JDBC : Java DataBase Connectivity (java数据库链接),是让java链接数据库的API
1. 基础使用步骤
? ? 第0步: 导包

?创建项目-创建lib文件-将mysql-connector-java-5.1.38-bin.jar 复制到lib中
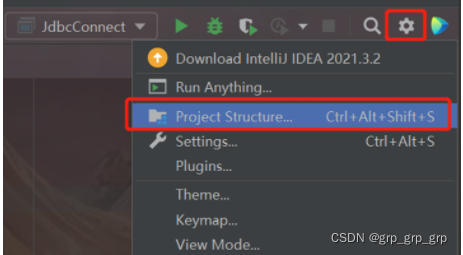
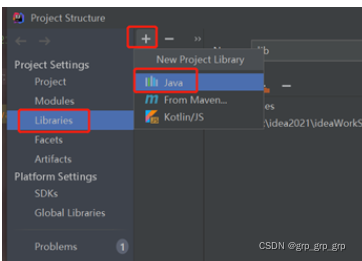 ? ? 第1步:注册驱动 (仅仅做一次) ? ? 第2步:建立连接(Connection) ? ? 第3步:创建运行SQL的语句对象(Statement) ? ? 第4步:运行语句 ? ? 第5步:处理运行结果(ResultSet) ? ? 第6步:释放资源?
2. 代码实现
public static void main(String[] args) throws Exception {
//第1步:注册驱动 (仅仅做一次)
Class.forName("com.mysql.jdbc.Driver");
//第2步:建立连接(Connection)
Connection conn= DriverManager.getConnection("jdbc:mysql://127.0.0.1:3306/_21_","root","root");
//第3步:创建运行SQL的语句对象(Statement)
String sql="select * from student";
Statement statement=conn.createStatement();
//第4步:运行语句,得到结果集
ResultSet resultSet = statement.executeQuery(sql);
//第5步:处理运行结果(ResultSet)
while(resultSet.next()){
System.out.println("索引打印"+resultSet.getString(2));
System.out.println("标签打印"+resultSet.getString("name"));;
}
//第6步:释放资源
resultSet.close();
statement.close();
conn.close();
}
2.1 代码优化
public static void main(String[] args) {
ResultSet resultSet=null;
Statement statement=null;
Connection conn=null;
try {
//第1步:注册驱动 (仅仅做一次)
Class.forName("com.mysql.jdbc.Driver");
//第2步:建立连接(Connection)
conn= DriverManager.getConnection("jdbc:mysql://127.0.0.1:3306/_21_","root","root");
//第3步:创建运行SQL的语句对象(Statement)
String sql="select * from student";
statement=conn.createStatement();
//第4步:运行语句,得到结果集
resultSet = statement.executeQuery(sql);
//第5步:处理运行结果(ResultSet)
while(resultSet.next()){
System.out.println("索引打印"+resultSet.getString(2));
System.out.println("标签打印"+resultSet.getString("name"));;
}
}catch (Exception e){
e.printStackTrace();
}finally {
try {
//第6步:释放资源
if(resultSet!=null){
resultSet.close();
}
if(statement!=null){
statement.close();
}
if(conn!=null){
conn.close();
}
}catch (Exception e){
e.printStackTrace();
}
}
}
3. DML
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 1 加载驱动
Class.forName("com.mysql.jdbc.Driver");
// 2 创建数据库连接对象
conn = DriverManager.getConnection(
"jdbc:mysql://127.0.0.1:3306/_06_", "root", "root");
// 3 语句传输对象
stmt = conn.createStatement();
String sql = "insert into test_jdbc (id,name,money)
values (4,'小小',999.9)";
// 如果是查询,就返回true,不是就返回false,价值不大,
所以用的不多,添加,删除,更新都可以用这个方法
// 返回值是int,返回影响了几条数据
(更改了几条/删除了几条/添加了几条),添加,删除,更新都可以用这个方法
int count = stmt.executeUpdate(sql);
System.out.println("影响了 " + count + " 条数据");
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
// 关闭资源,从上到下依次关闭,后打开的先关闭
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (conn != null) {
conn.close();
}
} catch (Exception e2) {
e2.printStackTrace();
}
}
4.?PreparedStatement
