Hi 大家好 首次见面 我是恰恰!写博客的目的很简单,想记录自己学习编程成为程序媛的心路历程,把自己所学的东西捋顺,假装自己是小老师分享给大家,如果写的不好多多见谅哦,我会慢慢摸索的 这是我的第一篇博客,分享的是仿造360彩票做的双色球彩票网 ,这是一个分布式项目,用的是SSM框架 注册登录模块利用springsecurity实现了用户和管理员登录–权限管理–密码明密文 自己封装了图片验证码和短信验证码等验证码功能 手机号登录用的是阿里云短信 头像上传千牛云 用户数据分析ECharts活跃用户 双色球摇号–帖子发评论–加入购物车 前端布局用了ElementUI、 jequery、vue等
1.搭建项目环境
1.首先是搭建项目环境,搭建SSM框架父子工程–分布式项目–启动zookeeper
2.在数据库里新建user表、authority权限表、user_authority中间表,在项目里有一个sql.txt里有sql语句 在java代码里记得修改自己的数据库连接 账号密码等
3.在pojo里新建User用户、Authority权限表
-
整合SSM的步骤: ① 先实现mybatis框架 ② 实现spring框架 ③ 实现springmvc框架 ④ springmvc整合mybatis ⑤ springmvc整合spirng -
cai_gou_parent:表示父工程,作用聚合所有的子工程,打pom包 cai_gou_common:表示通用的工程,打jar包 cai_gou_pojo:表示javabean,打jar包 cai_gou_dao:表示持久层,数据库实现CRUD cai_gou_interface:表示接口 cai_gou_web:表示controller,控制层 cai_gou_servcie:表示业务逻辑层
由于配置环境变量大多都是粘贴复制那些依赖配置,所以我在这里就不放代码了,我的源代码已经交给gitee管理了,有需要的伙伴可以去clone
引入静态资源到cai_gou_web/webapp下
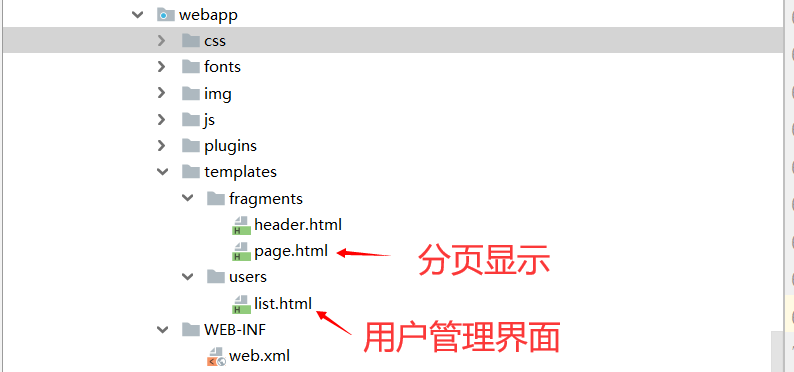
2.后端开发
1.javaBean新建User用户和Authority权限
1.新建User用户类
知识点 public class User implements Serializable {
Serializable作用 1.联网操作必须实现序列化接口 2.储存数据到数据库必须实现序列化接口
public class User implements Serializable {
private Long id;
private String name;
private String email;
private String username;
private String password;
private String avatar;
private String mobile;
private Integer state;
private float money;
private String uid;
private List<Authority> authorities;
public Collection<? extends GrantedAuthority> getAuthorities() {
List<SimpleGrantedAuthority> simpleAuthorities = new ArrayList<>();
for(GrantedAuthority authority : this.authorities){
simpleAuthorities.add(new SimpleGrantedAuthority(authority.getAuthority()));
}
return simpleAuthorities;
}
public void setEncodePassword(String password) {
