定义sqlsugar的helper
使用文档:
https://www.donet5.com/Home/Doc?typeId=1206
using SqlSugar;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace sugarTest.Provider
{
public class SqlSugarHelper
{
public static string ConnectionString = string.Empty;
public static SqlSugarClient db
{
get => new SqlSugarClient(new ConnectionConfig()
{
ConnectionString = ConnectionString,
DbType = DbType.Sqlite,
IsAutoCloseConnection = true,
InitKeyType = InitKeyType.Attribute
});
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace sugarTest.Provider
{
public class SqlSugarService
{
public static void SetConnectionStr(string ConnectionStr)
{
SqlSugarHelper.ConnectionString = ConnectionStr;
}
}
}
定义两个实体类
using SqlSugar;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace sugarTest.Model
{
public class Order
{
[SugarColumn(IsPrimaryKey = true, IsIdentity = true)]
public int Id { get; set; }
public string Name { get; set; }
public string Descript { get; set; }
[SugarColumn(IsIgnore = true)]
public List<OrderItem> OrderItems { get; set; } = new List<OrderItem>();
}
}
using SqlSugar;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace sugarTest.Model
{
public class OrderItem
{
[SugarColumn(IsPrimaryKey = true, IsIdentity = true)]
public int Id { get; set; }
public string ItemCode { get; set; }
public decimal Pirce { get; set; }
public int OrderId { get; set; }
[SugarColumn(IsIgnore = true)]
public Order Order { get; set; }
}
}
实现一对多查询
using sugarTest.Model;
using sugarTest.Provider;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace sugarTest
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
this.Load += Form1_Load;
}
private void Form1_Load(object sender, EventArgs e)
{
SqlSugarService.SetConnectionStr("Data Source=" + Application.StartupPath + str2);
}
string str2 = "\\Config\\Test.db;Pooling=true;FailIfMissing=false";
private void btnQuery_Click(object sender, EventArgs e)
{
SugarResponsitory sugarResponsitory = new SugarResponsitory();
var list = sugarResponsitory.Db.Queryable<Order>().
Mapper(it => it.OrderItems, it => it.OrderItems.First().OrderId).Where(it=>it.Id>2).ToList();
this.DGV.DataSource = list;
}
private void btnCreateDataBase_Click(object sender, EventArgs e)
{
SugarResponsitory sugarResponsitory = new SugarResponsitory();
sugarResponsitory.Db.CodeFirst.SetStringDefaultLength(200).InitTables(typeof(OrderItem));
sugarResponsitory.Db.CodeFirst.SetStringDefaultLength(200).InitTables(typeof(Order));
}
}
}
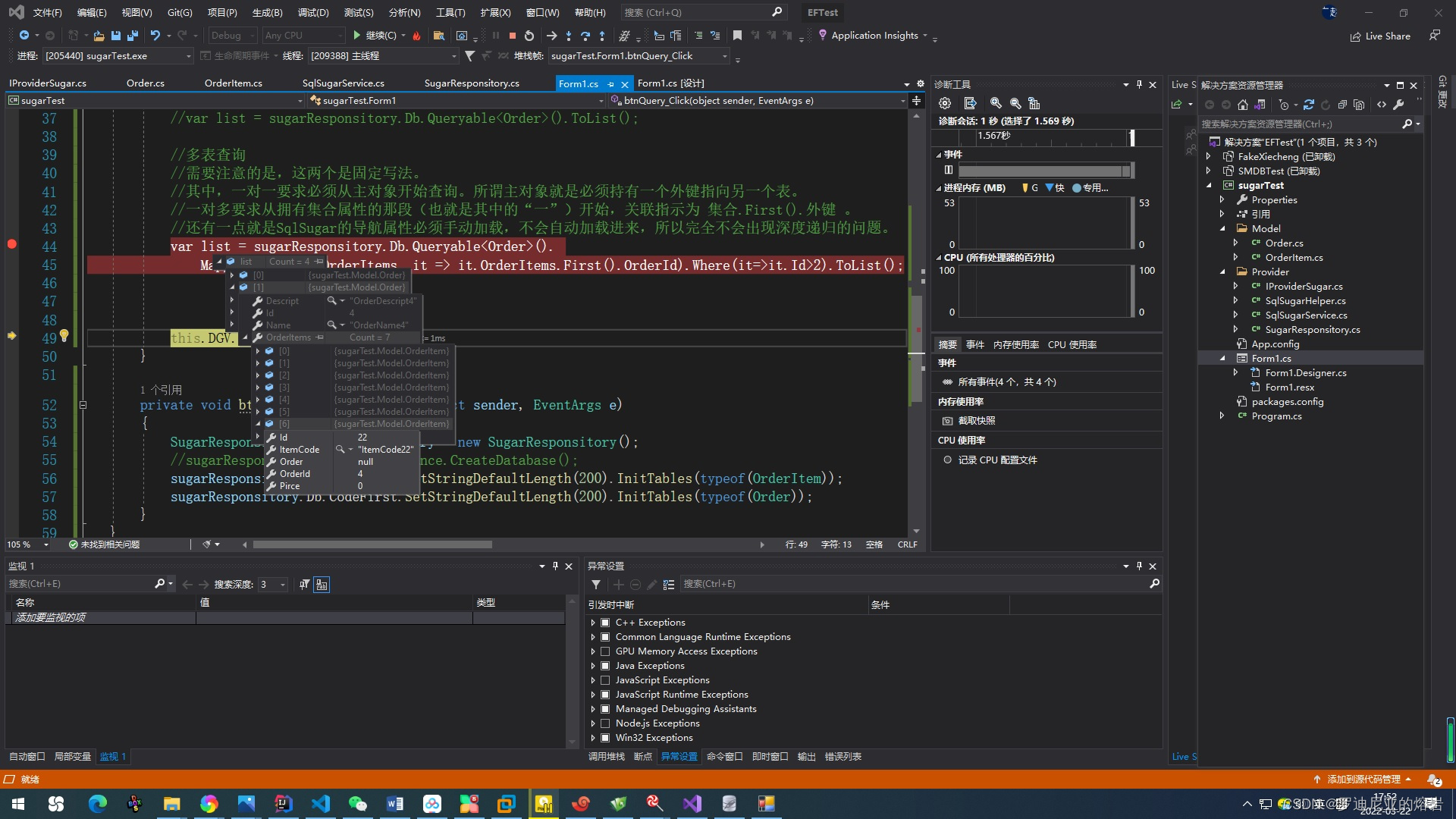
|