动态sql
if和foreach if 在自动生成的mapper.xml中的sql
<update id="updateByPrimaryKeySelective" parameterType="com.mjx.model.Book" >
update t_mvc_book
<set >
<if test="bname != null" >
bname = #{bname,jdbcType=VARCHAR},
</if>
<if test="price != null" >
price = #{price,jdbcType=REAL},
</if>
</set>
where bid = #{bid,jdbcType=INTEGER}
</update>
id是方法名字 parameterType对应的实体类 用if来判断是否传进来的对应的字段名 foreach
<select id="selectBooksIn" resultType="com.mjx.model.Book" parameterType="java.util.List">
select * from t_mvc_book where bid in
<foreach collection="bookIds" open="(" close=")" separator="," item="bid">
#{bid}
</foreach>
</select>
collection:集合的变量(相当于遍历里面的items) open 自动补充的前缀 close 自动补充的后缀 separator 分隔符 item 集合变量当前元素的引用(相当于遍历里面的var) 当我们需要查询多条数据时 select * from table where bid in(…) 以前我们是吧数据拼接成字符串再传一个string类型的字符串进来 现在可以直接传入一个数组/集合通过foreach来帮我们进行便利和拼接操作 bookService List selectBooksIn(List bookIds);
bookMapper List selectBooksIn(@Param(“bookIds”)List bookIds);
bookServiceImpl public List selectBooksIn(List bookIds) { return bookMapper.selectBooksIn(bookIds); } 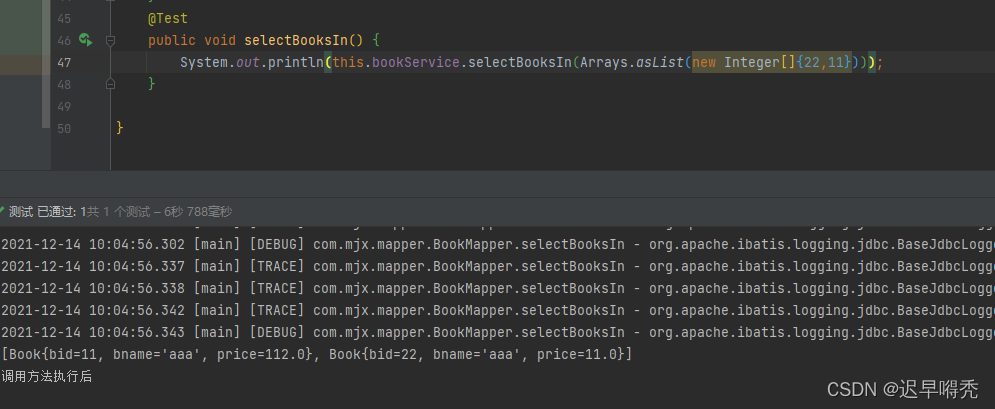 模糊查询
<select id="selectBooksLike1" resultType="com.javaxl.model.Book" parameterType="java.lang.String">
select * from t_mvc_book where bname like #{bname}
</select>
<select id="selectBooksLike2" resultType="com.javaxl.model.Book" parameterType="java.lang.String">
select * from t_mvc_book where bname like '${bname}'
</select>
<select id="selectBooksLike3" resultType="com.javaxl.model.Book" parameterType="java.lang.String">
select * from t_mvc_book where bname like concat(concat('%',#{bname}),'%')
</select>
#{…}:自带引号,模糊查询时%要在后台自己拼接
${…}:有SQL注入的风险,模糊查询时%要在后台自己拼接
concat:通过concat函数自动拼接% 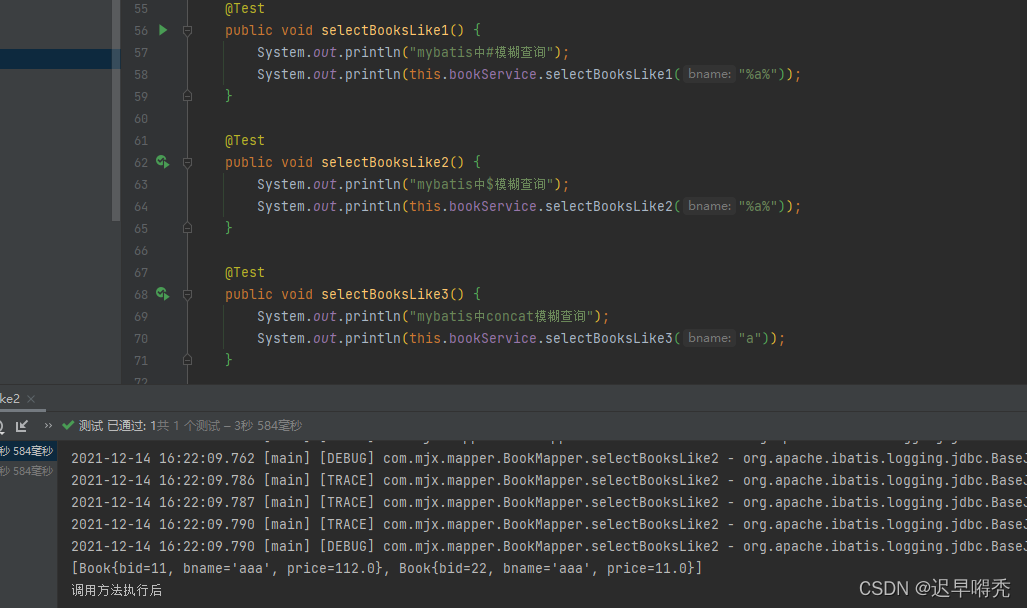
查询返回结果集的处理 bookmapper.xml
<select id="list1" resultMap="BaseResultMap">
select * from t_mvc_book
</select>
<select id="list2" resultType="com.mjx.model.Book">
