服务拆分成多个模块部署,由注册中心统一管理分配。 A服务需要调用B服务,需要先调用注册中心,再由注册中心找到B服务;
组合一:Dubbo+Zookeeper
-
Zookeeper 注册中心:应用程序协调服务; -
Dubbo 分布式服务框架:使各层解耦;
使用:需要使用docker安装镜像
(1)服务提供者需要进行的准备
第一步:创建一个服务提供者引入依赖
服务提供者为服务接口类
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>3.0.5</version>
</dependency>
<dependency>
<groupId>com.github.sgroschupf</groupId>
<artifactId>zkclient</artifactId>
<version>0.1</version>
</dependency>
第二步:配置相关属性
dubbo:
application:
name: provider
registry:
address: zookeeper://118.24.44.169:2181
scan:
base-packages: com.spgj.service
第三步:@Service发布服务 给需要发布的服务接口添加dubbo的@Service注解将服务发布出去,并添加@Compent声明。
(2)服务消费者需要进行的准备 第一步:引入依赖
<dependency>
<groupId>org.apache.dubbo</groupId>
<artifactId>dubbo-spring-boot-starter</artifactId>
<version>3.0.5</version>
</dependency>
<dependency>
<groupId>com.github.sgroschupf</groupId>
<artifactId>zkclient</artifactId>
<version>0.1</version>
</dependency>
第二步:配置dubbo注册中心地址
dubbo:
application:
name: customer
registry:
address: zookeeper://118.24.44.169:2181
第三步:引用服务
- 需要在消费者项目中放入和提供者全类名相同的服务接口,不需要实现内容;
- 在消费者接口中远程引用
@Reference 提供者接口对象; 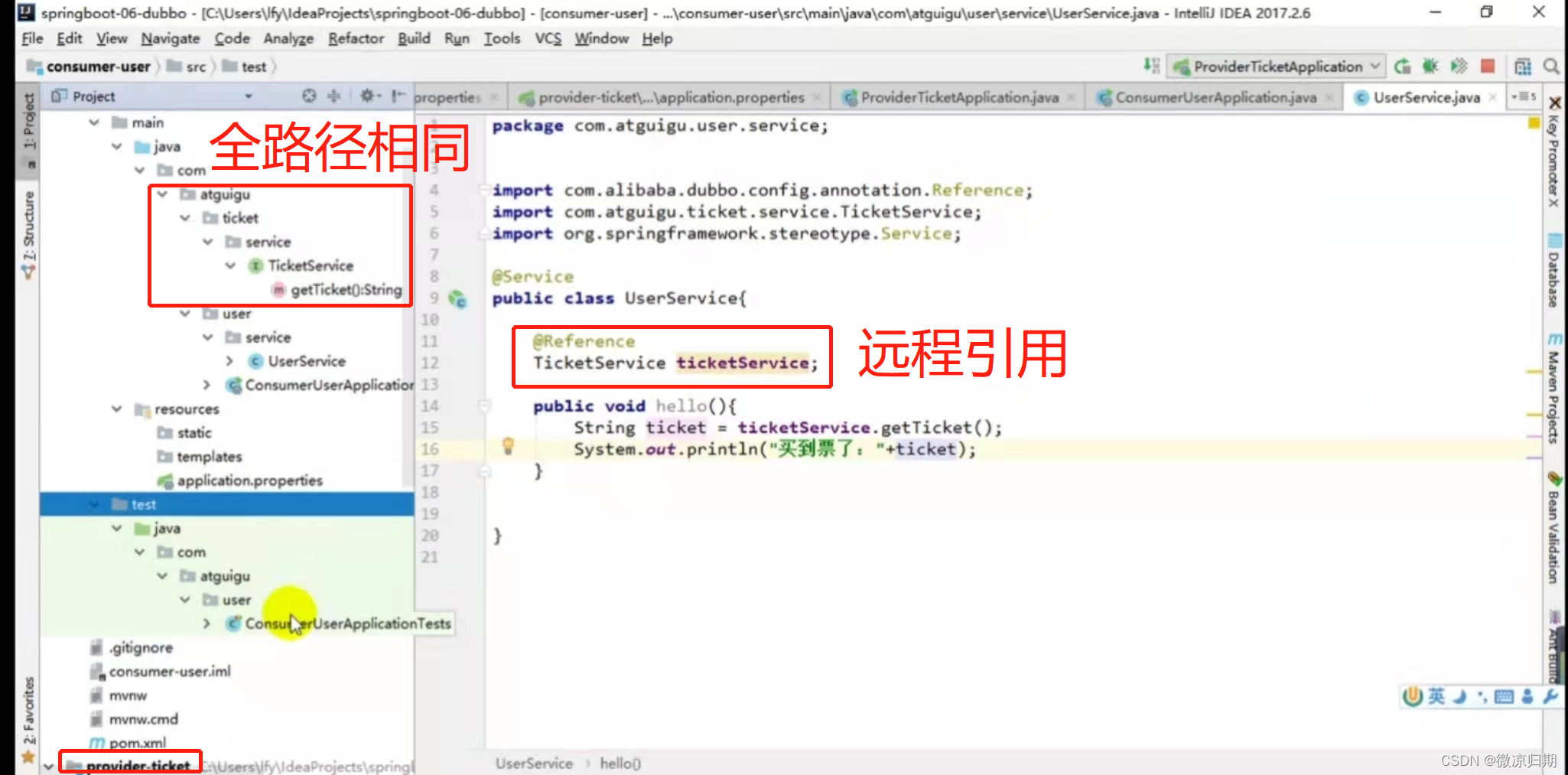
组合二:SpringBoot+Cloud
第一步:创建提供者、注册中心、消费者应用 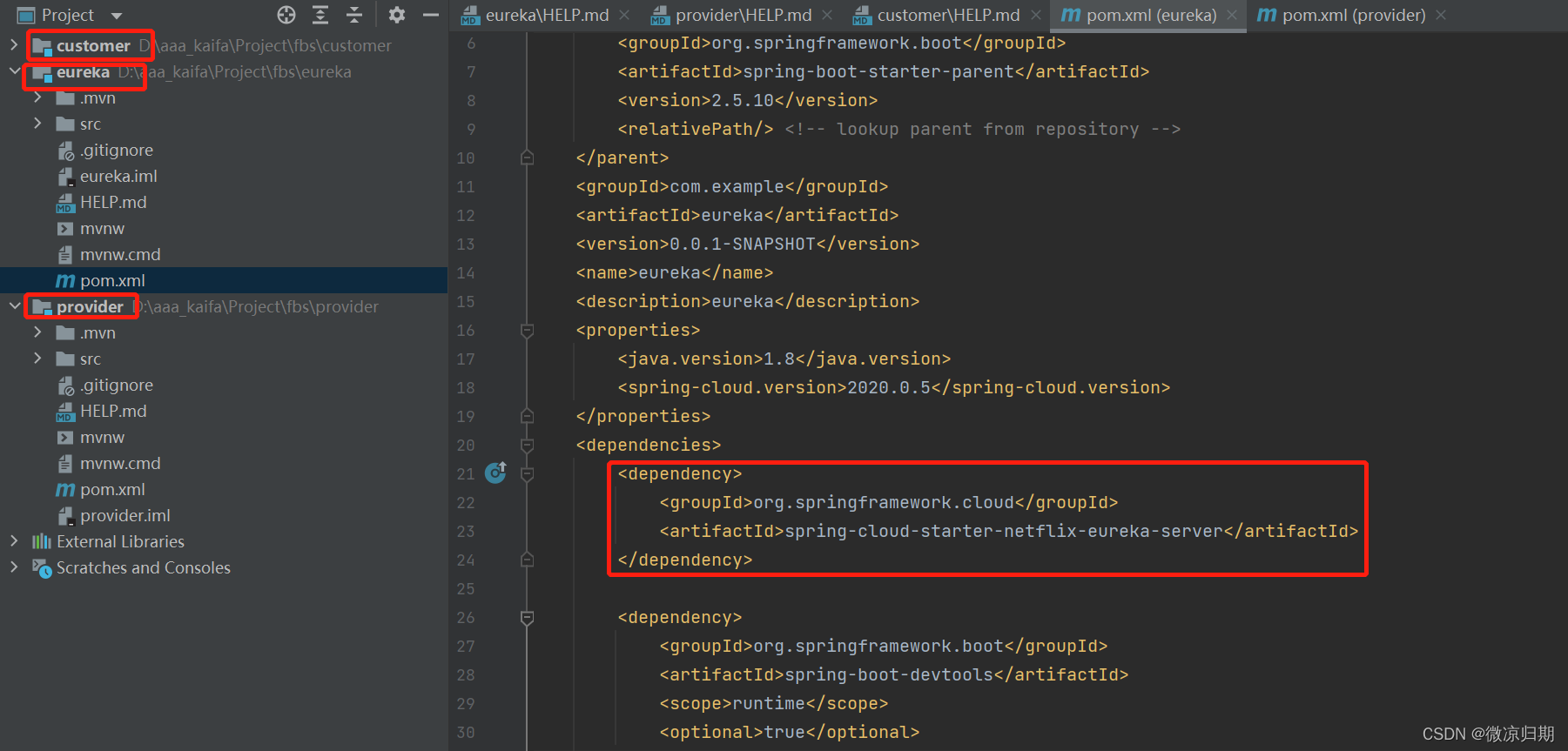
第二步:配置 (1)注册中心
eureka:
instance:
hostname: eureka
client:
register-with-eureka: false
fetch-registry: false
service-url:
defaultZone: http://localhost:8761/eureka/
@SpringBootApplication
@EnableEurekaServer
public class EurekaApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaApplication.class, args);
