首先,在本地搭建好mysql 5.6环境,将数据库的root用户密码改为root,创建godb数据库,在godb中执行以下SQL语句生成两个数据表:
SET FOREIGN_KEY_CHECKS=0;
DROP TABLE IF EXISTS `post`;
CREATE TABLE `post` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) DEFAULT NULL,
`title` varchar(60) COLLATE utf8_unicode_ci DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
DROP TABLE IF EXISTS `user`;
CREATE TABLE `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(100) COLLATE utf8_unicode_ci NOT NULL DEFAULT '',
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
DROP TABLE IF EXISTS `users`;
CREATE TABLE `users` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(60) COLLATE utf8_unicode_ci DEFAULT NULL,
`post_count` int(11) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
INSERT INTO `users` VALUES ('1', 'qqqqqq', '0');
第二步:创建一个beego项目,结构如下:
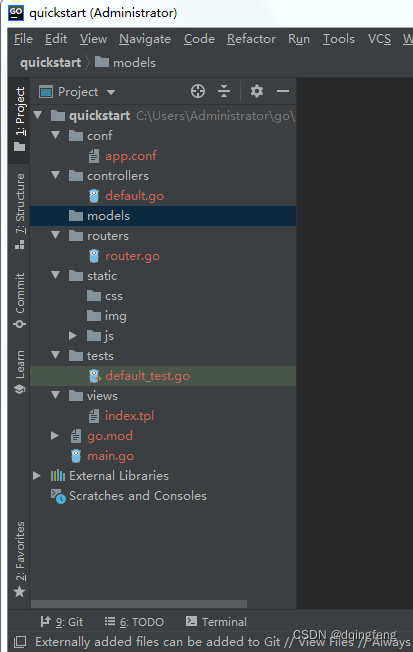
第三步:替换default.go代码:
package controllers
import (
"fmt"
"github.com/astaxie/beego"
"github.com/astaxie/beego/orm"
_ "github.com/go-sql-driver/mysql"
"quickstart/models"
)
type MainController struct {
beego.Controller
}
func (c *MainController) Get() {
c.Data["Website"] = "beego.me"
c.Data["Email"] = "astaxie@gmail.com"
c.TplName = "index.tpl"
}
func (c *MainController) TestOrm() {
o := orm.NewOrm()
user := models.User{Id: 1}
post := models.Post{Title: "北京来的",User: &user}
o.Begin()
res, err1 := o.Raw("UPDATE users SET post_count = post_count + 1 where id = 1").Exec()
var num11 int64 = 0
if err1 == nil {
num1, _ := res.RowsAffected()
num11 = num1
fmt.Println("mysql row affected nums: ", num11)
}
id, err := o.Insert(&post)
if err == nil && id > 0 && num11 > 0 {
o.Commit()
fmt.Println("事务处理成功")
} else {
o.Rollback()
fmt.Println("事务处理失败")
}
// delete
//num, err = o.Delete(&u)
//fmt.Printf("NUM: %d, ERR: %v\n", num, err)
c.Data["Website"] = "beego.me"
c.Data["Email"] = "astaxie@gmail.com"
c.TplName = "index.tpl"
}
第四步:替换models.go的代码:
package models
import "github.com/astaxie/beego/orm"
type User struct {
Id int
Name string `orm:"size(100)"`
}
type Post struct {
Id int `orm:"auto"`
Title string `orm:"size(100)"`
User *User `orm:"rel(fk)"`
}
func init() {
// set default database
orm.RegisterDataBase("default", "mysql", "root:root@tcp(127.0.0.1:3306)/godb?charset=utf8", 30)
// register model
orm.RegisterModel(new(User),new(Post))
// create table
orm.RunSyncdb("default", false, true)
}
第五步,routs.go添加路由后代码如下:
package routers
import (
"github.com/astaxie/beego"
"quickstart/controllers"
)
func init() {
beego.Router("/", &controllers.MainController{})
beego.Router("/orm", &controllers.MainController{},"*:TestOrm")
}
OK,到Terminal面板执行命令:
bee run
最后如果出现如下图,则表示服务器已成功运行:

?在浏览器输入:
http://localhost:8080/orm
此时出现界面
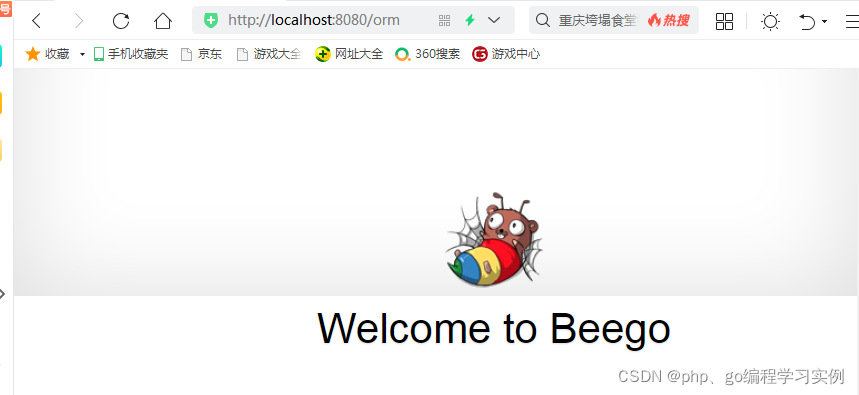
?此时数据库的两个表的数据应该类似如下图,即事务处理成功
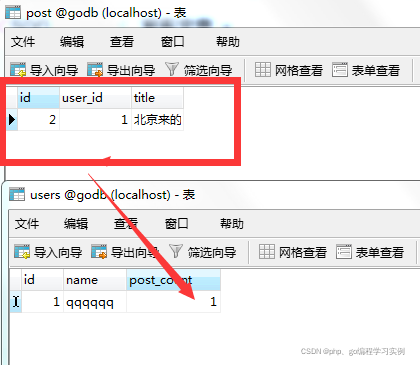
|