7. 事务操作
7.1 事务概念
Ⅰ、什么是事务
? 事务是数据库操作最基本单元,逻辑上一组操作,要么都成功,如果有一个失败则所有操作都失败
Ⅱ、典型场景
? 银行转账 * lucy 转账 100 元 给 mary
? lucy 少 100,mary 多 100
Ⅲ、事务四个特性(ACID)
(1)原子性
(2)一致性
(3)隔离性
(4)持久性
7.2 搭建事务环境
演示图:
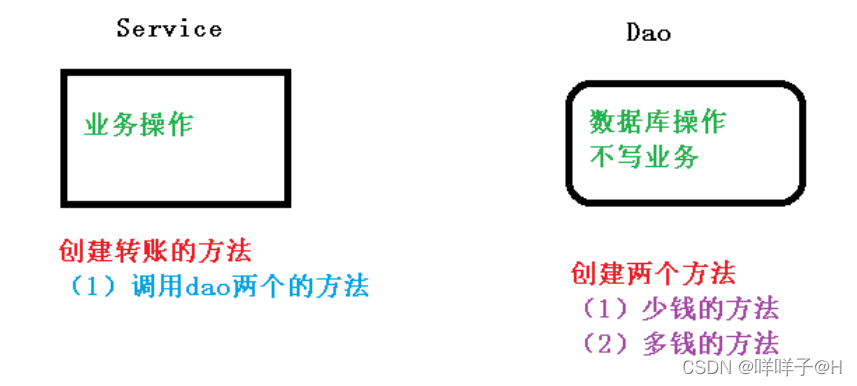
step1:创建数据库表并插入数据
create table t_account
(
id varchar(20) not null
primary key,
username varchar(50) not null,
money int not null
);
step2:创建Service和Dao类,并将JdbcTemplate注入到Dao,将Dao注入到Service
@Repository
public class UserDaoImpl implements UserDao{
@Autowired
private JdbcTemplate jdbcTemplate;
}
@Service
@Transactional(propagation = Propagation.REQUIRED,isolation = Isolation.DEFAULT,timeout = -1)
public class UserService {
@Autowired
private UserDao userDao;
step3:钱数改变方法的创建
@Override
public void reduceMoney(int money,String username) {
String sql = "update t_account set money=money-? where username=?";
jdbcTemplate.update(sql,money,username);
}
@Override
public void addMoney(int money,String username) {
String sql = "update t_account set money=money+? where username=?";
jdbcTemplate.update(sql,money,username);
}
step4:在Service种创建相应的转账业务方法
public void transfer(){
ApplicationContext context =
new ClassPathXmlApplicationContext("bean1.xml");
userDao.reduceMoney(100,"lucy");
userDao.addMoney(100,"mary");
}
问题:如果钱数减少之后,出现异常或者断电等特殊情况,无法继续执行,就会导致数据进入不一致状态
解决方法:事务--------下述方法为编程式事务
public void transfer(){
try{
ApplicationContext context =
new ClassPathXmlApplicationContext("bean1.xml");
userDao.reduceMoney(100,"lucy");
int i = 10/0;
userDao.addMoney(100,"mary");
} catch (Execption e){
}
}
7.3 Spring事务管理介绍
Ⅰ、事务添加到 JavaEE 三层结构里面 Service 层(业务逻辑层)
? 并不是强制要求添加到service层,但是事务一般也属于业务逻辑内容,所以多放在Service层进行处理
Ⅱ、Spring进行事务管理操作的方式
- 编程式事务------通过编写代码来实现
- 声明式事务------通过配置来实现(常用)
Ⅲ、声明式事务管理
Ⅳ、Spring声明式事务管理底层原理**
? 底层采用Aop原理,从前面的编程式事务中可以看出,在Service层添加事务,本质上就是对可能出现异常的部分通过try-catch进行包裹,如果正常执行完所有业务流程,就commit()提交。如果业务逻辑被异常所中断,则跳转至catch部分,此时未完成的事务显然需要回滚Rollback(),从而回到一个数据一致性状态。
为什么Aop可以实现声明式事务?
