一、什么是事务?
在人员管理系统中,你删除一个人员,你即需要删除人员的基本资料,也要删除和该人员相关的信息,如信箱,文章等等,这样,这些数据库操作语句就构成一个事务!
二、事务是必须满足4个条件(ACID)
事务的原子性( Atomicity):一组事务,要么成功;要么撤回。 一致性 (Consistency):事务执行后,数据库状态与其他业务规则保持一致。如转账业务,无论事务执行成功否,参与转账的两个账号余额之和应该是不变的。 隔离性(Isolation):事务独立运行。一个事务处理后的结果,影响了其他事务,那么其他事务会撤回。事务的100%隔离,需要牺牲速度。 持久性(Durability):软、硬件崩溃后,InnoDB数据表驱动会利用日志文件重构修改。可靠性和高速度不可兼得, innodb_flush_log_at_trx_commit 选项 决定什么时候吧事务保存到日志里。
三、Connection的三个方法与事务有关:
setAutoCommit(boolean):设置是否为自动提交事务,如果true(默认值为true)表示自动提交,也就是每条执行的SQL语句都是一个单独的事务,如果设置为false,那么相当于开启了事务了;con.setAutoCommit(false) 表示手动开启事务。 commit():提交结束事务。 rollback():回滚结束事务。
四、事务示例
- 表
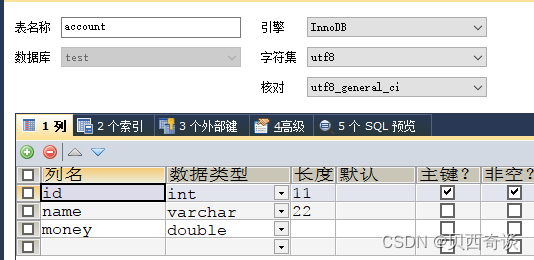 - 代码
public static void tranc(){
Connection conn=null;
PreparedStatement pst=null;
try {
conn = JdbcUtil.getConnection();
conn.setAutoCommit(false);
String sql1="update account set money=money-1000 where name='张三'";
pst = conn.prepareStatement(sql1);
pst.executeUpdate();
String sql2="update1 account set money=money+1000 where name='李四'";
pst = conn.prepareStatement(sql2);
pst.executeUpdate();
}catch (Exception e){
try {
conn.rollback();
}catch (SQLException e1){
}
e.printStackTrace();
}finally {
try {
conn.commit();
JdbcUtil.myClose(conn,pst);
}catch (SQLException e1){
}
}
}
五、完整登陆、注册案例
public void login(String name, String pwd){
Connection conn= null;
PreparedStatement pst=null;
try {
conn = JdbcUtil.getConnection();
String sql="select * from user where name=? and password=?";
pst = conn.prepareStatement(sql);
pst.setString(1, name);
pst.setString(2, pwd);
ResultSet rs = pst.executeQuery();
if(rs.next()){
System.out.println("登陆成功!");
}else{
System.out.println("用户名或者密码错误!");
}
}catch (Exception e){
JdbcUtil.myClose(conn,pst);
}
}
Connection conn = JdbcUtil.getConnection();
public void register(String name,String password){
PreparedStatement pst=null;
try {
boolean flag = nameExists(name);
if(flag){
return;
}else{
String sql="insert into user(name,password) values (?,?)";
pst = conn.prepareStatement(sql);
pst.setString(1, name);
pst.setString(2, password);
int i = pst.executeUpdate();
if(i>0){
System.out.println("注册成功!");
}
}
}catch (Exception e){
}finally {
JdbcUtil.myClose(conn, pst);
}
}
public boolean nameExists(String name){
try {
String sql="select * from user where name=?";
PreparedStatement pst = conn.prepareStatement(sql);
pst.setString(1, name);
ResultSet rs = pst.executeQuery();
if(rs.next()){
System.out.println("用户名已经存在,不允许在注册!");
return true;
}
}catch (Exception e){
}
return false;
}
|