1.不会运行直接私信,保姆级教学。 2.功能介绍,实现了管理员与收银员登录,管理员对收银员的增删改查,收银员对顾客的身份查询和商品价格查询。 直接上图 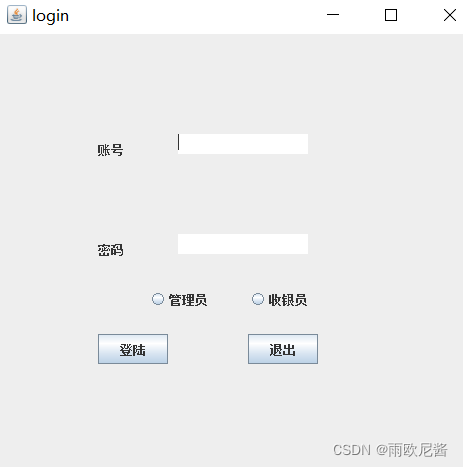
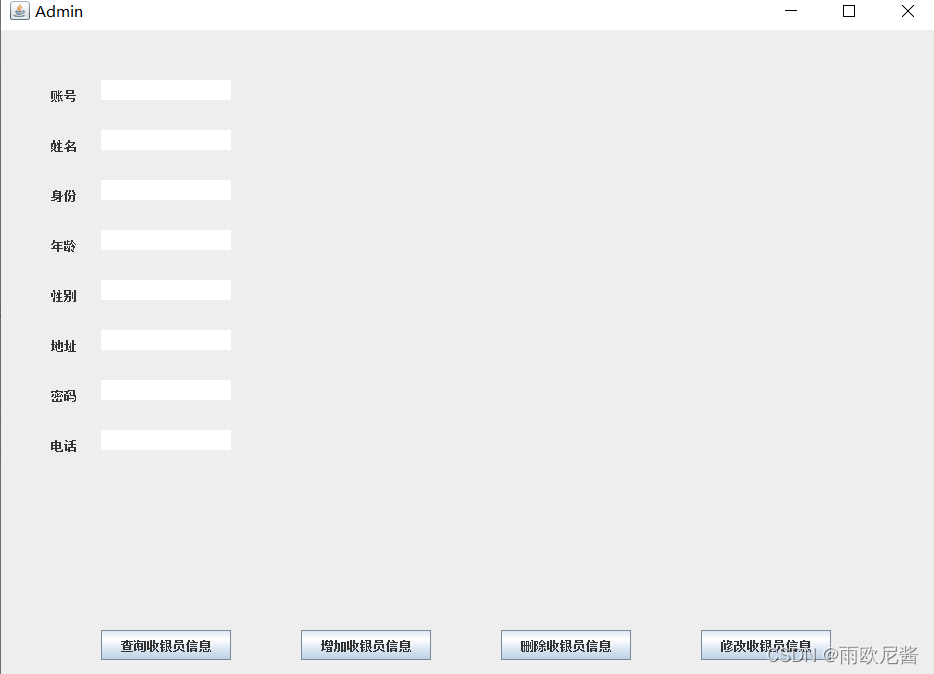 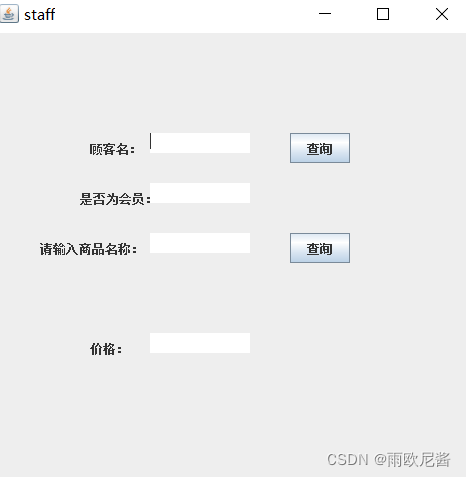 2.1登录页面代码
package com.yz.win;
import com.yz.util.JdbcUtil;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.*;
public class Login {
public Login(){
JFrame jFrame=new JFrame();
jFrame.setTitle("login");
jFrame.setLayout(null);
JButton jButton1=new JButton("登陆");
JButton jButton2 = new JButton("退出");
JLabel jLabel1 = new JLabel("账号");
JLabel jLabel2 = new JLabel("密码");
JLabel jLabel3 = new JLabel("收银员");
JLabel jLabel4 = new JLabel("管理员");
JTextArea jTextArea1 = new JTextArea();
JTextArea jTextArea2 = new JTextArea();
JRadioButton jRadioButton1 = new JRadioButton("管理员");
JRadioButton jRadioButton2 = new JRadioButton("收银员");
jRadioButton1.setSize(80,30);
jRadioButton2.setSize(80,30);
jRadioButton1.setLocation(150,250);
jRadioButton2.setLocation(250,250);
jLabel1.setSize(70,30);
jLabel2.setSize(70,30);
jLabel1.setLocation(100,100);
jLabel2.setLocation(100,200);
jLabel3.setSize(70,30);
jLabel4.setSize(70,30);
jLabel3.setLocation(100,250);
jLabel4.setLocation(300,250);
jButton1.setSize(70,30);
jButton2.setSize(70,30);
jButton1.setLocation(100,300);
jButton2.setLocation(250,300);
jTextArea1.setSize(130,20);
jTextArea1.setLocation(180,100);
jTextArea2.setSize(130,20);
jTextArea2.setLocation(180,200);
jFrame.add(jButton1);
jFrame.add(jButton2);
jFrame.add(jLabel1);
jFrame.add(jLabel2);
jFrame.add(jTextArea1);
jFrame.add(jTextArea2);
jFrame.add(jRadioButton1);
jFrame.add(jRadioButton2);
jFrame.setVisible(true);
jFrame.setSize(500,500);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.setLocation(500,200);
jButton1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String id=jTextArea1.getText().trim();
String password=jTextArea2.getText().trim();
Connection connection=JdbcUtil.getConnection();
String sql="select * from staff where staffNo=?";
PreparedStatement psta=null;
ResultSet res=null;
if (id.equals("") || password.equals("")) {
JOptionPane.showMessageDialog(jFrame, "用户信息不允许为空!");
return;
}
else {
try {
psta=connection.prepareStatement(sql);
psta.setString(1,id);
res=psta.executeQuery();
while (res.next()){
if(password.equals(res.getString(7)))
if(jRadioButton1.isSelected()){
new AdminWin();
jFrame.dispose();
return;
}
else if (jRadioButton2.isSelected()){
new StaffWin();
jFrame.dispose();
return;
}
else {
JOptionPane.showMessageDialog(jFrame,"请选择身份!");
}
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
}
});
jButton2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
jFrame.dispose();
}
});
}
public static void main(String[] args) {
new Login();
}
}
2.2管理员页面代码
package com.yz.win;
import com.yz.util.JdbcUtil;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.*;
public class AdminWin {
public AdminWin(){
JFrame jFrame = new JFrame();
JButton jButton1=new JButton("查询收银员信息");