**答:**从编程向声明式事务的转化,事务的使用断崖式简化。本质是对需要添加事务的方法,通过aop配置环绕通知,在其原本方法的前后,加上try-catch进行异常捕获,再根据情况进行提交或者回滚。当然更底层当然是代理模式,通过代理对象来实现,不过这里的代理对象是通过Spring框架来产生的。
Ⅴ、Spring事务管理
提供一个接口,代表事务管理器,这个接口针对不同的框架提供不同的实现类
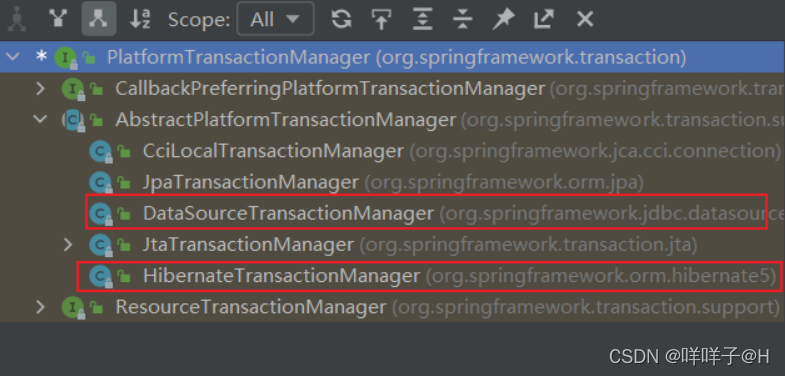
DataSourceTransactionManager :JdbcTemplate以及Mybatis使用 (SSM)
HibernateTransactionManager :Spring整合Hibernate进行数据库操作时使用 (SSH)
7.4 注解声明式事务管理
step1:在Spring配置文件配置事务管理器
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"></property>
</bean>
step2:引入tx名称空间,开启事务注解
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<tx:annotation-driven transaction-manager="transactionManager"/>
</beans>
step3:在需要启用事务的类或者方法上面加上@Transactional注解
@Service
@Transactional(propagation = Propagation.REQUIRED,isolation = Isolation.DEFAULT,timeout = -1)
public class UserService {
@Autowired
private UserDao userDao;
public void transfer(){
ApplicationContext context =
new ClassPathXmlApplicationContext("bean1.xml");
userDao.reduceMoney(100,"lucy");
userDao.addMoney(100,"mary");
}
}
注:(1)@Transactional,这个注解添加到类上面,也可以添加方法上面
(2)如果把这个注解添加类上面,这个类里面所有的方法都添加事务
(3)如果把这个注解添加方法上面,为这个方法添加事务
7.5 声明式注解的相关参数—@Transactional注解
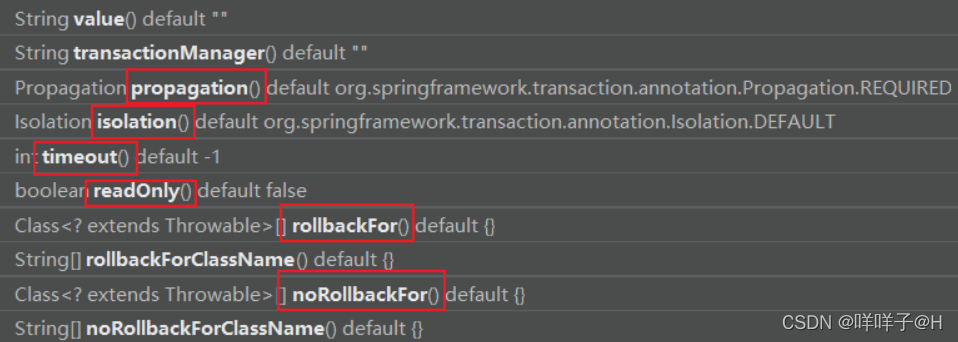
Ⅰ、propagation 事务传播行为
**Define:**多事务方法直接进行调用,这个过程中事务是如何进行管理的
图解:
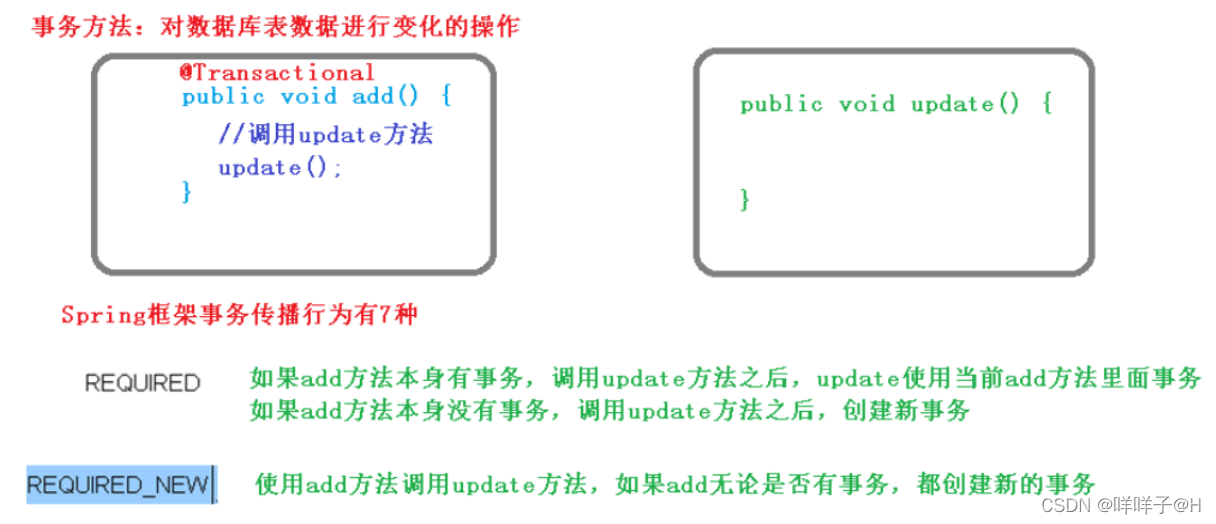 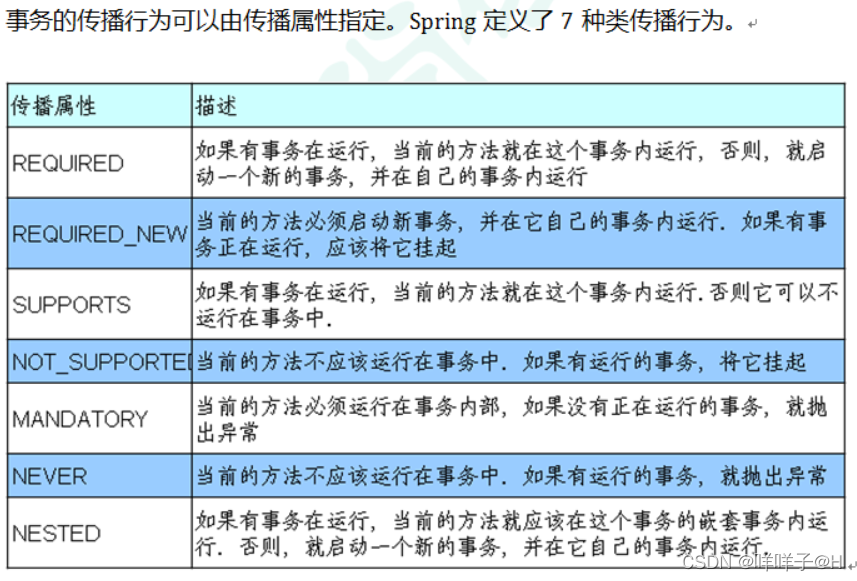
Ⅱ、isolation:事务隔离级别