PasswordEncoder encoder = new BCryptPasswordEncoder();
String encodePasswd = encoder.encode(password);
this.password = encodePasswd;
}
2.新建Authority
知识点 public class Authority implements GrantedAuthority { GrantedAuthority 已授予的权限 1.在Security中,角色和权限共用GrantedAuthority口,唯一的不同角色就是多了个前缀"ROLE_",而且它没有Shiro的那种从属关系 2.在Security提供的UserDetailsService默认实现JdbcDaoImpl中,角色和权限都存储在auhtorities表中
public class Authority implements GrantedAuthority {
private Long id;
private String name;
}
2.创建用户控制器UserController类
1.PageHelper中PageInfo成员属性详解
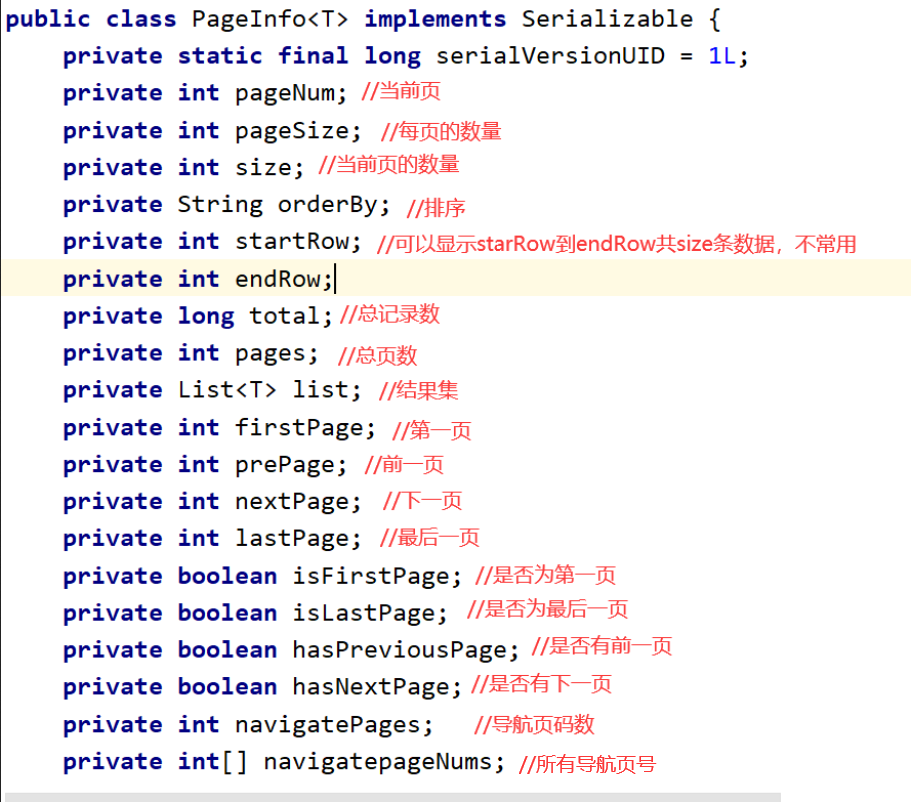
知识点 @RestController SpingBoot采用@RestController在修饰类 @RestController = @Controller + @ResponseBody组成 @Controller 将当前修饰的类注SpringBoot
IOC容器,使得从该类所在的项目跑起来的过程中,这个类就被实例化。当然也有语义化的作用,即代表该类是充当Controller的作用 @ResponseBody 它的作用简短截说就是指该类中所有的API接口返回的数据,甭管你对应的方法返回Map或是其他Object,它会以Json字符串的形式返回给客户端,如果返回的是String类型,则仍然是String。
知识点 1.@RequestMapping(produces = “text/html;charset=UTF-8”)指定响应体返回类型和编码
2.@RequestParam(value=“参数名”,required=“true/false”,defaultValue="") value:参数名,参数叫什么名字 required:是不是必须传,默认是true,改写为false是以防没有参数时它报错 defaultValue:默认值,默认是第一页,默认一页显示多少条数据
defaultValue =“1”,mybatis的插件,必须从第一页开始,不能从第0页开始 Model model 是把后端数据封装传给前端
- 实现分页查询所有数据 分页逻辑
- 分页分为上一页 如果上一页是第一页 不能点了
- 取消上一页的javascript
- 下一页 如果下一页等于总页数,也就是说数据总共有5页,当前页就是5
- 下一页不能点了,因为没有下一页了
- 鼠标点击鼠标悬停页跳转至该页
@RestController
@RequestMapping("/users")
public class UserController {
@Reference
private UserService userService;
@Reference
private AuthorityService authorityService;
@RequestMapping(produces = "text/html;charset=UTF-8")
public ModelAndView list(
@RequestParam(value = "pageNum",required = false,defaultValue ="1")int pageNum,