JButton jButton2 = new JButton("增加收银员信息");
JButton jButton3=new JButton("删除收银员信息");
JButton jButton4 = new JButton("修改收银员信息");
JLabel jLabel1 = new JLabel("账号");
JLabel jLabel2 = new JLabel("姓名");
JLabel jLabel3 = new JLabel("身份");
JLabel jLabel4 = new JLabel("年龄");
JLabel jLabel5 = new JLabel("性别");
JLabel jLabel6 = new JLabel("地址");
JLabel jLabel7 = new JLabel("密码");
JLabel jLabel8 = new JLabel("电话");
JTextArea jTextArea1 = new JTextArea();
JTextArea jTextArea2 = new JTextArea();
JTextArea jTextArea3 = new JTextArea();
JTextArea jTextArea4 = new JTextArea();
JTextArea jTextArea5 = new JTextArea();
JTextArea jTextArea6 = new JTextArea();
JTextArea jTextArea7 = new JTextArea();
JTextArea jTextArea8 = new JTextArea();
jLabel1.setSize(70,30);
jLabel2.setSize(70,30);
jLabel3.setSize(70,30);
jLabel4.setSize(70,30);
jLabel5.setSize(70,30);
jLabel6.setSize(70,30);
jLabel7.setSize(70,30);
jLabel8.setSize(70,30);
jLabel1.setLocation(50,50);
jLabel2.setLocation(50,100);
jLabel3.setLocation(50,150);
jLabel4.setLocation(50,200);
jLabel5.setLocation(50,250);
jLabel6.setLocation(50,300);
jLabel7.setLocation(50,350);
jLabel8.setLocation(50,400);
jTextArea1.setSize(130,20);
jTextArea2.setSize(130,20);
jTextArea3.setSize(130,20);
jTextArea4.setSize(130,20);
jTextArea5.setSize(130,20);
jTextArea6.setSize(130,20);
jTextArea7.setSize(130,20);
jTextArea8.setSize(130,20);
jTextArea1.setLocation(100,50);
jTextArea2.setLocation(100,100);
jTextArea3.setLocation(100,150);
jTextArea4.setLocation(100,200);
jTextArea5.setLocation(100,250);
jTextArea6.setLocation(100,300);
jTextArea7.setLocation(100,350);
jTextArea8.setLocation(100,400);
jButton1.setSize(130,30);
jButton2.setSize(130,30);
jButton3.setSize(130,30);
jButton4.setSize(130,30);
jButton1.setLocation(100,600);
jButton2.setLocation(300,600);
jButton3.setLocation(500,600);
jButton4.setLocation(700,600);
jFrame.add(jButton1);
jFrame.add(jButton2);
jFrame.add(jButton3);
jFrame.add(jButton4);
jFrame.add(jLabel1);
jFrame.add(jLabel2);
jFrame.add(jLabel3);
jFrame.add(jLabel4);
jFrame.add(jLabel5);
jFrame.add(jLabel6);
jFrame.add(jLabel7);
jFrame.add(jLabel8);
jFrame.add(jTextArea1);
jFrame.add(jTextArea2);
jFrame.add(jTextArea3);
jFrame.add(jTextArea4);
jFrame.add(jTextArea5);
jFrame.add(jTextArea6);
jFrame.add(jTextArea7);
jFrame.add(jTextArea8);
jFrame.setTitle("Admin");
jFrame.setLayout(null);
jFrame.setVisible(true);
jFrame.setSize(1000,700);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.setLocation(500,200);
jButton1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Connection con= JdbcUtil.getConnection();
PreparedStatement psta=null;
ResultSet res=null;
String sql="select * from staff where staffNo=?";
String id=jTextArea1.getText().trim();
try {
psta=con.prepareStatement(sql);
psta.setString(1,id);
res=psta.executeQuery();
while (res.next()) {
jTextArea1.setText(res.getString(1));
jTextArea2.setText(res.getString(2));
jTextArea3.setText(res.getString(3));
jTextArea4.setText(res.getString(4));