(1)事务有特性称为隔离性,多事务操作之间不会产生影响。不考虑隔离性产生很多问题
(2)有三个读问题:脏读、不可重复读、虚(幻)读
**(3)脏读:**一个未提交事务读取到另一个未提交事务的数据
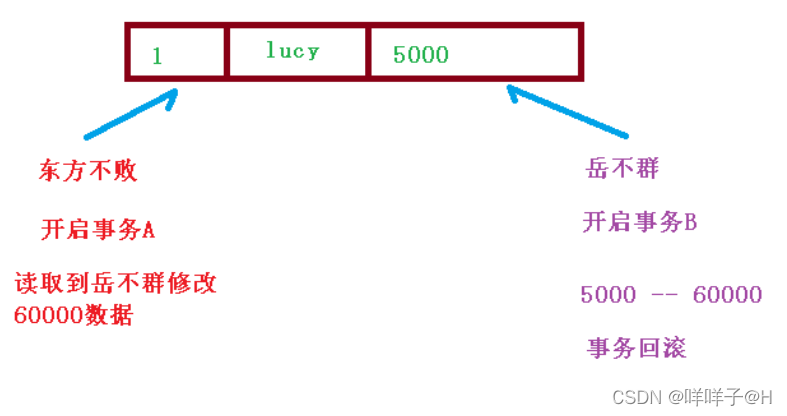
**(4)不可重复读:**一个未提交事务读取到另一提交事务修改数据-------针对Update
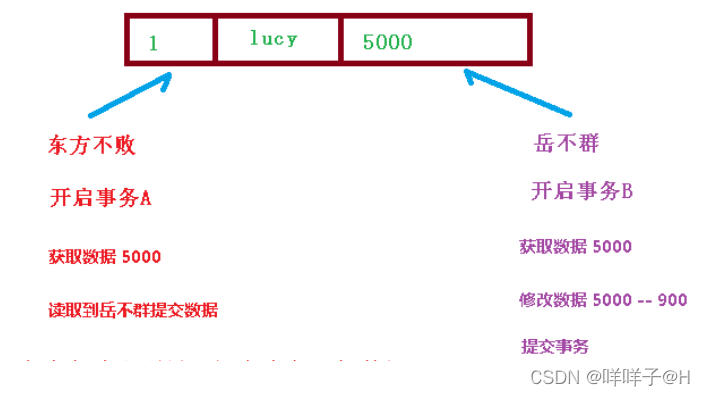
**(5)虚读:**一个未提交事务读取到另一提交事务添加数据-----针对Insert
**(6)解决:**通过设置事务隔离级别,解决读问题
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-5aoV1GlI-1644937341013)(D:\桌面\spring5\笔记\分析图\事务\事务隔离级别.bmp)]](https://img-blog.csdnimg.cn/acf912d997124ceb9435e9204877748a.png?x-oss-process=image/watermark,type_d3F5LXplbmhlaQ,shadow_50,text_Q1NETiBA5ZKp5ZKp5a2QQEg=,size_20,color_FFFFFF,t_70,g_se,x_16)
public enum Isolation {
DEFAULT(-1),
READ_UNCOMMITTED(1),
READ_COMMITTED(2),
REPEATABLE_READ(4),
SERIALIZABLE(8);
private final int value;
private Isolation(int value) {
this.value = value;
}
public int value() {
return this.value;
}
}
Ⅲ、timeout:超时时间
(1)事务需要在一定时间内进行提交,如果不提交进行回滚
(2)默认值是 -1 ,设置时间以秒单位进行计算
Ⅳ、readOnly:是否只读
(1)读:查询操作,写:添加修改删除操作
(2)readOnly 默认值 false,表示可以查询,可以添加修改删除操作
(3)设置 readOnly 值是 true,设置成 true 之后,只能查询
Ⅴ、rollbackFor:因哪些异常回滚
rollbackFor = {ExecutionException.class,NullPointerException.class}
设置可以为异常.class,并且可以设置多个,以数组形式添加
Ⅵ、noRollbackFor:不因哪些异常回滚
noRollbackFor = {ExecutionException.class,NullPointerException.class}
7.6 XML声明式事务管理
Ⅰ、在 spring 配置文件中进行配置
step1: 配置事务管理器
step2: 配置通知
step3: 配置切入点和切面
