最近有个小伙伴私信我,说刚开始接触c#,想做一个身份信息录入并且可以查询的软件,问我该怎么做?于是,我写了个demo,我们先来看一下效果
- 主界面效果图
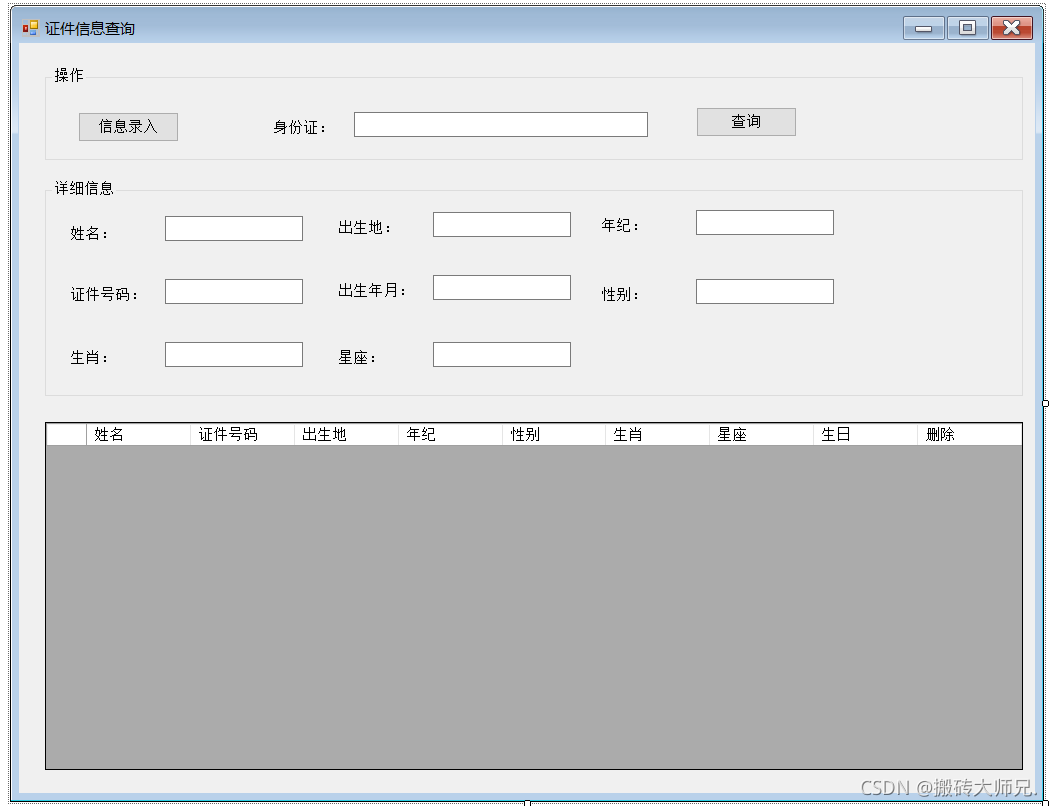 - 信息录入界面效果图
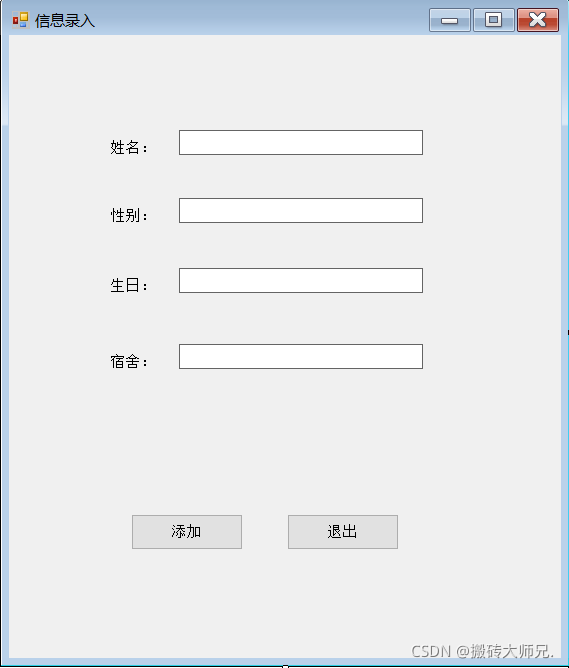
- 项目详解,为了方便数据储存,我使用了access数据库作为本次demo的数据库,c#操作access的数据库的核心代码如下【可以直接复制使用】:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Data;
using System.Data.OleDb;
namespace infoManagement
{
public class DataHelpher
{
static string filepath = System.Environment.CurrentDirectory + "\\info.accdb";
public static string GetDbPath(string path)
{
string str = "Provider = Microsoft.Ace.OLEDB.12.0;Persist Security Info=False;Data Source=" + path;
return str;
}
public static DataTable GetData(string sql)
{
DataTable dt = null;
OleDbConnection conn = new OleDbConnection(GetDbPath(filepath));
try
{
if (conn.State == ConnectionState.Closed)
{
conn.Open();
}
OleDbDataAdapter adapter = new OleDbDataAdapter(sql, conn);
DataSet set = new DataSet();
adapter.Fill(set);
dt = set.Tables[0];
}
catch (Exception ex)
{
return null;
}
finally
{
conn.Close();
}
return dt;
}
public static Boolean UpdateAndInsertAndDelete(string sql)
{
bool re = false;
OleDbConnection conn = new OleDbConnection(GetDbPath(filepath));
try
{
if (conn.State == ConnectionState.Closed)
{
conn.Open();
}
OleDbCommand cmd = conn.CreateCommand();
cmd.CommandText = sql;
cmd.ExecuteNonQuery();
re = true;
}
catch (Exception ex)
{
Console.WriteLine(ex.Message.ToString() + sql);
}
finally
{
conn.Close();
}
return re;
}
}
}
主界面的代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace infoManagement
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
StartPosition = FormStartPosition.CenterScreen;
this.dataGridView1.DataSource = GetList();
}
public List<InfoModel> GetList()
{
List<InfoModel> list = new List<InfoModel>();
DataTable dt = DataHelpher.GetData("select * from c_user");
if (dt != null && dt.Rows.Count > 0)
{
for (int i = 0; i < dt.Rows.Count; i++)
{
InfoModel ml = new InfoModel();
ml.bir = dt.Rows[i]["bir"].ToString();
ml.name = dt.Rows[i]["name"].ToString();
ml.room = dt.Rows[i]["room"].ToString();
ml.xb = dt.Rows[i]["xb"].ToString();
ml.updatetime = dt.Rows[i]["updatetime"].ToString();
ml.del = "删除";
ml.edit = "编辑";
list.Add(ml);
}
}
else
{
list = null;
}
return list;
}
private void button1_Click(object sender, EventArgs e)
{
InfoAddForm add = new InfoAddForm();
if (add.ShowDialog() == DialogResult.OK)
{
this.dataGridView1.DataSource = GetList();
}
}
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
string value = this.dataGridView1.Rows[e.RowIndex].Cells[e.ColumnIndex].Value.ToString();
if (value == "删除")
{
Delete(this.dataGridView1.SelectedRows[0].Cells[0].Value.ToString());
this.dataGridView1.DataSource = GetList();
}
else if (value == "编辑")
{
InfoEditForm form = new InfoEditForm(this.dataGridView1.SelectedRows[0].Cells[0].Value.ToString());
if (form.ShowDialog() == DialogResult.OK)
{
this.dataGridView1.DataSource = GetList();
}
}
}
public void Delete(string name)
