参考:https://blog.csdn.net/ThinkWon/article/details/103770879
https://github.com/alibaba/Sentinel/wiki/%E6%96%B0%E6%89%8B%E6%8C%87%E5%8D%97
官网下载:https://github.com/alibaba/Sentinel/tags
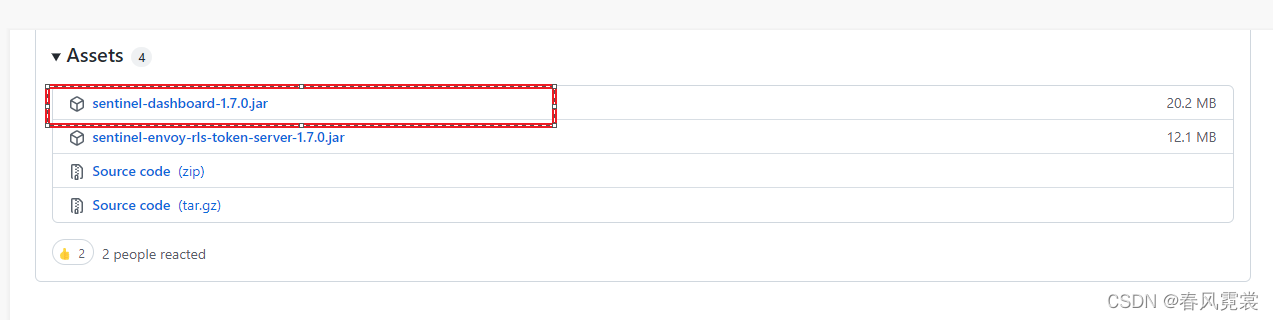
下载完成后在命令行输入如下命令运行Sentinel控制台:
java -jar sentinel-dashboard-1.7.0.jar
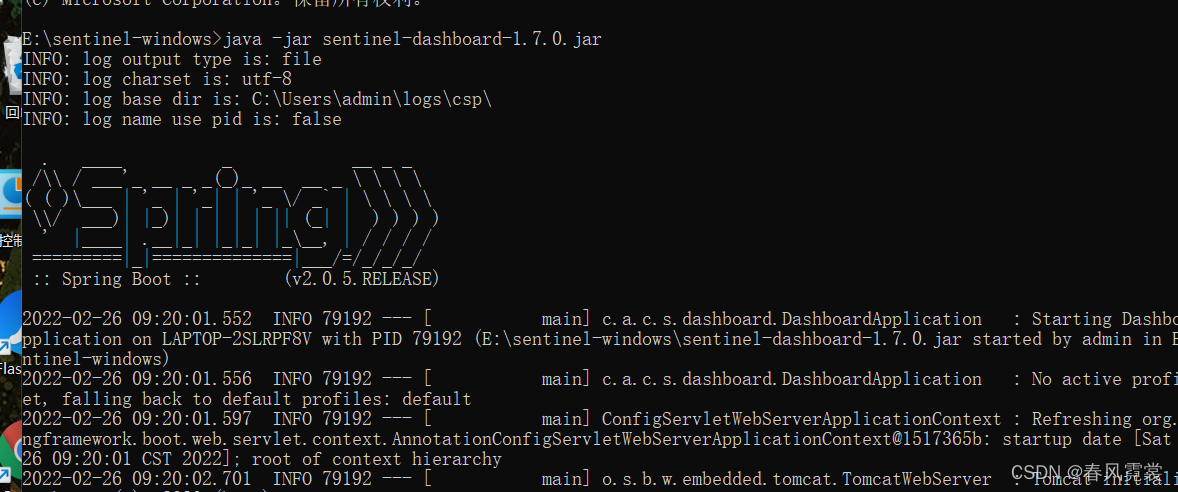 Sentinel控制台默认运行在8080端口上,登录账号密码均为sentinel,通过如下地址可以进行访问:http://localhost:8080
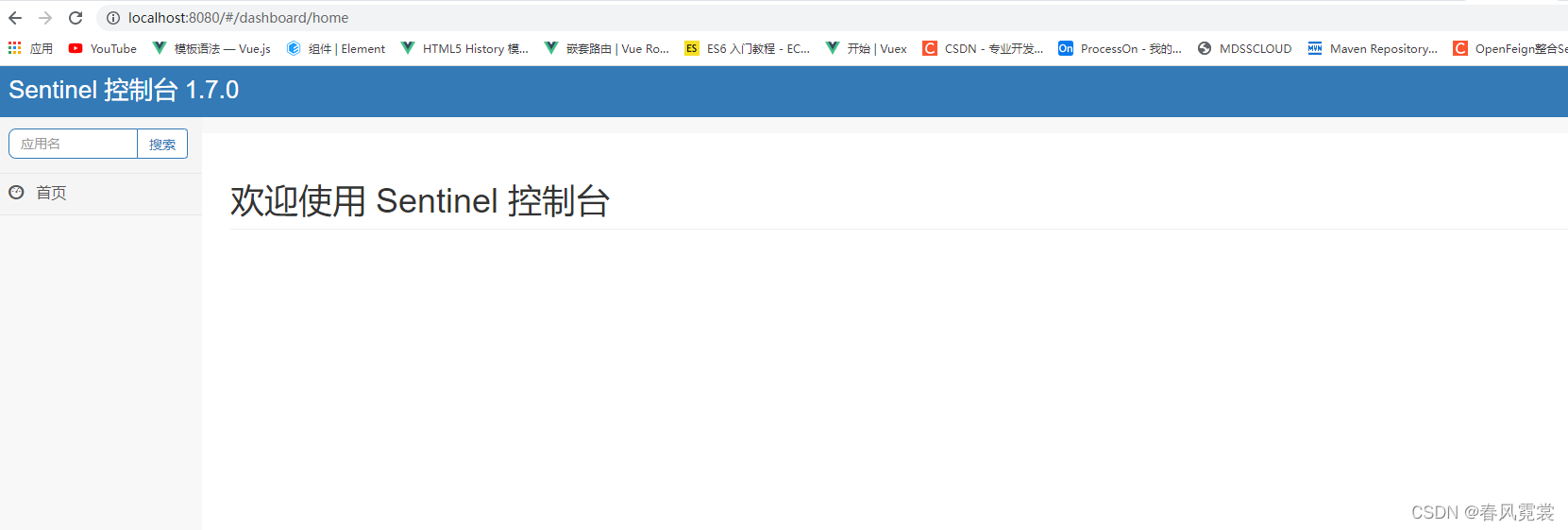 加pom:
<!-- https://mvnrepository.com/artifact/com.alibaba.cloud/spring-cloud-starter-alibaba-sentinel -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
<version>2.2.1.RELEASE</version>
</dependency>
在application.yml中添加相关配置,主要是配置了Nacos和Sentinel控制台的地址:
server:
port: 9093
spring:
application:
name: business-9093
redis:
database: 1
host: 49.235.125.47
port: 6379
password: 123456
timeout: 10000
jedis:
pool:
max-active: 8
max-idle: 8
min-idle: 0
max-wait: -1
cloud:
nacos:
discovery:
server-addr: 127.0.0.1:8848
sentinel:
transport:
dashboard: 127.0.0.1:8080
port: 8719
datasource:
ds1:
nacos:
server-addr: localhost:8848
dataId: cloudalibaba-sentinel-service
groupId: DEFAULT_GROUP
data-type: json
rule-type: flow
anagement:
endpoints:
web:
exposure:
include: '*'
feign:
sentinel:
enabled: true
要访问了controller之后,才会有值,懒加载!! 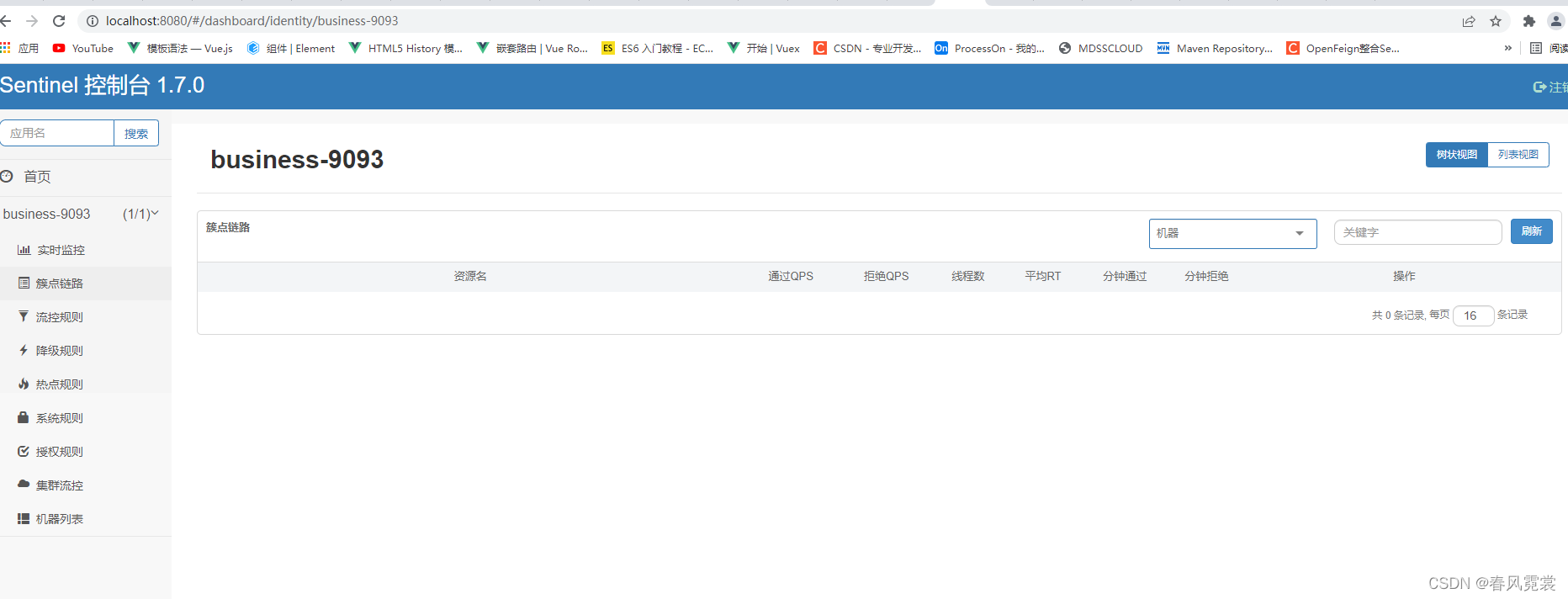
限流功能
- 按资源名称限流,需要指定限流处理逻辑
- 按url限流,有默认的限流处理逻辑
- 自定义限流处理逻辑
编写controller测试:
package com.example.businesssentinel9093.controller;
import cn.hutool.extra.tokenizer.Result;
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import com.alibaba.csp.sentinel.slots.block.BlockException;
import com.example.businesssentinel9093.myhandler.CustomerBlockHandler;
import com.example.dtestcommon.vo.Res;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/sentinel")