jTextArea5.setText(res.getString(5));
jTextArea6.setText(res.getString(6));
jTextArea7.setText(res.getString(7));
jTextArea8.setText(res.getString(8));
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
});
jButton3.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Connection con= JdbcUtil.getConnection();
PreparedStatement psta=null;
ResultSet res=null;
String sql="delete from staff where staffNo=?";
String id=jTextArea1.getText().trim();
try {
psta=con.prepareStatement(sql);
psta.setString(1,id);
int n=psta.executeUpdate();
if (n>0){
JOptionPane.showMessageDialog(jFrame,"success!");
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
});
jButton2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Connection con= JdbcUtil.getConnection();
PreparedStatement psta=null;
ResultSet res=null;
String sql="insert into staff values(?,?,?,?,?,?,?,?)";
String id=jTextArea1.getText().trim();
String id1=jTextArea2.getText().trim();
String id2=jTextArea3.getText().trim();
String id3=jTextArea4.getText().trim();
String id4=jTextArea5.getText().trim();
String id5=jTextArea6.getText().trim();
String id6=jTextArea7.getText().trim();
String id7=jTextArea8.getText().trim();
try {
psta=con.prepareStatement(sql);
psta.setString(1,id);
psta.setString(2,id1);
psta.setString(3,id2);
psta.setString(4,id3);
psta.setString(5,id4);
psta.setString(6,id5);
psta.setString(7,id6);
psta.setString(8,id7);
int n=psta.executeUpdate();
if (n>0){
JOptionPane.showMessageDialog(jFrame,"success!");
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
});
jButton4.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Connection con= JdbcUtil.getConnection();
PreparedStatement psta=null;
ResultSet res=null;
String sql="update staff set staffNo=?,staffName=?,position=?,age=?,sex=?,address=?,password=?,phone=? where staffNo=?";
String id=jTextArea1.getText().trim();
String id1=jTextArea2.getText().trim();
String id2=jTextArea3.getText().trim();
String id3=jTextArea4.getText().trim();
String id4=jTextArea5.getText().trim();
String id5=jTextArea6.getText().trim();
String id6=jTextArea7.getText().trim();
String id7=jTextArea8.getText().trim();
try {
psta=con.prepareStatement(sql);
psta.setString(1,id);
psta.setString(2,id1);
psta.setString(3,id2);
psta.setString(4,id3);
psta.setString(5,id4);
psta.setString(6,id5);
psta.setString(7,id6);
psta.setString(8,id7);
psta.setString(9,id);
int n=psta.executeUpdate();
if (n>0){
JOptionPane.showMessageDialog(jFrame,"success!");
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
});
}
}
2.3收银员页面代码
package com.yz.win;
import com.yz.util.JdbcUtil;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.*;
public class StaffWin {
public StaffWin(){
JFrame jFrame = new JFrame();
jFrame.setTitle("staff");
JButton jButton = new JButton("查询");
JButton jButton1 = new JButton("查询");
JLabel jLabel1 = new JLabel("请输入商品名称:");
JLabel jLabel2 = new JLabel("价格:");
JLabel jLabel4 = new JLabel("顾客名:");
