1. CRUD
- 在SQL中可以使用"
--空格+描述 "来注释说明 CRUD 即增加(create) 、查询(retrieve )、更新(update )、删除(delete )四个单词的首字母缩写。
2. 新增(Create)
先创建一张表:
mysql> create table student(
-> id int,
-> sn int comment'学号',
-> name varchar(20) comment'姓名'
-> );
插入语法:
insert into table_name
[(column [, column]...)]
values (value_list) [,(values_list)]...
values_list:value,[,value]...
2.1 单行数据+全列插入
mysql> insert into student values(100,10000,'唐三藏');
mysql> insert into student values (101,10001,'孙悟空');
2.2 多行数据+指定列插入
insert into student (id,sn,name) values
(102,20001,'曹孟德'),
(103,20002,'孙仲谋');
3. 查询(Retrieve)
语法:
select 查询关键字
[distinct] * |{column [, column]...}去重关键字(一个或多个字段去重)
[from table_name] 从哪张表过滤
[where...] 条件过滤
[order by column [asc| desc],...]根据指定的字段(可以有多个)来排序
limit...分页关键字
mysql> create table exam_result(
-> id int,
-> name varchar(20),
-> chinese decimal(3,1),
-> math decimal(3,1),
-> english decimal(3,1)
-> );
mysql> insert into exam_result (id,name,chinese,math,english) values
-> (1,'唐三藏',67,98,56),
-> (2,'孙悟空',87.5,78,77),
-> (3,'猪悟能',88,98.5,90),
-> (4,'曹孟德',82,84,67),
-> (5,'刘玄德',55.5,85,45),