@RequestParam(value = "pageSize",required = false,defaultValue ="20")int pageSize,
@RequestParam(value = "username",required = false,defaultValue ="")String username,
Model model) {
PageInfo<User>page =userService.listUserByNameLike(username,pageNum,20);
List<User>list=page.getList();
model.addAttribute("page",page);
model.addAttribute("userList",list);
model.addAttribute("username",username);
return new ModelAndView("users/list","userModel",model);
}
}
3.实现分页查询所有数据
1.UserService接口、UserService实现类根据用户名进行分页模糊查询
UserService接口
public interface UserService {
PageInfo<User> listUserByNameLike(String username, int pageNum, int pageSize);
}
UserService实现类
@Service(interfaceClass = UserService.class)
public class UserServiceImpl implements UserService {
@Autowired
private UserDao userDao;
@Override
public PageInfo<User> listUserByNameLike(String username, int pageNum, int pageSize) {
PageHelper.startPage(pageNum, pageSize);
Page<User> page = userDao.findByNameLike(username);
PageInfo<User> userPageInfo = new PageInfo<>(page);
return userPageInfo;
}
}
UserDao接口
public interface UserDao {
Page<User> findByNameLike(String username);
}
2.UserDao.xml分页查询 parameterType:参数类型 resultMap:返回值
<select id="findByNameLike" parameterType="string" resultMap="userMap">
select *from user
<where>
<if test="value!=null and value.length>0">
username like "%"#{value}"%"
</if>
</where>
</select>
3.在userDao.xml 添加级联查询,AuthorityDao.xml做权限查询
级联查询 知识点
resultMap属性
1. <resultMap id="userMap" type="user">
id是resultMap的标识,select语句中引用时用的
type为java实体类,查询返回的数据类型
2.<id column="id" property="id"/>
id一般对应到数据库中该行的id,设置此项可以提升Mybaties性能
3.<result property="avatar" column="avatar"></result>
JavaBean实体类的名字分别对应数据库表中列的名字
