SpringBoot 集成 ES 使用API(一)
创建Client客户端
@Configuration
public class ESConfig {
@Bean
public RestHighLevelClient restHighLevelClient(){
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(
new HttpHost("127.0.0.1", 9200, "http")
)
);
return client;
}
}
测试类
@SpringBootTest
class EsApiApplicationTests {
@Autowired
@Qualifier("restHighLevelClient")
private RestHighLevelClient client;
}
索引API 使用
- 创建索引
@Test
void testCreatedIndex() throws IOException {
CreateIndexRequest request = new CreateIndexRequest("caw_index");
CreateIndexResponse createIndexResponse = client.indices().create(request, RequestOptions.DEFAULT);
System.out.println(createIndexResponse);
}
- 删除索引
@Test
void textDeleteIndex() throws IOException {
DeleteIndexRequest request = new DeleteIndexRequest("caw_index");
AcknowledgedResponse delete = client.indices().delete(request, RequestOptions.DEFAULT);
System.out.println(delete.isAcknowledged());
}
- 判断索引是否存在
@Test
void textExistIndex() throws IOException {
GetIndexRequest request = new GetIndexRequest("caw_index");
boolean exists = client.indices().exists(request, RequestOptions.DEFAULT);
System.out.println(exists);
}
文档API 使用
- 创建文档
@Test
void creteDocument() throws IOException {
User user = new User("李四", 34);
IndexRequest request = new IndexRequest("caw_index");
request.id("3");
request.timeout(TimeValue.timeValueSeconds(1));
request.timeout("1s");
request.source(JSON.toJSONString(user), XContentType.JSON);
IndexResponse response = client.index(request, RequestOptions.DEFAULT);
System.out.println(response.toString());
System.out.println(response.status());
}
- 查看文档
@Test
void testGetDocument() throws IOException {
GetRequest request = new GetRequest("caw_index","1");
GetResponse response = client.get(request, RequestOptions.DEFAULT);
System.out.println(response.getSourceAsString());
System.out.println(response);
}
- 判断文档是否存在
@Test
void testIsExists() throws IOException {
GetRequest request = new GetRequest("caw_index", "1");
request.fetchSourceContext(new FetchSourceContext(false));
boolean exists = client.exists(request, RequestOptions.DEFAULT);
System.out.println(exists);
}
- 修改文档
@Test
void testUpdateDocument() throws IOException {
UpdateRequest request = new UpdateRequest("caw_index", "1");
User user = new User("李四学java",23);
request.doc(JSON.toJSONString(user), XContentType.JSON);
UpdateResponse response = client.update(request, RequestOptions.DEFAULT);
System.out.println(response.status());
}
- 删除文档
@Test
void testDeleteDocument() throws IOException {
DeleteRequest request = new DeleteRequest("caw_index", "id");
request.timeout("1s");
DeleteResponse response = client.delete(request, RequestOptions.DEFAULT);
System.out.println(response.status());
}
- 批量操作文档
@Test
void testBulkDocument() throws IOException {
BulkRequest request = new BulkRequest();
request.timeout("10s");
ArrayList<User> list = new ArrayList<>();
list.add(new User("请求", 11));
list.add(new User("亲戚", 13));
list.add(new User("融入", 10));
list.add(new User("一哦", 23));
list.add(new User("一样", 45));
list.add(new User("看看", 67));
list.add(new User("到底", 3));
for (int i = 0; i < list.size(); i++) {
request.add(new IndexRequest("caw_index")
.id(""+i)
.source(JSON.toJSONString(list.get(i)), XContentType.JSON));
}
client.bulk(request, RequestOptions.DEFAULT);
}
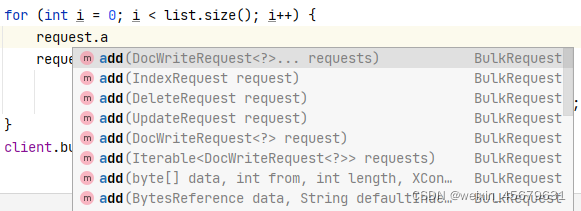 BulkRequest可执行多种操作 具体New 相对应的请求
- 文档集合查询
@Test
void testSearchDocument() throws IOException {
SearchRequest request = new SearchRequest("caw_index");
SearchSourceBuilder builder = new SearchSourceBuilder();
MatchAllQueryBuilder allQuery = QueryBuilders.matchAllQuery();
builder.query(allQuery);
builder.from(0);
builder.size(5);
builder.sort("age", SortOrder.DESC);
builder.timeout(new TimeValue(60, TimeUnit.SECONDS));
request.source(builder);
SearchResponse search = client.search(request, RequestOptions.DEFAULT);
System.out.println(JSON.toJSONString(search.getHits()));
System.out.println("=========================");
ArrayList<Object> list = new ArrayList<>();
for (SearchHit hit: search.getHits().getHits()) {
list.add(hit.getSourceAsString());
}
System.out.println(list);
}
|