}
}
- 启动注册中心并访问
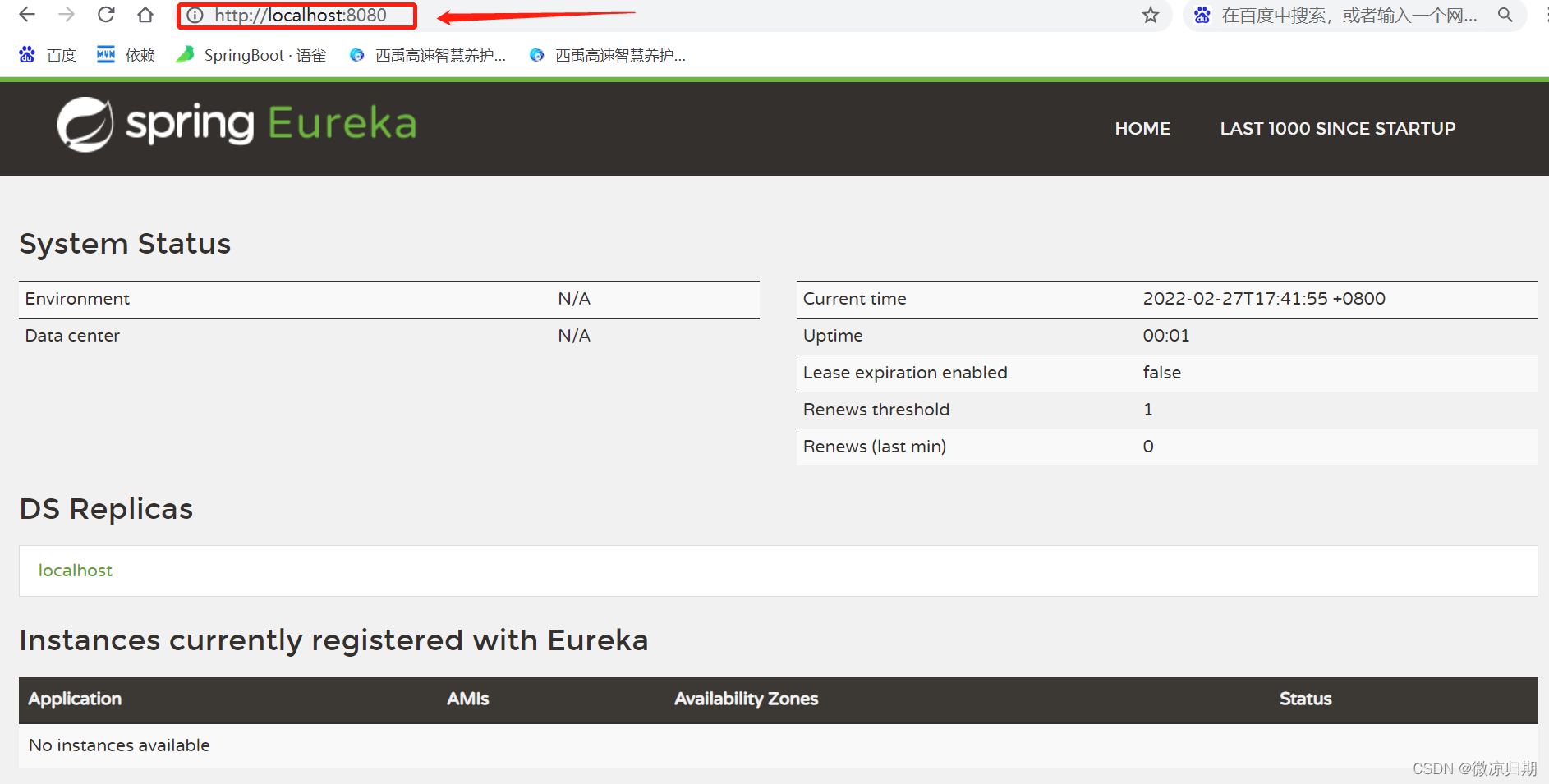
(2)服务提供者 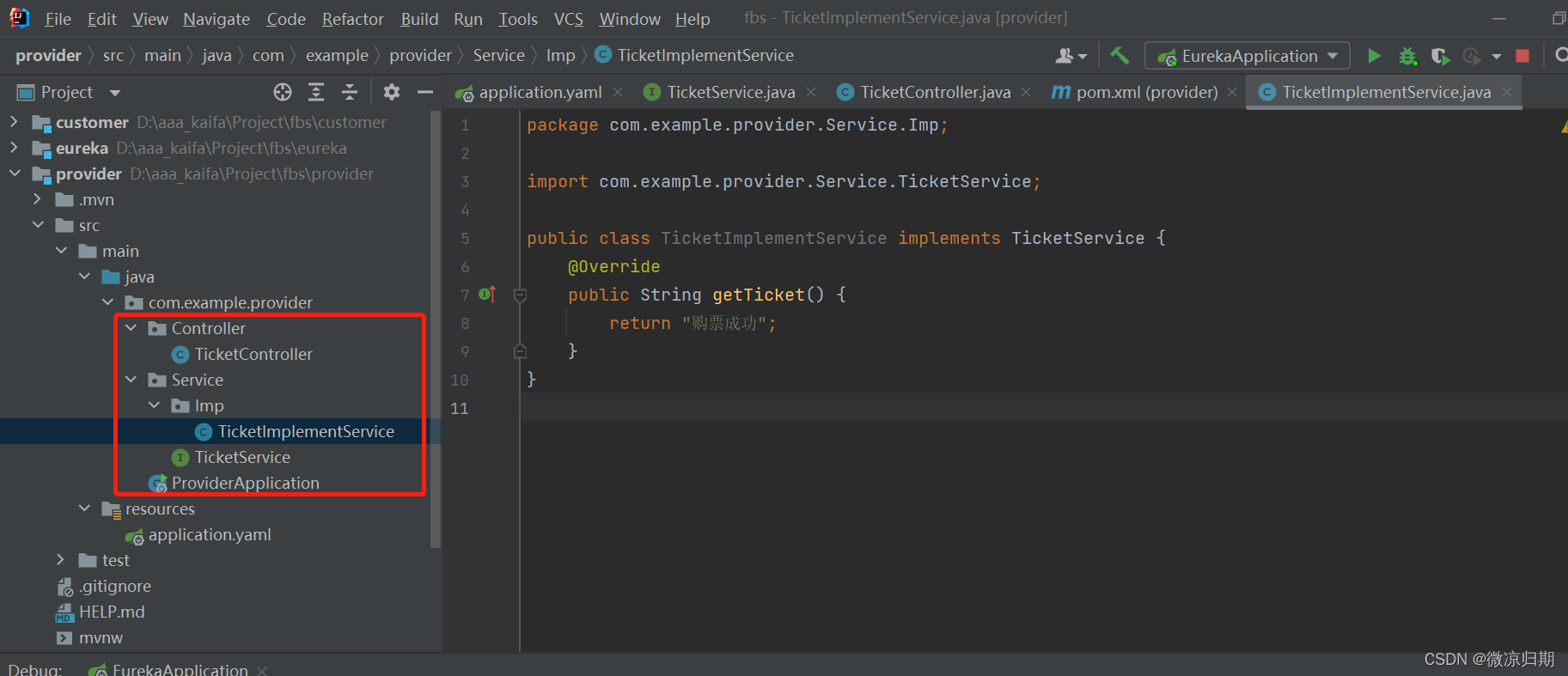
@RestController
public class TicketController {
@Autowired
TicketService ticketService;
@GetMapping("/get")
public String getTicket(){
return ticketService.getTicket();
}
}
Service
import org.springframework.stereotype.Service;
@Service
public interface TicketService {
public String getTicket();
}
ServiceImp
package com.example.provider.Service.Imp;
import com.example.provider.Service.TicketService;
public class TicketImplementService implements TicketService {
@Override
public String getTicket() {
return "购票成功";
}
}
server:
port: 8081
spring:
application:
name: provider
eureka:
instance:
prefer-ip-address: true
client:
service-url:
defaultZone: http://localhost:8761/eureka/
(3)服务消费
@RestController
public class CustomerController {
RestTemplate restTemplate;
@GetMapping("/get/ticket")
public String getTick(){
String a = restTemplate.getForObject("http://provider",String.class);
return a;
}
}
server:
port: 8083
spring:
application:
name: customer
eureka:
instance:
prefer-ip-address: true
client:
service-url:
defaultZone: http://localhost:8761/eureka
@SpringBootApplication
@EnableDiscoveryClient
public class CustomerApplication {
public static void main(String[] args) {
SpringApplication.run(CustomerApplication.class, args);
}
@Bean
public RestTemplate restTemplate(){
return new RestTemplate();
}
}
|