JSON数据格式简答
单个JSON
下面先贴上一个c语言下的json文件的样式。下面的JSON样式很全,既有了子键,而且有了数组,对于初学者相对来说比较全面。这里先注意一下 最开头的中括号 “[]”,下面解析JSON的时候会介绍到。用下面的代码做测试学习的时候,可以将首尾的中括号去掉,理解一下。
const char *message =
"[{ \
\"name\":\"mculover666\", \
\"age\": 22, \
\"weight\": 55.5, \
\"address\": \
{ \
\"country\": \"China\",\
\"zip-code\": 111111\
}, \
\"skill\": [\"c\", \"Java\", \"Python\"],\
\"student\": false \
}] \
";
下面是一段正常的JSON数据,更易查看。
[{
"name":"mculover666",
"age": 22,
"weight": 55.5,
"address":
{
"country": "China",
"zip-code": 111111
},
"skill": ["c", "Java", "Python"],
"student": false
}];
多个JSON
单个JSON和多个JSON块的不同就是需要用 逗号 将各个JSON隔开,并且每个JSON都需要用 {} 隔开。
const char *message =
"[{ \
\"name\":\"mculover666\", \
\"age\": 22, \
\"weight\": 55.5, \
\"address\": \
{ \
\"country\": \"China\",\
\"zip-code\": 111111\
}, \
\"skill\": [\"c\", \"Java\", \"Python\"],\
\"student\": false \
}, \
{ \
\"name\":\"dhn\", \
\"age\": 23, \
\"weight\": 72.6, \
\"address\": \
{ \
\"country\": \"China\",\
\"zip-code\": 222222\
}, \
\"skill\": [\"c\", \"linux\", \"Python\"],\
\"student\": true \
}]";
下面同样贴上正常的JSON样式,便于观看。
[{
"name":"mculover666",
"age": 22,
"weight": 55.5,
"address":
{
"country": "China",
"zip-code": 111111
},
"skill": ["c", "Java", "Python"],
"student": false
},
{
"name":"dhn",
"age": 23,
"weight": 72.6,
"address":
{
"country": "China",
"zip-code": 222222
},
"skill": ["c", "linux", "Python"],
"student": true
}]";
cJSON解析数据及打印显示
JSON数据解析和打印
#include <stdio.h>
#include <iostream>
using namespace std;
#include "../cJson/cJSON.h"
const char *message =
"[{ \
\"name\":\"mculover666\", \
\"age\": 22, \
\"weight\": 55.5, \
\"address\": \
{ \
\"country\": \"China\",\
\"zip-code\": 111111\
}, \
\"skill\": [\"c\", \"Java\", \"Python\"],\
\"student\": false \
}, \
{ \
\"name\":\"dhn\", \
\"age\": 23, \
\"weight\": 72.6, \
