1、MySQL安装
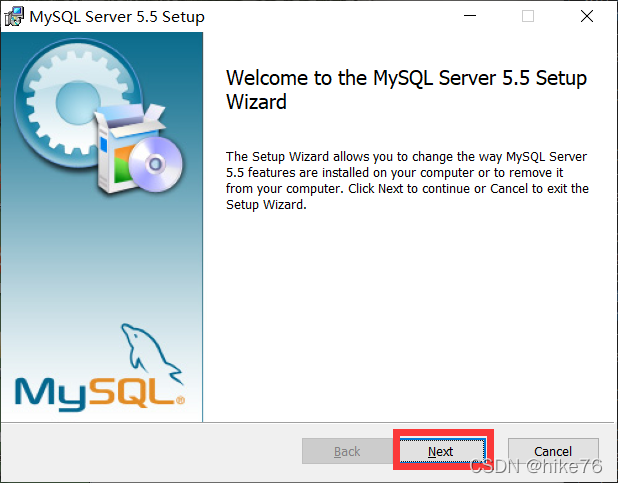
?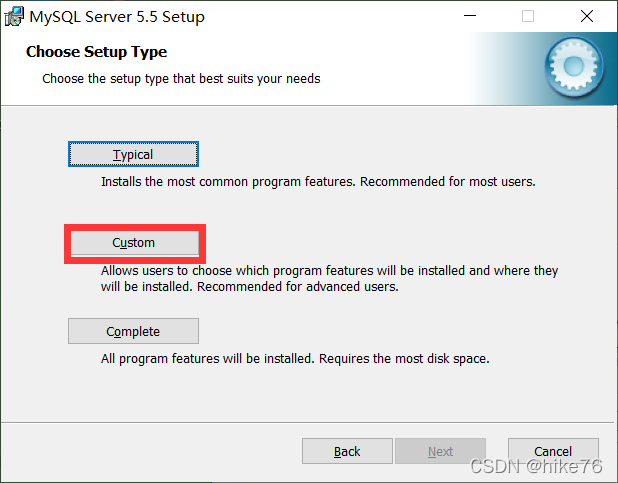
?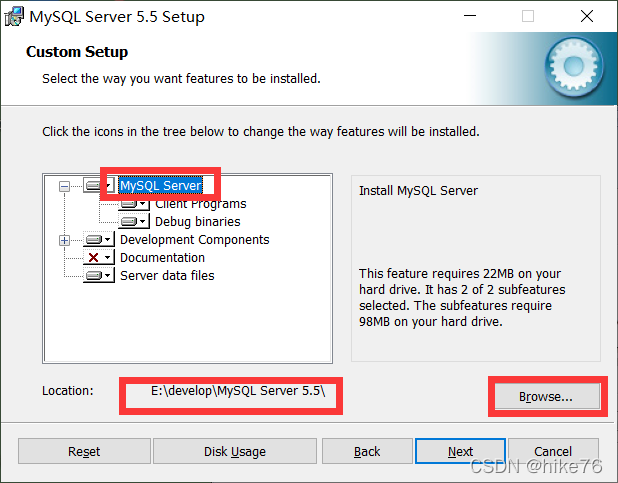
修改文件的安装目录,注意修改后MySQL与Server data files目录一致。
?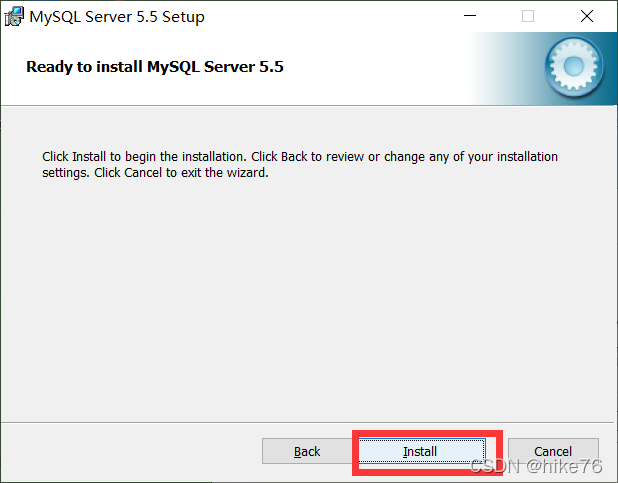
?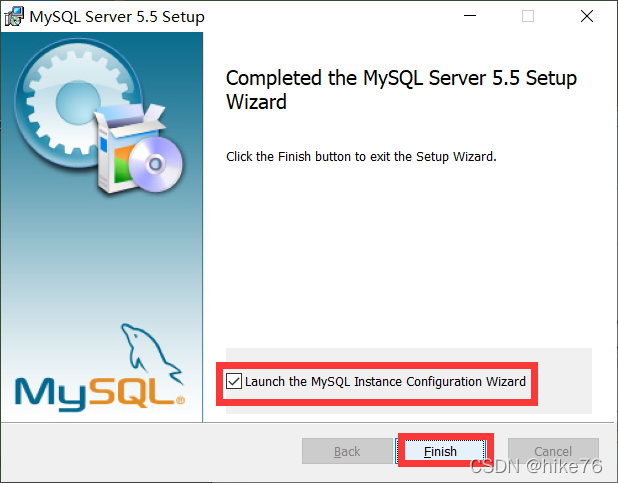
打开MySQL安装配置程序。?