-> (6,'孙权',70,73,78.5),
-> (7,'宋公明',75,65,30);
3.1 全列查询
select * from exam_result;
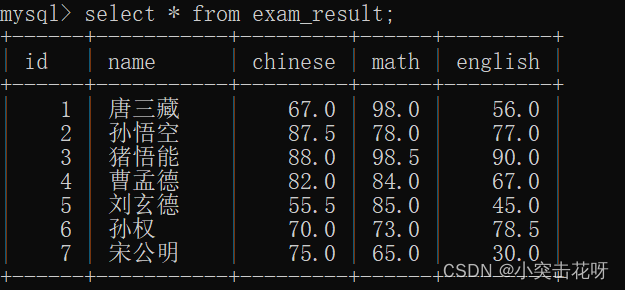
3.2 指定列查询
select id,name,english from exam_result;
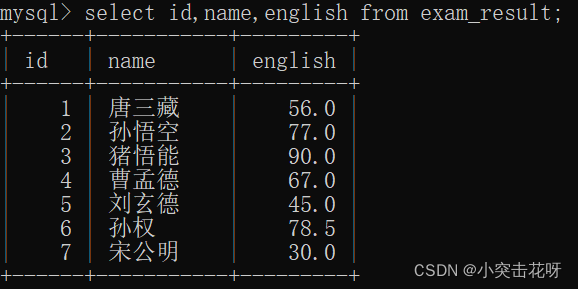
3.3 查询字段为表达式
select id,name,10 from exam_result;
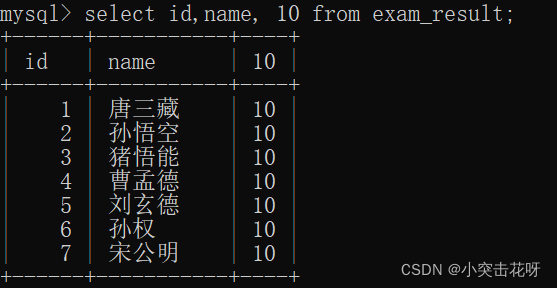
select id,name,english + 10 from exam_result;
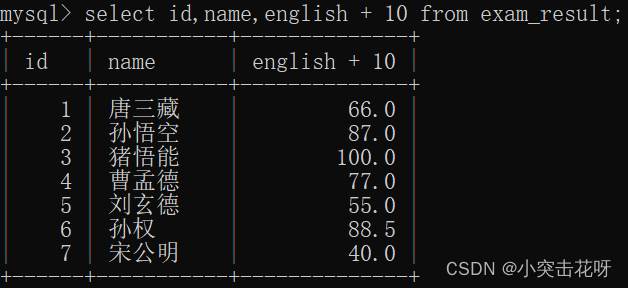
select id,name,chinese + math + english from exam_result;
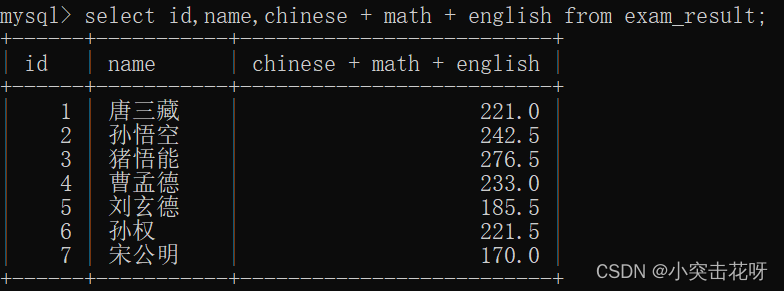 查询字段可以是:
- 表的字段
- 常量值
- 加减乘除表达式
3.4 别名
为查询结果中的列指定别名,表示返回的结果集中,以别名作为改列的名称,语法:
select column [as] alias_name [...] from table_name;
select id,name,chinese+math+english 总分 from exam_result;
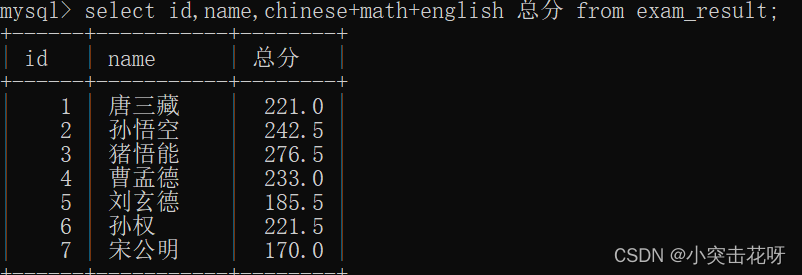
3.5 去重(Distinct)
select math from exam_result;
+
| math |
+
| 98.0 |
| 78.0 |
| 98.0 |
| 84.0 |
| 85.0 |
| 73.0 |
| 65.0 |
+
7 rows in set (0.00 sec)
mysql> select distinct math from exam_result;
+
| math |
+
| 98.0 |
| 78.0 |
| 84.0 |
| 85.0 |
| 73.0 |
| 65.0 |
+
6 rows in set (0.01 sec)
注意:可以跟多个字段,必须每个字段都相同,才会去重。
3.6 排序(ORDER BY)
语法:
select ... from table_name [where ...]