4. <collection property="authorities" column="id"
select="com.shiqiaqia.dao.AuthorityDao.findAuthorityListById">
</collection>
collection 复杂类型集合,说明这是一个一对多的关系,多就放在这里
property 接收的是多的多的的属性名字,一般写Java实体类名字
select 多的查询语句的位置
<resultMap id="userMap" type="user">
<id column="id" property="id"></id>
<result property="avatar" column="avatar"></result>
<result property="mobile" column="mobile"></result>
<result property="state" column="state"></result>
<result property="money" column="money"></result>
<result property="uid" column="uid"></result>
<result property="email" column="email"></result>
<result property="name" column="name"></result>
<result property="password" column="password"></result>
<result property="username" column="username"></result>
<collection property="authorities" column="id"
select="com.shiqiaqia.dao.AuthorityDao.findAuthorityListById"></collection>
</resultMap>
AuthorityService 接口
public interface AuthorityService {
List<Authority> findAuthorityListById(Integer userId);
}
AuthorityServiceImpl实现类
@Service(interfaceClass = AuthorityService.class)
public class AuthorityServiceImpl implements AuthorityService {
@Autowired
private AuthorityDao authorityDao;
@Override
public List<Authority> findAuthorityListById(Integer userId) {
List<Authority> authorityListById = authorityDao.findAuthorityListById(userId);
return authorityListById;
}
}
AuthorityDao接口
public interface AuthorityDao {
List<Authority>findAuthorityListById(Integer userId);
}
AuthorityDao.xml
<select id="findAuthorityListById" resultMap="authority" parameterType="int">
select *from authority where id in
(select authority_id from user_authority where user_id=#{userId})
</select>