\"address\": \
{ \
\"country\": \"China\",\
\"zip-code\": 222222\
}, \
\"skill\": [\"c\", \"linux\", \"Python\"],\
\"student\": true \
}]";
int main(){
cJSON* cjson_data = NULL;
cJSON* cjson_name = NULL;
cJSON* cjson_age = NULL;
cJSON* cjson_weight = NULL;
cJSON* cjson_address = NULL;
cJSON* cjson_address_country = NULL;
cJSON* cjson_address_zipcode = NULL;
cJSON* cjson_skill = NULL;
cJSON* cjson_student = NULL;
int skill_array_size = 0, i = 0;
cJSON* cjson_skill_item = NULL;
cjson_data = cJSON_Parse(message);
if (cjson_data == NULL){
cJSON_Delete(cjson_data);
cout << "parse json fail!" << endl;
return -1;
}
cout << "有格式的方式打印Json:" << endl;
char* without_format_print = cJSON_Print(cjson_data);
cout << without_format_print << endl;
free(without_format_print);
cout << "无格式的方式打印Json:" << endl;
cout << cJSON_PrintUnformatted(cjson_data) << endl;
cjson_name = cJSON_GetObjectItem(cjson_data, "name");
int tint = cJSON_GetArraySize(cjson_data);
cJSON* arr_item = cjson_data->child;
for (int i = 0; i < tint; i++) {
cjson_name = cJSON_GetObjectItem(arr_item, "name");
cjson_age = cJSON_GetObjectItem(arr_item, "age");
cjson_weight = cJSON_GetObjectItem(arr_item, "weight");
cout << cjson_name->valuestring << " " << cjson_age->valueint << " " << cjson_weight->valuedouble << endl;
cjson_address = cJSON_GetObjectItem(arr_item, "address");
cjson_address_country = cJSON_GetObjectItem(cjson_address, "country");
cjson_address_zipcode = cJSON_GetObjectItem(cjson_address, "zip-code");
cout << cjson_address_country->valuestring << " " << cjson_address_zipcode->valueint << endl;
cjson_skill = cJSON_GetObjectItem(arr_item, "skill");
skill_array_size = cJSON_GetArraySize(cjson_skill);
cout << "skill:[";
for (int j = 0; j < skill_array_size; j++){
cjson_skill_item = cJSON_GetArrayItem(cjson_skill, j);
