FactoryBean是什么东西
官方文档解释:
* Interface to be implemented by objects used within a {@link BeanFactory} which
* are themselves factories for individual objects. If a bean implements this
* interface, it is used as a factory for an object to expose, not directly as a
* bean instance that will be exposed itself.
大概的意思就是:实现FactoryBean接口的对象就会成为工厂,这些工厂可以生产特定的对象。如果一个Bean实现了这个接口,它就会被作为一个生产对象工厂暴露在Srping容器中,而不会直接暴露它可以生产的对象。 举个例子,上代码: 我们有一个Dog:
public class Dog {
private String name;
private int age;
public Dog() { }
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return "Dog{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
有一个实现了FactoryBean接口的DogFactoryBean:
import org.springframework.beans.factory.FactoryBean;
import org.springframework.stereotype.Component;
@Component
public class DogFactoryBean implements FactoryBean<Dog> {
public Dog getObject() throws Exception {
return new Dog();
}
public Class<?> getObjectType() {
return Dog.class;
}
public boolean isSingleton() {
return true;
}
}
配置类:
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
@Configuration
@ComponentScan
public class MyConfig {
}
在Test文件中打印Spring容器中的值,以及尝试用String和class取出容器中的Dog对象:
public static void main(String[] args) throws Exception {
ApplicationContext context = new AnnotationConfigApplicationContext(MyConfig.class);
System.out.println(Arrays.toString(context.getBeanDefinitionNames()).replaceAll(",","\n"));
System.out.println(context.getBean("dogFactoryBean"));
System.out.println(context.getBean("&dogFactoryBean"));
System.out.println(context.getBean(DogFactoryBean.class));
System.out.println(context.getBean(DogFactoryBean.class).getObject());
}
结果如下:
[org.springframework.context.annotation.internalConfigurationAnnotationProcessor
org.springframework.context.annotation.internalAutowiredAnnotationProcessor
org.springframework.context.annotation.internalCommonAnnotationProcessor
org.springframework.context.event.internalEventListenerProcessor
org.springframework.context.event.internalEventListenerFactory
myConfig
dogFactoryBean]
Dog{name='null', age=0}
register.factorybean.DogFactoryBean@429bd883
register.factorybean.DogFactoryBean@429bd883
Dog{name='null', age=0}
可以看到,Srping容器中并没有dog,只有dogFacotoryBean,当我们直接通过context.getBean(“dogFactoryBean”)方法直接取出的却是dog。这就是DogFactoryBean会被作为一个对象工厂暴露在Srping容器中,而不会直接暴露它可以生产的对象dog。 但是通过context.getBean(DogFactoryBean.class)和context.getBean("&dogFactoryBean")的方法,我们又会取出DogFactoryBean,通过DogFactoryBean.getObject方法就会取出dog对象。 关系图如下: 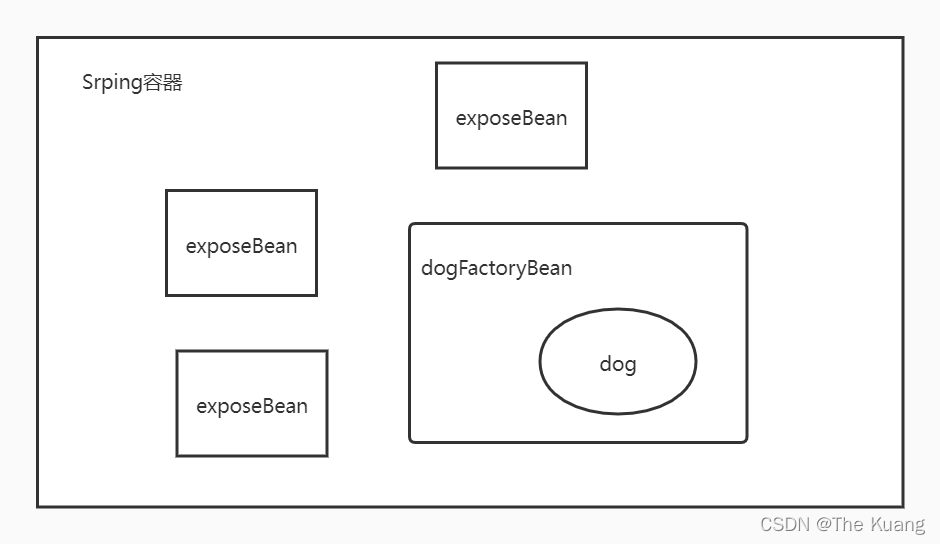
|