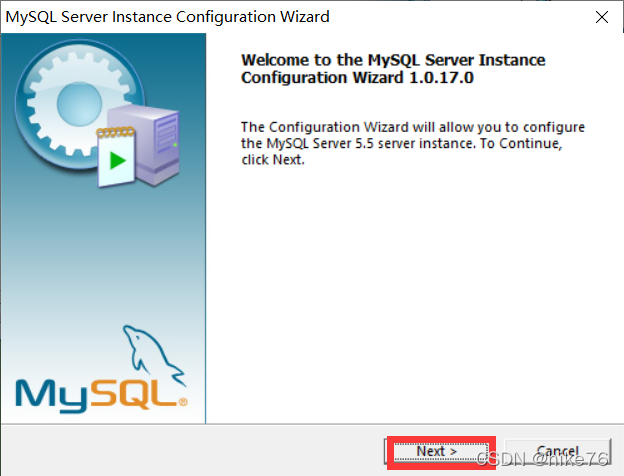
?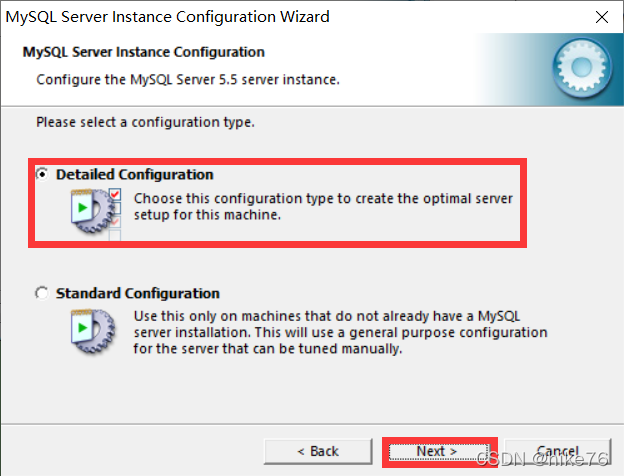
?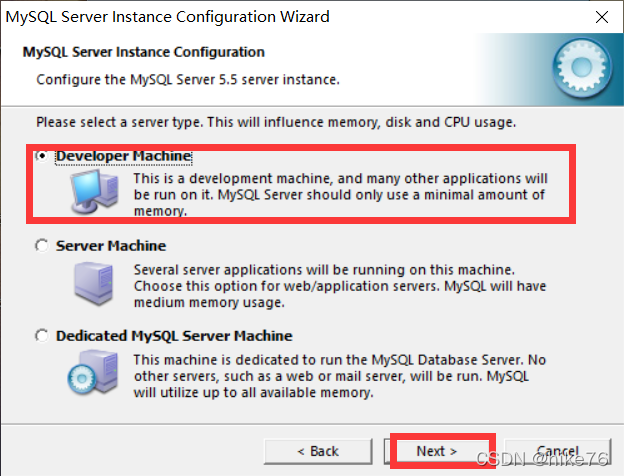
?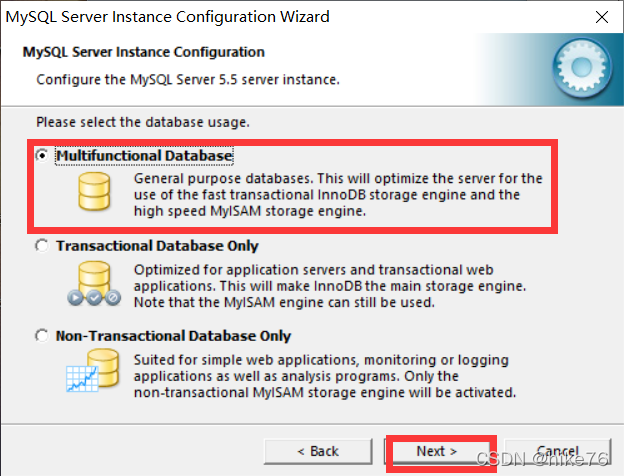
?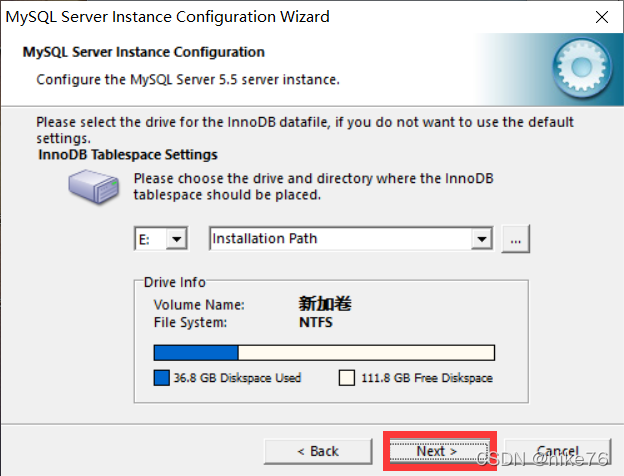