</mapper>
4.前端增删改查页面list.html
页面展示 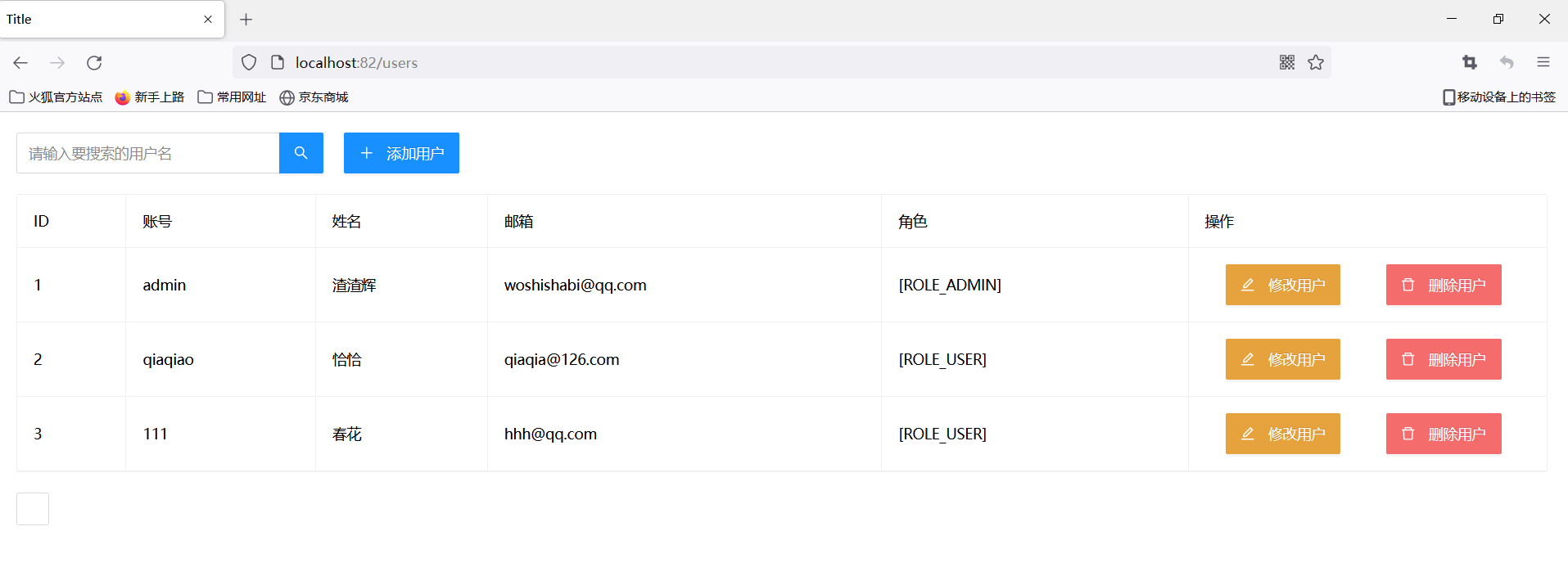
5.根据账号username在搜索框进行搜索数据
在</form></body>中间插入
<script src="/js/jquery.min.js" th:src="@{/js/jquery.min.js}"></script>
<script src="/js/layer/layer.js" th:src="@{/js/layer/layer.js}"></script>
<script src="/js/layer/common.js" th:src="@{/js/layer/common.js}"></script>
<script type="text/javascript">
$(function () {
$("#searchNameBth").click(function () {
document.forms.ec.submit();
});
});
</script>
6.分页显示数据page.html
页面展示
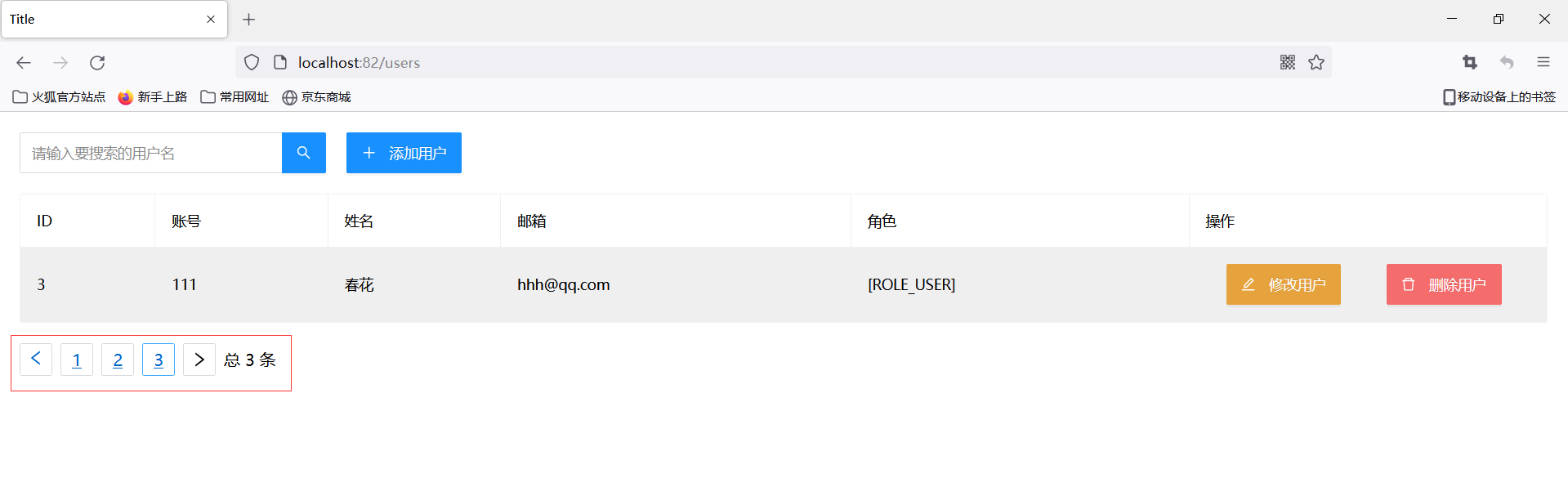
<div data-th-fragment="page">
<input type="hidden" name="pageSize" id="pageSize" th:value="${page.pageSize}">
<input type="hidden" name="pageNum" id="pageNum" th:value="${page.pageNum}">
<ul class="user-pagination">
<li class="user-pagination-prev" th:if="${page.isFirstPage}">
</li>
<li class="user-pagination-prev" th:if="${!page.isFirstPage}">
<a th:href="'javascript:document.forms.ec.pageNum.value='+${page.prePage}+';document.forms.ec.submit();'">
</a>
</li>
<li th:each="i:${page.navigatepageNums}" th:class="${i == page.pageNum} ? 'user-pagination-item user-pagination-item-active':'user-pagination-item'">
<a th:href="'javascript:document:document.forms.ec.pageNum.value='+${i}+';document.forms.ec.submit();'">
<span th:text="${i}"></span></a>
</li>
<li class="user-pagination-next" th:if="${page.isLastPage}">
</li>
<li class="user-pagination-next" th:if="${!page.isLastPage}">
<a th:href="'javascript:document.forms.ec.pageNum.value='+${page.nextPage}+';document.forms.ec.submit();'">
</a>
</li>
<span class="user-pagination-item-total">总 <span id="pageTotal" th:text="${page.total}"></span> 条</span>
</ul>
</div>
源码学习交流自取彩狗网
|