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"></property>
</bean>
<tx:advice id="txadvice">
<tx:attributes>
<tx:method name="transfer" propagation="REQUIRED"/>
</tx:attributes>
</tx:advice>
<aop:config>
<aop:pointcut id="ptx" expression="execution(* com.atguigu.spring5.service.UserService.*(..))"/>
<aop:advisor advice-ref="txadvice" pointcut-ref="ptx"></aop:advisor>
</aop:config>
7.7 完全注解式声明式事务
创建配置类,代替xml配置文件
@EnableTransactionManagement :开启事务
@Bean :创建对象
注:在加上@Bean 注解的类中的参数,会自动根据IOC容器中类型匹配的对象注入
@Configuration
@ComponentScan(basePackages = "com.atguigu.spring5")
@EnableTransactionManagement
public class TxConfig {
@Bean
public DruidDataSource getDruidDataSource(){
DruidDataSource dataSource = new DruidDataSource();
dataSource.setDriverClassName("com.mysql.jdbc.Driver");
dataSource.setUrl("jdbc:mysql:///user_db");
dataSource.setUsername("root");
dataSource.setPassword("root");
return dataSource;
}
@Bean
public JdbcTemplate getJdbcTemplate(DataSource dataSource){
JdbcTemplate jdbcTemplate = new JdbcTemplate();
jdbcTemplate.setDataSource(dataSource);
return jdbcTemplate;
}
@Bean
public DataSourceTransactionManager getDataSourceTransactionManager(DataSource dataSource){
DataSourceTransactionManager transactionManager = new DataSourceTransactionManager(dataSource);
return transactionManager;
}
}
|