order by column [asc|desc],[...];
- 没有order by子句的查询,返回的顺序是未定义的,永远不要依赖这个顺序
- 没有null数据排序,视为比任何值都小,升序出现在最上面,降序出现在最下面
- 使用表达式及别名排序
select name, chinese+math+english 总分 from exam_result
-> order by 总分 desc;
+
| name | 总分 |
+
| 猪悟能 | 276.0 |
| 孙悟空 | 242.5 |
| 曹孟德 | 233.0 |
| 孙权 | 221.5 |
| 唐三藏 | 221.0 |
| 刘玄德 | 185.5 |
| 宋公明 | 170.0 |
+
7 rows in set (0.00 sec)
- 可以对多个字段进行排序,排序优先级随书写顺序
mysql> select name,math,english,chinese from exam_result
-> order by math desc,english,chinese;
+
| name | math | english | chinese |
+
| 唐三藏 | 98.0 | 56.0 | 67.0 |
| 猪悟能 | 98.0 | 90.0 | 88.0 |
| 刘玄德 | 85.0 | 45.0 | 55.5 |
| 曹孟德 | 84.0 | 67.0 | 82.0 |
| 孙悟空 | 78.0 | 77.0 | 87.5 |
| 孙权 | 73.0 | 78.5 | 70.0 |
| 宋公明 | 65.0 | 30.0 | 75.0 |
+
3.7 条件查询(WHERE)
比较运算符:
运算符 | 说明 |
---|
>,>=,<,<= | 大于,大于等于,小于,小于等于 | = | 等于,NULL 不安全,例如 NULL = NULL 的结果是 NULL | <=> | 等于,NULL 安全,例如 NULL <=> NULL 的结果是 TRUE(1) | !=,<> | 不等于 | between a0 and a1 | 范围匹配,[a0, a1],如果 a0 <= value <= a1,返回 TRUE(1) | in(option,…) | 如果是 option 中的任意一个,返回 TRUE(1) | is null | 是null | is not null | 不是null | like | 模糊匹配。% 表示任意多个(包括 0 个)任意字符;_ 表示任意一个字符 |
注意: 写成x=null或x!=null都不会报错,但是显示空的结果集
逻辑运算符:
运算符 | 说明 |
---|
and | 多个条件必须都为 TRUE(1),结果才是 TRUE(1) | or | 任意一个条件为 TRUE(1), 结果为 TRUE(1) | not | 条件为 TRUE(1),结果为 FALSE(0) |
注意:
- where条件可以使用表达式,但不能使用别名。
- and的优先级高于or,在同时使用时,需要使用小括号()包裹优先执行的部分。
示例:
mysql> select name,english from exam_result where english<60;
+
| name | english |
+
| 唐三藏 | 56.0 |
| 刘玄德 | 45.0 |
| 宋公明 | 30.0 |
+
3 rows in set (0.01 sec)
mysql>
mysql> select name,chinese,english from exam_result where english<chinese;
+
| name | chinese | english |
+
| 唐三藏 | 67.0 | 56.0 |
| 孙悟空 | 87.5 | 77.0 |
| 曹孟德 | 82.0 | 67.0 |
| 刘玄德 | 55.5 | 45.0 |
| 宋公明 | 75.0 | 30.0 |
+
mysql>
-> where chinese+math+english <200;
+
| name | 总分 |
+
| 刘玄德 | 185.5 |
| 宋公明 | 170.0 |
+
mysql> select * from exam_result where chinese>80 and english>80;
+
| id | name | chinese | math | english |
+
| 3 | 猪悟能 | 88.0 | 98.0 | 90.0 |
+
mysql>
mysql> select * from exam_result where chinese >80 or english>80;
+
| id | name | chinese | math | english |
+
| 2 | 孙悟空 | 87.5 | 78.0 | 77.0 |
| 3 | 猪悟能 | 88.0 | 98.0 | 90.0 |
| 4 | 曹孟德 | 82.0 | 84.0 | 67.0 |
+
mysql> select * from exam_result where chinese >80 or math>70 and english>70;
+
| id | name | chinese | math | english |
+
| 2 | 孙悟空 | 87.5 | 78.0 | 77.0 |
| 3 | 猪悟能 | 88.0 | 98.0 | 90.0 |
| 4 | 曹孟德 | 82.0 | 84.0 | 67.0 |
| 6 | 孙权 | 70.0 | 73.0 | 78.5 |
+
mysql> select * from exam_result where (chinese >80 or math>70 ) and english>70;
+
| id | name | chinese | math | english |
+
| 2 | 孙悟空 | 87.5 | 78.0 | 77.0 |
| 3 | 猪悟能 | 88.0 | 98.0 | 90.0 |