JLabel jLabel5 = new JLabel("是否为会员:");
JTextArea jTextArea1 = new JTextArea();
JTextArea jTextArea2 = new JTextArea();
JTextArea jTextArea4 = new JTextArea();
JTextArea jTextArea5 = new JTextArea();
jButton.setSize(60,30);
jButton.setLocation(300,200);
jButton1.setSize(60,30);
jButton1.setLocation(300,100);
jTextArea1.setSize(100,20);
jTextArea1.setLocation(160,200);
jTextArea2.setSize(100,20);
jTextArea2.setLocation(160,300);
jTextArea4.setLocation(160,100);
jTextArea4.setSize(100,20);
jTextArea5.setLocation(160,150);
jTextArea5.setSize(100,20);
jLabel1.setSize(120,30);
jLabel2.setSize(70,30);
jLabel1.setLocation(50,200);
jLabel2.setLocation(100,300);
jLabel4.setSize(70,30);
jLabel4.setLocation(100,100);
jLabel5.setSize(90,30);
jLabel5.setLocation(90,150);
jFrame.add(jLabel5);
jFrame.add(jTextArea5);
jFrame.add(jButton1);
jFrame.add(jTextArea4);
jFrame.add(jLabel4);
jFrame.add(jButton);
jFrame.add(jTextArea1);
jFrame.add(jTextArea2);
jFrame.add(jLabel1);
jFrame.add(jLabel2);
jFrame.setLayout(null);
jFrame.setVisible(true);
jFrame.setSize(500,500);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.setLocation(500,200);
jButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String id=jTextArea1.getText().trim();
String password=jTextArea2.getText().trim();
Connection connection= JdbcUtil.getConnection();
String sql="select * from commodity where commodityName=?";
PreparedStatement psta=null;
ResultSet res=null;
try {
psta=connection.prepareStatement(sql);
psta.setString(1,id);
res=psta.executeQuery();
while (res.next()){
if (jTextArea5.getText().equals("会员")){
jTextArea2.setText(res.getString(5));
}
else {
jTextArea2.setText(res.getString(4));
}
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
});
jButton1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String id=jTextArea4.getText().trim();
String password=jTextArea2.getText().trim();
Connection connection= JdbcUtil.getConnection();
String sql="select * from member where memberName=?";
PreparedStatement psta=null;
ResultSet res=null;
try {
psta=connection.prepareStatement(sql);
psta.setString(1,id);
res=psta.executeQuery();
while (res.next()){
jTextArea5.setText(res.getString(3));
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
});
}
}
2.4,连接数据库的工具类
package com.yz.util;
import java.sql.*;
public class JdbcUtil {
private static String driver= "com.mysql.jdbc.Driver";
private static String url= "jdbc:mysql://127.0.0.1:3306/shopping?useUnicode=true&characterEncoding=UTF-8";
private static String user= "root";
private static String password= "123456";
static{
try {
Class.forName(driver);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public static Connection getConnection(){
try {
return DriverManager.getConnection(url, user, password);
} catch (SQLException e) {
e.printStackTrace();
return null;
}
}
public static void close(Connection con,Statement sta,ResultSet res){
if(res!=null){
try {
res.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if(sta!=null){