? preparedStatement 是?statement的子类,多用于更新数据 ? ? ? ? ? ? String sql="insert into student values (9,'aaaaa',1,99) "; ? ? ? ? ? ? preparedStatement = conn.prepareStatement(sql); ? ? ? ? ? ? //第4步:运行语句,得到结果集 ? ? ? ? ? ? int i = preparedStatement.executeUpdate(); ? ? ? ? ? ? System.out.println("影响了"+i+"条");
5.?Statement 和 PreparedStatement 的区别
?Statement用于执行静态SQL语句,在执行的时候,必须指定一个事先准备好的SQL语句,并且相对不安全,会有SQL注入的风险
?PreparedStatement是预编译的SQL语句对象,sql语句被预编译并保存在对象中, 被封装的sql语句中可以使用动态包含的参数 ? ,在执行的时候,可以为?传递参数
使用PreparedStatement对象执行sql的时候,sql被数据库进行预编译和预解析,然后被放到缓冲区,每当执行同一个PreparedStatement对象时,他就会被解析一次,但不会被再次编译 可以重复使用,可以减少编译次数,提高数据库性能并且能够避免SQL注入,相对安全(把’ 单引号 使用 \ 转义,避免SQL注入 )
6. DQL
public static void load(int id) {
Connection conn = null;
PreparedStatement prst = null;
ResultSet rs = null;
try {
// 1 加载驱动
Class.forName("com.mysql.jdbc.Driver");
// 2 创建数据库连接对象
conn = DriverManager.getConnection(
"jdbc:mysql://127.0.0.1:3306/_06_", "root", "root");
// 这里我们用? 问号代替值,可以叫占位符,也可以叫通配符
String sql = "select * from test_jdbc where id = ?";
// 3 语句传输对象
prst = conn.prepareStatement(sql);
// 设置第一个?的值
prst.setInt(1, id);
rs = prst.executeQuery();
while (rs.next()) {
System.out.print(rs.getInt("id") + " ");
System.out.print(rs.getString("name") + " ");
System.out.println(rs.getString("money") + " ");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
// 关闭资源,从上到下依次关闭,后打开的先关闭
if (rs != null) {
rs.close();
}
if (prst != null) {
prst.close();
}
if (conn != null) {
conn.close();
}
} catch (Exception e2) {
e2.printStackTrace();
}
}
}
7. 封装工具类
public static void main(String[] args) {
ResultSet resultSet=null;
Statement statement=null;
Connection conn=null;
try {
conn=JdbcUtil.getConnection();
//第3步:创建运行SQL的语句对象(Statement)
String sql="select * from student";
statement=conn.createStatement();
//第4步:运行语句,得到结果集
resultSet = statement.executeQuery(sql);
//第5步:处理运行结果(ResultSet)
while(resultSet.next()){
System.out.println("索引打印"+resultSet.getString(2));
System.out.println("标签打印"+resultSet.getString("name"));;
}
}catch (Exception e){
e.printStackTrace();
}finally {
//优化的关闭资源
JdbcUtil.closeResultSet(resultSet);
JdbcUtil.closeResultSet(statement);
JdbcUtil.closeResultSet(conn);
}
}
8. sql注入
所谓SQL注入,就是通过把SQL命令插入到Web表单提交或输入域名或页面请求的查询字符串,最终达到欺骗服务器执行恶意的SQL命令。具体来说,它是利用现有应用程序,将(恶意的)SQL命令注入到后台数据 库引擎执行的能力,它可以通过在Web表单中输入(恶意)SQL语句得到一个存在安全漏洞的网站上的数据库,而不是按照设计者意图去执行SQL语句。
String name="' or 'a'='a";
conn=JdbcUtil.getConnection();
//第3步:创建运行SQL的语句对象(Statement)
String sql="update student set name='a' where name=?";
9. 事务
在计算机术语中是指访问并可能更新数据库中各种数据项的一个程序执行单元
9.1 四大特性
原子性(atomicity):一个事务是一个不可分割的工作单位,事务中包括的操作要么都做,要么都不做。
一致性(consistency):事务必须是使数据库从一个一致性状态变到另一个一致性状态。一致性与原子性是密切相关的。
隔离性(isolation):一个事务的执行不能被其他事务干扰。即一个事务内部的操作及使用的数据对并发的其他事务是隔离的,并发执行的各个事务之间不能互相干扰。
持久性(durability):持久性也称永久性(permanence),指一个事务一旦提交,它对数据库中数据的改变就应该是永久性的。接下来的其他操作或故障不应该对其有任何影响。
|