客户关系系统:
该软件能够实现对客户对象的插入,修改和删除(用切片实现),并能够打印客户明细表
MVC架构,V--视图层 ,c--controller--控制层 M-model 连接数据库
MVC不展开讲,简要分析
V---视图---相关内容都是写在视图层这里
c--控制层【接口层】,相应调取的接口都是在这里编写
M-数据存储层--跟数据库相关的信息都是在这里编写
程序层级关系截图
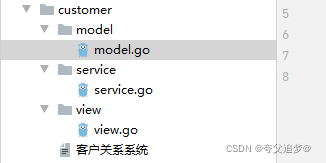
1-model层代码
package model
import "fmt"
//创建客户信息结构体
type Customer struct {
Id int
Name string
Gender string
Age int
Phone string
Email string
}
//提供工厂模式,返回Customer
func NewCustomer(id int,name string,gender string,age int,phone string,email string) Customer {
customer := Customer{
id,
name,
gender,
age,
phone,
email,
}
return customer
}
//提供工厂模式,返回Customer,不携带id
func NewCustomerNotID(name string,gender string,age int,phone string,email string) Customer {
customer := Customer{
Name:name,
Gender:gender,
Age:age,
Phone:phone,
Email:email,
}
return customer
}
//返回用户信息
func (this Customer) GetInfo() string {
info := fmt.Sprintf("%v\t\t%v\t%v\t\t%v\t\t%v\t\t%v\t",this.Id,this.Name,this.Gender,this.Age,this.Phone,this.Email)
return info
}
2.接口层---c--控制层? service.go代码
package service
import (
"fmt"
"homeaccount/customer/model"
)
//增删改查
type CustomerService struct {
Customers []model.Customer
//表示当前切片有多少客户
customerNum int
}
//创建一个工厂模式,返回CustomerService实例
func NewCustomerService() *CustomerService {
customerService := CustomerService{}
customerService.customerNum = 1
customer := model.NewCustomer(1, "老王", "男", 20, "666", "111111@qq.com")
customerService.Customers = append(customerService.Customers, customer)
return &customerService
}
//返回客户切片
func (this *CustomerService) List() []model.Customer {
return this.Customers
}
//添加客户到customers中
func (this *CustomerService) Add(customer model.Customer) bool {
//将添加顺序作为客户id
this.customerNum++
customer.Id = this.customerNum
this.Customers = append(this.Customers, customer)
return true
}
//根据id查找客户下标,没有就返回一个-1
func (this *CustomerService) FindById(id int) int {
index := -1
//遍历切片
for key, _ := range this.Customers {
if this.Customers[key].Id == id{
index = key
}
}
return index
}
//根据id删除客户
func (this *CustomerService) Delete(id int) bool {
index := this.FindById(id)
//判断index是否为-1
if index == -1 {
return false
} else {
this.Customers = append(this.Customers[:index],this.Customers[index+1:]...)
return true
}
}
//修改客户
func (this *CustomerService) Update(id int,customers model.Customer) bool {
index := this.FindById(id)
if index == -1 {
return false
} else {
customer := this.Customers[index]
fmt.Println(len(customers.Name))
if len(customers.Name) == 0 {
customers.Name = customer.Name
}
if len(customers.Gender) == 0 {
customers.Gender = customer.Gender
}
if customers.Age == 0 {
customers.Age = customer.Age
}
if len(customers.Phone) == 0 {
customers.Phone = customer.Phone
}
if len(customers.Email) == 0 {
customers.Email = customer.Email
}
customer = customers
customer.Id = id
this.Customers[index] = customer
return true
}
}
3.view.go视图层代码
package main
import (
"fmt"
"homeaccount/customer/model"
"homeaccount/customer/service"
)
type CustomerView struct {
//用于菜单选项
key string
//用于退出程序
loop bool
//用于添加客户名
name string
//用于添加客户性别
gender string
//用于添加客户年龄
age int
//用于添加客户电话
phone string
//用于添加客户邮箱号