?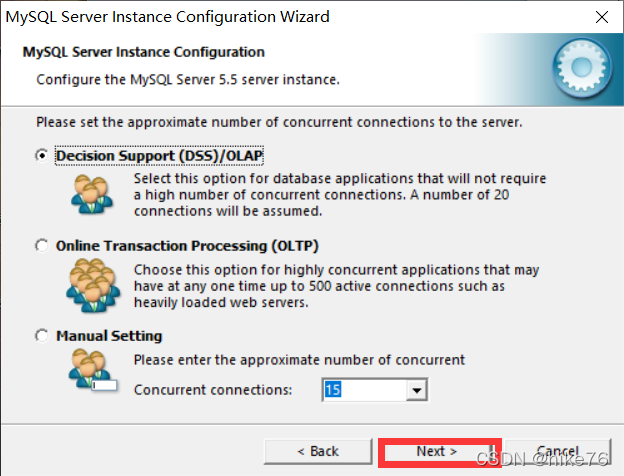
?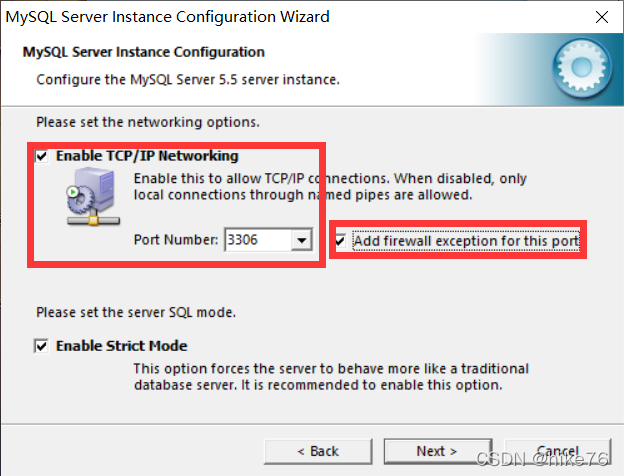
将右边的对勾选中,并选中下面“使用严格编码方式(Enable Strict Mode)”。
?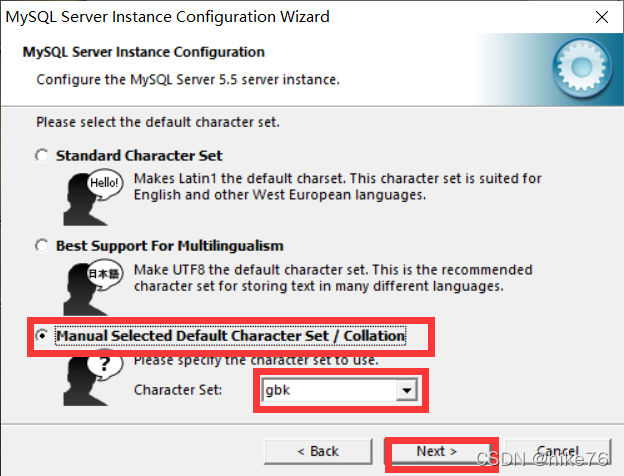
选择gbk编码方式。