select * from t_mvc_book
</select>
<select id="list3" resultType="com.mjx.model.Book" parameterType="com.mjx.vo.BookVo">
select * from t_mvc_book where bid in
<foreach collection="bookids" open="(" close=")" separator="," item="bid">
#{bid}
</foreach>
</select>
<select id="list4" resultType="java.util.Map">
select * from t_mvc_book
</select>
<select id="list5" resultType="java.util.Map" parameterType="java.util.Map">
select * from t_mvc_book where bid = #{bid}
</select>
bookmapper
List<Book> list1();
List<Book> list2();
Book list3(BookVo bookVo);
List<Map> list4();
Map list5(Map book);
BookVo
package com.mjx.vo;
import java.util.List;
public class BookVo {
private List<Integer> bookids;
private Float min;
private Float max;
public Float getMin() {
return min;
}
public void setMin(Float min) {
this.min = min;
}
public Float getMax() {
return max;
}
public void setMax(Float max) {
this.max = max;
}
public List<Integer> getBookids() {
return bookids;
}
public void setBookids(List<Integer> bookids) {
this.bookids = bookids;
}
}
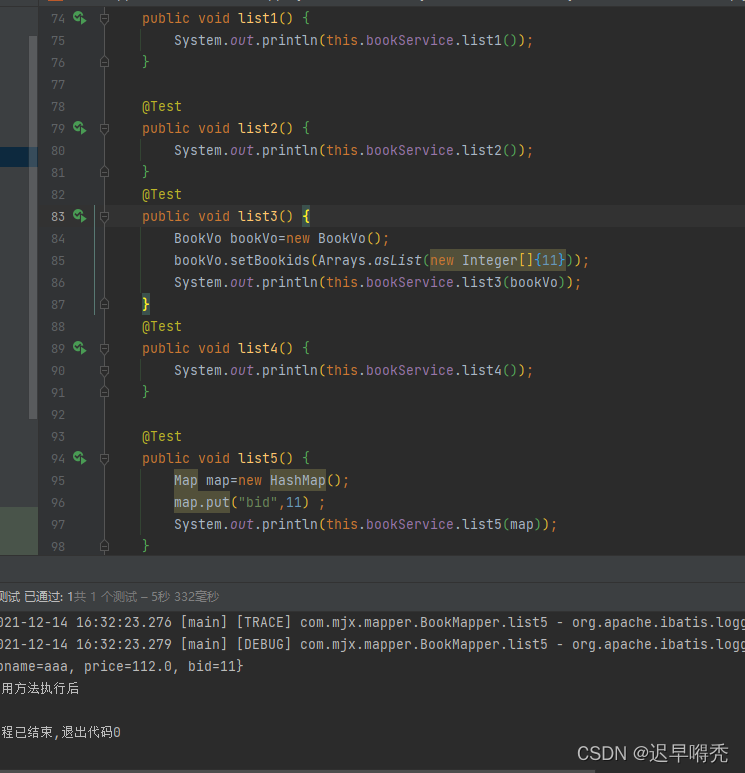 特殊字符 >(>) <(<) &(&) 空格(&) <![CDATA[ <= ]]>
<select id="list6" resultType="com.mjx.model.Book" parameterType="com.mjx.vo.BookVo">
select * from t_mvc_book
<where>
<if test="null != min and min != ''">
<![CDATA[ and #{min} < price ]]>
</if>
<if test="null != max and max != ''">
<![CDATA[ and #{max} > price ]]>
</if>
</where>
</select>
<select id="list7" resultType="com.mjx.model.Book" parameterType="com.mjx.vo.BookVo">
select * from t_mvc_book
<where>
<if test="null != min and min != ''">
and #{min} < price
</if>
<if test="null != max and max != ''">
and #{max} > price
</if>
</where>
</select>
在这里插入图片描述 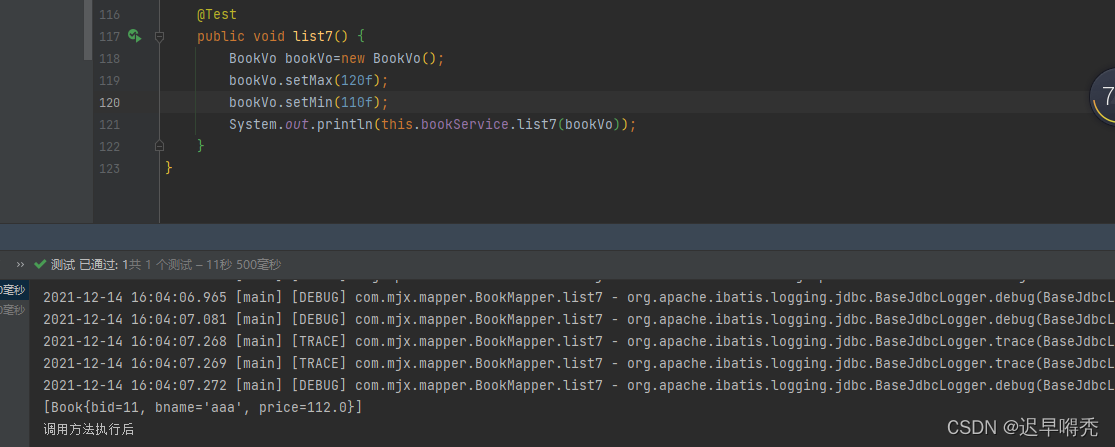
分页
分页查询 为什么要重写mybatis的分页? Mybatis的分页功能很弱,它是基于内存的分页(查出所有记录再按偏移量offset和边界limit取结果),在大数据量的情况下这样的分页基本上是没有用的
使用分页插件步奏 1、导入pom依赖 2、Mybatis.cfg.xml配置拦截器 3、使用PageHelper进行分页 4、处理分页结果 Pom依赖