email string
//用于删除客户的id
id int
//用于删除客户的确认
choice string
customerService *service.CustomerService
}
//显示所有客户信息
func (this *CustomerView) List() {
list := this.customerService.List()
fmt.Println("----------客户列表----------")
fmt.Println("编号\t姓名\t性别\t年龄\t电话\t邮箱")
for key, _ := range list {
fmt.Println(list[key].GetInfo())
}
fmt.Println("----------客户列表完成----------")
}
//添加用户
func (this *CustomerView) Add() {
fmt.Println("----------添加客户----------")
fmt.Println("姓名:")
fmt.Scanln(&this.name)
fmt.Println("性别:")
fmt.Scanln(&this.gender)
fmt.Println("年龄:")
fmt.Scanln(&this.age)
fmt.Println("电话:")
fmt.Scanln(&this.phone)
fmt.Println("邮箱号:")
fmt.Scanln(&this.email)
//id是唯一的,由系统分配
costomer := model.NewCustomerNotID(this.name,this.gender,this.age,this.phone,this.email)
if this.customerService.Add(costomer){
fmt.Println("----------添加成功----------")
} else {
fmt.Println("----------添加失败----------")
}
}
//删除客户
func (this *CustomerView) Delete() {
fmt.Println("----------删除客户----------")
fmt.Print("请选择要删除的客户编号(-1退出):")
fmt.Scanln(&this.id)
if this.id == -1 {
return
}
//判断是否确认删除
for {
fmt.Println("确认是否删除(y/n):")
fmt.Scanln(&this.choice)
if this.choice == "Y" || this.choice == "y"{
if this.customerService.Delete(this.id){
fmt.Println("----------删除成功----------")
return
}else {
fmt.Println("----------删除失败,id不存在----------")
return
}
} else if this.choice == "n" || this.choice == "N" {
fmt.Println("成功取消删除")
return
} else {
fmt.Println("请输入正确的选项")
}
}
}
//修改客户
func (this *CustomerView) Update() {
fmt.Println("----------修改客户----------")
fmt.Print("请选择要修改的客户编号(-1退出):")
fmt.Scanln(&this.id)
if this.id == -1 {
return
}
fmt.Println("姓名:")
fmt.Scanln(&this.name)
fmt.Println("性别:")
fmt.Scanln(&this.gender)
fmt.Println("年龄:")
fmt.Scanln(&this.age)
fmt.Println("电话:")
fmt.Scanln(&this.phone)
fmt.Println("邮箱号:")
fmt.Scanln(&this.email)
costomer := model.NewCustomerNotID(this.name,this.gender,this.age,this.phone,this.email)
if this.customerService.Update(this.id,costomer){
fmt.Println("----------修改成功----------")
return
}else {
fmt.Println("----------修改失败,id不存在----------")
return
}
}
//退出客户系统
func (this *CustomerView) Exit() {
for {
fmt.Println("是否确认退出系统(y/n):")
fmt.Scanln(&this.choice)
if this.choice == "y" || this.choice == "Y" {
fmt.Println("您退出客户信息管理软件")
this.loop = false
return
} else if this.choice == "n" || this.choice == "N" {
break
} else {
fmt.Println("请输入正确的选项")
}
}
}
//显示主菜单
func (this *CustomerView) mainMenu() {
for {
fmt.Println("----------客户信息管理软件----------")
fmt.Println( "1 添加客户")
fmt.Println( "2 修改客户")
fmt.Println( "3 删除客户")
fmt.Println( "4 客户列表")
fmt.Println( "5 退 出")
fmt.Print("请选择(1~5):")
fmt.Scanln(&this.key)
switch this.key {
case "1":
this.Add()
case "2":
this.Update()
case "3":
this.Delete()
case "4":
this.List()
case "5":
this.Exit()
default:
fmt.Println("请输入正确的选项")
}
if this.loop == false {
return
}
}
}
func main() {
view := CustomerView{
key:"",
loop:true,
name:"",
gender:"",
age:0,
phone:"",
id:-1,
choice:"",
}
//对customerService初始化
view.customerService = service.NewCustomerService()
//显示主菜单
view.mainMenu()
}
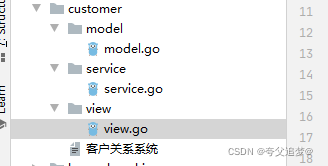
?
|