package yqq.study;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HColumnDescriptor;
import org.apache.hadoop.hbase.HTableDescriptor;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.HBaseAdmin;
import org.apache.hadoop.hbase.client.HTable;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import java.io.IOException;
public class HBaseDemo {
private HBaseAdmin admin = null;
private HTable table = null;
private String tableName = "phone";
@Before
public void init() throws IOException {
Configuration conf = new Configuration();
conf.set("hbase.zookeeper.quorum","node2,node3,node4");
admin = new HBaseAdmin(conf);
table = new HTable(conf,tableName.getBytes());
}
@After
public void close() throws IOException {
if(table!=null)
table.close();
if(admin!=null)
admin.close();
}
@Test
public void createTable() throws IOException {
HTableDescriptor desc = new HTableDescriptor(TableName.valueOf(tableName));
HColumnDescriptor famliy = new HColumnDescriptor("cf".getBytes());
desc.addFamily(famliy);
if(admin.tableExists(tableName)){
admin.disableTable(tableName);
admin.deleteTable(tableName);
}
admin.createTable(desc);
}
}
hbase(main):002:0> list
TABLE
phone
test
test1
3 row(s) in 0.0370 seconds
=> ["phone", "test", "test1"]
@Test
public void insert() throws InterruptedIOException, RetriesExhaustedWithDetailsException {
Put put = new Put("rk111".getBytes());
put.add("cf".getBytes(),"name".getBytes(),"yqq".getBytes());
put.add("cf".getBytes(),"age".getBytes(),"20".getBytes());
put.add("cf".getBytes(),"sex".getBytes(),"man".getBytes());
table.put(put);
}
hbase(main):003:0> scan "phone"
ROW COLUMN+CELL
rk111 column=cf:age, timestamp=1636971270134, value=20
rk111 column=cf:name, timestamp=1636971270134, value=yqq
rk111 column=cf:sex, timestamp=1636971270134, value=man
1 row(s) in 0.4770 seconds
@Test
public void get() throws IOException {
Get get = new Get("rk111".getBytes());
get.addColumn("cf".getBytes(),"name".getBytes());
get.addColumn("cf".getBytes(),"age".getBytes());
get.addColumn("cf".getBytes(),"sex".getBytes());
Result result = table.get(get);
Cell nameCell = result.getColumnLatestCell("cf".getBytes(),"name".getBytes());
Cell ageCell = result.getColumnLatestCell("cf".getBytes(), "age".getBytes());
Cell sexCell = result.getColumnLatestCell("cf".getBytes(), "sex".getBytes());
System.out.print(Bytes.toString(CellUtil.cloneValue(nameCell))+"\t");
System.out.print(Bytes.toString(CellUtil.cloneValue(ageCell))+"\t");
System.out.print(Bytes.toString(CellUtil.cloneValue(sexCell)));
}
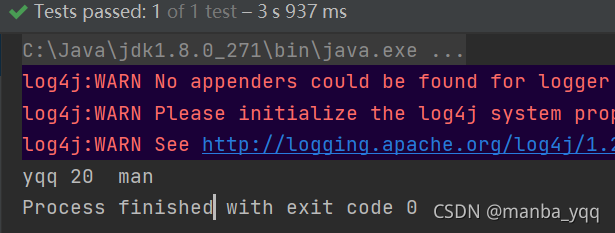
@Test
public void scan() throws IOException {
Scan scan = new Scan();
ResultScanner resultScanner = table.getScanner(scan);
for (Result result:resultScanner){
Cell nameCell = result.getColumnLatestCell("cf".getBytes(),"name".getBytes());
Cell ageCell = result.getColumnLatestCell("cf".getBytes(),"age".getBytes());
Cell sexCell = result.getColumnLatestCell("cf".getBytes(),"sex".getBytes());
System.out.print(Bytes.toString(CellUtil.cloneValue(nameCell))+"\t");
System.out.print(Bytes.toString(CellUtil.cloneValue(ageCell))+"\t");
System.out.println(Bytes.toString(CellUtil.cloneValue(sexCell))+"\t");
}
}
hbase(main):014:0> scan "phone"
ROW COLUMN+CELL
rk111 column=cf:age, timestamp=1636973404628, value=20
rk111 column=cf:name, timestamp=1636973404628, value=yqq
rk111 column=cf:sex, timestamp=1636973404628, value=man
rk112 column=cf:age, timestamp=1636973427738, value=20
rk112 column=cf:name, timestamp=1636973427738, value=wt