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
<version>5.1.2</version>
</dependency>
Mybatis.cfg.xml配置拦截器
<plugins>
<plugin interceptor="com.github.pagehelper.PageInterceptor">
</plugin>
</plugins>
bookmapper.xml
<select id="listPager" resultType="java.util.Map" parameterType="java.util.Map">
select * from t_mvc_book where bname like concat(concat('%',#{bname}),'%')
</select>
Mapper层
List<Map> listPager(Map map);
Service层
List<Map> listPager(Map map, PageBean pageBean);
测试
@Override
public List<Map> listPager(Map map, PageBean pageBean) {
if(pageBean != null && pageBean.isPagination()){
PageHelper.startPage(pageBean.getPage(),pageBean.getRows());
}
List<Map> list = bookMapper.listPager(map);
if(pageBean != null && pageBean.isPagination()){
PageInfo pageInfo = new PageInfo(list);
System.out.println("页码:"+pageInfo.getPageNum());
System.out.println("页大小:"+pageInfo.getPageSize());
System.out.println("总记录:"+pageInfo.getTotal());
pageBean.setTotal(pageInfo.getTotal()+"");
}
return list;
}
导入两个帮助类 pagebean
package com.mjx.util;
import java.io.Serializable;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
public class PageBean implements Serializable {
private static final long serialVersionUID = 2422581023658455731L;
private int page=1;
private int rows=10;
private int total=0;
private boolean isPagination=true;
private String url;
private Map<String,String[]> map;
public PageBean() {
super();
}
public void setRequest(HttpServletRequest req) {
String page=req.getParameter("page");
String rows=req.getParameter("rows");
String pagination=req.getParameter("pagination");
this.setPage(page);
this.setRows(rows);
this.setPagination(pagination);
this.url=req.getContextPath()+req.getServletPath();
this.map=req.getParameterMap();
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public Map<String, String[]> getMap() {