public class BusinessSentinelController {
@PostMapping("/test")
public String test(){
return "9093";
}
@PostMapping("/byResource")
@SentinelResource(value = "byResource", blockHandler = "handleException")
public Res byResource() {
return Res.success(200,"按资源名称限流");
}
@PostMapping("byUrl")
@SentinelResource(value = "byUrl", blockHandler = "handleException")
public Res byUrl() {
return Res.success(200,"按url限流");
}
public Res handleException(BlockException exception) {
return Res.success(200,exception.getClass().getCanonicalName());
}
@PostMapping("/customBlockHandler")
@SentinelResource(value = "customBlockHandler", blockHandler = "handlerException", blockHandlerClass = CustomerBlockHandler.class)
public Res blockHandler() {
return Res.success(200,"限流成功");
}
}
package com.example.businesssentinel9093.myhandler;
import com.alibaba.csp.sentinel.slots.block.BlockException;
import com.example.dtestcommon.vo.Res;
public class CustomerBlockHandler {
public static Res handlerException(BlockException exception)
{
return Res.success(444,"客户自定义限流处理信息1,global handlerException-----1");
}
public static Res handlerException2(BlockException exception)
{
return Res.success(444,"客户自定义限流处理信息2,global handlerException-----2");
}
}
1. 按资源名称限流,需要指定限流处理逻辑
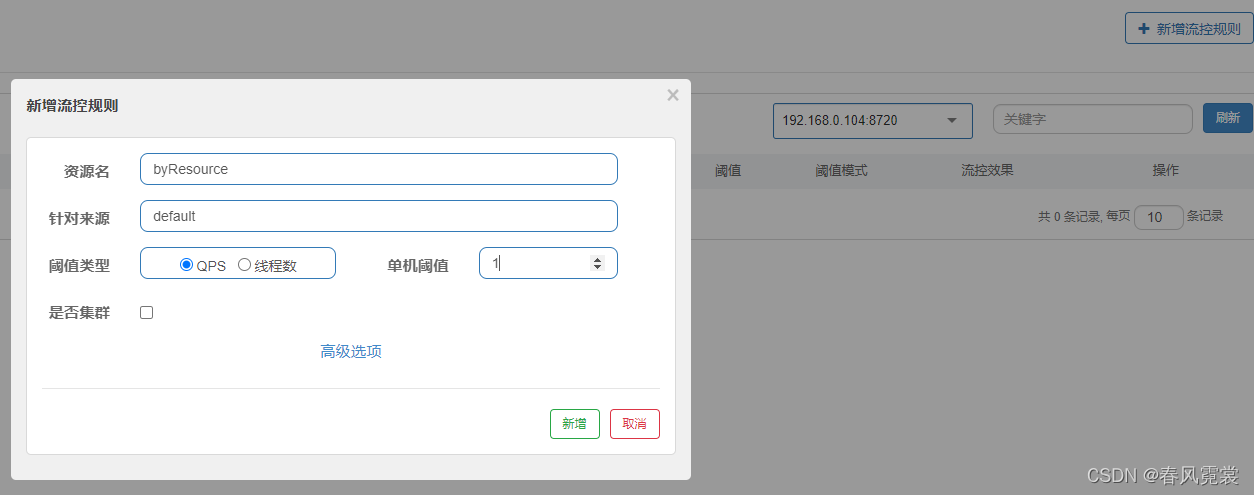
qps和线程的区别:
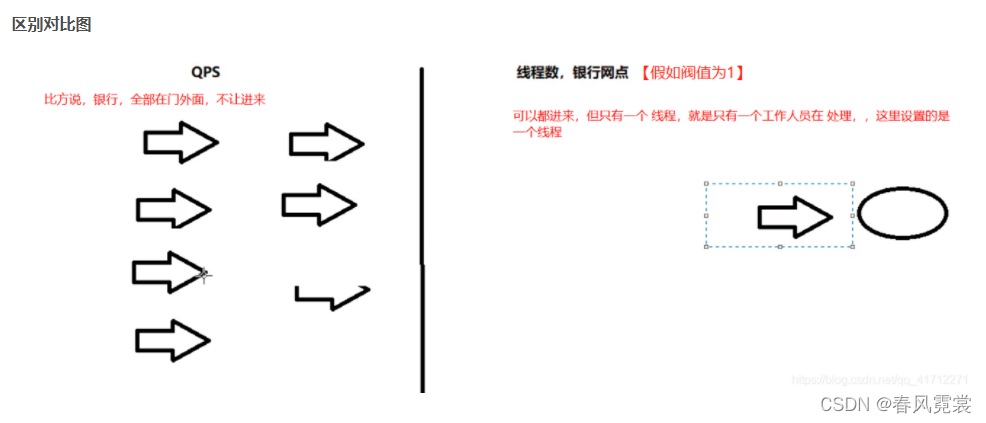
测试: 前: 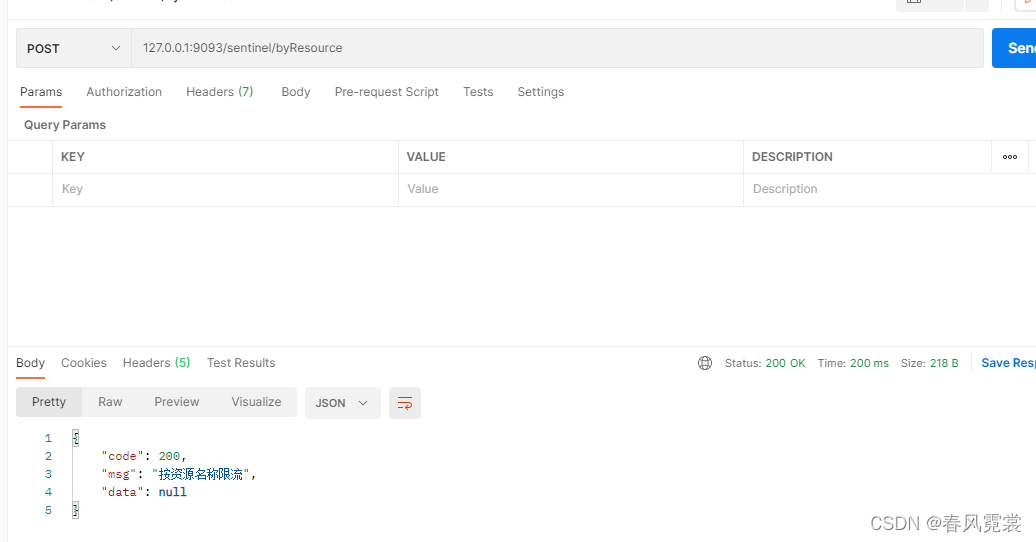 超过阈值后: 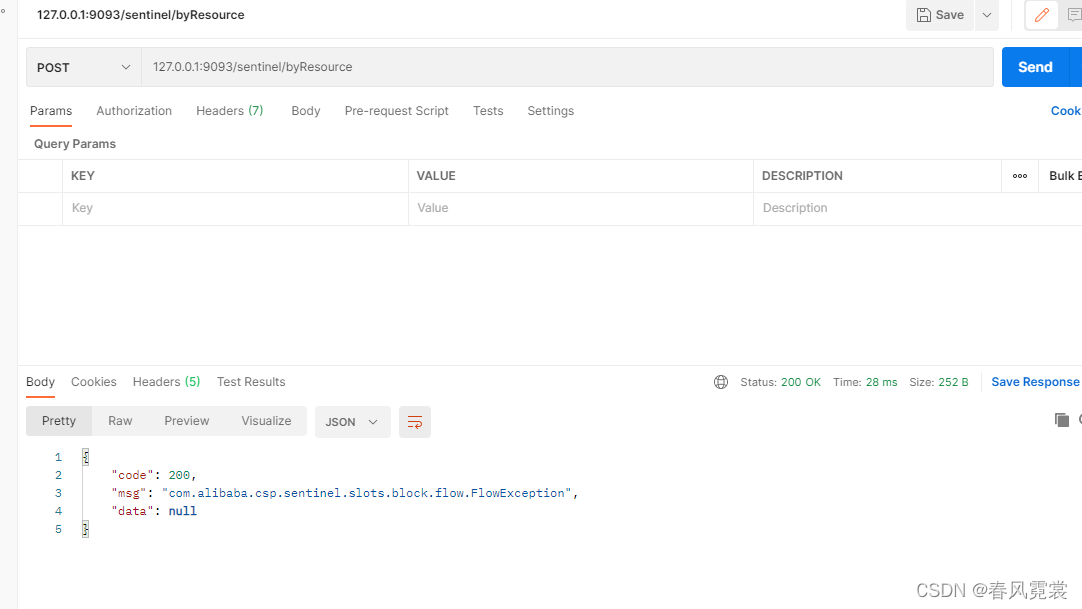
2. 按url限流,有默认的限流处理逻辑
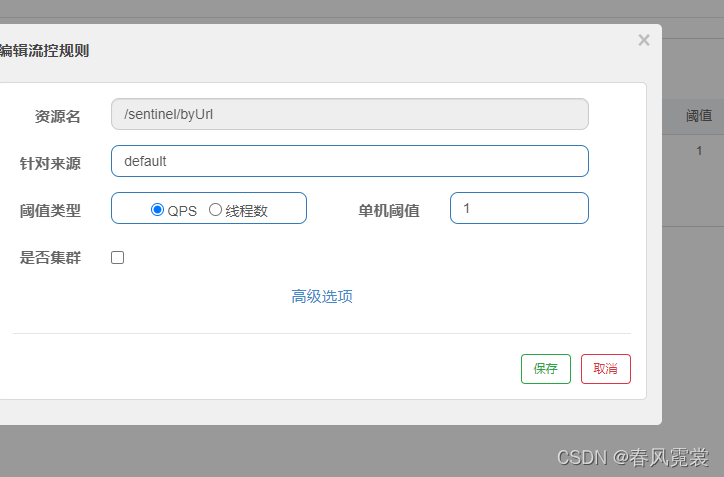
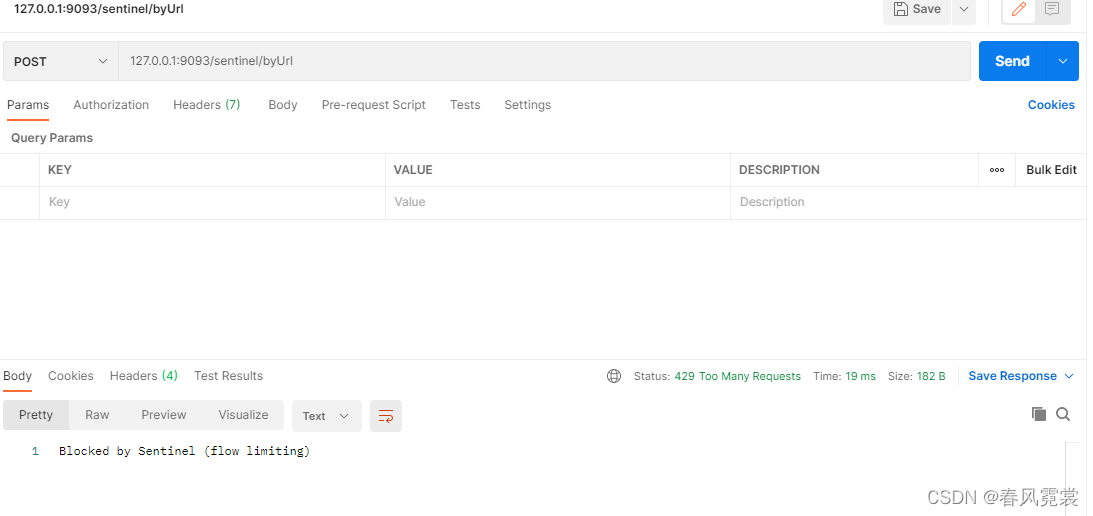
3. 自定义限流处理逻辑
配置可以自己选择,资源吗或者路径的!!! 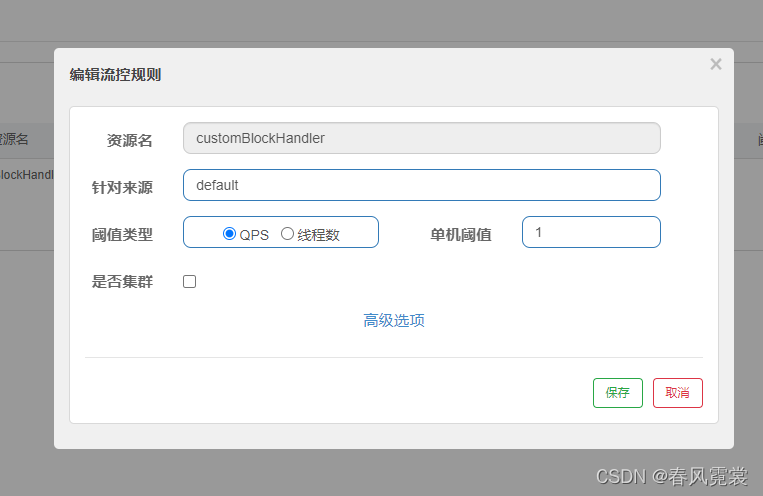
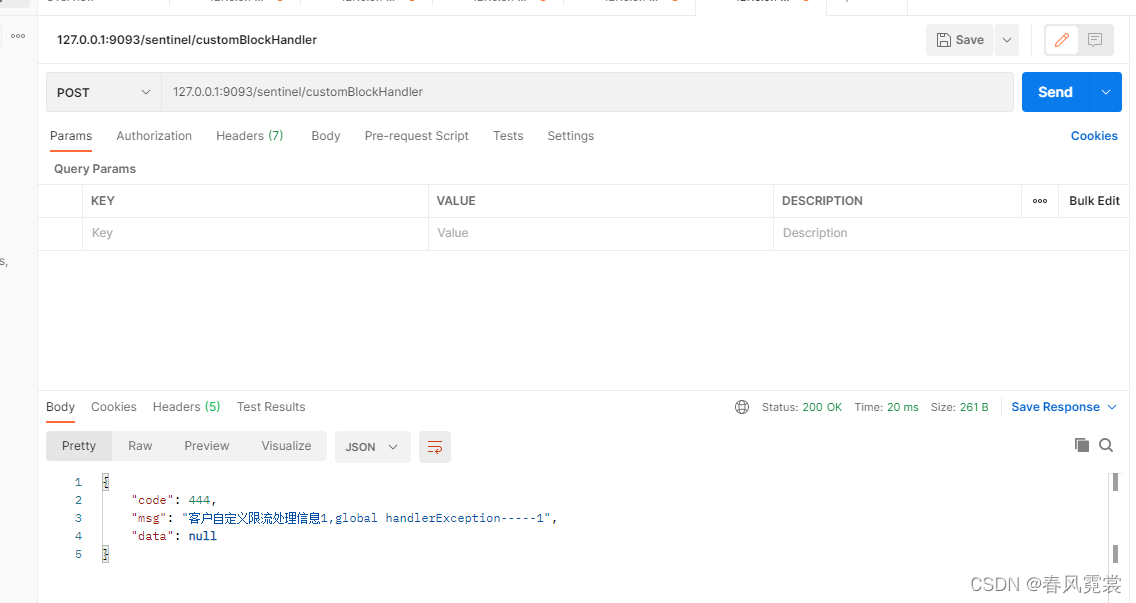
关联:
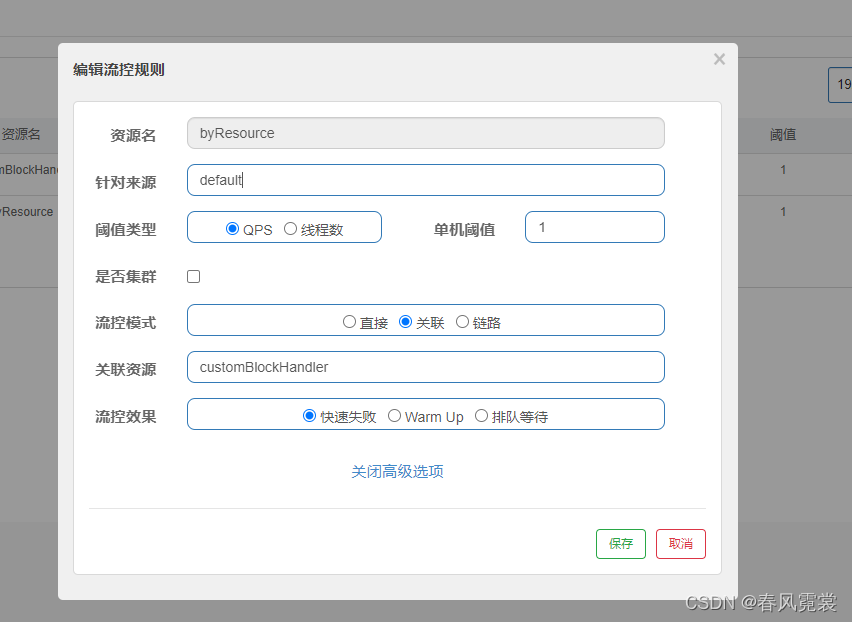