{
string sql = string.Format("delete from c_user where name='{0}'", name);
DataHelpher.UpdateAndInsertAndDelete(sql);
}
private void button2_Click(object sender, EventArgs e)
{
string text = this.textBox1.Text;
if (string.IsNullOrEmpty(text)&&(!radioButton_all.Checked))
{
MessageBox.Show("请输入参数");
}
else
{
string sql = string.Format("select * from c_user where name='{0}'", text);
if (radioButton_bir.Checked)
{
sql = string.Format("select * from c_user where bir='{0}'", text);
}
else if (radioButton_room.Checked)
{
sql = string.Format("select * from c_user where room='{0}'", text);
}
else if (radioButton_xb.Checked)
{
sql = string.Format("select * from c_user where xb='{0}'", text);
}
else if (radioButton_all.Checked) {
sql = string.Format("select * from c_user");
}
DataTable dt = DataHelpher.GetData(sql);
List<InfoModel> list = new List<InfoModel>();
if (dt != null && dt.Rows.Count > 0)
{
for (int i = 0; i < dt.Rows.Count; i++)
{
InfoModel ml = new InfoModel();
ml.bir = dt.Rows[i]["bir"].ToString();
ml.name = dt.Rows[i]["name"].ToString();
ml.room = dt.Rows[i]["room"].ToString();
ml.xb = dt.Rows[i]["xb"].ToString();
ml.updatetime = dt.Rows[i]["updatetime"].ToString();
ml.del = "删除";
ml.edit = "编辑";
list.Add(ml);
}
}
this.dataGridView1.DataSource = list;
}
}
}
}
信息录入代码如下:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Data;
namespace infoManagement
{
public partial class InfoAddForm : Form
{
public InfoAddForm()
{
InitializeComponent();
StartPosition = FormStartPosition.CenterScreen;
}
private void button2_Click(object sender, EventArgs e)
{
this.Close();
}
private void Button1_Click(object sender, EventArgs e)
{
InfoModel mod = new InfoModel();
mod.bir = this.textBox_bir.Text;
mod.name = this.textBox_xm.Text.ToString();
mod.room = this.textBox_room.Text.ToString();
mod.updatetime = DateTime.Now.ToString();
mod.xb = this.textBox_xb.Text.ToString();
if (string.IsNullOrEmpty(mod.bir) || string.IsNullOrEmpty(mod.name) || string.IsNullOrEmpty(mod.room) || string.IsNullOrEmpty(mod.xb))
{
MessageBox.Show("参数不全");
}
else
{
if (Add(mod))
{
MessageBox.Show("成功");
DialogResult = System.Windows.Forms.DialogResult.OK;
}
else
{
MessageBox.Show("失败");
}
}
}
public Boolean Add(InfoModel info)
{
bool re = false;
try
{
DataTable dt = DataHelpher.GetData(string.Format("select * from c_user where name='{0}'", info.name));
if (dt != null && dt.Rows.Count > 0)
{
MessageBox.Show("该信息已经存在");
re = false;
}
else
{
string sql = string.Format("insert into c_user(name,xb,bir,room,updatetime) values ('{0}','{1}','{2}','{3}','{4}')", info.name, info.xb, info.bir, info.room, info.updatetime);
if (DataHelpher.UpdateAndInsertAndDelete(sql))
{
re = true;
}
else
{
re = false;
}
}
}
catch (Exception ex)
{
Console.WriteLine(ex.Message.ToString());
}
return re;
}
}
}
这样我们的一个简单的信息查询的demo就完成了,为了方便大家的学习,我把demo分享在下面,欢迎各位感兴趣的朋友随时分享下载哈!如有任何问题可及时的联系哈!
链接:https://pan.baidu.com/s/1ERpJ9zIkcndz7Gtml_IIsg
提取码:6dsb
--来自百度网盘超级会员V3的分享
|