安装Elasticsearch-php
https://github.com/elastic/elasticsearch-php
使用composer安装:
在项目目录下,执行以下命令
composer require elasticsearch/elasticsearch
配置php.ini
配置php.ini的sys_temp_dir
;sys_temp_dir = "/tmp"
sys_temp_dir = "D:\phpstudy_pro\Extensions\tmp\tmp"
否则,使用过程中可能会出现以下报错
?
?继续composer安装:
composer require tamayo/laravel-scout-elastic
composer require laravel/scout
php artisan vendor:publish
选择:Laravel\Scout\ScoutServiceProvider
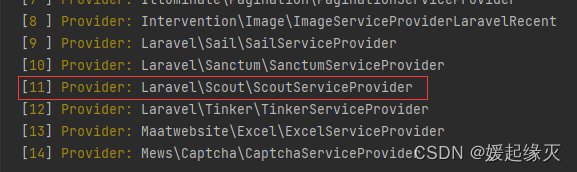
?
?修改驱动为?elasticsearch :
'driver' => env('SCOUT_DRIVER', 'elasticsearch'),
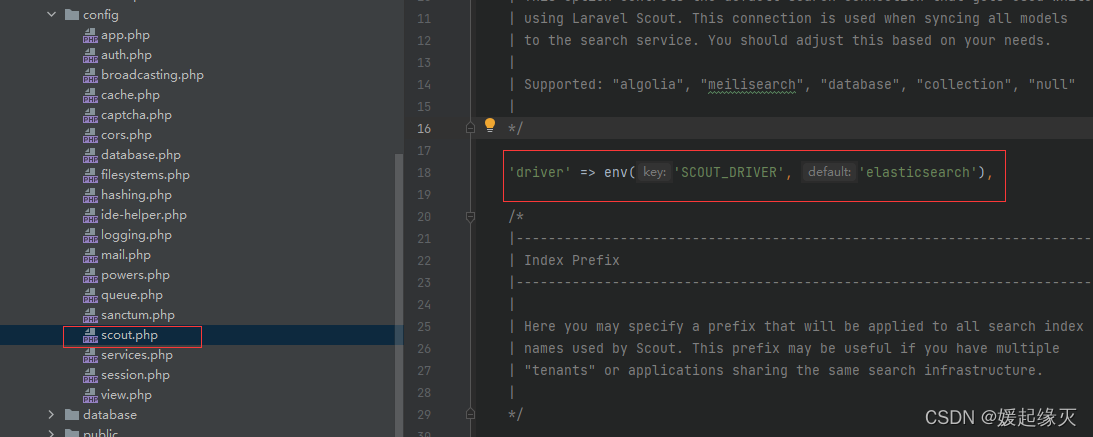
创建索引用 Laravel 自带的 Artisan 命令行功能。
php artisan make:command ESOpenCommand

?下面就可以借助插件,创建我们的 Index,直接看代码:
namespace App\Console\Commands;
use Elasticsearch\ClientBuilder;
use Illuminate\Console\Command;
public function handle()
{
$host = config('scout.elasticsearch.hosts');
$index = config('scout.elasticsearch.index');
$client = ClientBuilder::create()->setHosts($host)->build();
if ($client->indices()->exists(['index' => $index])) {
$this->warn("Index {$index} exists, deleting...");
$client->indices()->delete(['index' => $index]);
}
$this->info("Creating index: {$index}");
return $client->indices()->create([
'index' => $index,
'body' => [
'settings' => [
'number_of_shards' => 1,
'number_of_replicas' => 0
],
'mappings' => [
'_source' => [
'enabled' => true
],
'properties' => [
'id' => [
'type' => 'long'
],
'title' => [
'type' => 'text',
'analyzer' => 'ik_max_word',
'search_analyzer' => 'ik_smart'
],
'subtitle' => [
'type' => 'text',
'analyzer' => 'ik_max_word',
'search_analyzer' => 'ik_smart'
],
'content' => [
'type' => 'text',
'analyzer' => 'ik_max_word',
'search_analyzer' => 'ik_smart'
]
],
]
]
]);
}
?创建mapping
php artisan esoc:mapping
Laravel Model 使用
Laravel 框架已经为我们推荐使用 Scout 全文搜索,我们只需要在 Article Model 加上官方所说的内容即可,很简单,推荐大家看 Scout 使用文档:Scout 全文搜索《Laravel 6 中文文档》,下面直接上代码:
Scout 全文搜索 | 官方扩展包 |《Laravel 6 中文文档 6.x》| Laravel China 社区 (learnku.com)
Scout 提供了 Artisan 命令 import 用来导入所有已存在的记录到搜索索引中。
php artisan scout:import "App\Article"
<?php
namespace App;
use App\Tools\Markdowner;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Database\Eloquent\SoftDeletes;
use Laravel\Scout\Searchable;
class Article extends Model
{
use Searchable;
protected $connection = 'blog';
protected $table = 'articles';
use SoftDeletes;
/**
* The attributes that should be mutated to dates.
*
* @var array
*/
protected $dates = ['published_at', 'created_at', 'deleted_at'];
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'user_id',
'last_user_id',
'category_id',
'title',
'subtitle',
'slug',
'page_image',
'content',
'meta_description',
'is_draft',
'is_original',
'published_at',
'wechat_url',
];
protected $casts = [
'content' => 'array'
];
/**
* Set the content attribute.
*
* @param $value
*/
public function setContentAttribute($value)
{
$data = [
'raw' => $value,
'html' => (new Markdowner)->convertMarkdownToHtml($value)
];
$this->attributes['content'] = json_encode($data);
}
/**
* 获取模型的可搜索数据
*
* @return array
*/
public function toSearchableArray()
{
$data = [
'id' => $this->id,
'title' => $this->title,
'subtitle' => $this->subtitle,
'content' => $this->content['html']
];
return $data;
}
public function searchableAs()
{
return '_doc';
}
}
有了数据,我们可以测试看看能不能查询到数据。
还是一样的,创建一个命令:
php artisan make:command ElasearchCommand
class ElasearchCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'command:search {query}';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Create a new command instance.
*
* @return void
*/
public function __construct()
{
parent::__construct();
}
/**
* Execute the console command.
*
* @return mixed
*/
public function handle()
{
$article = Article::search($this->argument('query'))->first();
$this->info($article->title);
}
}
?IK 分词器安装
?Releases · medcl/elasticsearch-analysis-ik · GitHub
?总结:
Elasticsearch 安装; Elasticsearch IK 分词器插件安装; Elasticsearch 可视化工具 ElasticHQ 和 Kibana 的安装和简单使用; Scout 的使用; Elasticsearch 和 Scout 结合使用。
Laravel + Elasticsearch 实现中文搜索 | Laravel China 社区 (learnku.com)
|