
<%}
else{%>
数据插入操作失败!<br>
<%}
}catch(Exception e){%>
更新过程出现异常错误!<br>
<%=e.getMessage()%>
<%;
}
if(pstmt!=null){ pstmt.close(); }
if(conn!=null){ conn.close(); }
%>
</body>
</html>
!需要注意的是,该代码能够实现的前提是配置好连接数据库的环境,需要下载对应的数据库驱动器,并把jar文件复制到lib文件下才可实现。
|