rk112 column=cf:sex, timestamp=1636973427738, value=man
rk113 column=cf:age, timestamp=1636973446974, value=20
rk113 column=cf:name, timestamp=1636973446974, value=lwl
rk113 column=cf:sex, timestamp=1636973446974, value=man
rk114 column=cf:age, timestamp=1636973469295, value=20
rk114 column=cf:name, timestamp=1636973469295, value=db
rk114 column=cf:sex, timestamp=1636973469295, value=man
4 row(s) in 0.0840 seconds
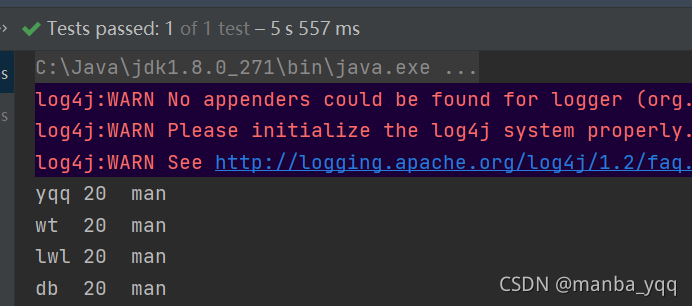
@Test
public void scan() throws IOException {
Scan scan = new Scan();
scan.setStartRow("rk112".getBytes());
scan.setStopRow("rk114".getBytes());
scan.addColumn("cf".getBytes(),"name".getBytes());
scan.addColumn("cf".getBytes(),"age".getBytes());
scan.addColumn("cf".getBytes(),"sex".getBytes());
ResultScanner resultScanner = table.getScanner(scan);
for (Result result:resultScanner){
Cell nameCell = result.getColumnLatestCell("cf".getBytes(),"name".getBytes());
Cell ageCell = result.getColumnLatestCell("cf".getBytes(),"age".getBytes());
Cell sexCell = result.getColumnLatestCell("cf".getBytes(),"sex".getBytes());
System.out.print(Bytes.toString(CellUtil.cloneValue(nameCell))+"\t");
System.out.print(Bytes.toString(CellUtil.cloneValue(ageCell))+"\t");
System.out.println(Bytes.toString(CellUtil.cloneValue(sexCell))+"\t");
}
}
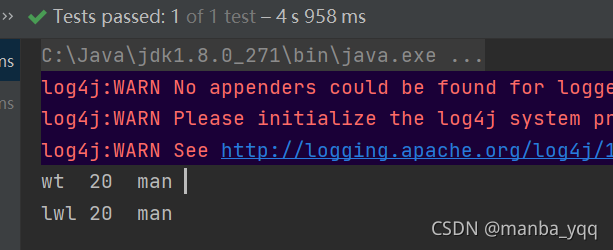 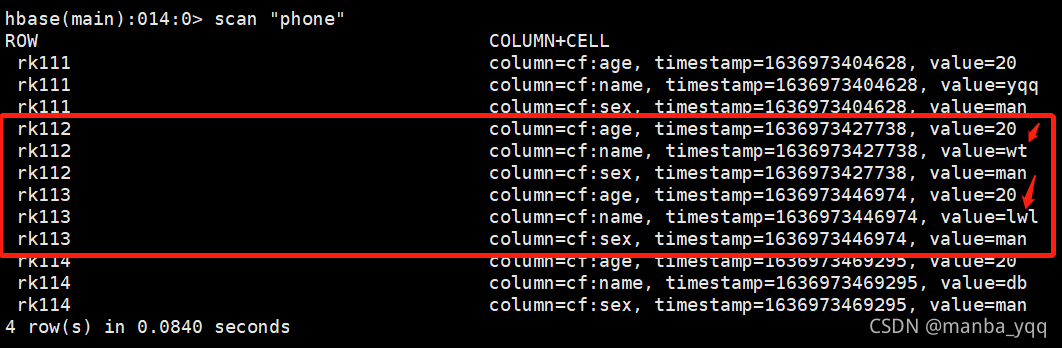
@Test
public void addFamliy() throws IOException {
TableName tableNameObj = TableName.valueOf(tableName);
admin.disableTable(tableNameObj);
HTableDescriptor tableDescriptor = admin.getTableDescriptor(tableNameObj);
HColumnDescriptor famliy = new HColumnDescriptor("cf2".getBytes());
tableDescriptor.addFamily(famliy);
admin.modifyTable(tableName,tableDescriptor);
admin.enableTable(tableNameObj);
}
hbase(main):002:0> describe "phone"
Table phone is ENABLED
phone
COLUMN FAMILIES DESCRIPTION
{NAME => 'cf', BLOOMFILTER => 'ROW', VERSIONS => '1', IN_MEMORY => 'false', KEEP_DELETED_CELLS => 'FALSE', DATA_BLOCK_ENCODING => 'NONE', TTL => 'FOREVER', COMPRESSION =>
'NONE', MIN_VERSIONS => '0', BLOCKCACHE => 'true', BLOCKSIZE => '65536', REPLICATION_SCOPE => '0'}
{NAME => 'cf2', BLOOMFILTER => 'ROW', VERSIONS => '1', IN_MEMORY => 'false', KEEP_DELETED_CELLS => 'FALSE', DATA_BLOCK_ENCODING => 'NONE', TTL => 'FOREVER', COMPRESSION =>
'NONE', MIN_VERSIONS => '0', BLOCKCACHE => 'true', BLOCKSIZE => '65536', REPLICATION_SCOPE => '0'}
2 row(s) in 0.3080 seconds
@Test
public void delete() throws IOException {
Delete delete = new Delete("rk112".getBytes());
delete.deleteColumn("cf2".getBytes(),"phone_num".getBytes());
table.delete(delete);
}
hbase(main):010:0> scan 'phone'
ROW COLUMN+CELL
rk112 column=cf2:phone_num, timestamp=1637029441291, value=18224667889
rk113 column=cf:age, timestamp=1636973446974, value=20
rk113 column=cf:name, timestamp=1636973446974, value=lwl
rk113 column=cf:sex, timestamp=1636973446974, value=man
rk114 column=cf:age, timestamp=1636973469295, value=20
rk114 column=cf:name, timestamp=1636973469295, value=db
rk114 column=cf:sex, timestamp=1636973469295, value=man
3 row(s) in 0.0340 seconds
hbase(main):011:0> scan 'phone'
ROW COLUMN+CELL
rk113 column=cf:age, timestamp=1636973446974, value=20
rk113 column=cf:name, timestamp=1636973446974, value=lwl
rk113 column=cf:sex, timestamp=1636973446974, value=man
rk114 column=cf:age, timestamp=1636973469295, value=20
rk114 column=cf:name, timestamp=1636973469295, value=db
rk114 column=cf:sex, timestamp=1636973469295, value=man
2 row(s) in 0.0450 seconds
|