?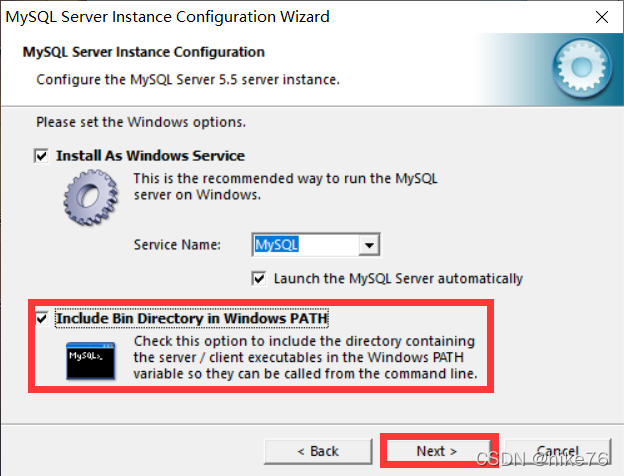
?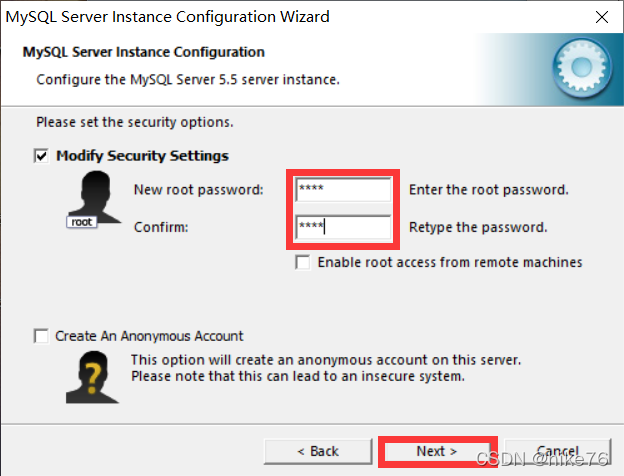
自定义输入用户密码。
?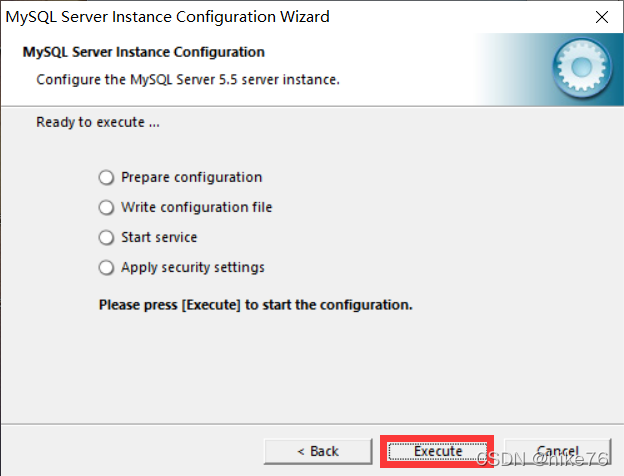
?等待安装即可。
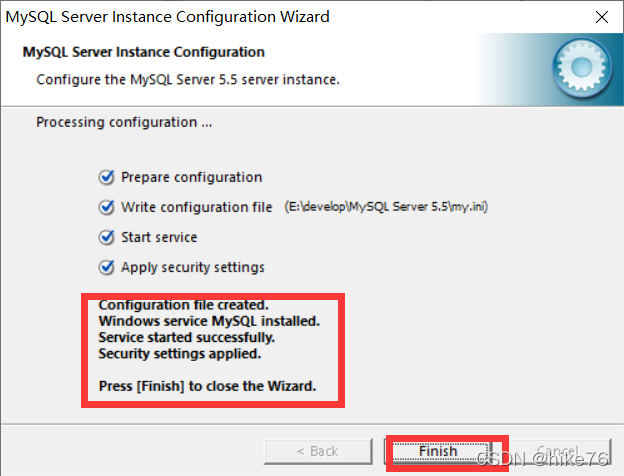
配置完成!