当关联的资源达到限流条件时,该资源才会开启限流 配置请求 /customBlockHandler限流QPS,关联 /byResource
当关联的资源达到阈值时customBlockHandler,会对该资源byResource进行限流
使用jmeter进行对customBlockHandler资源的访问: 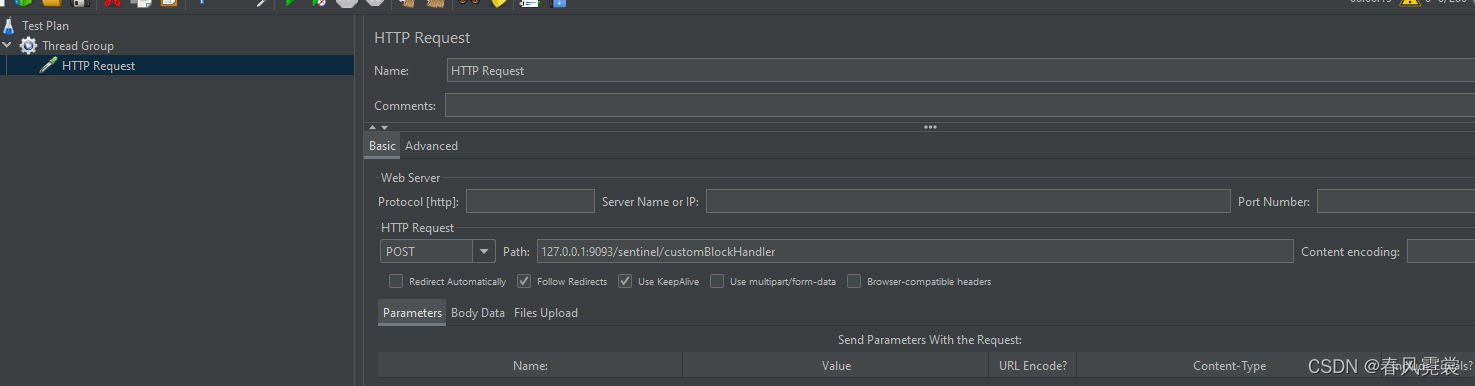
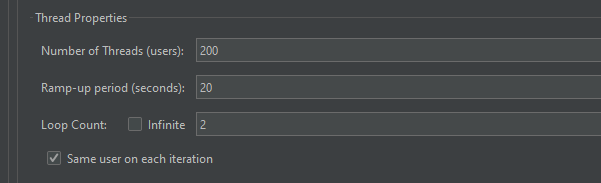
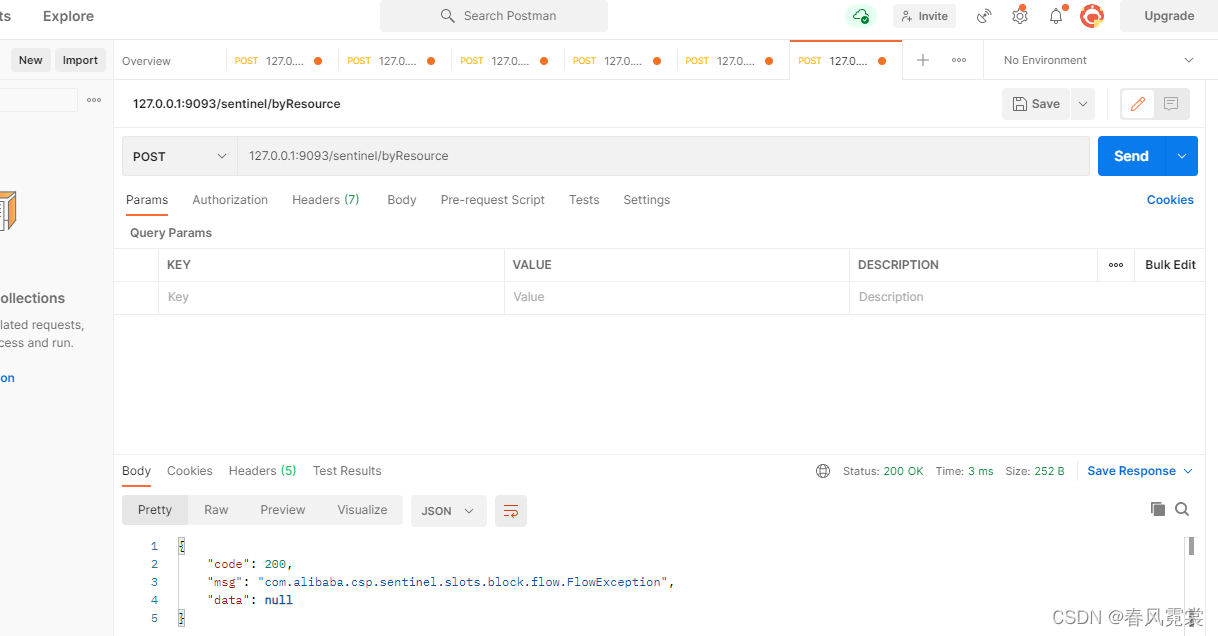
降级
1、配置rt:
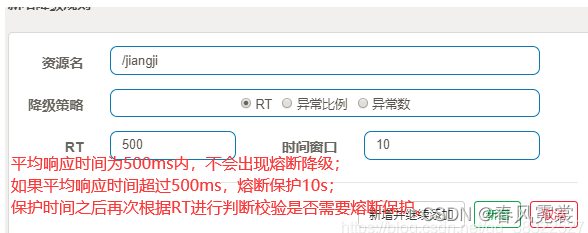
写controller测试:
package com.example.businesssentinel9093.controller;
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import com.example.businesssentinel9093.myhandler.CustomerfallbackHandler;
import com.example.dtestcommon.vo.Res;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.Date;
@RestController
@RequestMapping("/downGroud")
public class DownGrandSentinelController {
@GetMapping("/time")
public Res time(){
try {
Thread.sleep(600);
} catch (InterruptedException e) {
e.printStackTrace();
}