| 6 | 孙权 | 70.0 | 73.0 | 78.5 |
+
注意: and优先级比or高,如果需要让or先执行,需要加()
mysql> select name,chinese from exam_result where chinese between 80 and 90;
+
| name | chinese |
+
| 孙悟空 | 87.5 |
| 猪悟能 | 88.0 |
| 曹孟德 | 82.0 |
+
mysql> select name,chinese from exam_result where chinese >=80 and chinese<=90;
+
| name | chinese |
+
| 孙悟空 | 87.5 |
| 猪悟能 | 88.0 |
| 曹孟德 | 82.0 |
+
2.in
mysql> select name,math from exam_result where math in(58,59,98,99);
+
| name | math |
+
| 唐三藏 | 98.0 |
| 猪悟能 | 98.0 |
+
mysql> select name,math from
-> exam_result where math =58 or math=59 or math=98 or math=99;
+
| name | math |
+
| 唐三藏 | 98.0 |
| 猪悟能 | 98.0 |
+
mysql> select name from exam_result where name like '孙%';
+
| name |
+
| 孙悟空 |
| 孙权 |
+
mysql> select name from exam_result where name like '孙_';
+
| name |
+
| 孙权 |
+
SELECT name, qq_mail FROM student WHERE qq_mail IS NOT NULL;
SELECT name, qq_mail FROM student WHERE qq_mail IS NULL;
3.8 分页查询(limit)
语法:
SELECT ... FROM table_name [WHERE ...] [ORDER BY ...] LIMIT n;
SELECT ... FROM table_name [WHERE ...] [ORDER BY ...] LIMIT s, n;
SELECT ... FROM table_name [WHERE ...] [ORDER BY ...] LIMIT n OFFSET s;
示例: 按id进行分页,每页3条记录,分别显示第1,2 ,3页
mysql> select id,name,math,english,chinese from exam_result order by id limit 3 offset 0;
+
| id | name | math | english | chinese |
+
| 1 | 唐三藏 | 98.0 | 56.0 | 67.0 |
| 2 | 孙悟空 | 78.0 | 77.0 | 87.5 |
| 3 | 猪悟能 | 98.0 | 90.0 | 88.0 |
+
mysql> select id,name,math,english,chinese from exam_result order by id limit 3 offset 3;
+
| id | name | math | english | chinese |
+
| 4 | 曹孟德 | 84.0 | 67.0 | 82.0 |
| 5 | 刘玄德 | 85.0 | 45.0 | 55.5 |
| 6 | 孙权 | 73.0 | 78.5 | 70.0 |
+
mysql> select id,name,math,english,chinese from exam_result order by id limit 3 offset 6;
+
| id | name | math | english | chinese |
+
| 7 | 宋公明 | 65.0 | 30.0 | 75.0 |
+
4. 修改(update)
语法:
UPDATE table_name SET column = expr [, column = expr ...]
[WHERE ...] [ORDER BY ...] [LIMIT ...]
示例:
UPDATE exam_result SET math = 80 WHERE name = '孙悟空';
UPDATE exam_result SET math = 60, chinese = 70 WHERE name = '曹孟德';
UPDATE exam_result SET math = math + 30 ORDER BY chinese + math + english LIMIT
3;
UPDATE exam_result SET chinese = chinese * 2;
DELETE FROM table_name [WHERE ...] [ORDER BY ..
5. 删除(delete)
语法:
DELETE FROM table_name [WHERE ...] [ORDER BY ...] [LIMIT...]
示例:
mysql> delete from exam_result where name='孙悟空';
mysql>
mysql> drop table if exists for_delete;
create table for_delete(
-> id int,
-> name varchar(20)
-> );
-> insert into for_delete(name) values ('A'),('B'),('C');
delete from for_delete;
注意: 修改和删除操作都是要非常谨慎的,建议把修改和删除sql转换为查询sql,先看是否是想删除或者修改的,再执行修改或者删除的语句。
|