2、基本操作
????????MySQL是C/S架构的程序,服务器程序是 mysqld.exe 客户端程序是 mysql.exe。
????????想要连接到服务器需要在win + R 进入cmd ,输入musql -u用户名 -p密码 -h127.0.0.1(本机地址) -P3306(端口号),其中-h与-P可省略。
????????也可以快速连接到MySQL服务器:在桌面新建“启动MySQL客户端.bat”,输入以下内容 mysql -h127.0.0.1 -P3306 -uroot -p密码,保存,双击即可快速连接到MySQL服务器。
????????出现以下内容,即证明安装成功。
(1)查看服务器中的所有数据库:show databases;? ? ? ??
数据库以目录的形式保存在服务器,安装目录在/data目录下
(2)创建新的数据库:create database company;
(3)切换数据库:use company;
(4)查看当前数据中的所有表:show tables;
(5)把.sql文件中数据导入数据库:source 文件路径 文件名;如?source E:\develop\MyWork\company.sql
https://download.csdn.net/download/weixin_43923463/77661592下载company.sql,world.sql
(6)查看表中的所有数据:select * from 表名;如 select * from employees;
(7)查看当前所在的数据库?select database();
(8)在company数据库下创建表:create table 表名;
create table customer(
id int,
name varchar(20),
age int,
email varchar(50),
gender enum('男','女')
);
(9)查看表结构:describe 表名; 如describe customer;
(10)插入数据:insert into 表名(属性) values(属性值);
insert into customer(
id,
name,
age,
email,
gender
) values (
1,
'张三',
25,
'zhangsan@qq.com',
'男'
);
insert into customer(
id,
name,
age,
email,
gender
) values (
2,
'李四',
50,
'lisi@qq.com',
'女'
);
insert into customer(
id,
name,
age,
email,
gender
) values (
3,
'王五',
28,
'王五@qq.com',
'男'
);
(11)修改数据:update 表名 set 属性=修改后的数据 where 限定条件,如果没有where会修改所有数据。
update customer set
age = 16,
email = 'RRR'
where
id = 2;
(12)删除数据:delete from 表名 where 限定条件,没有where会删除所有数据。
delete from customer
where id = 3;
MySQL服务器架构模式:
????????服务器管理若干个数据库,每个数据库下管理若干个表,每个表下管理若干个记录。
SQL注意点:
????SQL 语言大小写不敏感。? ?? ?SQL 可以写在一行或者多行 ?? ?关键字不能被缩写也不能分行 ?? ?各子句一般要分行写。 ?? ?使用缩进提高语句的可读性。
3、数据查询
(1)select 列限定条件(列名)?from 表名;
select
population,
name,
code
from
country;
????????给列起别名,可以省略as关键字
select
population as pop,
name,
code
from
country;
????????别名中如果有特殊符号,可以使用""
select
population as pop,
name "国家 名称",
code
from
country;
(2)查看表结构:desc 表名;
desc country;
+----------------+---------------------------------------------------------------------------------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+----------------+---------------------------------------------------------------------------------------+------+-----+---------+-------+
| Code | char(3) | NO | PRI | | |
| Name | char(52) | NO | | | |
| Continent | enum('Asia','Europe','North America','Africa','Oceania','Antarctica','South America') | NO | | Asia | |
| Region | char(26) | NO | | | |
| SurfaceArea | float(10,2) | NO | | 0.00 | |
| IndepYear | smallint(6) | YES | | NULL | |
| Population | int(11) | NO | | 0 | |
| LifeExpectancy | float(3,1) | YES | | NULL | |
| GNP | float(10,2) | YES | | NULL | |
| GNPOld | float(10,2) | YES | | NULL | |
| LocalName | char(45) | NO | | | |
| GovernmentForm | char(45) | NO | | | |
| HeadOfState | char(60) | YES | | NULL | |
| Capital | int(11) | YES | | NULL | |
| Code2 | char(2) | NO | | | |
+----------------+---------------------------------------------------------------------------------------+------+-----+---------+-------+
desc city;
+-------------+----------+------+-----+---------+----------------+
| Field | Type | Null | Key | Default | Extra |
+-------------+----------+------+-----+---------+----------------+
| ID | int(11) | NO | PRI | NULL | auto_increment |
| Name | char(35) | NO | | | |
| CountryCode | char(3) | NO | MUL | | |
| District | char(20) | NO | | | |
| Population | int(11) | NO | | 0 | |
+-------------+----------+------+-----+---------+----------------+
desc countrylanguage;
+-------------+---------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------------+---------------+------+-----+---------+-------+
| CountryCode | char(3) | NO | PRI | | |
| Language | char(30) | NO | PRI | | |
| IsOfficial | enum('T','F') | NO | | F | |
| Percentage | float(4,1) | NO | | 0.0 | |
+-------------+---------------+------+-----+---------+-------+
(3)比较运算(过滤行查询)
????????执行顺序 : 先from, 再where 最后select。
select employee_id, last_name, job_id, department_id
from employees
where department_id = 90 ;
? ? ? ? 给每一列取别名,where中不可以使用列的别名,因为此时虚表还没有生成。
select
employee_id empId,
last_name name,
job_id job,
department_id deptId
from
employees
where
department_id = 90 ;
?????????查询人口总数大于1亿的国家的名称和大洲及人口, 给人口列起别名.