try {
sta.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if(con!=null){
try {
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public static void close(Connection con,Statement sta){
close(con, sta, null);
}
public static void close(Connection con,PreparedStatement sta,ResultSet res){
close(con, (Statement)sta, res);
}
public static void close(Connection con,PreparedStatement sta){
close(con, (Statement)sta, null);
}
public static void main(String[] args) {
System.out.println(JdbcUtil.getConnection());
}
}
2.5数据库代码
;
;
;
;
;
;
CREATE DATABASE `shopping` ;
USE `shopping`;
DROP TABLE IF EXISTS `commodity`;
CREATE TABLE `commodity` (
`commodityNo` varchar(20) NOT NULL,
`commodityName` varchar(60) NOT NULL,
`purchaseprice` varchar(30) NOT NULL,
`saleprice` varchar(30) NOT NULL,
`memberprice` varchar(30) NOT NULL,
`stock` varchar(20) NOT NULL,
PRIMARY KEY (`commodityNo`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
insert into `commodity`(`commodityNo`,`commodityName`,`purchaseprice`,`saleprice`,`memberprice`,`stock`) values ('211089','涔愪簨钖墖','2','8','6.6','300'),('211345','鍗緳澶ц荆妫?,'1.5','4','3.2','500'),('211890','寰风宸у厠鍔?,'16','35','29.5','500'),('266456','浜哄瓧鎷?,'6','25','22.8','600'),('533908','娲楁磥绮?,'8','38','33.6','780'),('677758','鐫¤。','35','89','78.5','370');
DROP TABLE IF EXISTS `member`;
CREATE TABLE `member` (
`memberNo` varchar(30) NOT NULL,
`memberName` varchar(50) NOT NULL,
`cardNo` varchar(30) NOT NULL,
`age` varchar(20) DEFAULT NULL,
`sex` varchar(20) DEFAULT NULL,
`address` varchar(60) DEFAULT NULL,
`phone` varchar(40) NOT NULL,
PRIMARY KEY (`memberNo`,`cardNo`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
insert into `member`(`memberNo`,`memberName`,`cardNo`,`age`,`sex`,`address`,`phone`) values ('266789','瀛欐倓鎮?,'546789','20',NULL,NULL,'2615467465'),('322568','鐜嬫櫠鏅?,'344567','23','濂?,'鍖楁箹灏忓尯','1346785786'),('455787','榫欎含鑵?,'244356',NULL,NULL,NULL,'1456783456'),('456230','闊︽嫑璐?,'453266','59','鐢?,'缈绘枟鑺卞洯','3451234789'),('456900','鏋楀瓩绉?,'456436',NULL,NULL,NULL,'3565778543');
DROP TABLE IF EXISTS `staff`;
CREATE TABLE `staff` (
`staffNo` varchar(20) NOT NULL,
`staffName` varchar(50) NOT NULL,
`position` varchar(50) NOT NULL,
`age` varchar(10) DEFAULT NULL,
`sex` varchar(20) DEFAULT NULL,
`address` varchar(60) DEFAULT NULL,
`password` varchar(60) NOT NULL,
`phone` varchar(30) DEFAULT NULL,
PRIMARY KEY (`staffNo`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
insert into `staff`(`staffNo`,`staffName`,`position`,`age`,`sex`,`address`,`password`,`phone`) values ('145890','鑻忓崡','鏀堕摱鍛?,'20','濂?,'鎰忎箟灏忓尯','345678','1453455590'),('189654','寮犲紶','鏀堕摱鍛?,'27','鐢?,NULL,'456789','1786897564'),('189675','鐜嬩紵','鏀堕摱鍛?,'19','鐢?,NULL,'567890','1896543897'),('564890','瀛欎咕绉?,'绠$悊鍛?,'34','鐢?,'鍓嶆櫙灏忓尯','234567','1674567907'),('567345','寮犲墠杩?,'绠$悊鍛?,'46','鐢?,'缈绘枟鑺卞洯','123456','1567894567'),('786453','璁告','鏀堕摱鍛?,'25','濂?,'閲戞鑺卞洯','012345','3467564474');
;
;
;
;
|