cout << cjson_skill_item->valuestring << ",";
}
cout << "\b]" << endl;
cjson_student = cJSON_GetObjectItem(arr_item, "student");
cout << (cjson_student->valueint == 0 ? "false" : "true") << endl;
arr_item = arr_item->next;
}
cJSON_Delete(cjson_data);
return 0;
}
有格式下的数据打印 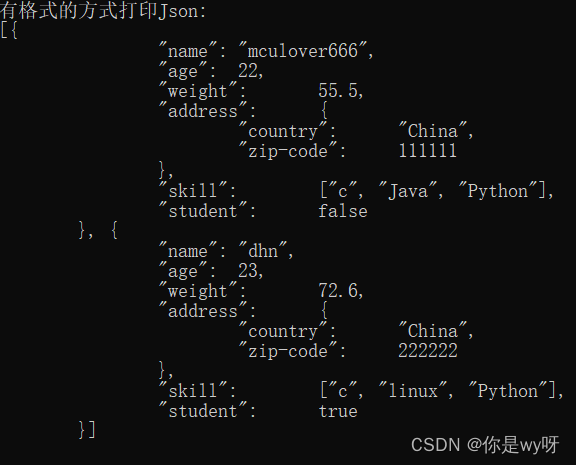 无格式下的数据打印,相比于有格式的打印,此打印方法更加节省空间。 
!!!!!!!,注意:一定要记得释放内存。
上面对中括号的解答。若加了中括号,利用上述代码块的单个JSON数据的解析。 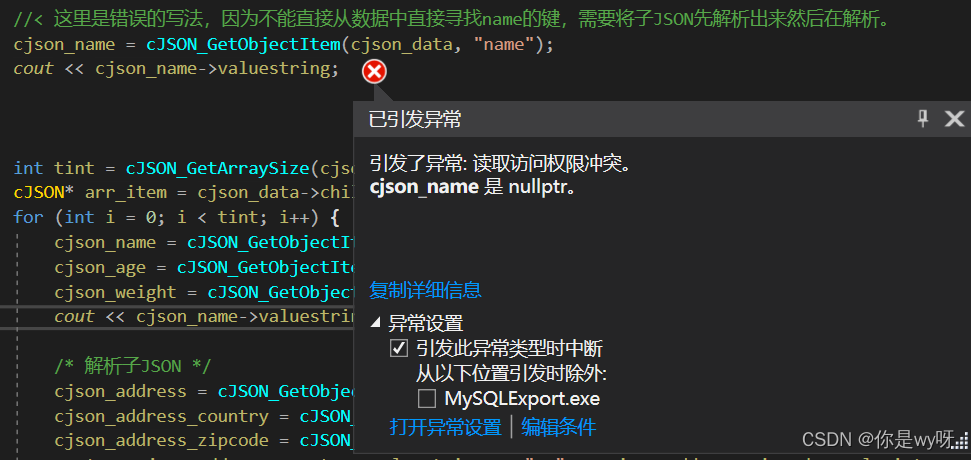 下面是程序完整的运行结果 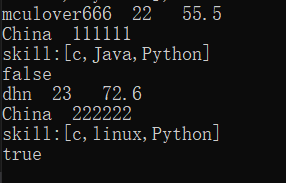
注:以上都是对已知的数据格式进行解析,若不知道文件的数据格式,可以利用cJSON结构体当中的type进行判断, 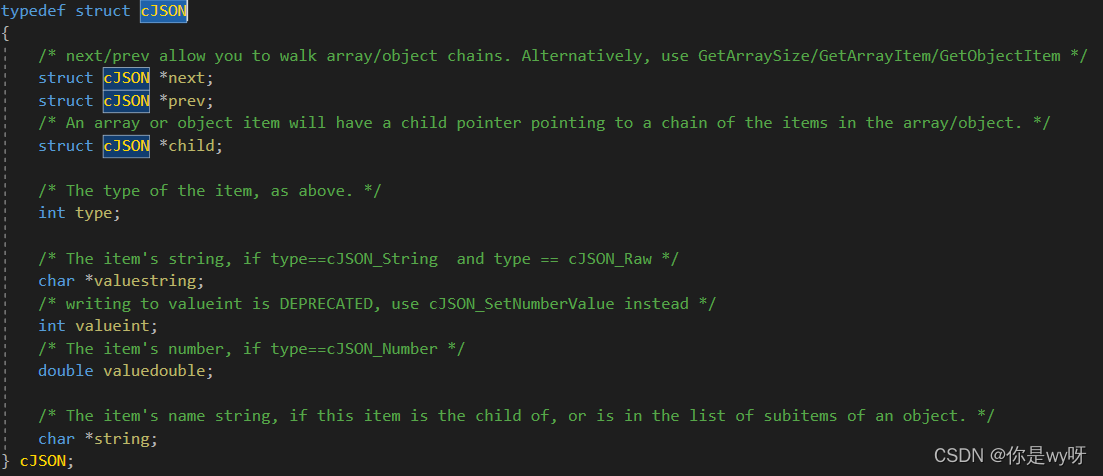 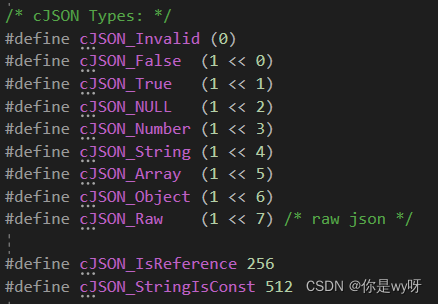
根据判断的类型,在决定数据的格式。
cJSON添加数据
添加数据比较简单,这里直接附上代码了。
#include <stdio.h>
#include "../cJson/cJSON.h"
int main()
{
cJSON* cjson_test = NULL;
cJSON* cjson_address = NULL;
cJSON* cjson_skill = NULL;
char* str = NULL;
cjson_test = cJSON_CreateObject();
cJSON_AddStringToObject(cjson_test, "name", "mculover666");
cJSON_AddNumberToObject(cjson_test, "age", 22);
cJSON_AddNumberToObject(cjson_test, "weight", 55.5);
cjson_address = cJSON_CreateObject();
cJSON_AddStringToObject(cjson_address, "country", "China");
cJSON_AddNumberToObject(cjson_address, "zip-code", 111111);
cJSON_AddItemToObject(cjson_test, "address", cjson_address);
cjson_skill = cJSON_CreateArray();
cJSON_AddItemToArray(cjson_skill, cJSON_CreateString("C"));
cJSON_AddItemToArray(cjson_skill, cJSON_CreateString("Java"));
cJSON_AddItemToArray(cjson_skill, cJSON_CreateString("Python"));
cJSON_AddItemToObject(cjson_test, "skill", cjson_skill);
cJSON_AddFalseToObject(cjson_test, "student");
str = cJSON_Print(cjson_test);
printf("%s\n", str);
cJSON_Delete(cjson_test);
return 0;
}
在挣扎一下:!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!一定要记得释放内存。 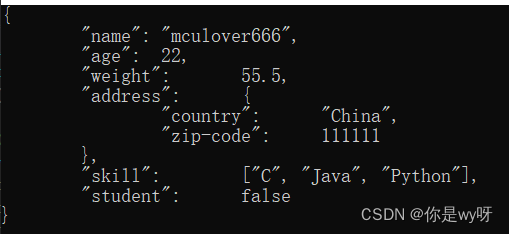
创作不易,若对您有帮助,请不要吝啬您的点赞呦。
|