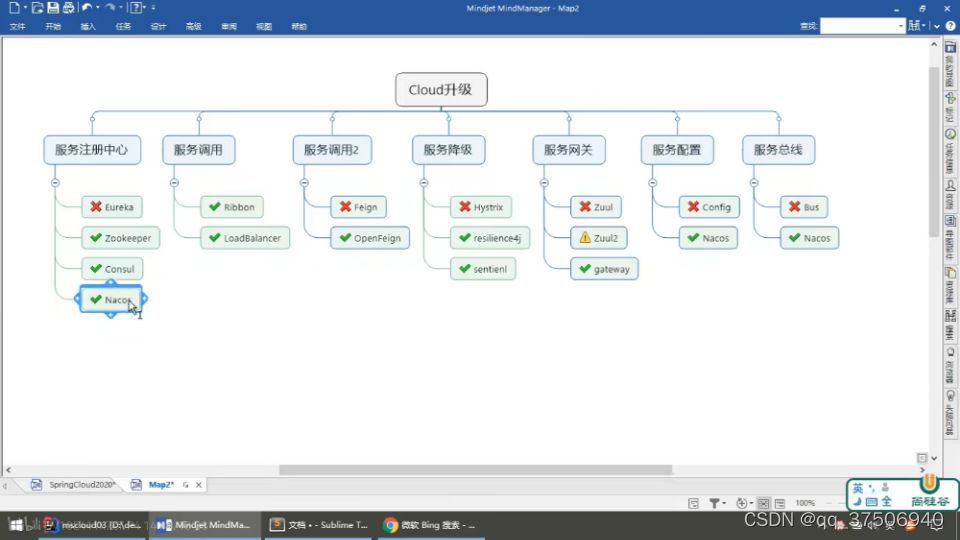
首先,要注意spring boot和spring cloud的版本匹配 微服务模块:
- 见模块
- 改POM
- 写YML
- 主启动
- 业务类
Eureka
1.服务提供端要向eureka提供心跳 2.分为eureka client和eureka server eureka client:对eureka进行注册 eureka server:向服务端提供注册服务 3.集群:集群中的每个eureka都要注册其他的eureka,也就是相互注册
搭建Eureka集群
包括eureka7001,eureka7002,cloud-provider-payment8001,cloud-provider-payment8002,cloud-consumer-order80 其中: payment端口提供服务,而order端口使用服务
eureka集群中的每个eureka都要在defaultZone中指向其他的eureka
eureka7001:
使用@EnableEurekaServer  yml文件如下:
server:
port: 7001
eureka:
instance:
hostname: eureka7001.com
client:
register-with-eureka: false
fetch-registry: false
service-url:
defaultZone: http://localhost:7002/eureka/
spring:
datasource:
url: jdbc:mysql://localhost:3306/db2019?useUnicode=true&characterEncoding=utf-8&useSSL=false
username: root
password: root
driver-class-name: com.mysql.jdbc.Driver
payment8001:
使用@EnableEurekaClient 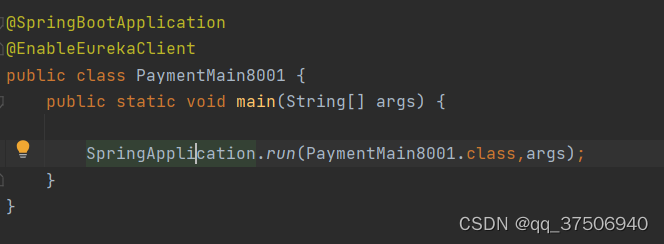 yaml文件:
server:
port: 8001
spring:
application:
name: cloud-payment-service
datasource:
url: jdbc:mysql://localhost:3306/db2019?useUnicode=true&characterEncoding=utf-8&useSSL=false
username: root
password: root
driver-class-name: com.mysql.jdbc.Driver
mybatis:
mapperLocations: classpath:mapper/*.xml
type-aliases-package: com.atguigu.springcloud.entities
eureka:
client:
register-with-eureka: true
fetchRegistry: true
service-url:
defaultZone: http://localhost:7001/eureka,http://localhost:7002/eureka
order80:
使用@EnableEurekaClient  yml文件:
server:
port: 80
spring:
application:
name: cloud-order-service
eureka:
client:
register-with-eureka: true
fetchRegistry: true
service-url:
defaultZone: http://localhost:7001/eureka,http://localhost:7002/eureka
注册restTemplate
@Configuration
public class ApplicationContextConfig {
@Bean
@LoadBalanced
public RestTemplate getRestTemplate(){
return new RestTemplate();
}
}
Controller:
@RestController
@Slf4j
public class OrderController {
public static final String PAYMENT_URL = "http://CLOUD-PAYMENT-SERVICE";
@Autowired
private RestTemplate restTemplate;
@GetMapping("/consumer/payment/create")
public CommonResult<Payment> create(Payment payment){
return restTemplate.postForObject(PAYMENT_URL+"/payment/create",payment,CommonResult.class);
}
@GetMapping("/consumer/payment/get/{id}")
public CommonResult<Payment> getPayment(@PathVariable("id") Long id){
return restTemplate.getForObject(PAYMENT_URL+"/payment/get/"+id,CommonResult.class);
}
}
|