return Res.success("jiangji test 当前时间:"+new Date().getTime());
}
@GetMapping("/time2")
@SentinelResource(value = "time2",blockHandler = "handlerException",blockHandlerClass = CustomerfallbackHandler.class)
public Res time2(){
try {
Thread.sleep(600);
} catch (InterruptedException e) {
e.printStackTrace();
}
return Res.success("jiangji test 当前时间:"+new Date().getTime());
}
@GetMapping("/fallbackException/{id}")
@SentinelResource(value = "fallbackException", fallback = "handleFallback2", exceptionsToIgnore = {NullPointerException.class})
public Res fallbackException(@PathVariable Long id) {
if (id == 1) {
throw new IndexOutOfBoundsException();
} else if (id == 2) {
throw new NullPointerException();
}
return Res.success(1,"异常降级!!!");
}
public Res handleFallback(Long id) {
return Res.success(200,"服务降级返回1");
}
public Res handleFallback2(Long id, Throwable e) {
return Res.success(200,"服务降级返回2");
}
}
package com.example.businesssentinel9093.myhandler;
import com.alibaba.csp.sentinel.slots.block.BlockException;
import com.alibaba.csp.sentinel.slots.block.degrade.DegradeException;
import com.example.dtestcommon.vo.Res;
public class CustomerfallbackHandler {
public static Res handlerException(BlockException exception)
{
return Res.success(444,"客户自定义限流处理信息1,global handlerException-----1");
}
public static Res handlerException2(BlockException exception)
{
return Res.success(444,"客户自定义限流处理信息2,global handlerException-----2");
}
}
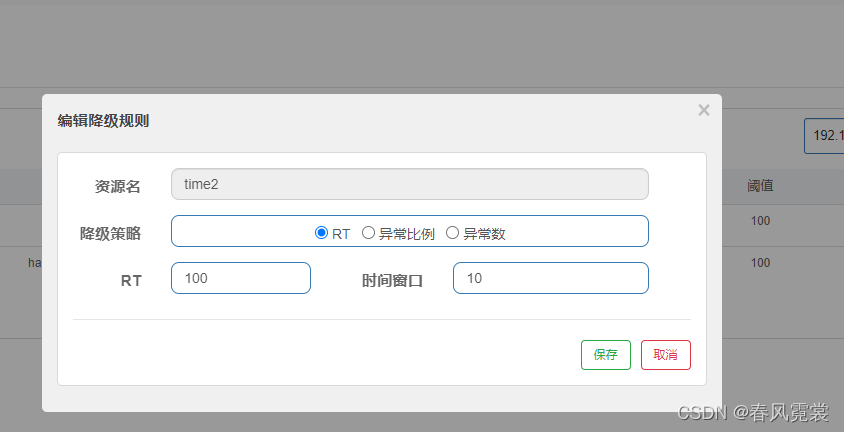
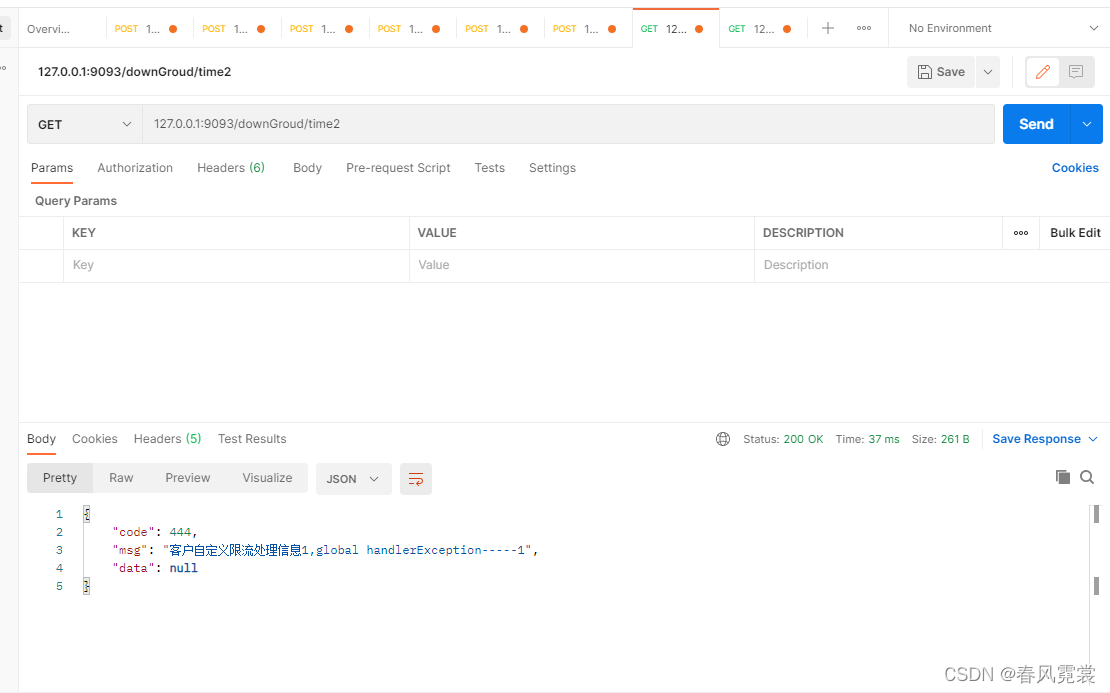
若时不用@SentinelResource注解,会使用默认的 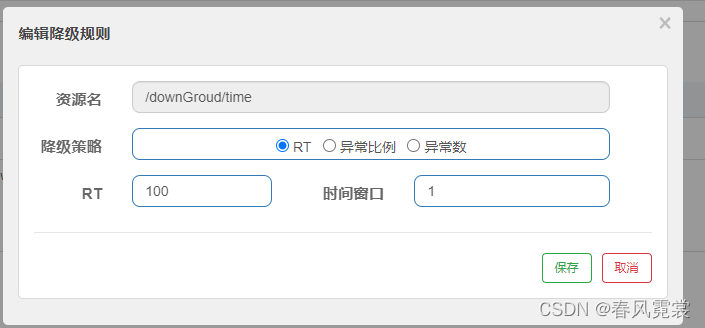
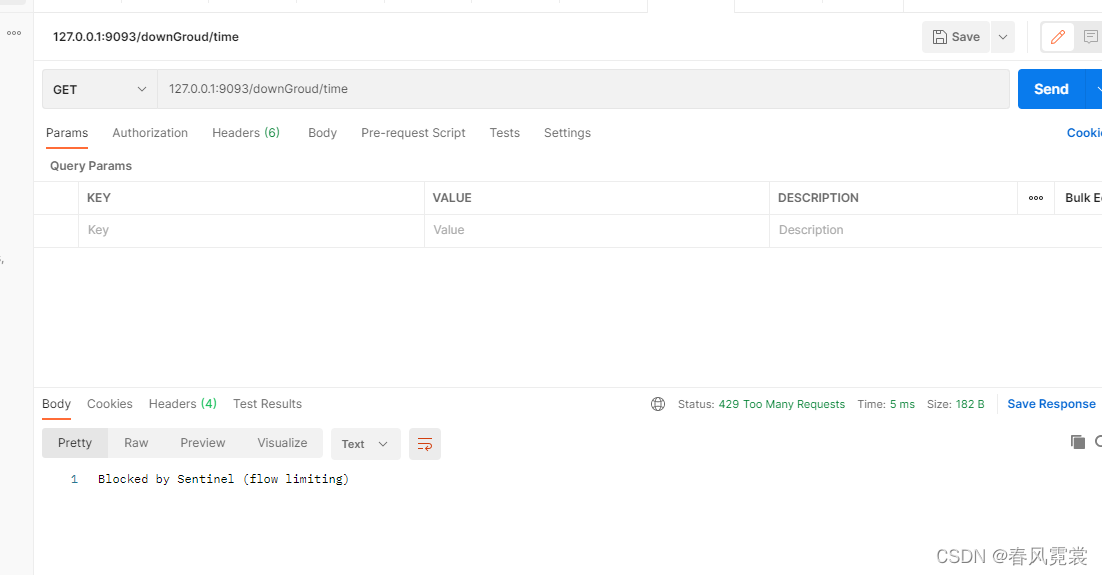
2、异常降级
package com.example.businesssentinel9093.controller;