select
name,
continent,
population as pop
from
country
where
population > 100000000;
????????Between a and b 都包含
SELECT last_name, salary
FROM ? employees
WHERE ?salary BETWEEN 2500 AND 3500;
相当于 salary >= 2500 and salary <= 3500
? ? ? ? in 都包含?
SELECT employee_id, last_name, salary, manager_id
FROM ? employees
WHERE ?manager_id IN (100, 101, 201);
相当于 manager_id = 101 or manager_id = 100 or manager_id = 201
????????查询所有的亚洲或欧洲国家的名称和大洲及国家代码
select
name,
continent,
code
from
country
where
continent in ('asia','europe');
????????% 表示任意个任意字符
select
code,
name,
continent
from
country
where
name like '%na%';
????????_ 表示一个任意字符?? ?
select
code,
name,
continent
from
country
where
name like '__na%';
????????查询人均寿命小于50岁的国家
select
name,
LifeExpectancy
from
country
where
LifeExpectancy < 50;
????????查询城市名称包含ek的城市 world.city
select
name,
id,
countrycode
from
city
where
name like '%ek%';
????????查询姓名中第2个字母是o其他无所谓
SELECT last_name
FROM employees
WHERE last_name LIKE '_o%';
????????查询哪些国家没有首都,只要有null参与比较运算, 结果一定是false
select
name,
capital
from
country
where
capital is null;
????????查询哪些国家有首都
select
name,
continent,
capital
from
country
where
capital is not null;
????????查询所有亚洲国家 (人口大于1亿或者尚未独立)的代码、名称、大洲及人口
select
name,
code,
continent,
population,
indepyear
from
country
where
continent = 'asia'
and
(
population > 100000000
or
indepyear is null
);
????????where 也支持算术运算, 结果为0表示假非0表示真
select
code,
name
from
country
where
population + 100;
select
code,
name
from
country
where
2 - 2;
????????distinct 去重, 要求列真的有重复,否则没有效果。当两个列同时去重时,保留范围大的。
select
distinct
continent
from
country;
select
distinct
region,
continent
from
country ;
????????查询中国有哪些不同的省份
select
distinct
district
from
city
where
countrycode = 'chn';
????????where 过滤行,select过滤列。先处理行,后处理列。
4、排序
????????order by ?可以排序, 只是给结果集虚表排序,默认是升序(asc),降序必须指定(desc)。order by 可以使用列的别名,因为order by最后执行。
select
distinct
district dis
from
city
where
countrycode = 'chn'
order by
dis desc;
????????order by ?列1, 列2 先以列1排序, 再在相同的列1数据中, 依据列2排序。
SELECT
last_name,
department_id,
salary
FROM
employees
ORDER BY
department_id,
salary DESC;
????????运算步骤 :? ????????1) from 基表 ????????2) where 过滤哪些行 ????????3) select 选择哪些列 ????????4) order by 以哪些列为排序依据.?
????????查看国家的人口, 以大洲排序, 查看每个大洲中人口最多的国家。
select
code,
name,
population,
continent
from
country
order by
continent,
population;
|