首先在composer.json 加上以下代码。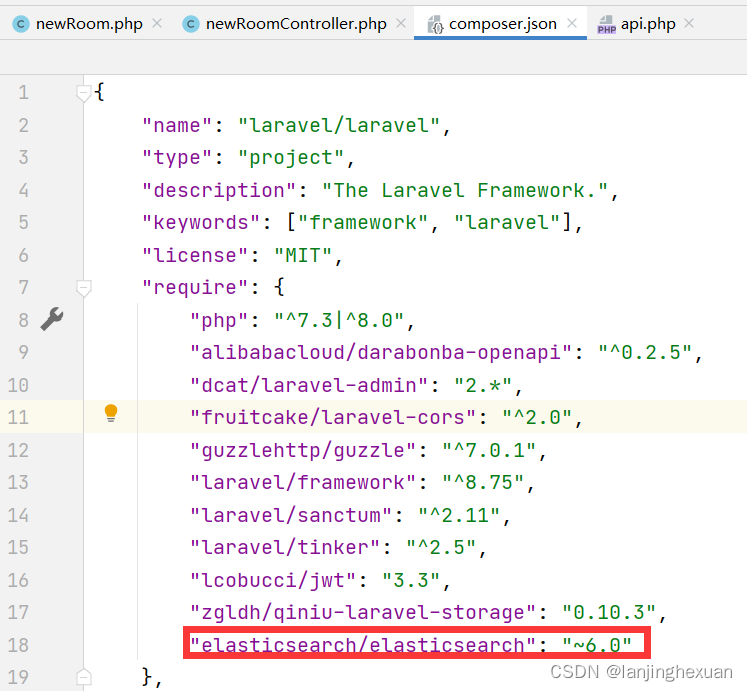
"elasticsearch/elasticsearch": "~6.0"
使用composer 完成拓展的加载
composer update elasticsearch/elasticsearch
添加索引
public function createIndex()
{
$client = ClientBuilder::create()->build();
$params = [
'index' => 'new_home',
//索引名
'body' => [
'settings' => [
//分片
'number_of_shards' => 3,
'number_of_replicas' => 2
],
'mappings' => [
'_source' => [
'enabled' => true
],
'properties' => [
//字段名称
'name' => [
//文字
'type' => 'text',
//ik分词器
"analyzer" => "ik_max_word",
"search_analyzer" => "ik_max_word"
],
'id' => [
//数值
'type' => 'integer'
]
]
]
]
];
$response = $client->indices()->create($params);
var_dump($response);
}
elasticsearch添加数据
public function addEs($id, $name)
{
$client = ClientBuilder::create()->build();
$params = [
//索引名
'index' => 'new_home',
'type' => '_doc',
//指定id
'id' => $id,
//内容
'body' => [
'name' => $name,
'id' => $id
]
];
$response = $client->index($params);
print_r($response);
echo "<br>";
}
elasticsearch搜索
public function searchEs(Request $request)
{
$name = $request['name'];
if (empty($name)) {
return showMsg(500, '查询条件不能为空');
}
$client = ClientBuilder::create()->build();
$params = [
//索引名
'index' => 'new_home',
'type' => '_doc',
'body' => [
'query' => [
'match' => [
//条件
'name' => $name
]
],
//高亮显示
'highlight' => [
'pre_tags' => ["<font color='red'>"],
'post_tags' => ["</font>"],
'fields' => [
"name" => new \stdClass()
]
]
]
];
//前台高亮显示
/**
* {!! $变量 !!}
*/
$results = $client->search($params);
return showMsg(200, '查询成功', $results['hits']['hits']);
}
以下为全部代码
<?php
namespace App\Http\Controllers;
//composer update elasticsearch/elasticsearch
use Illuminate\Http\Request;
use Elasticsearch\ClientBuilder;
class newRoomController extends Controller
{
/**
* 创建索引
*/
public function createIndex()
{
$client = ClientBuilder::create()->build();
$params = [
'index' => 'new_home',
//索引名
'body' => [
'settings' => [
//分片
'number_of_shards' => 3,
'number_of_replicas' => 2
],
'mappings' => [
'_source' => [
'enabled' => true
],
'properties' => [
//字段名称
'name' => [
//文字
'type' => 'text',
//ik分词器
"analyzer" => "ik_max_word",
"search_analyzer" => "ik_max_word"
],
'id' => [
//数值
'type' => 'integer'
]
]
]
]
];
$response = $client->indices()->create($params);
var_dump($response);
}
/**
* es添加数据
* @param $id
* @param $name
*/
public function addEs($id, $name)
{
$client = ClientBuilder::create()->build();
$params = [
//索引名
'index' => 'new_home',
'type' => '_doc',
//指定id
'id' => $id,
//内容
'body' => [
'name' => $name,
'id' => $id
]
];
$response = $client->index($params);
print_r($response);
echo "<br>";
}
/**
* mysql同步es
*/
public function mysql_es()
{
$date = \App\Models\newRoom::select('id', 'title')->get();
foreach ($date as $v) {
$this->addEs($v['id'], $v['title']);
}
}
/**
* es搜索
* @param Request $request
* @return false|string
*/
public function searchEs(Request $request)
{
$name = $request['name'];
if (empty($name)) {
return showMsg(500, '查询条件不能为空');
}
$client = ClientBuilder::create()->build();
$params = [
//索引名
'index' => 'new_home',
'type' => '_doc',
'body' => [
'query' => [
'match' => [
//条件
'name' => $name
]
],
//高亮显示
'highlight' => [
'pre_tags' => ["<font color='red'>"],
'post_tags' => ["</font>"],
'fields' => [
"name" => new \stdClass()
]
]
]
];
//前台高亮显示
/**
* {!! $变量 !!}
*/
$results = $client->search($params);
return showMsg(200, '查询成功', $results['hits']['hits']);
}
}
|