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import com.example.businesssentinel9093.myhandler.CustomerfallbackHandler;
import com.example.dtestcommon.vo.Res;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.Date;
@RestController
@RequestMapping("/downGroud")
public class DownGrandSentinelController {
@GetMapping("/fallbackException/{id}")
@SentinelResource(value = "fallbackException", fallback = "handleFallback2", exceptionsToIgnore = {NullPointerException.class})
public Res fallbackException(@PathVariable Long id) {
if (id == 1) {
throw new IndexOutOfBoundsException();
} else if (id == 2) {
throw new NullPointerException();
}
return Res.success(1,"异常降级!!!");
}
public Res handleFallback(Long id) {
return Res.success(200,"服务降级返回1");
}
public Res handleFallback2(Long id, Throwable e) {
return Res.success(200,"服务降级返回2");
}
}
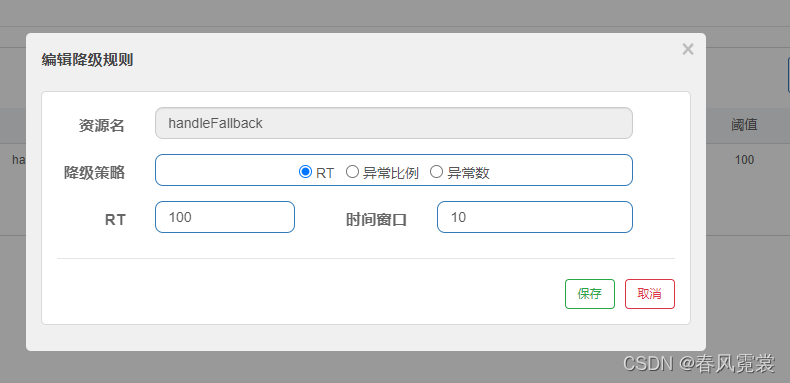 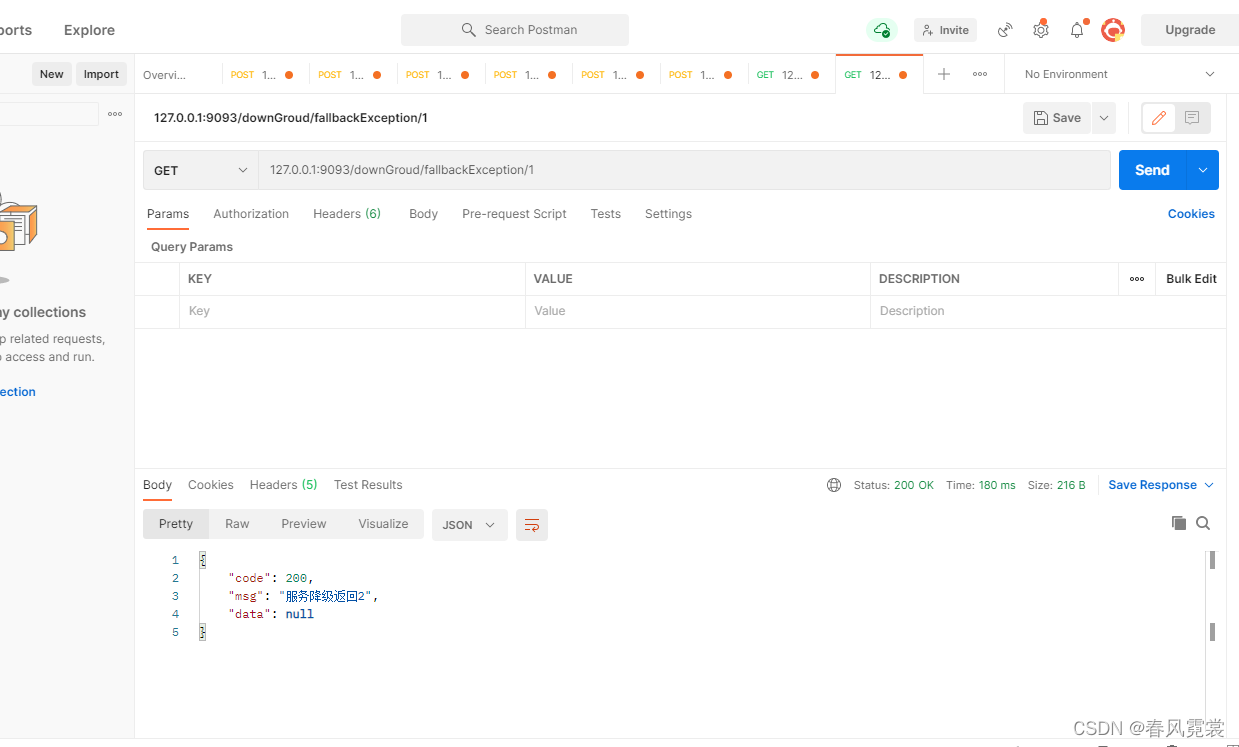
|