return map;
}
public void setMap(Map<String, String[]> map) {
this.map = map;
}
public int getPage() {
return page;
}
public void setPage(int page) {
this.page = page;
}
public void setPage(String page) {
if(null!=page&&!"".equals(page.trim()))
this.page = Integer.parseInt(page);
}
public int getRows() {
return rows;
}
public void setRows(int rows) {
this.rows = rows;
}
public void setRows(String rows) {
if(null!=rows&&!"".equals(rows.trim()))
this.rows = Integer.parseInt(rows);
}
public int getTotal() {
return total;
}
public void setTotal(int total) {
this.total = total;
}
public void setTotal(String total) {
this.total = Integer.parseInt(total);
}
public boolean isPagination() {
return isPagination;
}
public void setPagination(boolean isPagination) {
this.isPagination = isPagination;
}
public void setPagination(String isPagination) {
if(null!=isPagination&&!"".equals(isPagination.trim()))
this.isPagination = Boolean.parseBoolean(isPagination);
}
public int getStartIndex() {
return (this.getPage()-1)*this.rows;
}
public int getMaxPage() {
int totalpage=this.total/this.rows;
if(this.total%this.rows!=0)
totalpage++;
return totalpage;
}
public int getNextPage() {
int nextPage=this.page+1;
if(this.page>=this.getMaxPage())
nextPage=this.getMaxPage();
return nextPage;
}
public int getPreivousPage() {
int previousPage=this.page-1;
if(previousPage<1)
previousPage=1;
return previousPage;
}
@Override
public String toString() {
return "PageBean [page=" + page + ", rows=" + rows + ", total=" + total + ", isPagination=" + isPagination
+ "]";
}
}
StringUtils
package com.mjx.util;
public class StringUtils {
private StringUtils() {
}
public static boolean isBlank(String s) {
boolean b = false;
if (null == s || s.trim().equals("")) {
b = true;
}
return b;
}
public static boolean isNotBlank(String s) {
return !isBlank(s);
}
}
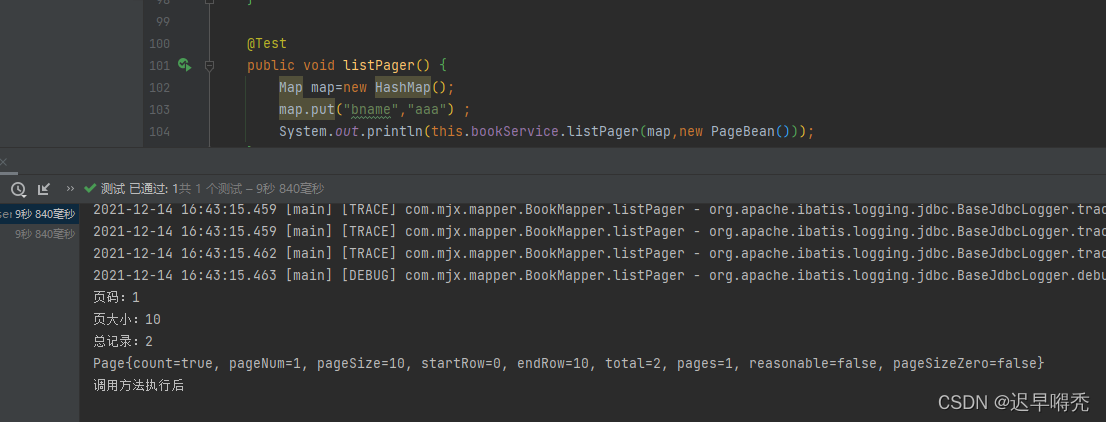
|